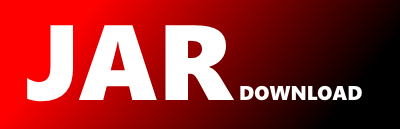
org.yamcs.protobuf.Yamcs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: yamcs/protobuf/yamcs.proto
package org.yamcs.protobuf;
public final class Yamcs {
private Yamcs() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code yamcs.protobuf.EndAction}
*/
public enum EndAction
implements com.google.protobuf.ProtocolMessageEnum {
/**
* LOOP = 1;
*/
LOOP(1),
/**
* QUIT = 2;
*/
QUIT(2),
/**
* STOP = 3;
*/
STOP(3),
;
/**
* LOOP = 1;
*/
public static final int LOOP_VALUE = 1;
/**
* QUIT = 2;
*/
public static final int QUIT_VALUE = 2;
/**
* STOP = 3;
*/
public static final int STOP_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static EndAction valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static EndAction forNumber(int value) {
switch (value) {
case 1: return LOOP;
case 2: return QUIT;
case 3: return STOP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
EndAction> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public EndAction findValueByNumber(int number) {
return EndAction.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.getDescriptor().getEnumTypes().get(0);
}
private static final EndAction[] VALUES = values();
public static EndAction valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private EndAction(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:yamcs.protobuf.EndAction)
}
public interface ValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.Value)
com.google.protobuf.MessageOrBuilder {
/**
* required .yamcs.protobuf.Value.Type type = 1;
* @return Whether the type field is set.
*/
boolean hasType();
/**
* required .yamcs.protobuf.Value.Type type = 1;
* @return The type.
*/
org.yamcs.protobuf.Yamcs.Value.Type getType();
/**
* optional float floatValue = 2;
* @return Whether the floatValue field is set.
*/
boolean hasFloatValue();
/**
* optional float floatValue = 2;
* @return The floatValue.
*/
float getFloatValue();
/**
* optional double doubleValue = 3;
* @return Whether the doubleValue field is set.
*/
boolean hasDoubleValue();
/**
* optional double doubleValue = 3;
* @return The doubleValue.
*/
double getDoubleValue();
/**
* optional sint32 sint32Value = 4;
* @return Whether the sint32Value field is set.
*/
boolean hasSint32Value();
/**
* optional sint32 sint32Value = 4;
* @return The sint32Value.
*/
int getSint32Value();
/**
* optional uint32 uint32Value = 5;
* @return Whether the uint32Value field is set.
*/
boolean hasUint32Value();
/**
* optional uint32 uint32Value = 5;
* @return The uint32Value.
*/
int getUint32Value();
/**
* optional bytes binaryValue = 6;
* @return Whether the binaryValue field is set.
*/
boolean hasBinaryValue();
/**
* optional bytes binaryValue = 6;
* @return The binaryValue.
*/
com.google.protobuf.ByteString getBinaryValue();
/**
* optional string stringValue = 7;
* @return Whether the stringValue field is set.
*/
boolean hasStringValue();
/**
* optional string stringValue = 7;
* @return The stringValue.
*/
java.lang.String getStringValue();
/**
* optional string stringValue = 7;
* @return The bytes for stringValue.
*/
com.google.protobuf.ByteString
getStringValueBytes();
/**
* optional int64 timestampValue = 8;
* @return Whether the timestampValue field is set.
*/
boolean hasTimestampValue();
/**
* optional int64 timestampValue = 8;
* @return The timestampValue.
*/
long getTimestampValue();
/**
* optional uint64 uint64Value = 9;
* @return Whether the uint64Value field is set.
*/
boolean hasUint64Value();
/**
* optional uint64 uint64Value = 9;
* @return The uint64Value.
*/
long getUint64Value();
/**
* optional sint64 sint64Value = 10;
* @return Whether the sint64Value field is set.
*/
boolean hasSint64Value();
/**
* optional sint64 sint64Value = 10;
* @return The sint64Value.
*/
long getSint64Value();
/**
* optional bool booleanValue = 11;
* @return Whether the booleanValue field is set.
*/
boolean hasBooleanValue();
/**
* optional bool booleanValue = 11;
* @return The booleanValue.
*/
boolean getBooleanValue();
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
* @return Whether the aggregateValue field is set.
*/
boolean hasAggregateValue();
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
* @return The aggregateValue.
*/
org.yamcs.protobuf.Yamcs.AggregateValue getAggregateValue();
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
*/
org.yamcs.protobuf.Yamcs.AggregateValueOrBuilder getAggregateValueOrBuilder();
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
java.util.List
getArrayValueList();
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
org.yamcs.protobuf.Yamcs.Value getArrayValue(int index);
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
int getArrayValueCount();
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
java.util.List extends org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getArrayValueOrBuilderList();
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
org.yamcs.protobuf.Yamcs.ValueOrBuilder getArrayValueOrBuilder(
int index);
}
/**
*
* Union type for storing a value
*
*
* Protobuf type {@code yamcs.protobuf.Value}
*/
public static final class Value extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.Value)
ValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use Value.newBuilder() to construct.
private Value(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Value() {
type_ = 0;
binaryValue_ = com.google.protobuf.ByteString.EMPTY;
stringValue_ = "";
arrayValue_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Value();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Value(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.Value.Type value = org.yamcs.protobuf.Yamcs.Value.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = rawValue;
}
break;
}
case 21: {
bitField0_ |= 0x00000002;
floatValue_ = input.readFloat();
break;
}
case 25: {
bitField0_ |= 0x00000004;
doubleValue_ = input.readDouble();
break;
}
case 32: {
bitField0_ |= 0x00000008;
sint32Value_ = input.readSInt32();
break;
}
case 40: {
bitField0_ |= 0x00000010;
uint32Value_ = input.readUInt32();
break;
}
case 50: {
bitField0_ |= 0x00000020;
binaryValue_ = input.readBytes();
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
stringValue_ = bs;
break;
}
case 64: {
bitField0_ |= 0x00000080;
timestampValue_ = input.readInt64();
break;
}
case 72: {
bitField0_ |= 0x00000100;
uint64Value_ = input.readUInt64();
break;
}
case 80: {
bitField0_ |= 0x00000200;
sint64Value_ = input.readSInt64();
break;
}
case 88: {
bitField0_ |= 0x00000400;
booleanValue_ = input.readBool();
break;
}
case 98: {
org.yamcs.protobuf.Yamcs.AggregateValue.Builder subBuilder = null;
if (((bitField0_ & 0x00000800) != 0)) {
subBuilder = aggregateValue_.toBuilder();
}
aggregateValue_ = input.readMessage(org.yamcs.protobuf.Yamcs.AggregateValue.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(aggregateValue_);
aggregateValue_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000800;
break;
}
case 106: {
if (!((mutable_bitField0_ & 0x00001000) != 0)) {
arrayValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00001000;
}
arrayValue_.add(
input.readMessage(org.yamcs.protobuf.Yamcs.Value.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00001000) != 0)) {
arrayValue_ = java.util.Collections.unmodifiableList(arrayValue_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_Value_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_Value_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.Value.class, org.yamcs.protobuf.Yamcs.Value.Builder.class);
}
/**
* Protobuf enum {@code yamcs.protobuf.Value.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* FLOAT = 0;
*/
FLOAT(0),
/**
* DOUBLE = 1;
*/
DOUBLE(1),
/**
* UINT32 = 2;
*/
UINT32(2),
/**
* SINT32 = 3;
*/
SINT32(3),
/**
* BINARY = 4;
*/
BINARY(4),
/**
* STRING = 5;
*/
STRING(5),
/**
* TIMESTAMP = 6;
*/
TIMESTAMP(6),
/**
* UINT64 = 7;
*/
UINT64(7),
/**
* SINT64 = 8;
*/
SINT64(8),
/**
* BOOLEAN = 9;
*/
BOOLEAN(9),
/**
* AGGREGATE = 10;
*/
AGGREGATE(10),
/**
* ARRAY = 11;
*/
ARRAY(11),
/**
*
* Enumerated values have both an integer (sint64Value) and a string representation
*
*
* ENUMERATED = 12;
*/
ENUMERATED(12),
/**
* NONE = 13;
*/
NONE(13),
;
/**
* FLOAT = 0;
*/
public static final int FLOAT_VALUE = 0;
/**
* DOUBLE = 1;
*/
public static final int DOUBLE_VALUE = 1;
/**
* UINT32 = 2;
*/
public static final int UINT32_VALUE = 2;
/**
* SINT32 = 3;
*/
public static final int SINT32_VALUE = 3;
/**
* BINARY = 4;
*/
public static final int BINARY_VALUE = 4;
/**
* STRING = 5;
*/
public static final int STRING_VALUE = 5;
/**
* TIMESTAMP = 6;
*/
public static final int TIMESTAMP_VALUE = 6;
/**
* UINT64 = 7;
*/
public static final int UINT64_VALUE = 7;
/**
* SINT64 = 8;
*/
public static final int SINT64_VALUE = 8;
/**
* BOOLEAN = 9;
*/
public static final int BOOLEAN_VALUE = 9;
/**
* AGGREGATE = 10;
*/
public static final int AGGREGATE_VALUE = 10;
/**
* ARRAY = 11;
*/
public static final int ARRAY_VALUE = 11;
/**
*
* Enumerated values have both an integer (sint64Value) and a string representation
*
*
* ENUMERATED = 12;
*/
public static final int ENUMERATED_VALUE = 12;
/**
* NONE = 13;
*/
public static final int NONE_VALUE = 13;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Type forNumber(int value) {
switch (value) {
case 0: return FLOAT;
case 1: return DOUBLE;
case 2: return UINT32;
case 3: return SINT32;
case 4: return BINARY;
case 5: return STRING;
case 6: return TIMESTAMP;
case 7: return UINT64;
case 8: return SINT64;
case 9: return BOOLEAN;
case 10: return AGGREGATE;
case 11: return ARRAY;
case 12: return ENUMERATED;
case 13: return NONE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.Value.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:yamcs.protobuf.Value.Type)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* required .yamcs.protobuf.Value.Type type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required .yamcs.protobuf.Value.Type type = 1;
* @return The type.
*/
@java.lang.Override public org.yamcs.protobuf.Yamcs.Value.Type getType() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.Value.Type result = org.yamcs.protobuf.Yamcs.Value.Type.valueOf(type_);
return result == null ? org.yamcs.protobuf.Yamcs.Value.Type.FLOAT : result;
}
public static final int FLOATVALUE_FIELD_NUMBER = 2;
private float floatValue_;
/**
* optional float floatValue = 2;
* @return Whether the floatValue field is set.
*/
@java.lang.Override
public boolean hasFloatValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional float floatValue = 2;
* @return The floatValue.
*/
@java.lang.Override
public float getFloatValue() {
return floatValue_;
}
public static final int DOUBLEVALUE_FIELD_NUMBER = 3;
private double doubleValue_;
/**
* optional double doubleValue = 3;
* @return Whether the doubleValue field is set.
*/
@java.lang.Override
public boolean hasDoubleValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional double doubleValue = 3;
* @return The doubleValue.
*/
@java.lang.Override
public double getDoubleValue() {
return doubleValue_;
}
public static final int SINT32VALUE_FIELD_NUMBER = 4;
private int sint32Value_;
/**
* optional sint32 sint32Value = 4;
* @return Whether the sint32Value field is set.
*/
@java.lang.Override
public boolean hasSint32Value() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional sint32 sint32Value = 4;
* @return The sint32Value.
*/
@java.lang.Override
public int getSint32Value() {
return sint32Value_;
}
public static final int UINT32VALUE_FIELD_NUMBER = 5;
private int uint32Value_;
/**
* optional uint32 uint32Value = 5;
* @return Whether the uint32Value field is set.
*/
@java.lang.Override
public boolean hasUint32Value() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional uint32 uint32Value = 5;
* @return The uint32Value.
*/
@java.lang.Override
public int getUint32Value() {
return uint32Value_;
}
public static final int BINARYVALUE_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString binaryValue_;
/**
* optional bytes binaryValue = 6;
* @return Whether the binaryValue field is set.
*/
@java.lang.Override
public boolean hasBinaryValue() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional bytes binaryValue = 6;
* @return The binaryValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBinaryValue() {
return binaryValue_;
}
public static final int STRINGVALUE_FIELD_NUMBER = 7;
private volatile java.lang.Object stringValue_;
/**
* optional string stringValue = 7;
* @return Whether the stringValue field is set.
*/
@java.lang.Override
public boolean hasStringValue() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional string stringValue = 7;
* @return The stringValue.
*/
@java.lang.Override
public java.lang.String getStringValue() {
java.lang.Object ref = stringValue_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stringValue_ = s;
}
return s;
}
}
/**
* optional string stringValue = 7;
* @return The bytes for stringValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = stringValue_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stringValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMESTAMPVALUE_FIELD_NUMBER = 8;
private long timestampValue_;
/**
* optional int64 timestampValue = 8;
* @return Whether the timestampValue field is set.
*/
@java.lang.Override
public boolean hasTimestampValue() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional int64 timestampValue = 8;
* @return The timestampValue.
*/
@java.lang.Override
public long getTimestampValue() {
return timestampValue_;
}
public static final int UINT64VALUE_FIELD_NUMBER = 9;
private long uint64Value_;
/**
* optional uint64 uint64Value = 9;
* @return Whether the uint64Value field is set.
*/
@java.lang.Override
public boolean hasUint64Value() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional uint64 uint64Value = 9;
* @return The uint64Value.
*/
@java.lang.Override
public long getUint64Value() {
return uint64Value_;
}
public static final int SINT64VALUE_FIELD_NUMBER = 10;
private long sint64Value_;
/**
* optional sint64 sint64Value = 10;
* @return Whether the sint64Value field is set.
*/
@java.lang.Override
public boolean hasSint64Value() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional sint64 sint64Value = 10;
* @return The sint64Value.
*/
@java.lang.Override
public long getSint64Value() {
return sint64Value_;
}
public static final int BOOLEANVALUE_FIELD_NUMBER = 11;
private boolean booleanValue_;
/**
* optional bool booleanValue = 11;
* @return Whether the booleanValue field is set.
*/
@java.lang.Override
public boolean hasBooleanValue() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional bool booleanValue = 11;
* @return The booleanValue.
*/
@java.lang.Override
public boolean getBooleanValue() {
return booleanValue_;
}
public static final int AGGREGATEVALUE_FIELD_NUMBER = 12;
private org.yamcs.protobuf.Yamcs.AggregateValue aggregateValue_;
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
* @return Whether the aggregateValue field is set.
*/
@java.lang.Override
public boolean hasAggregateValue() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
* @return The aggregateValue.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.AggregateValue getAggregateValue() {
return aggregateValue_ == null ? org.yamcs.protobuf.Yamcs.AggregateValue.getDefaultInstance() : aggregateValue_;
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.AggregateValueOrBuilder getAggregateValueOrBuilder() {
return aggregateValue_ == null ? org.yamcs.protobuf.Yamcs.AggregateValue.getDefaultInstance() : aggregateValue_;
}
public static final int ARRAYVALUE_FIELD_NUMBER = 13;
private java.util.List arrayValue_;
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
@java.lang.Override
public java.util.List getArrayValueList() {
return arrayValue_;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getArrayValueOrBuilderList() {
return arrayValue_;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
@java.lang.Override
public int getArrayValueCount() {
return arrayValue_.size();
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value getArrayValue(int index) {
return arrayValue_.get(index);
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getArrayValueOrBuilder(
int index) {
return arrayValue_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (hasAggregateValue()) {
if (!getAggregateValue().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getArrayValueCount(); i++) {
if (!getArrayValue(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeFloat(2, floatValue_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeDouble(3, doubleValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeSInt32(4, sint32Value_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeUInt32(5, uint32Value_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeBytes(6, binaryValue_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, stringValue_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeInt64(8, timestampValue_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeUInt64(9, uint64Value_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeSInt64(10, sint64Value_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeBool(11, booleanValue_);
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeMessage(12, getAggregateValue());
}
for (int i = 0; i < arrayValue_.size(); i++) {
output.writeMessage(13, arrayValue_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(2, floatValue_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, doubleValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(4, sint32Value_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, uint32Value_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, binaryValue_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, stringValue_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, timestampValue_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(9, uint64Value_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(10, sint64Value_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, booleanValue_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getAggregateValue());
}
for (int i = 0; i < arrayValue_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, arrayValue_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.Value)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.Value other = (org.yamcs.protobuf.Yamcs.Value) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (hasFloatValue() != other.hasFloatValue()) return false;
if (hasFloatValue()) {
if (java.lang.Float.floatToIntBits(getFloatValue())
!= java.lang.Float.floatToIntBits(
other.getFloatValue())) return false;
}
if (hasDoubleValue() != other.hasDoubleValue()) return false;
if (hasDoubleValue()) {
if (java.lang.Double.doubleToLongBits(getDoubleValue())
!= java.lang.Double.doubleToLongBits(
other.getDoubleValue())) return false;
}
if (hasSint32Value() != other.hasSint32Value()) return false;
if (hasSint32Value()) {
if (getSint32Value()
!= other.getSint32Value()) return false;
}
if (hasUint32Value() != other.hasUint32Value()) return false;
if (hasUint32Value()) {
if (getUint32Value()
!= other.getUint32Value()) return false;
}
if (hasBinaryValue() != other.hasBinaryValue()) return false;
if (hasBinaryValue()) {
if (!getBinaryValue()
.equals(other.getBinaryValue())) return false;
}
if (hasStringValue() != other.hasStringValue()) return false;
if (hasStringValue()) {
if (!getStringValue()
.equals(other.getStringValue())) return false;
}
if (hasTimestampValue() != other.hasTimestampValue()) return false;
if (hasTimestampValue()) {
if (getTimestampValue()
!= other.getTimestampValue()) return false;
}
if (hasUint64Value() != other.hasUint64Value()) return false;
if (hasUint64Value()) {
if (getUint64Value()
!= other.getUint64Value()) return false;
}
if (hasSint64Value() != other.hasSint64Value()) return false;
if (hasSint64Value()) {
if (getSint64Value()
!= other.getSint64Value()) return false;
}
if (hasBooleanValue() != other.hasBooleanValue()) return false;
if (hasBooleanValue()) {
if (getBooleanValue()
!= other.getBooleanValue()) return false;
}
if (hasAggregateValue() != other.hasAggregateValue()) return false;
if (hasAggregateValue()) {
if (!getAggregateValue()
.equals(other.getAggregateValue())) return false;
}
if (!getArrayValueList()
.equals(other.getArrayValueList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasFloatValue()) {
hash = (37 * hash) + FLOATVALUE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getFloatValue());
}
if (hasDoubleValue()) {
hash = (37 * hash) + DOUBLEVALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getDoubleValue()));
}
if (hasSint32Value()) {
hash = (37 * hash) + SINT32VALUE_FIELD_NUMBER;
hash = (53 * hash) + getSint32Value();
}
if (hasUint32Value()) {
hash = (37 * hash) + UINT32VALUE_FIELD_NUMBER;
hash = (53 * hash) + getUint32Value();
}
if (hasBinaryValue()) {
hash = (37 * hash) + BINARYVALUE_FIELD_NUMBER;
hash = (53 * hash) + getBinaryValue().hashCode();
}
if (hasStringValue()) {
hash = (37 * hash) + STRINGVALUE_FIELD_NUMBER;
hash = (53 * hash) + getStringValue().hashCode();
}
if (hasTimestampValue()) {
hash = (37 * hash) + TIMESTAMPVALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestampValue());
}
if (hasUint64Value()) {
hash = (37 * hash) + UINT64VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUint64Value());
}
if (hasSint64Value()) {
hash = (37 * hash) + SINT64VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSint64Value());
}
if (hasBooleanValue()) {
hash = (37 * hash) + BOOLEANVALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getBooleanValue());
}
if (hasAggregateValue()) {
hash = (37 * hash) + AGGREGATEVALUE_FIELD_NUMBER;
hash = (53 * hash) + getAggregateValue().hashCode();
}
if (getArrayValueCount() > 0) {
hash = (37 * hash) + ARRAYVALUE_FIELD_NUMBER;
hash = (53 * hash) + getArrayValueList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.Value parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.Value parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.Value parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.Value prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Union type for storing a value
*
*
* Protobuf type {@code yamcs.protobuf.Value}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.Value)
org.yamcs.protobuf.Yamcs.ValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_Value_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_Value_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.Value.class, org.yamcs.protobuf.Yamcs.Value.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.Value.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAggregateValueFieldBuilder();
getArrayValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
floatValue_ = 0F;
bitField0_ = (bitField0_ & ~0x00000002);
doubleValue_ = 0D;
bitField0_ = (bitField0_ & ~0x00000004);
sint32Value_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
uint32Value_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
binaryValue_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
stringValue_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
timestampValue_ = 0L;
bitField0_ = (bitField0_ & ~0x00000080);
uint64Value_ = 0L;
bitField0_ = (bitField0_ & ~0x00000100);
sint64Value_ = 0L;
bitField0_ = (bitField0_ & ~0x00000200);
booleanValue_ = false;
bitField0_ = (bitField0_ & ~0x00000400);
if (aggregateValueBuilder_ == null) {
aggregateValue_ = null;
} else {
aggregateValueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
if (arrayValueBuilder_ == null) {
arrayValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00001000);
} else {
arrayValueBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_Value_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.Value.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value build() {
org.yamcs.protobuf.Yamcs.Value result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value buildPartial() {
org.yamcs.protobuf.Yamcs.Value result = new org.yamcs.protobuf.Yamcs.Value(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.floatValue_ = floatValue_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.doubleValue_ = doubleValue_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.sint32Value_ = sint32Value_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.uint32Value_ = uint32Value_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.binaryValue_ = binaryValue_;
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.stringValue_ = stringValue_;
if (((from_bitField0_ & 0x00000080) != 0)) {
result.timestampValue_ = timestampValue_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.uint64Value_ = uint64Value_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.sint64Value_ = sint64Value_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.booleanValue_ = booleanValue_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
if (aggregateValueBuilder_ == null) {
result.aggregateValue_ = aggregateValue_;
} else {
result.aggregateValue_ = aggregateValueBuilder_.build();
}
to_bitField0_ |= 0x00000800;
}
if (arrayValueBuilder_ == null) {
if (((bitField0_ & 0x00001000) != 0)) {
arrayValue_ = java.util.Collections.unmodifiableList(arrayValue_);
bitField0_ = (bitField0_ & ~0x00001000);
}
result.arrayValue_ = arrayValue_;
} else {
result.arrayValue_ = arrayValueBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.Value) {
return mergeFrom((org.yamcs.protobuf.Yamcs.Value)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.Value other) {
if (other == org.yamcs.protobuf.Yamcs.Value.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasFloatValue()) {
setFloatValue(other.getFloatValue());
}
if (other.hasDoubleValue()) {
setDoubleValue(other.getDoubleValue());
}
if (other.hasSint32Value()) {
setSint32Value(other.getSint32Value());
}
if (other.hasUint32Value()) {
setUint32Value(other.getUint32Value());
}
if (other.hasBinaryValue()) {
setBinaryValue(other.getBinaryValue());
}
if (other.hasStringValue()) {
bitField0_ |= 0x00000040;
stringValue_ = other.stringValue_;
onChanged();
}
if (other.hasTimestampValue()) {
setTimestampValue(other.getTimestampValue());
}
if (other.hasUint64Value()) {
setUint64Value(other.getUint64Value());
}
if (other.hasSint64Value()) {
setSint64Value(other.getSint64Value());
}
if (other.hasBooleanValue()) {
setBooleanValue(other.getBooleanValue());
}
if (other.hasAggregateValue()) {
mergeAggregateValue(other.getAggregateValue());
}
if (arrayValueBuilder_ == null) {
if (!other.arrayValue_.isEmpty()) {
if (arrayValue_.isEmpty()) {
arrayValue_ = other.arrayValue_;
bitField0_ = (bitField0_ & ~0x00001000);
} else {
ensureArrayValueIsMutable();
arrayValue_.addAll(other.arrayValue_);
}
onChanged();
}
} else {
if (!other.arrayValue_.isEmpty()) {
if (arrayValueBuilder_.isEmpty()) {
arrayValueBuilder_.dispose();
arrayValueBuilder_ = null;
arrayValue_ = other.arrayValue_;
bitField0_ = (bitField0_ & ~0x00001000);
arrayValueBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getArrayValueFieldBuilder() : null;
} else {
arrayValueBuilder_.addAllMessages(other.arrayValue_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasType()) {
return false;
}
if (hasAggregateValue()) {
if (!getAggregateValue().isInitialized()) {
return false;
}
}
for (int i = 0; i < getArrayValueCount(); i++) {
if (!getArrayValue(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.Value parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.Value) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 0;
/**
* required .yamcs.protobuf.Value.Type type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required .yamcs.protobuf.Value.Type type = 1;
* @return The type.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value.Type getType() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.Value.Type result = org.yamcs.protobuf.Yamcs.Value.Type.valueOf(type_);
return result == null ? org.yamcs.protobuf.Yamcs.Value.Type.FLOAT : result;
}
/**
* required .yamcs.protobuf.Value.Type type = 1;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.yamcs.protobuf.Yamcs.Value.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* required .yamcs.protobuf.Value.Type type = 1;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
onChanged();
return this;
}
private float floatValue_ ;
/**
* optional float floatValue = 2;
* @return Whether the floatValue field is set.
*/
@java.lang.Override
public boolean hasFloatValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional float floatValue = 2;
* @return The floatValue.
*/
@java.lang.Override
public float getFloatValue() {
return floatValue_;
}
/**
* optional float floatValue = 2;
* @param value The floatValue to set.
* @return This builder for chaining.
*/
public Builder setFloatValue(float value) {
bitField0_ |= 0x00000002;
floatValue_ = value;
onChanged();
return this;
}
/**
* optional float floatValue = 2;
* @return This builder for chaining.
*/
public Builder clearFloatValue() {
bitField0_ = (bitField0_ & ~0x00000002);
floatValue_ = 0F;
onChanged();
return this;
}
private double doubleValue_ ;
/**
* optional double doubleValue = 3;
* @return Whether the doubleValue field is set.
*/
@java.lang.Override
public boolean hasDoubleValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional double doubleValue = 3;
* @return The doubleValue.
*/
@java.lang.Override
public double getDoubleValue() {
return doubleValue_;
}
/**
* optional double doubleValue = 3;
* @param value The doubleValue to set.
* @return This builder for chaining.
*/
public Builder setDoubleValue(double value) {
bitField0_ |= 0x00000004;
doubleValue_ = value;
onChanged();
return this;
}
/**
* optional double doubleValue = 3;
* @return This builder for chaining.
*/
public Builder clearDoubleValue() {
bitField0_ = (bitField0_ & ~0x00000004);
doubleValue_ = 0D;
onChanged();
return this;
}
private int sint32Value_ ;
/**
* optional sint32 sint32Value = 4;
* @return Whether the sint32Value field is set.
*/
@java.lang.Override
public boolean hasSint32Value() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional sint32 sint32Value = 4;
* @return The sint32Value.
*/
@java.lang.Override
public int getSint32Value() {
return sint32Value_;
}
/**
* optional sint32 sint32Value = 4;
* @param value The sint32Value to set.
* @return This builder for chaining.
*/
public Builder setSint32Value(int value) {
bitField0_ |= 0x00000008;
sint32Value_ = value;
onChanged();
return this;
}
/**
* optional sint32 sint32Value = 4;
* @return This builder for chaining.
*/
public Builder clearSint32Value() {
bitField0_ = (bitField0_ & ~0x00000008);
sint32Value_ = 0;
onChanged();
return this;
}
private int uint32Value_ ;
/**
* optional uint32 uint32Value = 5;
* @return Whether the uint32Value field is set.
*/
@java.lang.Override
public boolean hasUint32Value() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional uint32 uint32Value = 5;
* @return The uint32Value.
*/
@java.lang.Override
public int getUint32Value() {
return uint32Value_;
}
/**
* optional uint32 uint32Value = 5;
* @param value The uint32Value to set.
* @return This builder for chaining.
*/
public Builder setUint32Value(int value) {
bitField0_ |= 0x00000010;
uint32Value_ = value;
onChanged();
return this;
}
/**
* optional uint32 uint32Value = 5;
* @return This builder for chaining.
*/
public Builder clearUint32Value() {
bitField0_ = (bitField0_ & ~0x00000010);
uint32Value_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString binaryValue_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes binaryValue = 6;
* @return Whether the binaryValue field is set.
*/
@java.lang.Override
public boolean hasBinaryValue() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional bytes binaryValue = 6;
* @return The binaryValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBinaryValue() {
return binaryValue_;
}
/**
* optional bytes binaryValue = 6;
* @param value The binaryValue to set.
* @return This builder for chaining.
*/
public Builder setBinaryValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
binaryValue_ = value;
onChanged();
return this;
}
/**
* optional bytes binaryValue = 6;
* @return This builder for chaining.
*/
public Builder clearBinaryValue() {
bitField0_ = (bitField0_ & ~0x00000020);
binaryValue_ = getDefaultInstance().getBinaryValue();
onChanged();
return this;
}
private java.lang.Object stringValue_ = "";
/**
* optional string stringValue = 7;
* @return Whether the stringValue field is set.
*/
public boolean hasStringValue() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional string stringValue = 7;
* @return The stringValue.
*/
public java.lang.String getStringValue() {
java.lang.Object ref = stringValue_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stringValue_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string stringValue = 7;
* @return The bytes for stringValue.
*/
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = stringValue_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stringValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string stringValue = 7;
* @param value The stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
stringValue_ = value;
onChanged();
return this;
}
/**
* optional string stringValue = 7;
* @return This builder for chaining.
*/
public Builder clearStringValue() {
bitField0_ = (bitField0_ & ~0x00000040);
stringValue_ = getDefaultInstance().getStringValue();
onChanged();
return this;
}
/**
* optional string stringValue = 7;
* @param value The bytes for stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
stringValue_ = value;
onChanged();
return this;
}
private long timestampValue_ ;
/**
* optional int64 timestampValue = 8;
* @return Whether the timestampValue field is set.
*/
@java.lang.Override
public boolean hasTimestampValue() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional int64 timestampValue = 8;
* @return The timestampValue.
*/
@java.lang.Override
public long getTimestampValue() {
return timestampValue_;
}
/**
* optional int64 timestampValue = 8;
* @param value The timestampValue to set.
* @return This builder for chaining.
*/
public Builder setTimestampValue(long value) {
bitField0_ |= 0x00000080;
timestampValue_ = value;
onChanged();
return this;
}
/**
* optional int64 timestampValue = 8;
* @return This builder for chaining.
*/
public Builder clearTimestampValue() {
bitField0_ = (bitField0_ & ~0x00000080);
timestampValue_ = 0L;
onChanged();
return this;
}
private long uint64Value_ ;
/**
* optional uint64 uint64Value = 9;
* @return Whether the uint64Value field is set.
*/
@java.lang.Override
public boolean hasUint64Value() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional uint64 uint64Value = 9;
* @return The uint64Value.
*/
@java.lang.Override
public long getUint64Value() {
return uint64Value_;
}
/**
* optional uint64 uint64Value = 9;
* @param value The uint64Value to set.
* @return This builder for chaining.
*/
public Builder setUint64Value(long value) {
bitField0_ |= 0x00000100;
uint64Value_ = value;
onChanged();
return this;
}
/**
* optional uint64 uint64Value = 9;
* @return This builder for chaining.
*/
public Builder clearUint64Value() {
bitField0_ = (bitField0_ & ~0x00000100);
uint64Value_ = 0L;
onChanged();
return this;
}
private long sint64Value_ ;
/**
* optional sint64 sint64Value = 10;
* @return Whether the sint64Value field is set.
*/
@java.lang.Override
public boolean hasSint64Value() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional sint64 sint64Value = 10;
* @return The sint64Value.
*/
@java.lang.Override
public long getSint64Value() {
return sint64Value_;
}
/**
* optional sint64 sint64Value = 10;
* @param value The sint64Value to set.
* @return This builder for chaining.
*/
public Builder setSint64Value(long value) {
bitField0_ |= 0x00000200;
sint64Value_ = value;
onChanged();
return this;
}
/**
* optional sint64 sint64Value = 10;
* @return This builder for chaining.
*/
public Builder clearSint64Value() {
bitField0_ = (bitField0_ & ~0x00000200);
sint64Value_ = 0L;
onChanged();
return this;
}
private boolean booleanValue_ ;
/**
* optional bool booleanValue = 11;
* @return Whether the booleanValue field is set.
*/
@java.lang.Override
public boolean hasBooleanValue() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional bool booleanValue = 11;
* @return The booleanValue.
*/
@java.lang.Override
public boolean getBooleanValue() {
return booleanValue_;
}
/**
* optional bool booleanValue = 11;
* @param value The booleanValue to set.
* @return This builder for chaining.
*/
public Builder setBooleanValue(boolean value) {
bitField0_ |= 0x00000400;
booleanValue_ = value;
onChanged();
return this;
}
/**
* optional bool booleanValue = 11;
* @return This builder for chaining.
*/
public Builder clearBooleanValue() {
bitField0_ = (bitField0_ & ~0x00000400);
booleanValue_ = false;
onChanged();
return this;
}
private org.yamcs.protobuf.Yamcs.AggregateValue aggregateValue_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.AggregateValue, org.yamcs.protobuf.Yamcs.AggregateValue.Builder, org.yamcs.protobuf.Yamcs.AggregateValueOrBuilder> aggregateValueBuilder_;
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
* @return Whether the aggregateValue field is set.
*/
public boolean hasAggregateValue() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
* @return The aggregateValue.
*/
public org.yamcs.protobuf.Yamcs.AggregateValue getAggregateValue() {
if (aggregateValueBuilder_ == null) {
return aggregateValue_ == null ? org.yamcs.protobuf.Yamcs.AggregateValue.getDefaultInstance() : aggregateValue_;
} else {
return aggregateValueBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
*/
public Builder setAggregateValue(org.yamcs.protobuf.Yamcs.AggregateValue value) {
if (aggregateValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
aggregateValue_ = value;
onChanged();
} else {
aggregateValueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
*/
public Builder setAggregateValue(
org.yamcs.protobuf.Yamcs.AggregateValue.Builder builderForValue) {
if (aggregateValueBuilder_ == null) {
aggregateValue_ = builderForValue.build();
onChanged();
} else {
aggregateValueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
*/
public Builder mergeAggregateValue(org.yamcs.protobuf.Yamcs.AggregateValue value) {
if (aggregateValueBuilder_ == null) {
if (((bitField0_ & 0x00000800) != 0) &&
aggregateValue_ != null &&
aggregateValue_ != org.yamcs.protobuf.Yamcs.AggregateValue.getDefaultInstance()) {
aggregateValue_ =
org.yamcs.protobuf.Yamcs.AggregateValue.newBuilder(aggregateValue_).mergeFrom(value).buildPartial();
} else {
aggregateValue_ = value;
}
onChanged();
} else {
aggregateValueBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
*/
public Builder clearAggregateValue() {
if (aggregateValueBuilder_ == null) {
aggregateValue_ = null;
onChanged();
} else {
aggregateValueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
*/
public org.yamcs.protobuf.Yamcs.AggregateValue.Builder getAggregateValueBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getAggregateValueFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
*/
public org.yamcs.protobuf.Yamcs.AggregateValueOrBuilder getAggregateValueOrBuilder() {
if (aggregateValueBuilder_ != null) {
return aggregateValueBuilder_.getMessageOrBuilder();
} else {
return aggregateValue_ == null ?
org.yamcs.protobuf.Yamcs.AggregateValue.getDefaultInstance() : aggregateValue_;
}
}
/**
* optional .yamcs.protobuf.AggregateValue aggregateValue = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.AggregateValue, org.yamcs.protobuf.Yamcs.AggregateValue.Builder, org.yamcs.protobuf.Yamcs.AggregateValueOrBuilder>
getAggregateValueFieldBuilder() {
if (aggregateValueBuilder_ == null) {
aggregateValueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.AggregateValue, org.yamcs.protobuf.Yamcs.AggregateValue.Builder, org.yamcs.protobuf.Yamcs.AggregateValueOrBuilder>(
getAggregateValue(),
getParentForChildren(),
isClean());
aggregateValue_ = null;
}
return aggregateValueBuilder_;
}
private java.util.List arrayValue_ =
java.util.Collections.emptyList();
private void ensureArrayValueIsMutable() {
if (!((bitField0_ & 0x00001000) != 0)) {
arrayValue_ = new java.util.ArrayList(arrayValue_);
bitField0_ |= 0x00001000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder> arrayValueBuilder_;
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public java.util.List getArrayValueList() {
if (arrayValueBuilder_ == null) {
return java.util.Collections.unmodifiableList(arrayValue_);
} else {
return arrayValueBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public int getArrayValueCount() {
if (arrayValueBuilder_ == null) {
return arrayValue_.size();
} else {
return arrayValueBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public org.yamcs.protobuf.Yamcs.Value getArrayValue(int index) {
if (arrayValueBuilder_ == null) {
return arrayValue_.get(index);
} else {
return arrayValueBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public Builder setArrayValue(
int index, org.yamcs.protobuf.Yamcs.Value value) {
if (arrayValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArrayValueIsMutable();
arrayValue_.set(index, value);
onChanged();
} else {
arrayValueBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public Builder setArrayValue(
int index, org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (arrayValueBuilder_ == null) {
ensureArrayValueIsMutable();
arrayValue_.set(index, builderForValue.build());
onChanged();
} else {
arrayValueBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public Builder addArrayValue(org.yamcs.protobuf.Yamcs.Value value) {
if (arrayValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArrayValueIsMutable();
arrayValue_.add(value);
onChanged();
} else {
arrayValueBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public Builder addArrayValue(
int index, org.yamcs.protobuf.Yamcs.Value value) {
if (arrayValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArrayValueIsMutable();
arrayValue_.add(index, value);
onChanged();
} else {
arrayValueBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public Builder addArrayValue(
org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (arrayValueBuilder_ == null) {
ensureArrayValueIsMutable();
arrayValue_.add(builderForValue.build());
onChanged();
} else {
arrayValueBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public Builder addArrayValue(
int index, org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (arrayValueBuilder_ == null) {
ensureArrayValueIsMutable();
arrayValue_.add(index, builderForValue.build());
onChanged();
} else {
arrayValueBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public Builder addAllArrayValue(
java.lang.Iterable extends org.yamcs.protobuf.Yamcs.Value> values) {
if (arrayValueBuilder_ == null) {
ensureArrayValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, arrayValue_);
onChanged();
} else {
arrayValueBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public Builder clearArrayValue() {
if (arrayValueBuilder_ == null) {
arrayValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
} else {
arrayValueBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public Builder removeArrayValue(int index) {
if (arrayValueBuilder_ == null) {
ensureArrayValueIsMutable();
arrayValue_.remove(index);
onChanged();
} else {
arrayValueBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder getArrayValueBuilder(
int index) {
return getArrayValueFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getArrayValueOrBuilder(
int index) {
if (arrayValueBuilder_ == null) {
return arrayValue_.get(index); } else {
return arrayValueBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public java.util.List extends org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getArrayValueOrBuilderList() {
if (arrayValueBuilder_ != null) {
return arrayValueBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(arrayValue_);
}
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder addArrayValueBuilder() {
return getArrayValueFieldBuilder().addBuilder(
org.yamcs.protobuf.Yamcs.Value.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder addArrayValueBuilder(
int index) {
return getArrayValueFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Yamcs.Value.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.Value arrayValue = 13;
*/
public java.util.List
getArrayValueBuilderList() {
return getArrayValueFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getArrayValueFieldBuilder() {
if (arrayValueBuilder_ == null) {
arrayValueBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>(
arrayValue_,
((bitField0_ & 0x00001000) != 0),
getParentForChildren(),
isClean());
arrayValue_ = null;
}
return arrayValueBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.Value)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.Value)
private static final org.yamcs.protobuf.Yamcs.Value DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.Value();
}
public static org.yamcs.protobuf.Yamcs.Value getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Value parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Value(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AggregateValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.AggregateValue)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string name = 1;
* @return A list containing the name.
*/
java.util.List
getNameList();
/**
* repeated string name = 1;
* @return The count of name.
*/
int getNameCount();
/**
* repeated string name = 1;
* @param index The index of the element to return.
* @return The name at the given index.
*/
java.lang.String getName(int index);
/**
* repeated string name = 1;
* @param index The index of the value to return.
* @return The bytes of the name at the given index.
*/
com.google.protobuf.ByteString
getNameBytes(int index);
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
java.util.List
getValueList();
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
org.yamcs.protobuf.Yamcs.Value getValue(int index);
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
int getValueCount();
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
java.util.List extends org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValueOrBuilderList();
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder(
int index);
}
/**
*
* An aggregate value is an ordered list of (member name, member value).
* Two arrays are used in order to be able to send just the values (since
* the names will not change)
*
*
* Protobuf type {@code yamcs.protobuf.AggregateValue}
*/
public static final class AggregateValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.AggregateValue)
AggregateValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use AggregateValue.newBuilder() to construct.
private AggregateValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AggregateValue() {
name_ = com.google.protobuf.LazyStringArrayList.EMPTY;
value_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AggregateValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AggregateValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
name_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
name_.add(bs);
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
value_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
value_.add(
input.readMessage(org.yamcs.protobuf.Yamcs.Value.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
name_ = name_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
value_ = java.util.Collections.unmodifiableList(value_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_AggregateValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_AggregateValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.AggregateValue.class, org.yamcs.protobuf.Yamcs.AggregateValue.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList name_;
/**
* repeated string name = 1;
* @return A list containing the name.
*/
public com.google.protobuf.ProtocolStringList
getNameList() {
return name_;
}
/**
* repeated string name = 1;
* @return The count of name.
*/
public int getNameCount() {
return name_.size();
}
/**
* repeated string name = 1;
* @param index The index of the element to return.
* @return The name at the given index.
*/
public java.lang.String getName(int index) {
return name_.get(index);
}
/**
* repeated string name = 1;
* @param index The index of the value to return.
* @return The bytes of the name at the given index.
*/
public com.google.protobuf.ByteString
getNameBytes(int index) {
return name_.getByteString(index);
}
public static final int VALUE_FIELD_NUMBER = 2;
private java.util.List value_;
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
@java.lang.Override
public java.util.List getValueList() {
return value_;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValueOrBuilderList() {
return value_;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
@java.lang.Override
public int getValueCount() {
return value_.size();
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.Value getValue(int index) {
return value_.get(index);
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder(
int index) {
return value_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getValueCount(); i++) {
if (!getValue(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < name_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_.getRaw(i));
}
for (int i = 0; i < value_.size(); i++) {
output.writeMessage(2, value_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < name_.size(); i++) {
dataSize += computeStringSizeNoTag(name_.getRaw(i));
}
size += dataSize;
size += 1 * getNameList().size();
}
for (int i = 0; i < value_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, value_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.AggregateValue)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.AggregateValue other = (org.yamcs.protobuf.Yamcs.AggregateValue) obj;
if (!getNameList()
.equals(other.getNameList())) return false;
if (!getValueList()
.equals(other.getValueList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getNameCount() > 0) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getNameList().hashCode();
}
if (getValueCount() > 0) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValueList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.AggregateValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.AggregateValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An aggregate value is an ordered list of (member name, member value).
* Two arrays are used in order to be able to send just the values (since
* the names will not change)
*
*
* Protobuf type {@code yamcs.protobuf.AggregateValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.AggregateValue)
org.yamcs.protobuf.Yamcs.AggregateValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_AggregateValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_AggregateValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.AggregateValue.class, org.yamcs.protobuf.Yamcs.AggregateValue.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.AggregateValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (valueBuilder_ == null) {
value_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
valueBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_AggregateValue_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.AggregateValue getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.AggregateValue.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.AggregateValue build() {
org.yamcs.protobuf.Yamcs.AggregateValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.AggregateValue buildPartial() {
org.yamcs.protobuf.Yamcs.AggregateValue result = new org.yamcs.protobuf.Yamcs.AggregateValue(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
name_ = name_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.name_ = name_;
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
value_ = java.util.Collections.unmodifiableList(value_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.value_ = value_;
} else {
result.value_ = valueBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.AggregateValue) {
return mergeFrom((org.yamcs.protobuf.Yamcs.AggregateValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.AggregateValue other) {
if (other == org.yamcs.protobuf.Yamcs.AggregateValue.getDefaultInstance()) return this;
if (!other.name_.isEmpty()) {
if (name_.isEmpty()) {
name_ = other.name_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNameIsMutable();
name_.addAll(other.name_);
}
onChanged();
}
if (valueBuilder_ == null) {
if (!other.value_.isEmpty()) {
if (value_.isEmpty()) {
value_ = other.value_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureValueIsMutable();
value_.addAll(other.value_);
}
onChanged();
}
} else {
if (!other.value_.isEmpty()) {
if (valueBuilder_.isEmpty()) {
valueBuilder_.dispose();
valueBuilder_ = null;
value_ = other.value_;
bitField0_ = (bitField0_ & ~0x00000002);
valueBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getValueFieldBuilder() : null;
} else {
valueBuilder_.addAllMessages(other.value_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getValueCount(); i++) {
if (!getValue(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.AggregateValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.AggregateValue) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList name_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureNameIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
name_ = new com.google.protobuf.LazyStringArrayList(name_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string name = 1;
* @return A list containing the name.
*/
public com.google.protobuf.ProtocolStringList
getNameList() {
return name_.getUnmodifiableView();
}
/**
* repeated string name = 1;
* @return The count of name.
*/
public int getNameCount() {
return name_.size();
}
/**
* repeated string name = 1;
* @param index The index of the element to return.
* @return The name at the given index.
*/
public java.lang.String getName(int index) {
return name_.get(index);
}
/**
* repeated string name = 1;
* @param index The index of the value to return.
* @return The bytes of the name at the given index.
*/
public com.google.protobuf.ByteString
getNameBytes(int index) {
return name_.getByteString(index);
}
/**
* repeated string name = 1;
* @param index The index to set the value at.
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureNameIsMutable();
name_.set(index, value);
onChanged();
return this;
}
/**
* repeated string name = 1;
* @param value The name to add.
* @return This builder for chaining.
*/
public Builder addName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureNameIsMutable();
name_.add(value);
onChanged();
return this;
}
/**
* repeated string name = 1;
* @param values The name to add.
* @return This builder for chaining.
*/
public Builder addAllName(
java.lang.Iterable values) {
ensureNameIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, name_);
onChanged();
return this;
}
/**
* repeated string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string name = 1;
* @param value The bytes of the name to add.
* @return This builder for chaining.
*/
public Builder addNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureNameIsMutable();
name_.add(value);
onChanged();
return this;
}
private java.util.List value_ =
java.util.Collections.emptyList();
private void ensureValueIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
value_ = new java.util.ArrayList(value_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder> valueBuilder_;
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public java.util.List getValueList() {
if (valueBuilder_ == null) {
return java.util.Collections.unmodifiableList(value_);
} else {
return valueBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public int getValueCount() {
if (valueBuilder_ == null) {
return value_.size();
} else {
return valueBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.Value getValue(int index) {
if (valueBuilder_ == null) {
return value_.get(index);
} else {
return valueBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public Builder setValue(
int index, org.yamcs.protobuf.Yamcs.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueIsMutable();
value_.set(index, value);
onChanged();
} else {
valueBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public Builder setValue(
int index, org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.set(index, builderForValue.build());
onChanged();
} else {
valueBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public Builder addValue(org.yamcs.protobuf.Yamcs.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueIsMutable();
value_.add(value);
onChanged();
} else {
valueBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public Builder addValue(
int index, org.yamcs.protobuf.Yamcs.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValueIsMutable();
value_.add(index, value);
onChanged();
} else {
valueBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public Builder addValue(
org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.add(builderForValue.build());
onChanged();
} else {
valueBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public Builder addValue(
int index, org.yamcs.protobuf.Yamcs.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.add(index, builderForValue.build());
onChanged();
} else {
valueBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public Builder addAllValue(
java.lang.Iterable extends org.yamcs.protobuf.Yamcs.Value> values) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, value_);
onChanged();
} else {
valueBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public Builder clearValue() {
if (valueBuilder_ == null) {
value_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
valueBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public Builder removeValue(int index) {
if (valueBuilder_ == null) {
ensureValueIsMutable();
value_.remove(index);
onChanged();
} else {
valueBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder getValueBuilder(
int index) {
return getValueFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.ValueOrBuilder getValueOrBuilder(
int index) {
if (valueBuilder_ == null) {
return value_.get(index); } else {
return valueBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public java.util.List extends org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValueOrBuilderList() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(value_);
}
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder addValueBuilder() {
return getValueFieldBuilder().addBuilder(
org.yamcs.protobuf.Yamcs.Value.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public org.yamcs.protobuf.Yamcs.Value.Builder addValueBuilder(
int index) {
return getValueFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Yamcs.Value.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.Value value = 2;
*/
public java.util.List
getValueBuilderList() {
return getValueFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.Value, org.yamcs.protobuf.Yamcs.Value.Builder, org.yamcs.protobuf.Yamcs.ValueOrBuilder>(
value_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.AggregateValue)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.AggregateValue)
private static final org.yamcs.protobuf.Yamcs.AggregateValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.AggregateValue();
}
public static org.yamcs.protobuf.Yamcs.AggregateValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AggregateValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AggregateValue(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.AggregateValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NamedObjectIdOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.NamedObjectId)
com.google.protobuf.MessageOrBuilder {
/**
* required string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* required string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* required string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional string namespace = 2;
* @return Whether the namespace field is set.
*/
boolean hasNamespace();
/**
* optional string namespace = 2;
* @return The namespace.
*/
java.lang.String getNamespace();
/**
* optional string namespace = 2;
* @return The bytes for namespace.
*/
com.google.protobuf.ByteString
getNamespaceBytes();
}
/**
*
* Used by external clients to identify an item in the Mission Database
* If namespace is set, then the name is that of an alias, rather than
* the qualified name.
*
*
* Protobuf type {@code yamcs.protobuf.NamedObjectId}
*/
public static final class NamedObjectId extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.NamedObjectId)
NamedObjectIdOrBuilder {
private static final long serialVersionUID = 0L;
// Use NamedObjectId.newBuilder() to construct.
private NamedObjectId(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NamedObjectId() {
name_ = "";
namespace_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new NamedObjectId();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NamedObjectId(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
namespace_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectId_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectId_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.NamedObjectId.class, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* required string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAMESPACE_FIELD_NUMBER = 2;
private volatile java.lang.Object namespace_;
/**
* optional string namespace = 2;
* @return Whether the namespace field is set.
*/
@java.lang.Override
public boolean hasNamespace() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string namespace = 2;
* @return The namespace.
*/
@java.lang.Override
public java.lang.String getNamespace() {
java.lang.Object ref = namespace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
namespace_ = s;
}
return s;
}
}
/**
* optional string namespace = 2;
* @return The bytes for namespace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNamespaceBytes() {
java.lang.Object ref = namespace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
namespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, namespace_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, namespace_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.NamedObjectId)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.NamedObjectId other = (org.yamcs.protobuf.Yamcs.NamedObjectId) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasNamespace() != other.hasNamespace()) return false;
if (hasNamespace()) {
if (!getNamespace()
.equals(other.getNamespace())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasNamespace()) {
hash = (37 * hash) + NAMESPACE_FIELD_NUMBER;
hash = (53 * hash) + getNamespace().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.NamedObjectId prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Used by external clients to identify an item in the Mission Database
* If namespace is set, then the name is that of an alias, rather than
* the qualified name.
*
*
* Protobuf type {@code yamcs.protobuf.NamedObjectId}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.NamedObjectId)
org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectId_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectId_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.NamedObjectId.class, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.NamedObjectId.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
namespace_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectId_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectId getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectId build() {
org.yamcs.protobuf.Yamcs.NamedObjectId result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectId buildPartial() {
org.yamcs.protobuf.Yamcs.NamedObjectId result = new org.yamcs.protobuf.Yamcs.NamedObjectId(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.namespace_ = namespace_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.NamedObjectId) {
return mergeFrom((org.yamcs.protobuf.Yamcs.NamedObjectId)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.NamedObjectId other) {
if (other == org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasNamespace()) {
bitField0_ |= 0x00000002;
namespace_ = other.namespace_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.NamedObjectId parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.NamedObjectId) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* required string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private java.lang.Object namespace_ = "";
/**
* optional string namespace = 2;
* @return Whether the namespace field is set.
*/
public boolean hasNamespace() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string namespace = 2;
* @return The namespace.
*/
public java.lang.String getNamespace() {
java.lang.Object ref = namespace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
namespace_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string namespace = 2;
* @return The bytes for namespace.
*/
public com.google.protobuf.ByteString
getNamespaceBytes() {
java.lang.Object ref = namespace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
namespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string namespace = 2;
* @param value The namespace to set.
* @return This builder for chaining.
*/
public Builder setNamespace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
namespace_ = value;
onChanged();
return this;
}
/**
* optional string namespace = 2;
* @return This builder for chaining.
*/
public Builder clearNamespace() {
bitField0_ = (bitField0_ & ~0x00000002);
namespace_ = getDefaultInstance().getNamespace();
onChanged();
return this;
}
/**
* optional string namespace = 2;
* @param value The bytes for namespace to set.
* @return This builder for chaining.
*/
public Builder setNamespaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
namespace_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.NamedObjectId)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.NamedObjectId)
private static final org.yamcs.protobuf.Yamcs.NamedObjectId DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.NamedObjectId();
}
public static org.yamcs.protobuf.Yamcs.NamedObjectId getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NamedObjectId parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NamedObjectId(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectId getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NamedObjectListOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.NamedObjectList)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
java.util.List
getListList();
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
org.yamcs.protobuf.Yamcs.NamedObjectId getList(int index);
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
int getListCount();
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getListOrBuilderList();
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getListOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.NamedObjectList}
*/
public static final class NamedObjectList extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.NamedObjectList)
NamedObjectListOrBuilder {
private static final long serialVersionUID = 0L;
// Use NamedObjectList.newBuilder() to construct.
private NamedObjectList(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NamedObjectList() {
list_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new NamedObjectList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NamedObjectList(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
list_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
list_.add(
input.readMessage(org.yamcs.protobuf.Yamcs.NamedObjectId.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
list_ = java.util.Collections.unmodifiableList(list_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.NamedObjectList.class, org.yamcs.protobuf.Yamcs.NamedObjectList.Builder.class);
}
public static final int LIST_FIELD_NUMBER = 1;
private java.util.List list_;
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
@java.lang.Override
public java.util.List getListList() {
return list_;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getListOrBuilderList() {
return list_;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
@java.lang.Override
public int getListCount() {
return list_.size();
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectId getList(int index) {
return list_.get(index);
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getListOrBuilder(
int index) {
return list_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getListCount(); i++) {
if (!getList(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < list_.size(); i++) {
output.writeMessage(1, list_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < list_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, list_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.NamedObjectList)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.NamedObjectList other = (org.yamcs.protobuf.Yamcs.NamedObjectList) obj;
if (!getListList()
.equals(other.getListList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getListCount() > 0) {
hash = (37 * hash) + LIST_FIELD_NUMBER;
hash = (53 * hash) + getListList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.NamedObjectList prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.NamedObjectList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.NamedObjectList)
org.yamcs.protobuf.Yamcs.NamedObjectListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.NamedObjectList.class, org.yamcs.protobuf.Yamcs.NamedObjectList.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.NamedObjectList.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getListFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (listBuilder_ == null) {
list_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
listBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_NamedObjectList_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectList getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.NamedObjectList.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectList build() {
org.yamcs.protobuf.Yamcs.NamedObjectList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectList buildPartial() {
org.yamcs.protobuf.Yamcs.NamedObjectList result = new org.yamcs.protobuf.Yamcs.NamedObjectList(this);
int from_bitField0_ = bitField0_;
if (listBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
list_ = java.util.Collections.unmodifiableList(list_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.list_ = list_;
} else {
result.list_ = listBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.NamedObjectList) {
return mergeFrom((org.yamcs.protobuf.Yamcs.NamedObjectList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.NamedObjectList other) {
if (other == org.yamcs.protobuf.Yamcs.NamedObjectList.getDefaultInstance()) return this;
if (listBuilder_ == null) {
if (!other.list_.isEmpty()) {
if (list_.isEmpty()) {
list_ = other.list_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureListIsMutable();
list_.addAll(other.list_);
}
onChanged();
}
} else {
if (!other.list_.isEmpty()) {
if (listBuilder_.isEmpty()) {
listBuilder_.dispose();
listBuilder_ = null;
list_ = other.list_;
bitField0_ = (bitField0_ & ~0x00000001);
listBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getListFieldBuilder() : null;
} else {
listBuilder_.addAllMessages(other.list_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getListCount(); i++) {
if (!getList(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.NamedObjectList parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.NamedObjectList) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List list_ =
java.util.Collections.emptyList();
private void ensureListIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
list_ = new java.util.ArrayList(list_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder> listBuilder_;
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public java.util.List getListList() {
if (listBuilder_ == null) {
return java.util.Collections.unmodifiableList(list_);
} else {
return listBuilder_.getMessageList();
}
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public int getListCount() {
if (listBuilder_ == null) {
return list_.size();
} else {
return listBuilder_.getCount();
}
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId getList(int index) {
if (listBuilder_ == null) {
return list_.get(index);
} else {
return listBuilder_.getMessage(index);
}
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public Builder setList(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (listBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureListIsMutable();
list_.set(index, value);
onChanged();
} else {
listBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public Builder setList(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (listBuilder_ == null) {
ensureListIsMutable();
list_.set(index, builderForValue.build());
onChanged();
} else {
listBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public Builder addList(org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (listBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureListIsMutable();
list_.add(value);
onChanged();
} else {
listBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public Builder addList(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (listBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureListIsMutable();
list_.add(index, value);
onChanged();
} else {
listBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public Builder addList(
org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (listBuilder_ == null) {
ensureListIsMutable();
list_.add(builderForValue.build());
onChanged();
} else {
listBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public Builder addList(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (listBuilder_ == null) {
ensureListIsMutable();
list_.add(index, builderForValue.build());
onChanged();
} else {
listBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public Builder addAllList(
java.lang.Iterable extends org.yamcs.protobuf.Yamcs.NamedObjectId> values) {
if (listBuilder_ == null) {
ensureListIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, list_);
onChanged();
} else {
listBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public Builder clearList() {
if (listBuilder_ == null) {
list_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
listBuilder_.clear();
}
return this;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public Builder removeList(int index) {
if (listBuilder_ == null) {
ensureListIsMutable();
list_.remove(index);
onChanged();
} else {
listBuilder_.remove(index);
}
return this;
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder getListBuilder(
int index) {
return getListFieldBuilder().getBuilder(index);
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getListOrBuilder(
int index) {
if (listBuilder_ == null) {
return list_.get(index); } else {
return listBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getListOrBuilderList() {
if (listBuilder_ != null) {
return listBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(list_);
}
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder addListBuilder() {
return getListFieldBuilder().addBuilder(
org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder addListBuilder(
int index) {
return getListFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance());
}
/**
* repeated .yamcs.protobuf.NamedObjectId list = 1;
*/
public java.util.List
getListBuilderList() {
return getListFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getListFieldBuilder() {
if (listBuilder_ == null) {
listBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>(
list_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
list_ = null;
}
return listBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.NamedObjectList)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.NamedObjectList)
private static final org.yamcs.protobuf.Yamcs.NamedObjectList DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.NamedObjectList();
}
public static org.yamcs.protobuf.Yamcs.NamedObjectList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NamedObjectList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NamedObjectList(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectList getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ArchiveRecordOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.ArchiveRecord)
com.google.protobuf.MessageOrBuilder {
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
* @return The id.
*/
org.yamcs.protobuf.Yamcs.NamedObjectId getId();
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
*/
org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getIdOrBuilder();
/**
* optional int32 num = 4;
* @return Whether the num field is set.
*/
boolean hasNum();
/**
* optional int32 num = 4;
* @return The num.
*/
int getNum();
/**
* optional int64 seqFirst = 6;
* @return Whether the seqFirst field is set.
*/
boolean hasSeqFirst();
/**
* optional int64 seqFirst = 6;
* @return The seqFirst.
*/
long getSeqFirst();
/**
* optional int64 seqLast = 7;
* @return Whether the seqLast field is set.
*/
boolean hasSeqLast();
/**
* optional int64 seqLast = 7;
* @return The seqLast.
*/
long getSeqLast();
/**
* optional .google.protobuf.Timestamp first = 8;
* @return Whether the first field is set.
*/
boolean hasFirst();
/**
* optional .google.protobuf.Timestamp first = 8;
* @return The first.
*/
com.google.protobuf.Timestamp getFirst();
/**
* optional .google.protobuf.Timestamp first = 8;
*/
com.google.protobuf.TimestampOrBuilder getFirstOrBuilder();
/**
* optional .google.protobuf.Timestamp last = 9;
* @return Whether the last field is set.
*/
boolean hasLast();
/**
* optional .google.protobuf.Timestamp last = 9;
* @return The last.
*/
com.google.protobuf.Timestamp getLast();
/**
* optional .google.protobuf.Timestamp last = 9;
*/
com.google.protobuf.TimestampOrBuilder getLastOrBuilder();
/**
* map<string, string> extra = 10;
*/
int getExtraCount();
/**
* map<string, string> extra = 10;
*/
boolean containsExtra(
java.lang.String key);
/**
* Use {@link #getExtraMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getExtra();
/**
* map<string, string> extra = 10;
*/
java.util.Map
getExtraMap();
/**
* map<string, string> extra = 10;
*/
java.lang.String getExtraOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
* map<string, string> extra = 10;
*/
java.lang.String getExtraOrThrow(
java.lang.String key);
}
/**
*
*contains histogram data
*
*
* Protobuf type {@code yamcs.protobuf.ArchiveRecord}
*/
public static final class ArchiveRecord extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.ArchiveRecord)
ArchiveRecordOrBuilder {
private static final long serialVersionUID = 0L;
// Use ArchiveRecord.newBuilder() to construct.
private ArchiveRecord(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ArchiveRecord() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ArchiveRecord();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ArchiveRecord(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.yamcs.protobuf.Yamcs.NamedObjectId.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = id_.toBuilder();
}
id_ = input.readMessage(org.yamcs.protobuf.Yamcs.NamedObjectId.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(id_);
id_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 32: {
bitField0_ |= 0x00000002;
num_ = input.readInt32();
break;
}
case 48: {
bitField0_ |= 0x00000004;
seqFirst_ = input.readInt64();
break;
}
case 56: {
bitField0_ |= 0x00000008;
seqLast_ = input.readInt64();
break;
}
case 66: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) != 0)) {
subBuilder = first_.toBuilder();
}
first_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(first_);
first_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 74: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) != 0)) {
subBuilder = last_.toBuilder();
}
last_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(last_);
last_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 82: {
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
extra_ = com.google.protobuf.MapField.newMapField(
ExtraDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000040;
}
com.google.protobuf.MapEntry
extra__ = input.readMessage(
ExtraDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
extra_.getMutableMap().put(
extra__.getKey(), extra__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ArchiveRecord_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 10:
return internalGetExtra();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ArchiveRecord_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ArchiveRecord.class, org.yamcs.protobuf.Yamcs.ArchiveRecord.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private org.yamcs.protobuf.Yamcs.NamedObjectId id_;
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
* @return The id.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectId getId() {
return id_ == null ? org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance() : id_;
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getIdOrBuilder() {
return id_ == null ? org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance() : id_;
}
public static final int NUM_FIELD_NUMBER = 4;
private int num_;
/**
* optional int32 num = 4;
* @return Whether the num field is set.
*/
@java.lang.Override
public boolean hasNum() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 num = 4;
* @return The num.
*/
@java.lang.Override
public int getNum() {
return num_;
}
public static final int SEQFIRST_FIELD_NUMBER = 6;
private long seqFirst_;
/**
* optional int64 seqFirst = 6;
* @return Whether the seqFirst field is set.
*/
@java.lang.Override
public boolean hasSeqFirst() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int64 seqFirst = 6;
* @return The seqFirst.
*/
@java.lang.Override
public long getSeqFirst() {
return seqFirst_;
}
public static final int SEQLAST_FIELD_NUMBER = 7;
private long seqLast_;
/**
* optional int64 seqLast = 7;
* @return Whether the seqLast field is set.
*/
@java.lang.Override
public boolean hasSeqLast() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int64 seqLast = 7;
* @return The seqLast.
*/
@java.lang.Override
public long getSeqLast() {
return seqLast_;
}
public static final int FIRST_FIELD_NUMBER = 8;
private com.google.protobuf.Timestamp first_;
/**
* optional .google.protobuf.Timestamp first = 8;
* @return Whether the first field is set.
*/
@java.lang.Override
public boolean hasFirst() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .google.protobuf.Timestamp first = 8;
* @return The first.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getFirst() {
return first_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : first_;
}
/**
* optional .google.protobuf.Timestamp first = 8;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getFirstOrBuilder() {
return first_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : first_;
}
public static final int LAST_FIELD_NUMBER = 9;
private com.google.protobuf.Timestamp last_;
/**
* optional .google.protobuf.Timestamp last = 9;
* @return Whether the last field is set.
*/
@java.lang.Override
public boolean hasLast() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .google.protobuf.Timestamp last = 9;
* @return The last.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getLast() {
return last_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : last_;
}
/**
* optional .google.protobuf.Timestamp last = 9;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getLastOrBuilder() {
return last_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : last_;
}
public static final int EXTRA_FIELD_NUMBER = 10;
private static final class ExtraDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ArchiveRecord_ExtraEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> extra_;
private com.google.protobuf.MapField
internalGetExtra() {
if (extra_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ExtraDefaultEntryHolder.defaultEntry);
}
return extra_;
}
public int getExtraCount() {
return internalGetExtra().getMap().size();
}
/**
* map<string, string> extra = 10;
*/
@java.lang.Override
public boolean containsExtra(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetExtra().getMap().containsKey(key);
}
/**
* Use {@link #getExtraMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getExtra() {
return getExtraMap();
}
/**
* map<string, string> extra = 10;
*/
@java.lang.Override
public java.util.Map getExtraMap() {
return internalGetExtra().getMap();
}
/**
* map<string, string> extra = 10;
*/
@java.lang.Override
public java.lang.String getExtraOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetExtra().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> extra = 10;
*/
@java.lang.Override
public java.lang.String getExtraOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetExtra().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasId()) {
if (!getId().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getId());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(4, num_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(6, seqFirst_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt64(7, seqLast_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(8, getFirst());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(9, getLast());
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetExtra(),
ExtraDefaultEntryHolder.defaultEntry,
10);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getId());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, num_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, seqFirst_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, seqLast_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getFirst());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getLast());
}
for (java.util.Map.Entry entry
: internalGetExtra().getMap().entrySet()) {
com.google.protobuf.MapEntry
extra__ = ExtraDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, extra__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.ArchiveRecord)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.ArchiveRecord other = (org.yamcs.protobuf.Yamcs.ArchiveRecord) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (hasNum() != other.hasNum()) return false;
if (hasNum()) {
if (getNum()
!= other.getNum()) return false;
}
if (hasSeqFirst() != other.hasSeqFirst()) return false;
if (hasSeqFirst()) {
if (getSeqFirst()
!= other.getSeqFirst()) return false;
}
if (hasSeqLast() != other.hasSeqLast()) return false;
if (hasSeqLast()) {
if (getSeqLast()
!= other.getSeqLast()) return false;
}
if (hasFirst() != other.hasFirst()) return false;
if (hasFirst()) {
if (!getFirst()
.equals(other.getFirst())) return false;
}
if (hasLast() != other.hasLast()) return false;
if (hasLast()) {
if (!getLast()
.equals(other.getLast())) return false;
}
if (!internalGetExtra().equals(
other.internalGetExtra())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasNum()) {
hash = (37 * hash) + NUM_FIELD_NUMBER;
hash = (53 * hash) + getNum();
}
if (hasSeqFirst()) {
hash = (37 * hash) + SEQFIRST_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSeqFirst());
}
if (hasSeqLast()) {
hash = (37 * hash) + SEQLAST_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSeqLast());
}
if (hasFirst()) {
hash = (37 * hash) + FIRST_FIELD_NUMBER;
hash = (53 * hash) + getFirst().hashCode();
}
if (hasLast()) {
hash = (37 * hash) + LAST_FIELD_NUMBER;
hash = (53 * hash) + getLast().hashCode();
}
if (!internalGetExtra().getMap().isEmpty()) {
hash = (37 * hash) + EXTRA_FIELD_NUMBER;
hash = (53 * hash) + internalGetExtra().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.ArchiveRecord prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*contains histogram data
*
*
* Protobuf type {@code yamcs.protobuf.ArchiveRecord}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.ArchiveRecord)
org.yamcs.protobuf.Yamcs.ArchiveRecordOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ArchiveRecord_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 10:
return internalGetExtra();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 10:
return internalGetMutableExtra();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ArchiveRecord_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ArchiveRecord.class, org.yamcs.protobuf.Yamcs.ArchiveRecord.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.ArchiveRecord.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getIdFieldBuilder();
getFirstFieldBuilder();
getLastFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (idBuilder_ == null) {
id_ = null;
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
num_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
seqFirst_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
seqLast_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
if (firstBuilder_ == null) {
first_ = null;
} else {
firstBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (lastBuilder_ == null) {
last_ = null;
} else {
lastBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
internalGetMutableExtra().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ArchiveRecord_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ArchiveRecord getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.ArchiveRecord.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ArchiveRecord build() {
org.yamcs.protobuf.Yamcs.ArchiveRecord result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ArchiveRecord buildPartial() {
org.yamcs.protobuf.Yamcs.ArchiveRecord result = new org.yamcs.protobuf.Yamcs.ArchiveRecord(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.num_ = num_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.seqFirst_ = seqFirst_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.seqLast_ = seqLast_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
if (firstBuilder_ == null) {
result.first_ = first_;
} else {
result.first_ = firstBuilder_.build();
}
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
if (lastBuilder_ == null) {
result.last_ = last_;
} else {
result.last_ = lastBuilder_.build();
}
to_bitField0_ |= 0x00000020;
}
result.extra_ = internalGetExtra();
result.extra_.makeImmutable();
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.ArchiveRecord) {
return mergeFrom((org.yamcs.protobuf.Yamcs.ArchiveRecord)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.ArchiveRecord other) {
if (other == org.yamcs.protobuf.Yamcs.ArchiveRecord.getDefaultInstance()) return this;
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasNum()) {
setNum(other.getNum());
}
if (other.hasSeqFirst()) {
setSeqFirst(other.getSeqFirst());
}
if (other.hasSeqLast()) {
setSeqLast(other.getSeqLast());
}
if (other.hasFirst()) {
mergeFirst(other.getFirst());
}
if (other.hasLast()) {
mergeLast(other.getLast());
}
internalGetMutableExtra().mergeFrom(
other.internalGetExtra());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasId()) {
if (!getId().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.ArchiveRecord parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.ArchiveRecord) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.yamcs.protobuf.Yamcs.NamedObjectId id_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder> idBuilder_;
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
* @return Whether the id field is set.
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
* @return The id.
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId getId() {
if (idBuilder_ == null) {
return id_ == null ? org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance() : id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
*/
public Builder setId(org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
*/
public Builder setId(
org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
*/
public Builder mergeId(org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (idBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
id_ != null &&
id_ != org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance()) {
id_ =
org.yamcs.protobuf.Yamcs.NamedObjectId.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = null;
onChanged();
} else {
idBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder getIdBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_ == null ?
org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance() : id_;
}
}
/**
* optional .yamcs.protobuf.NamedObjectId id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>(
getId(),
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
private int num_ ;
/**
* optional int32 num = 4;
* @return Whether the num field is set.
*/
@java.lang.Override
public boolean hasNum() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 num = 4;
* @return The num.
*/
@java.lang.Override
public int getNum() {
return num_;
}
/**
* optional int32 num = 4;
* @param value The num to set.
* @return This builder for chaining.
*/
public Builder setNum(int value) {
bitField0_ |= 0x00000002;
num_ = value;
onChanged();
return this;
}
/**
* optional int32 num = 4;
* @return This builder for chaining.
*/
public Builder clearNum() {
bitField0_ = (bitField0_ & ~0x00000002);
num_ = 0;
onChanged();
return this;
}
private long seqFirst_ ;
/**
* optional int64 seqFirst = 6;
* @return Whether the seqFirst field is set.
*/
@java.lang.Override
public boolean hasSeqFirst() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int64 seqFirst = 6;
* @return The seqFirst.
*/
@java.lang.Override
public long getSeqFirst() {
return seqFirst_;
}
/**
* optional int64 seqFirst = 6;
* @param value The seqFirst to set.
* @return This builder for chaining.
*/
public Builder setSeqFirst(long value) {
bitField0_ |= 0x00000004;
seqFirst_ = value;
onChanged();
return this;
}
/**
* optional int64 seqFirst = 6;
* @return This builder for chaining.
*/
public Builder clearSeqFirst() {
bitField0_ = (bitField0_ & ~0x00000004);
seqFirst_ = 0L;
onChanged();
return this;
}
private long seqLast_ ;
/**
* optional int64 seqLast = 7;
* @return Whether the seqLast field is set.
*/
@java.lang.Override
public boolean hasSeqLast() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int64 seqLast = 7;
* @return The seqLast.
*/
@java.lang.Override
public long getSeqLast() {
return seqLast_;
}
/**
* optional int64 seqLast = 7;
* @param value The seqLast to set.
* @return This builder for chaining.
*/
public Builder setSeqLast(long value) {
bitField0_ |= 0x00000008;
seqLast_ = value;
onChanged();
return this;
}
/**
* optional int64 seqLast = 7;
* @return This builder for chaining.
*/
public Builder clearSeqLast() {
bitField0_ = (bitField0_ & ~0x00000008);
seqLast_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Timestamp first_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> firstBuilder_;
/**
* optional .google.protobuf.Timestamp first = 8;
* @return Whether the first field is set.
*/
public boolean hasFirst() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .google.protobuf.Timestamp first = 8;
* @return The first.
*/
public com.google.protobuf.Timestamp getFirst() {
if (firstBuilder_ == null) {
return first_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : first_;
} else {
return firstBuilder_.getMessage();
}
}
/**
* optional .google.protobuf.Timestamp first = 8;
*/
public Builder setFirst(com.google.protobuf.Timestamp value) {
if (firstBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
first_ = value;
onChanged();
} else {
firstBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .google.protobuf.Timestamp first = 8;
*/
public Builder setFirst(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (firstBuilder_ == null) {
first_ = builderForValue.build();
onChanged();
} else {
firstBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .google.protobuf.Timestamp first = 8;
*/
public Builder mergeFirst(com.google.protobuf.Timestamp value) {
if (firstBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
first_ != null &&
first_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
first_ =
com.google.protobuf.Timestamp.newBuilder(first_).mergeFrom(value).buildPartial();
} else {
first_ = value;
}
onChanged();
} else {
firstBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .google.protobuf.Timestamp first = 8;
*/
public Builder clearFirst() {
if (firstBuilder_ == null) {
first_ = null;
onChanged();
} else {
firstBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .google.protobuf.Timestamp first = 8;
*/
public com.google.protobuf.Timestamp.Builder getFirstBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getFirstFieldBuilder().getBuilder();
}
/**
* optional .google.protobuf.Timestamp first = 8;
*/
public com.google.protobuf.TimestampOrBuilder getFirstOrBuilder() {
if (firstBuilder_ != null) {
return firstBuilder_.getMessageOrBuilder();
} else {
return first_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : first_;
}
}
/**
* optional .google.protobuf.Timestamp first = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getFirstFieldBuilder() {
if (firstBuilder_ == null) {
firstBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getFirst(),
getParentForChildren(),
isClean());
first_ = null;
}
return firstBuilder_;
}
private com.google.protobuf.Timestamp last_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> lastBuilder_;
/**
* optional .google.protobuf.Timestamp last = 9;
* @return Whether the last field is set.
*/
public boolean hasLast() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .google.protobuf.Timestamp last = 9;
* @return The last.
*/
public com.google.protobuf.Timestamp getLast() {
if (lastBuilder_ == null) {
return last_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : last_;
} else {
return lastBuilder_.getMessage();
}
}
/**
* optional .google.protobuf.Timestamp last = 9;
*/
public Builder setLast(com.google.protobuf.Timestamp value) {
if (lastBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
last_ = value;
onChanged();
} else {
lastBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .google.protobuf.Timestamp last = 9;
*/
public Builder setLast(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (lastBuilder_ == null) {
last_ = builderForValue.build();
onChanged();
} else {
lastBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .google.protobuf.Timestamp last = 9;
*/
public Builder mergeLast(com.google.protobuf.Timestamp value) {
if (lastBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
last_ != null &&
last_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
last_ =
com.google.protobuf.Timestamp.newBuilder(last_).mergeFrom(value).buildPartial();
} else {
last_ = value;
}
onChanged();
} else {
lastBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .google.protobuf.Timestamp last = 9;
*/
public Builder clearLast() {
if (lastBuilder_ == null) {
last_ = null;
onChanged();
} else {
lastBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .google.protobuf.Timestamp last = 9;
*/
public com.google.protobuf.Timestamp.Builder getLastBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getLastFieldBuilder().getBuilder();
}
/**
* optional .google.protobuf.Timestamp last = 9;
*/
public com.google.protobuf.TimestampOrBuilder getLastOrBuilder() {
if (lastBuilder_ != null) {
return lastBuilder_.getMessageOrBuilder();
} else {
return last_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : last_;
}
}
/**
* optional .google.protobuf.Timestamp last = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLastFieldBuilder() {
if (lastBuilder_ == null) {
lastBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLast(),
getParentForChildren(),
isClean());
last_ = null;
}
return lastBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> extra_;
private com.google.protobuf.MapField
internalGetExtra() {
if (extra_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ExtraDefaultEntryHolder.defaultEntry);
}
return extra_;
}
private com.google.protobuf.MapField
internalGetMutableExtra() {
onChanged();;
if (extra_ == null) {
extra_ = com.google.protobuf.MapField.newMapField(
ExtraDefaultEntryHolder.defaultEntry);
}
if (!extra_.isMutable()) {
extra_ = extra_.copy();
}
return extra_;
}
public int getExtraCount() {
return internalGetExtra().getMap().size();
}
/**
* map<string, string> extra = 10;
*/
@java.lang.Override
public boolean containsExtra(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetExtra().getMap().containsKey(key);
}
/**
* Use {@link #getExtraMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getExtra() {
return getExtraMap();
}
/**
* map<string, string> extra = 10;
*/
@java.lang.Override
public java.util.Map getExtraMap() {
return internalGetExtra().getMap();
}
/**
* map<string, string> extra = 10;
*/
@java.lang.Override
public java.lang.String getExtraOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetExtra().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> extra = 10;
*/
@java.lang.Override
public java.lang.String getExtraOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetExtra().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearExtra() {
internalGetMutableExtra().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> extra = 10;
*/
public Builder removeExtra(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableExtra().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableExtra() {
return internalGetMutableExtra().getMutableMap();
}
/**
* map<string, string> extra = 10;
*/
public Builder putExtra(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableExtra().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, string> extra = 10;
*/
public Builder putAllExtra(
java.util.Map values) {
internalGetMutableExtra().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.ArchiveRecord)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.ArchiveRecord)
private static final org.yamcs.protobuf.Yamcs.ArchiveRecord DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.ArchiveRecord();
}
public static org.yamcs.protobuf.Yamcs.ArchiveRecord getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ArchiveRecord parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ArchiveRecord(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ArchiveRecord getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReplaySpeedOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.ReplaySpeed)
com.google.protobuf.MessageOrBuilder {
/**
* required .yamcs.protobuf.ReplaySpeed.ReplaySpeedType type = 1;
* @return Whether the type field is set.
*/
boolean hasType();
/**
* required .yamcs.protobuf.ReplaySpeed.ReplaySpeedType type = 1;
* @return The type.
*/
org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType getType();
/**
* optional float param = 2;
* @return Whether the param field is set.
*/
boolean hasParam();
/**
* optional float param = 2;
* @return The param.
*/
float getParam();
}
/**
* Protobuf type {@code yamcs.protobuf.ReplaySpeed}
*/
public static final class ReplaySpeed extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.ReplaySpeed)
ReplaySpeedOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReplaySpeed.newBuilder() to construct.
private ReplaySpeed(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReplaySpeed() {
type_ = 1;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ReplaySpeed();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ReplaySpeed(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType value = org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = rawValue;
}
break;
}
case 21: {
bitField0_ |= 0x00000002;
param_ = input.readFloat();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplaySpeed_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplaySpeed_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ReplaySpeed.class, org.yamcs.protobuf.Yamcs.ReplaySpeed.Builder.class);
}
/**
* Protobuf enum {@code yamcs.protobuf.ReplaySpeed.ReplaySpeedType}
*/
public enum ReplaySpeedType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* AFAP = 1;
*/
AFAP(1),
/**
* FIXED_DELAY = 2;
*/
FIXED_DELAY(2),
/**
* REALTIME = 3;
*/
REALTIME(3),
/**
* STEP_BY_STEP = 4;
*/
STEP_BY_STEP(4),
;
/**
* AFAP = 1;
*/
public static final int AFAP_VALUE = 1;
/**
* FIXED_DELAY = 2;
*/
public static final int FIXED_DELAY_VALUE = 2;
/**
* REALTIME = 3;
*/
public static final int REALTIME_VALUE = 3;
/**
* STEP_BY_STEP = 4;
*/
public static final int STEP_BY_STEP_VALUE = 4;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ReplaySpeedType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ReplaySpeedType forNumber(int value) {
switch (value) {
case 1: return AFAP;
case 2: return FIXED_DELAY;
case 3: return REALTIME;
case 4: return STEP_BY_STEP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ReplaySpeedType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ReplaySpeedType findValueByNumber(int number) {
return ReplaySpeedType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.ReplaySpeed.getDescriptor().getEnumTypes().get(0);
}
private static final ReplaySpeedType[] VALUES = values();
public static ReplaySpeedType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ReplaySpeedType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:yamcs.protobuf.ReplaySpeed.ReplaySpeedType)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* required .yamcs.protobuf.ReplaySpeed.ReplaySpeedType type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required .yamcs.protobuf.ReplaySpeed.ReplaySpeedType type = 1;
* @return The type.
*/
@java.lang.Override public org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType getType() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType result = org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType.valueOf(type_);
return result == null ? org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType.AFAP : result;
}
public static final int PARAM_FIELD_NUMBER = 2;
private float param_;
/**
* optional float param = 2;
* @return Whether the param field is set.
*/
@java.lang.Override
public boolean hasParam() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional float param = 2;
* @return The param.
*/
@java.lang.Override
public float getParam() {
return param_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeFloat(2, param_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(2, param_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.ReplaySpeed)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.ReplaySpeed other = (org.yamcs.protobuf.Yamcs.ReplaySpeed) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (hasParam() != other.hasParam()) return false;
if (hasParam()) {
if (java.lang.Float.floatToIntBits(getParam())
!= java.lang.Float.floatToIntBits(
other.getParam())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasParam()) {
hash = (37 * hash) + PARAM_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getParam());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.ReplaySpeed prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.ReplaySpeed}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.ReplaySpeed)
org.yamcs.protobuf.Yamcs.ReplaySpeedOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplaySpeed_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplaySpeed_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ReplaySpeed.class, org.yamcs.protobuf.Yamcs.ReplaySpeed.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.ReplaySpeed.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 1;
bitField0_ = (bitField0_ & ~0x00000001);
param_ = 0F;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplaySpeed_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplaySpeed getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.ReplaySpeed.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplaySpeed build() {
org.yamcs.protobuf.Yamcs.ReplaySpeed result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplaySpeed buildPartial() {
org.yamcs.protobuf.Yamcs.ReplaySpeed result = new org.yamcs.protobuf.Yamcs.ReplaySpeed(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.param_ = param_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.ReplaySpeed) {
return mergeFrom((org.yamcs.protobuf.Yamcs.ReplaySpeed)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.ReplaySpeed other) {
if (other == org.yamcs.protobuf.Yamcs.ReplaySpeed.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasParam()) {
setParam(other.getParam());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasType()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.ReplaySpeed parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.ReplaySpeed) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 1;
/**
* required .yamcs.protobuf.ReplaySpeed.ReplaySpeedType type = 1;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required .yamcs.protobuf.ReplaySpeed.ReplaySpeedType type = 1;
* @return The type.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType getType() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType result = org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType.valueOf(type_);
return result == null ? org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType.AFAP : result;
}
/**
* required .yamcs.protobuf.ReplaySpeed.ReplaySpeedType type = 1;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.yamcs.protobuf.Yamcs.ReplaySpeed.ReplaySpeedType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* required .yamcs.protobuf.ReplaySpeed.ReplaySpeedType type = 1;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 1;
onChanged();
return this;
}
private float param_ ;
/**
* optional float param = 2;
* @return Whether the param field is set.
*/
@java.lang.Override
public boolean hasParam() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional float param = 2;
* @return The param.
*/
@java.lang.Override
public float getParam() {
return param_;
}
/**
* optional float param = 2;
* @param value The param to set.
* @return This builder for chaining.
*/
public Builder setParam(float value) {
bitField0_ |= 0x00000002;
param_ = value;
onChanged();
return this;
}
/**
* optional float param = 2;
* @return This builder for chaining.
*/
public Builder clearParam() {
bitField0_ = (bitField0_ & ~0x00000002);
param_ = 0F;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.ReplaySpeed)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.ReplaySpeed)
private static final org.yamcs.protobuf.Yamcs.ReplaySpeed DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.ReplaySpeed();
}
public static org.yamcs.protobuf.Yamcs.ReplaySpeed getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ReplaySpeed parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ReplaySpeed(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplaySpeed getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReplayRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.ReplayRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
* @return Whether the start field is set.
*/
boolean hasStart();
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
* @return The start.
*/
com.google.protobuf.Timestamp getStart();
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
*/
com.google.protobuf.TimestampOrBuilder getStartOrBuilder();
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
* @return Whether the stop field is set.
*/
boolean hasStop();
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
* @return The stop.
*/
com.google.protobuf.Timestamp getStop();
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
*/
com.google.protobuf.TimestampOrBuilder getStopOrBuilder();
/**
*
*what should happen at the end of the replay
*
*
* optional .yamcs.protobuf.EndAction endAction = 3 [default = QUIT];
* @return Whether the endAction field is set.
*/
boolean hasEndAction();
/**
*
*what should happen at the end of the replay
*
*
* optional .yamcs.protobuf.EndAction endAction = 3 [default = QUIT];
* @return The endAction.
*/
org.yamcs.protobuf.Yamcs.EndAction getEndAction();
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
* @return Whether the speed field is set.
*/
boolean hasSpeed();
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
* @return The speed.
*/
org.yamcs.protobuf.Yamcs.ReplaySpeed getSpeed();
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
*/
org.yamcs.protobuf.Yamcs.ReplaySpeedOrBuilder getSpeedOrBuilder();
/**
*
* Reverse the direction of the replay
*
*
* optional bool reverse = 15;
* @return Whether the reverse field is set.
*/
boolean hasReverse();
/**
*
* Reverse the direction of the replay
*
*
* optional bool reverse = 15;
* @return The reverse.
*/
boolean getReverse();
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
* @return Whether the parameterRequest field is set.
*/
boolean hasParameterRequest();
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
* @return The parameterRequest.
*/
org.yamcs.protobuf.Yamcs.ParameterReplayRequest getParameterRequest();
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
*/
org.yamcs.protobuf.Yamcs.ParameterReplayRequestOrBuilder getParameterRequestOrBuilder();
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
* @return Whether the packetRequest field is set.
*/
boolean hasPacketRequest();
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
* @return The packetRequest.
*/
org.yamcs.protobuf.Yamcs.PacketReplayRequest getPacketRequest();
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
*/
org.yamcs.protobuf.Yamcs.PacketReplayRequestOrBuilder getPacketRequestOrBuilder();
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
* @return Whether the eventRequest field is set.
*/
boolean hasEventRequest();
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
* @return The eventRequest.
*/
org.yamcs.protobuf.Yamcs.EventReplayRequest getEventRequest();
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
*/
org.yamcs.protobuf.Yamcs.EventReplayRequestOrBuilder getEventRequestOrBuilder();
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
* @return Whether the commandHistoryRequest field is set.
*/
boolean hasCommandHistoryRequest();
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
* @return The commandHistoryRequest.
*/
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest getCommandHistoryRequest();
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
*/
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequestOrBuilder getCommandHistoryRequestOrBuilder();
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
* @return Whether the ppRequest field is set.
*/
boolean hasPpRequest();
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
* @return The ppRequest.
*/
org.yamcs.protobuf.Yamcs.PpReplayRequest getPpRequest();
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
*/
org.yamcs.protobuf.Yamcs.PpReplayRequestOrBuilder getPpRequestOrBuilder();
/**
*
* Start the replay following initialization
* Defaults to true, if unspecified
*
*
* optional bool autostart = 16 [default = true];
* @return Whether the autostart field is set.
*/
boolean hasAutostart();
/**
*
* Start the replay following initialization
* Defaults to true, if unspecified
*
*
* optional bool autostart = 16 [default = true];
* @return The autostart.
*/
boolean getAutostart();
}
/**
*
*used to replay (concurrently) TM packets, parameters and events
*
*
* Protobuf type {@code yamcs.protobuf.ReplayRequest}
*/
public static final class ReplayRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.ReplayRequest)
ReplayRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReplayRequest.newBuilder() to construct.
private ReplayRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReplayRequest() {
endAction_ = 2;
autostart_ = true;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ReplayRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ReplayRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 24: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.EndAction value = org.yamcs.protobuf.Yamcs.EndAction.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
endAction_ = rawValue;
}
break;
}
case 34: {
org.yamcs.protobuf.Yamcs.ReplaySpeed.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) != 0)) {
subBuilder = speed_.toBuilder();
}
speed_ = input.readMessage(org.yamcs.protobuf.Yamcs.ReplaySpeed.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(speed_);
speed_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 66: {
org.yamcs.protobuf.Yamcs.ParameterReplayRequest.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) != 0)) {
subBuilder = parameterRequest_.toBuilder();
}
parameterRequest_ = input.readMessage(org.yamcs.protobuf.Yamcs.ParameterReplayRequest.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(parameterRequest_);
parameterRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 74: {
org.yamcs.protobuf.Yamcs.PacketReplayRequest.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) != 0)) {
subBuilder = packetRequest_.toBuilder();
}
packetRequest_ = input.readMessage(org.yamcs.protobuf.Yamcs.PacketReplayRequest.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(packetRequest_);
packetRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 82: {
org.yamcs.protobuf.Yamcs.EventReplayRequest.Builder subBuilder = null;
if (((bitField0_ & 0x00000080) != 0)) {
subBuilder = eventRequest_.toBuilder();
}
eventRequest_ = input.readMessage(org.yamcs.protobuf.Yamcs.EventReplayRequest.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(eventRequest_);
eventRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000080;
break;
}
case 90: {
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) != 0)) {
subBuilder = commandHistoryRequest_.toBuilder();
}
commandHistoryRequest_ = input.readMessage(org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(commandHistoryRequest_);
commandHistoryRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
case 98: {
org.yamcs.protobuf.Yamcs.PpReplayRequest.Builder subBuilder = null;
if (((bitField0_ & 0x00000200) != 0)) {
subBuilder = ppRequest_.toBuilder();
}
ppRequest_ = input.readMessage(org.yamcs.protobuf.Yamcs.PpReplayRequest.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(ppRequest_);
ppRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000200;
break;
}
case 106: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = start_.toBuilder();
}
start_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(start_);
start_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 114: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = stop_.toBuilder();
}
stop_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(stop_);
stop_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 120: {
bitField0_ |= 0x00000010;
reverse_ = input.readBool();
break;
}
case 128: {
bitField0_ |= 0x00000400;
autostart_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ReplayRequest.class, org.yamcs.protobuf.Yamcs.ReplayRequest.Builder.class);
}
private int bitField0_;
public static final int START_FIELD_NUMBER = 13;
private com.google.protobuf.Timestamp start_;
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
* @return Whether the start field is set.
*/
@java.lang.Override
public boolean hasStart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
* @return The start.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getStart() {
return start_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : start_;
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getStartOrBuilder() {
return start_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : start_;
}
public static final int STOP_FIELD_NUMBER = 14;
private com.google.protobuf.Timestamp stop_;
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
* @return Whether the stop field is set.
*/
@java.lang.Override
public boolean hasStop() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
* @return The stop.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getStop() {
return stop_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : stop_;
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getStopOrBuilder() {
return stop_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : stop_;
}
public static final int ENDACTION_FIELD_NUMBER = 3;
private int endAction_;
/**
*
*what should happen at the end of the replay
*
*
* optional .yamcs.protobuf.EndAction endAction = 3 [default = QUIT];
* @return Whether the endAction field is set.
*/
@java.lang.Override public boolean hasEndAction() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*what should happen at the end of the replay
*
*
* optional .yamcs.protobuf.EndAction endAction = 3 [default = QUIT];
* @return The endAction.
*/
@java.lang.Override public org.yamcs.protobuf.Yamcs.EndAction getEndAction() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.EndAction result = org.yamcs.protobuf.Yamcs.EndAction.valueOf(endAction_);
return result == null ? org.yamcs.protobuf.Yamcs.EndAction.QUIT : result;
}
public static final int SPEED_FIELD_NUMBER = 4;
private org.yamcs.protobuf.Yamcs.ReplaySpeed speed_;
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
* @return Whether the speed field is set.
*/
@java.lang.Override
public boolean hasSpeed() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
* @return The speed.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplaySpeed getSpeed() {
return speed_ == null ? org.yamcs.protobuf.Yamcs.ReplaySpeed.getDefaultInstance() : speed_;
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplaySpeedOrBuilder getSpeedOrBuilder() {
return speed_ == null ? org.yamcs.protobuf.Yamcs.ReplaySpeed.getDefaultInstance() : speed_;
}
public static final int REVERSE_FIELD_NUMBER = 15;
private boolean reverse_;
/**
*
* Reverse the direction of the replay
*
*
* optional bool reverse = 15;
* @return Whether the reverse field is set.
*/
@java.lang.Override
public boolean hasReverse() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Reverse the direction of the replay
*
*
* optional bool reverse = 15;
* @return The reverse.
*/
@java.lang.Override
public boolean getReverse() {
return reverse_;
}
public static final int PARAMETERREQUEST_FIELD_NUMBER = 8;
private org.yamcs.protobuf.Yamcs.ParameterReplayRequest parameterRequest_;
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
* @return Whether the parameterRequest field is set.
*/
@java.lang.Override
public boolean hasParameterRequest() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
* @return The parameterRequest.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ParameterReplayRequest getParameterRequest() {
return parameterRequest_ == null ? org.yamcs.protobuf.Yamcs.ParameterReplayRequest.getDefaultInstance() : parameterRequest_;
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ParameterReplayRequestOrBuilder getParameterRequestOrBuilder() {
return parameterRequest_ == null ? org.yamcs.protobuf.Yamcs.ParameterReplayRequest.getDefaultInstance() : parameterRequest_;
}
public static final int PACKETREQUEST_FIELD_NUMBER = 9;
private org.yamcs.protobuf.Yamcs.PacketReplayRequest packetRequest_;
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
* @return Whether the packetRequest field is set.
*/
@java.lang.Override
public boolean hasPacketRequest() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
* @return The packetRequest.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PacketReplayRequest getPacketRequest() {
return packetRequest_ == null ? org.yamcs.protobuf.Yamcs.PacketReplayRequest.getDefaultInstance() : packetRequest_;
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PacketReplayRequestOrBuilder getPacketRequestOrBuilder() {
return packetRequest_ == null ? org.yamcs.protobuf.Yamcs.PacketReplayRequest.getDefaultInstance() : packetRequest_;
}
public static final int EVENTREQUEST_FIELD_NUMBER = 10;
private org.yamcs.protobuf.Yamcs.EventReplayRequest eventRequest_;
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
* @return Whether the eventRequest field is set.
*/
@java.lang.Override
public boolean hasEventRequest() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
* @return The eventRequest.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.EventReplayRequest getEventRequest() {
return eventRequest_ == null ? org.yamcs.protobuf.Yamcs.EventReplayRequest.getDefaultInstance() : eventRequest_;
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.EventReplayRequestOrBuilder getEventRequestOrBuilder() {
return eventRequest_ == null ? org.yamcs.protobuf.Yamcs.EventReplayRequest.getDefaultInstance() : eventRequest_;
}
public static final int COMMANDHISTORYREQUEST_FIELD_NUMBER = 11;
private org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest commandHistoryRequest_;
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
* @return Whether the commandHistoryRequest field is set.
*/
@java.lang.Override
public boolean hasCommandHistoryRequest() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
* @return The commandHistoryRequest.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest getCommandHistoryRequest() {
return commandHistoryRequest_ == null ? org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.getDefaultInstance() : commandHistoryRequest_;
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequestOrBuilder getCommandHistoryRequestOrBuilder() {
return commandHistoryRequest_ == null ? org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.getDefaultInstance() : commandHistoryRequest_;
}
public static final int PPREQUEST_FIELD_NUMBER = 12;
private org.yamcs.protobuf.Yamcs.PpReplayRequest ppRequest_;
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
* @return Whether the ppRequest field is set.
*/
@java.lang.Override
public boolean hasPpRequest() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
* @return The ppRequest.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PpReplayRequest getPpRequest() {
return ppRequest_ == null ? org.yamcs.protobuf.Yamcs.PpReplayRequest.getDefaultInstance() : ppRequest_;
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PpReplayRequestOrBuilder getPpRequestOrBuilder() {
return ppRequest_ == null ? org.yamcs.protobuf.Yamcs.PpReplayRequest.getDefaultInstance() : ppRequest_;
}
public static final int AUTOSTART_FIELD_NUMBER = 16;
private boolean autostart_;
/**
*
* Start the replay following initialization
* Defaults to true, if unspecified
*
*
* optional bool autostart = 16 [default = true];
* @return Whether the autostart field is set.
*/
@java.lang.Override
public boolean hasAutostart() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Start the replay following initialization
* Defaults to true, if unspecified
*
*
* optional bool autostart = 16 [default = true];
* @return The autostart.
*/
@java.lang.Override
public boolean getAutostart() {
return autostart_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasSpeed()) {
if (!getSpeed().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasParameterRequest()) {
if (!getParameterRequest().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasPacketRequest()) {
if (!getPacketRequest().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasCommandHistoryRequest()) {
if (!getCommandHistoryRequest().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000004) != 0)) {
output.writeEnum(3, endAction_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(4, getSpeed());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(8, getParameterRequest());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(9, getPacketRequest());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(10, getEventRequest());
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeMessage(11, getCommandHistoryRequest());
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeMessage(12, getPpRequest());
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(13, getStart());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(14, getStop());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBool(15, reverse_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeBool(16, autostart_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, endAction_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getSpeed());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getParameterRequest());
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getPacketRequest());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getEventRequest());
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getCommandHistoryRequest());
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getPpRequest());
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getStart());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getStop());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, reverse_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(16, autostart_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.ReplayRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.ReplayRequest other = (org.yamcs.protobuf.Yamcs.ReplayRequest) obj;
if (hasStart() != other.hasStart()) return false;
if (hasStart()) {
if (!getStart()
.equals(other.getStart())) return false;
}
if (hasStop() != other.hasStop()) return false;
if (hasStop()) {
if (!getStop()
.equals(other.getStop())) return false;
}
if (hasEndAction() != other.hasEndAction()) return false;
if (hasEndAction()) {
if (endAction_ != other.endAction_) return false;
}
if (hasSpeed() != other.hasSpeed()) return false;
if (hasSpeed()) {
if (!getSpeed()
.equals(other.getSpeed())) return false;
}
if (hasReverse() != other.hasReverse()) return false;
if (hasReverse()) {
if (getReverse()
!= other.getReverse()) return false;
}
if (hasParameterRequest() != other.hasParameterRequest()) return false;
if (hasParameterRequest()) {
if (!getParameterRequest()
.equals(other.getParameterRequest())) return false;
}
if (hasPacketRequest() != other.hasPacketRequest()) return false;
if (hasPacketRequest()) {
if (!getPacketRequest()
.equals(other.getPacketRequest())) return false;
}
if (hasEventRequest() != other.hasEventRequest()) return false;
if (hasEventRequest()) {
if (!getEventRequest()
.equals(other.getEventRequest())) return false;
}
if (hasCommandHistoryRequest() != other.hasCommandHistoryRequest()) return false;
if (hasCommandHistoryRequest()) {
if (!getCommandHistoryRequest()
.equals(other.getCommandHistoryRequest())) return false;
}
if (hasPpRequest() != other.hasPpRequest()) return false;
if (hasPpRequest()) {
if (!getPpRequest()
.equals(other.getPpRequest())) return false;
}
if (hasAutostart() != other.hasAutostart()) return false;
if (hasAutostart()) {
if (getAutostart()
!= other.getAutostart()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStart()) {
hash = (37 * hash) + START_FIELD_NUMBER;
hash = (53 * hash) + getStart().hashCode();
}
if (hasStop()) {
hash = (37 * hash) + STOP_FIELD_NUMBER;
hash = (53 * hash) + getStop().hashCode();
}
if (hasEndAction()) {
hash = (37 * hash) + ENDACTION_FIELD_NUMBER;
hash = (53 * hash) + endAction_;
}
if (hasSpeed()) {
hash = (37 * hash) + SPEED_FIELD_NUMBER;
hash = (53 * hash) + getSpeed().hashCode();
}
if (hasReverse()) {
hash = (37 * hash) + REVERSE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getReverse());
}
if (hasParameterRequest()) {
hash = (37 * hash) + PARAMETERREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getParameterRequest().hashCode();
}
if (hasPacketRequest()) {
hash = (37 * hash) + PACKETREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getPacketRequest().hashCode();
}
if (hasEventRequest()) {
hash = (37 * hash) + EVENTREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getEventRequest().hashCode();
}
if (hasCommandHistoryRequest()) {
hash = (37 * hash) + COMMANDHISTORYREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getCommandHistoryRequest().hashCode();
}
if (hasPpRequest()) {
hash = (37 * hash) + PPREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getPpRequest().hashCode();
}
if (hasAutostart()) {
hash = (37 * hash) + AUTOSTART_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAutostart());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.ReplayRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*used to replay (concurrently) TM packets, parameters and events
*
*
* Protobuf type {@code yamcs.protobuf.ReplayRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.ReplayRequest)
org.yamcs.protobuf.Yamcs.ReplayRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ReplayRequest.class, org.yamcs.protobuf.Yamcs.ReplayRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.ReplayRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStartFieldBuilder();
getStopFieldBuilder();
getSpeedFieldBuilder();
getParameterRequestFieldBuilder();
getPacketRequestFieldBuilder();
getEventRequestFieldBuilder();
getCommandHistoryRequestFieldBuilder();
getPpRequestFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (startBuilder_ == null) {
start_ = null;
} else {
startBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (stopBuilder_ == null) {
stop_ = null;
} else {
stopBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
endAction_ = 2;
bitField0_ = (bitField0_ & ~0x00000004);
if (speedBuilder_ == null) {
speed_ = null;
} else {
speedBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
reverse_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
if (parameterRequestBuilder_ == null) {
parameterRequest_ = null;
} else {
parameterRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (packetRequestBuilder_ == null) {
packetRequest_ = null;
} else {
packetRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
if (eventRequestBuilder_ == null) {
eventRequest_ = null;
} else {
eventRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
if (commandHistoryRequestBuilder_ == null) {
commandHistoryRequest_ = null;
} else {
commandHistoryRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
if (ppRequestBuilder_ == null) {
ppRequest_ = null;
} else {
ppRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
autostart_ = true;
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.ReplayRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayRequest build() {
org.yamcs.protobuf.Yamcs.ReplayRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayRequest buildPartial() {
org.yamcs.protobuf.Yamcs.ReplayRequest result = new org.yamcs.protobuf.Yamcs.ReplayRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
if (startBuilder_ == null) {
result.start_ = start_;
} else {
result.start_ = startBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
if (stopBuilder_ == null) {
result.stop_ = stop_;
} else {
result.stop_ = stopBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.endAction_ = endAction_;
if (((from_bitField0_ & 0x00000008) != 0)) {
if (speedBuilder_ == null) {
result.speed_ = speed_;
} else {
result.speed_ = speedBuilder_.build();
}
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.reverse_ = reverse_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
if (parameterRequestBuilder_ == null) {
result.parameterRequest_ = parameterRequest_;
} else {
result.parameterRequest_ = parameterRequestBuilder_.build();
}
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
if (packetRequestBuilder_ == null) {
result.packetRequest_ = packetRequest_;
} else {
result.packetRequest_ = packetRequestBuilder_.build();
}
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
if (eventRequestBuilder_ == null) {
result.eventRequest_ = eventRequest_;
} else {
result.eventRequest_ = eventRequestBuilder_.build();
}
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
if (commandHistoryRequestBuilder_ == null) {
result.commandHistoryRequest_ = commandHistoryRequest_;
} else {
result.commandHistoryRequest_ = commandHistoryRequestBuilder_.build();
}
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
if (ppRequestBuilder_ == null) {
result.ppRequest_ = ppRequest_;
} else {
result.ppRequest_ = ppRequestBuilder_.build();
}
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
to_bitField0_ |= 0x00000400;
}
result.autostart_ = autostart_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.ReplayRequest) {
return mergeFrom((org.yamcs.protobuf.Yamcs.ReplayRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.ReplayRequest other) {
if (other == org.yamcs.protobuf.Yamcs.ReplayRequest.getDefaultInstance()) return this;
if (other.hasStart()) {
mergeStart(other.getStart());
}
if (other.hasStop()) {
mergeStop(other.getStop());
}
if (other.hasEndAction()) {
setEndAction(other.getEndAction());
}
if (other.hasSpeed()) {
mergeSpeed(other.getSpeed());
}
if (other.hasReverse()) {
setReverse(other.getReverse());
}
if (other.hasParameterRequest()) {
mergeParameterRequest(other.getParameterRequest());
}
if (other.hasPacketRequest()) {
mergePacketRequest(other.getPacketRequest());
}
if (other.hasEventRequest()) {
mergeEventRequest(other.getEventRequest());
}
if (other.hasCommandHistoryRequest()) {
mergeCommandHistoryRequest(other.getCommandHistoryRequest());
}
if (other.hasPpRequest()) {
mergePpRequest(other.getPpRequest());
}
if (other.hasAutostart()) {
setAutostart(other.getAutostart());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasSpeed()) {
if (!getSpeed().isInitialized()) {
return false;
}
}
if (hasParameterRequest()) {
if (!getParameterRequest().isInitialized()) {
return false;
}
}
if (hasPacketRequest()) {
if (!getPacketRequest().isInitialized()) {
return false;
}
}
if (hasCommandHistoryRequest()) {
if (!getCommandHistoryRequest().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.ReplayRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.ReplayRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.Timestamp start_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> startBuilder_;
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
* @return Whether the start field is set.
*/
public boolean hasStart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
* @return The start.
*/
public com.google.protobuf.Timestamp getStart() {
if (startBuilder_ == null) {
return start_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : start_;
} else {
return startBuilder_.getMessage();
}
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
*/
public Builder setStart(com.google.protobuf.Timestamp value) {
if (startBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
start_ = value;
onChanged();
} else {
startBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
*/
public Builder setStart(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (startBuilder_ == null) {
start_ = builderForValue.build();
onChanged();
} else {
startBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
*/
public Builder mergeStart(com.google.protobuf.Timestamp value) {
if (startBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
start_ != null &&
start_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
start_ =
com.google.protobuf.Timestamp.newBuilder(start_).mergeFrom(value).buildPartial();
} else {
start_ = value;
}
onChanged();
} else {
startBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
*/
public Builder clearStart() {
if (startBuilder_ == null) {
start_ = null;
onChanged();
} else {
startBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
*/
public com.google.protobuf.Timestamp.Builder getStartBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getStartFieldBuilder().getBuilder();
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
*/
public com.google.protobuf.TimestampOrBuilder getStartOrBuilder() {
if (startBuilder_ != null) {
return startBuilder_.getMessageOrBuilder();
} else {
return start_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : start_;
}
}
/**
*
* **Required.** The time at which the replay should start.
*
*
* optional .google.protobuf.Timestamp start = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getStartFieldBuilder() {
if (startBuilder_ == null) {
startBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getStart(),
getParentForChildren(),
isClean());
start_ = null;
}
return startBuilder_;
}
private com.google.protobuf.Timestamp stop_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> stopBuilder_;
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
* @return Whether the stop field is set.
*/
public boolean hasStop() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
* @return The stop.
*/
public com.google.protobuf.Timestamp getStop() {
if (stopBuilder_ == null) {
return stop_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : stop_;
} else {
return stopBuilder_.getMessage();
}
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
*/
public Builder setStop(com.google.protobuf.Timestamp value) {
if (stopBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
stop_ = value;
onChanged();
} else {
stopBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
*/
public Builder setStop(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (stopBuilder_ == null) {
stop_ = builderForValue.build();
onChanged();
} else {
stopBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
*/
public Builder mergeStop(com.google.protobuf.Timestamp value) {
if (stopBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
stop_ != null &&
stop_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
stop_ =
com.google.protobuf.Timestamp.newBuilder(stop_).mergeFrom(value).buildPartial();
} else {
stop_ = value;
}
onChanged();
} else {
stopBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
*/
public Builder clearStop() {
if (stopBuilder_ == null) {
stop_ = null;
onChanged();
} else {
stopBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
*/
public com.google.protobuf.Timestamp.Builder getStopBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getStopFieldBuilder().getBuilder();
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
*/
public com.google.protobuf.TimestampOrBuilder getStopOrBuilder() {
if (stopBuilder_ != null) {
return stopBuilder_.getMessageOrBuilder();
} else {
return stop_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : stop_;
}
}
/**
*
* The time at which the replay should stop.
* If unspecified, the replay will keep going as long as there is remaining data.
*
*
* optional .google.protobuf.Timestamp stop = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getStopFieldBuilder() {
if (stopBuilder_ == null) {
stopBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getStop(),
getParentForChildren(),
isClean());
stop_ = null;
}
return stopBuilder_;
}
private int endAction_ = 2;
/**
*
*what should happen at the end of the replay
*
*
* optional .yamcs.protobuf.EndAction endAction = 3 [default = QUIT];
* @return Whether the endAction field is set.
*/
@java.lang.Override public boolean hasEndAction() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*what should happen at the end of the replay
*
*
* optional .yamcs.protobuf.EndAction endAction = 3 [default = QUIT];
* @return The endAction.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.EndAction getEndAction() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.EndAction result = org.yamcs.protobuf.Yamcs.EndAction.valueOf(endAction_);
return result == null ? org.yamcs.protobuf.Yamcs.EndAction.QUIT : result;
}
/**
*
*what should happen at the end of the replay
*
*
* optional .yamcs.protobuf.EndAction endAction = 3 [default = QUIT];
* @param value The endAction to set.
* @return This builder for chaining.
*/
public Builder setEndAction(org.yamcs.protobuf.Yamcs.EndAction value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
endAction_ = value.getNumber();
onChanged();
return this;
}
/**
*
*what should happen at the end of the replay
*
*
* optional .yamcs.protobuf.EndAction endAction = 3 [default = QUIT];
* @return This builder for chaining.
*/
public Builder clearEndAction() {
bitField0_ = (bitField0_ & ~0x00000004);
endAction_ = 2;
onChanged();
return this;
}
private org.yamcs.protobuf.Yamcs.ReplaySpeed speed_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.ReplaySpeed, org.yamcs.protobuf.Yamcs.ReplaySpeed.Builder, org.yamcs.protobuf.Yamcs.ReplaySpeedOrBuilder> speedBuilder_;
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
* @return Whether the speed field is set.
*/
public boolean hasSpeed() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
* @return The speed.
*/
public org.yamcs.protobuf.Yamcs.ReplaySpeed getSpeed() {
if (speedBuilder_ == null) {
return speed_ == null ? org.yamcs.protobuf.Yamcs.ReplaySpeed.getDefaultInstance() : speed_;
} else {
return speedBuilder_.getMessage();
}
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
*/
public Builder setSpeed(org.yamcs.protobuf.Yamcs.ReplaySpeed value) {
if (speedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
speed_ = value;
onChanged();
} else {
speedBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
*/
public Builder setSpeed(
org.yamcs.protobuf.Yamcs.ReplaySpeed.Builder builderForValue) {
if (speedBuilder_ == null) {
speed_ = builderForValue.build();
onChanged();
} else {
speedBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
*/
public Builder mergeSpeed(org.yamcs.protobuf.Yamcs.ReplaySpeed value) {
if (speedBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
speed_ != null &&
speed_ != org.yamcs.protobuf.Yamcs.ReplaySpeed.getDefaultInstance()) {
speed_ =
org.yamcs.protobuf.Yamcs.ReplaySpeed.newBuilder(speed_).mergeFrom(value).buildPartial();
} else {
speed_ = value;
}
onChanged();
} else {
speedBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
*/
public Builder clearSpeed() {
if (speedBuilder_ == null) {
speed_ = null;
onChanged();
} else {
speedBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
*/
public org.yamcs.protobuf.Yamcs.ReplaySpeed.Builder getSpeedBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getSpeedFieldBuilder().getBuilder();
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
*/
public org.yamcs.protobuf.Yamcs.ReplaySpeedOrBuilder getSpeedOrBuilder() {
if (speedBuilder_ != null) {
return speedBuilder_.getMessageOrBuilder();
} else {
return speed_ == null ?
org.yamcs.protobuf.Yamcs.ReplaySpeed.getDefaultInstance() : speed_;
}
}
/**
*
*how fast the replay should go
*
*
* optional .yamcs.protobuf.ReplaySpeed speed = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.ReplaySpeed, org.yamcs.protobuf.Yamcs.ReplaySpeed.Builder, org.yamcs.protobuf.Yamcs.ReplaySpeedOrBuilder>
getSpeedFieldBuilder() {
if (speedBuilder_ == null) {
speedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.ReplaySpeed, org.yamcs.protobuf.Yamcs.ReplaySpeed.Builder, org.yamcs.protobuf.Yamcs.ReplaySpeedOrBuilder>(
getSpeed(),
getParentForChildren(),
isClean());
speed_ = null;
}
return speedBuilder_;
}
private boolean reverse_ ;
/**
*
* Reverse the direction of the replay
*
*
* optional bool reverse = 15;
* @return Whether the reverse field is set.
*/
@java.lang.Override
public boolean hasReverse() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Reverse the direction of the replay
*
*
* optional bool reverse = 15;
* @return The reverse.
*/
@java.lang.Override
public boolean getReverse() {
return reverse_;
}
/**
*
* Reverse the direction of the replay
*
*
* optional bool reverse = 15;
* @param value The reverse to set.
* @return This builder for chaining.
*/
public Builder setReverse(boolean value) {
bitField0_ |= 0x00000010;
reverse_ = value;
onChanged();
return this;
}
/**
*
* Reverse the direction of the replay
*
*
* optional bool reverse = 15;
* @return This builder for chaining.
*/
public Builder clearReverse() {
bitField0_ = (bitField0_ & ~0x00000010);
reverse_ = false;
onChanged();
return this;
}
private org.yamcs.protobuf.Yamcs.ParameterReplayRequest parameterRequest_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.ParameterReplayRequest, org.yamcs.protobuf.Yamcs.ParameterReplayRequest.Builder, org.yamcs.protobuf.Yamcs.ParameterReplayRequestOrBuilder> parameterRequestBuilder_;
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
* @return Whether the parameterRequest field is set.
*/
public boolean hasParameterRequest() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
* @return The parameterRequest.
*/
public org.yamcs.protobuf.Yamcs.ParameterReplayRequest getParameterRequest() {
if (parameterRequestBuilder_ == null) {
return parameterRequest_ == null ? org.yamcs.protobuf.Yamcs.ParameterReplayRequest.getDefaultInstance() : parameterRequest_;
} else {
return parameterRequestBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
*/
public Builder setParameterRequest(org.yamcs.protobuf.Yamcs.ParameterReplayRequest value) {
if (parameterRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
parameterRequest_ = value;
onChanged();
} else {
parameterRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
*/
public Builder setParameterRequest(
org.yamcs.protobuf.Yamcs.ParameterReplayRequest.Builder builderForValue) {
if (parameterRequestBuilder_ == null) {
parameterRequest_ = builderForValue.build();
onChanged();
} else {
parameterRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
*/
public Builder mergeParameterRequest(org.yamcs.protobuf.Yamcs.ParameterReplayRequest value) {
if (parameterRequestBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
parameterRequest_ != null &&
parameterRequest_ != org.yamcs.protobuf.Yamcs.ParameterReplayRequest.getDefaultInstance()) {
parameterRequest_ =
org.yamcs.protobuf.Yamcs.ParameterReplayRequest.newBuilder(parameterRequest_).mergeFrom(value).buildPartial();
} else {
parameterRequest_ = value;
}
onChanged();
} else {
parameterRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
*/
public Builder clearParameterRequest() {
if (parameterRequestBuilder_ == null) {
parameterRequest_ = null;
onChanged();
} else {
parameterRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
*/
public org.yamcs.protobuf.Yamcs.ParameterReplayRequest.Builder getParameterRequestBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getParameterRequestFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
*/
public org.yamcs.protobuf.Yamcs.ParameterReplayRequestOrBuilder getParameterRequestOrBuilder() {
if (parameterRequestBuilder_ != null) {
return parameterRequestBuilder_.getMessageOrBuilder();
} else {
return parameterRequest_ == null ?
org.yamcs.protobuf.Yamcs.ParameterReplayRequest.getDefaultInstance() : parameterRequest_;
}
}
/**
* optional .yamcs.protobuf.ParameterReplayRequest parameterRequest = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.ParameterReplayRequest, org.yamcs.protobuf.Yamcs.ParameterReplayRequest.Builder, org.yamcs.protobuf.Yamcs.ParameterReplayRequestOrBuilder>
getParameterRequestFieldBuilder() {
if (parameterRequestBuilder_ == null) {
parameterRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.ParameterReplayRequest, org.yamcs.protobuf.Yamcs.ParameterReplayRequest.Builder, org.yamcs.protobuf.Yamcs.ParameterReplayRequestOrBuilder>(
getParameterRequest(),
getParentForChildren(),
isClean());
parameterRequest_ = null;
}
return parameterRequestBuilder_;
}
private org.yamcs.protobuf.Yamcs.PacketReplayRequest packetRequest_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.PacketReplayRequest, org.yamcs.protobuf.Yamcs.PacketReplayRequest.Builder, org.yamcs.protobuf.Yamcs.PacketReplayRequestOrBuilder> packetRequestBuilder_;
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
* @return Whether the packetRequest field is set.
*/
public boolean hasPacketRequest() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
* @return The packetRequest.
*/
public org.yamcs.protobuf.Yamcs.PacketReplayRequest getPacketRequest() {
if (packetRequestBuilder_ == null) {
return packetRequest_ == null ? org.yamcs.protobuf.Yamcs.PacketReplayRequest.getDefaultInstance() : packetRequest_;
} else {
return packetRequestBuilder_.getMessage();
}
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
*/
public Builder setPacketRequest(org.yamcs.protobuf.Yamcs.PacketReplayRequest value) {
if (packetRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
packetRequest_ = value;
onChanged();
} else {
packetRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
*/
public Builder setPacketRequest(
org.yamcs.protobuf.Yamcs.PacketReplayRequest.Builder builderForValue) {
if (packetRequestBuilder_ == null) {
packetRequest_ = builderForValue.build();
onChanged();
} else {
packetRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
*/
public Builder mergePacketRequest(org.yamcs.protobuf.Yamcs.PacketReplayRequest value) {
if (packetRequestBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
packetRequest_ != null &&
packetRequest_ != org.yamcs.protobuf.Yamcs.PacketReplayRequest.getDefaultInstance()) {
packetRequest_ =
org.yamcs.protobuf.Yamcs.PacketReplayRequest.newBuilder(packetRequest_).mergeFrom(value).buildPartial();
} else {
packetRequest_ = value;
}
onChanged();
} else {
packetRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
*/
public Builder clearPacketRequest() {
if (packetRequestBuilder_ == null) {
packetRequest_ = null;
onChanged();
} else {
packetRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
*/
public org.yamcs.protobuf.Yamcs.PacketReplayRequest.Builder getPacketRequestBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getPacketRequestFieldBuilder().getBuilder();
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
*/
public org.yamcs.protobuf.Yamcs.PacketReplayRequestOrBuilder getPacketRequestOrBuilder() {
if (packetRequestBuilder_ != null) {
return packetRequestBuilder_.getMessageOrBuilder();
} else {
return packetRequest_ == null ?
org.yamcs.protobuf.Yamcs.PacketReplayRequest.getDefaultInstance() : packetRequest_;
}
}
/**
*
* By default all Packets, Events, CommandHistory are part of the replay
* Unless one or more of the below requests are specified.
*
*
* optional .yamcs.protobuf.PacketReplayRequest packetRequest = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.PacketReplayRequest, org.yamcs.protobuf.Yamcs.PacketReplayRequest.Builder, org.yamcs.protobuf.Yamcs.PacketReplayRequestOrBuilder>
getPacketRequestFieldBuilder() {
if (packetRequestBuilder_ == null) {
packetRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.PacketReplayRequest, org.yamcs.protobuf.Yamcs.PacketReplayRequest.Builder, org.yamcs.protobuf.Yamcs.PacketReplayRequestOrBuilder>(
getPacketRequest(),
getParentForChildren(),
isClean());
packetRequest_ = null;
}
return packetRequestBuilder_;
}
private org.yamcs.protobuf.Yamcs.EventReplayRequest eventRequest_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.EventReplayRequest, org.yamcs.protobuf.Yamcs.EventReplayRequest.Builder, org.yamcs.protobuf.Yamcs.EventReplayRequestOrBuilder> eventRequestBuilder_;
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
* @return Whether the eventRequest field is set.
*/
public boolean hasEventRequest() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
* @return The eventRequest.
*/
public org.yamcs.protobuf.Yamcs.EventReplayRequest getEventRequest() {
if (eventRequestBuilder_ == null) {
return eventRequest_ == null ? org.yamcs.protobuf.Yamcs.EventReplayRequest.getDefaultInstance() : eventRequest_;
} else {
return eventRequestBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
*/
public Builder setEventRequest(org.yamcs.protobuf.Yamcs.EventReplayRequest value) {
if (eventRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
eventRequest_ = value;
onChanged();
} else {
eventRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
*/
public Builder setEventRequest(
org.yamcs.protobuf.Yamcs.EventReplayRequest.Builder builderForValue) {
if (eventRequestBuilder_ == null) {
eventRequest_ = builderForValue.build();
onChanged();
} else {
eventRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
*/
public Builder mergeEventRequest(org.yamcs.protobuf.Yamcs.EventReplayRequest value) {
if (eventRequestBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
eventRequest_ != null &&
eventRequest_ != org.yamcs.protobuf.Yamcs.EventReplayRequest.getDefaultInstance()) {
eventRequest_ =
org.yamcs.protobuf.Yamcs.EventReplayRequest.newBuilder(eventRequest_).mergeFrom(value).buildPartial();
} else {
eventRequest_ = value;
}
onChanged();
} else {
eventRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
*/
public Builder clearEventRequest() {
if (eventRequestBuilder_ == null) {
eventRequest_ = null;
onChanged();
} else {
eventRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
*/
public org.yamcs.protobuf.Yamcs.EventReplayRequest.Builder getEventRequestBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getEventRequestFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
*/
public org.yamcs.protobuf.Yamcs.EventReplayRequestOrBuilder getEventRequestOrBuilder() {
if (eventRequestBuilder_ != null) {
return eventRequestBuilder_.getMessageOrBuilder();
} else {
return eventRequest_ == null ?
org.yamcs.protobuf.Yamcs.EventReplayRequest.getDefaultInstance() : eventRequest_;
}
}
/**
* optional .yamcs.protobuf.EventReplayRequest eventRequest = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.EventReplayRequest, org.yamcs.protobuf.Yamcs.EventReplayRequest.Builder, org.yamcs.protobuf.Yamcs.EventReplayRequestOrBuilder>
getEventRequestFieldBuilder() {
if (eventRequestBuilder_ == null) {
eventRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.EventReplayRequest, org.yamcs.protobuf.Yamcs.EventReplayRequest.Builder, org.yamcs.protobuf.Yamcs.EventReplayRequestOrBuilder>(
getEventRequest(),
getParentForChildren(),
isClean());
eventRequest_ = null;
}
return eventRequestBuilder_;
}
private org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest commandHistoryRequest_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest, org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.Builder, org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequestOrBuilder> commandHistoryRequestBuilder_;
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
* @return Whether the commandHistoryRequest field is set.
*/
public boolean hasCommandHistoryRequest() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
* @return The commandHistoryRequest.
*/
public org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest getCommandHistoryRequest() {
if (commandHistoryRequestBuilder_ == null) {
return commandHistoryRequest_ == null ? org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.getDefaultInstance() : commandHistoryRequest_;
} else {
return commandHistoryRequestBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
*/
public Builder setCommandHistoryRequest(org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest value) {
if (commandHistoryRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
commandHistoryRequest_ = value;
onChanged();
} else {
commandHistoryRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
*/
public Builder setCommandHistoryRequest(
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.Builder builderForValue) {
if (commandHistoryRequestBuilder_ == null) {
commandHistoryRequest_ = builderForValue.build();
onChanged();
} else {
commandHistoryRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
*/
public Builder mergeCommandHistoryRequest(org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest value) {
if (commandHistoryRequestBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0) &&
commandHistoryRequest_ != null &&
commandHistoryRequest_ != org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.getDefaultInstance()) {
commandHistoryRequest_ =
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.newBuilder(commandHistoryRequest_).mergeFrom(value).buildPartial();
} else {
commandHistoryRequest_ = value;
}
onChanged();
} else {
commandHistoryRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
*/
public Builder clearCommandHistoryRequest() {
if (commandHistoryRequestBuilder_ == null) {
commandHistoryRequest_ = null;
onChanged();
} else {
commandHistoryRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
*/
public org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.Builder getCommandHistoryRequestBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getCommandHistoryRequestFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
*/
public org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequestOrBuilder getCommandHistoryRequestOrBuilder() {
if (commandHistoryRequestBuilder_ != null) {
return commandHistoryRequestBuilder_.getMessageOrBuilder();
} else {
return commandHistoryRequest_ == null ?
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.getDefaultInstance() : commandHistoryRequest_;
}
}
/**
* optional .yamcs.protobuf.CommandHistoryReplayRequest commandHistoryRequest = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest, org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.Builder, org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequestOrBuilder>
getCommandHistoryRequestFieldBuilder() {
if (commandHistoryRequestBuilder_ == null) {
commandHistoryRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest, org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.Builder, org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequestOrBuilder>(
getCommandHistoryRequest(),
getParentForChildren(),
isClean());
commandHistoryRequest_ = null;
}
return commandHistoryRequestBuilder_;
}
private org.yamcs.protobuf.Yamcs.PpReplayRequest ppRequest_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.PpReplayRequest, org.yamcs.protobuf.Yamcs.PpReplayRequest.Builder, org.yamcs.protobuf.Yamcs.PpReplayRequestOrBuilder> ppRequestBuilder_;
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
* @return Whether the ppRequest field is set.
*/
public boolean hasPpRequest() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
* @return The ppRequest.
*/
public org.yamcs.protobuf.Yamcs.PpReplayRequest getPpRequest() {
if (ppRequestBuilder_ == null) {
return ppRequest_ == null ? org.yamcs.protobuf.Yamcs.PpReplayRequest.getDefaultInstance() : ppRequest_;
} else {
return ppRequestBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
*/
public Builder setPpRequest(org.yamcs.protobuf.Yamcs.PpReplayRequest value) {
if (ppRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ppRequest_ = value;
onChanged();
} else {
ppRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
*/
public Builder setPpRequest(
org.yamcs.protobuf.Yamcs.PpReplayRequest.Builder builderForValue) {
if (ppRequestBuilder_ == null) {
ppRequest_ = builderForValue.build();
onChanged();
} else {
ppRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
*/
public Builder mergePpRequest(org.yamcs.protobuf.Yamcs.PpReplayRequest value) {
if (ppRequestBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0) &&
ppRequest_ != null &&
ppRequest_ != org.yamcs.protobuf.Yamcs.PpReplayRequest.getDefaultInstance()) {
ppRequest_ =
org.yamcs.protobuf.Yamcs.PpReplayRequest.newBuilder(ppRequest_).mergeFrom(value).buildPartial();
} else {
ppRequest_ = value;
}
onChanged();
} else {
ppRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
*/
public Builder clearPpRequest() {
if (ppRequestBuilder_ == null) {
ppRequest_ = null;
onChanged();
} else {
ppRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
*/
public org.yamcs.protobuf.Yamcs.PpReplayRequest.Builder getPpRequestBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getPpRequestFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
*/
public org.yamcs.protobuf.Yamcs.PpReplayRequestOrBuilder getPpRequestOrBuilder() {
if (ppRequestBuilder_ != null) {
return ppRequestBuilder_.getMessageOrBuilder();
} else {
return ppRequest_ == null ?
org.yamcs.protobuf.Yamcs.PpReplayRequest.getDefaultInstance() : ppRequest_;
}
}
/**
* optional .yamcs.protobuf.PpReplayRequest ppRequest = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.PpReplayRequest, org.yamcs.protobuf.Yamcs.PpReplayRequest.Builder, org.yamcs.protobuf.Yamcs.PpReplayRequestOrBuilder>
getPpRequestFieldBuilder() {
if (ppRequestBuilder_ == null) {
ppRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.PpReplayRequest, org.yamcs.protobuf.Yamcs.PpReplayRequest.Builder, org.yamcs.protobuf.Yamcs.PpReplayRequestOrBuilder>(
getPpRequest(),
getParentForChildren(),
isClean());
ppRequest_ = null;
}
return ppRequestBuilder_;
}
private boolean autostart_ = true;
/**
*
* Start the replay following initialization
* Defaults to true, if unspecified
*
*
* optional bool autostart = 16 [default = true];
* @return Whether the autostart field is set.
*/
@java.lang.Override
public boolean hasAutostart() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Start the replay following initialization
* Defaults to true, if unspecified
*
*
* optional bool autostart = 16 [default = true];
* @return The autostart.
*/
@java.lang.Override
public boolean getAutostart() {
return autostart_;
}
/**
*
* Start the replay following initialization
* Defaults to true, if unspecified
*
*
* optional bool autostart = 16 [default = true];
* @param value The autostart to set.
* @return This builder for chaining.
*/
public Builder setAutostart(boolean value) {
bitField0_ |= 0x00000400;
autostart_ = value;
onChanged();
return this;
}
/**
*
* Start the replay following initialization
* Defaults to true, if unspecified
*
*
* optional bool autostart = 16 [default = true];
* @return This builder for chaining.
*/
public Builder clearAutostart() {
bitField0_ = (bitField0_ & ~0x00000400);
autostart_ = true;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.ReplayRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.ReplayRequest)
private static final org.yamcs.protobuf.Yamcs.ReplayRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.ReplayRequest();
}
public static org.yamcs.protobuf.Yamcs.ReplayRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ReplayRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ReplayRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ParameterReplayRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.ParameterReplayRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
java.util.List
getNameFilterList();
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
org.yamcs.protobuf.Yamcs.NamedObjectId getNameFilter(int index);
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
int getNameFilterCount();
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterOrBuilderList();
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getNameFilterOrBuilder(
int index);
/**
* optional bool sendRaw = 2 [default = false];
* @return Whether the sendRaw field is set.
*/
boolean hasSendRaw();
/**
* optional bool sendRaw = 2 [default = false];
* @return The sendRaw.
*/
boolean getSendRaw();
/**
*
*i.e. out of limit checking
*
*
* optional bool performMonitoring = 3 [default = false];
* @return Whether the performMonitoring field is set.
*/
boolean hasPerformMonitoring();
/**
*
*i.e. out of limit checking
*
*
* optional bool performMonitoring = 3 [default = false];
* @return The performMonitoring.
*/
boolean getPerformMonitoring();
}
/**
* Protobuf type {@code yamcs.protobuf.ParameterReplayRequest}
*/
public static final class ParameterReplayRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.ParameterReplayRequest)
ParameterReplayRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ParameterReplayRequest.newBuilder() to construct.
private ParameterReplayRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ParameterReplayRequest() {
nameFilter_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ParameterReplayRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ParameterReplayRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
nameFilter_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
nameFilter_.add(
input.readMessage(org.yamcs.protobuf.Yamcs.NamedObjectId.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
sendRaw_ = input.readBool();
break;
}
case 24: {
bitField0_ |= 0x00000002;
performMonitoring_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
nameFilter_ = java.util.Collections.unmodifiableList(nameFilter_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ParameterReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ParameterReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ParameterReplayRequest.class, org.yamcs.protobuf.Yamcs.ParameterReplayRequest.Builder.class);
}
private int bitField0_;
public static final int NAMEFILTER_FIELD_NUMBER = 1;
private java.util.List nameFilter_;
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public java.util.List getNameFilterList() {
return nameFilter_;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterOrBuilderList() {
return nameFilter_;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public int getNameFilterCount() {
return nameFilter_.size();
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectId getNameFilter(int index) {
return nameFilter_.get(index);
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getNameFilterOrBuilder(
int index) {
return nameFilter_.get(index);
}
public static final int SENDRAW_FIELD_NUMBER = 2;
private boolean sendRaw_;
/**
* optional bool sendRaw = 2 [default = false];
* @return Whether the sendRaw field is set.
*/
@java.lang.Override
public boolean hasSendRaw() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bool sendRaw = 2 [default = false];
* @return The sendRaw.
*/
@java.lang.Override
public boolean getSendRaw() {
return sendRaw_;
}
public static final int PERFORMMONITORING_FIELD_NUMBER = 3;
private boolean performMonitoring_;
/**
*
*i.e. out of limit checking
*
*
* optional bool performMonitoring = 3 [default = false];
* @return Whether the performMonitoring field is set.
*/
@java.lang.Override
public boolean hasPerformMonitoring() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*i.e. out of limit checking
*
*
* optional bool performMonitoring = 3 [default = false];
* @return The performMonitoring.
*/
@java.lang.Override
public boolean getPerformMonitoring() {
return performMonitoring_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getNameFilterCount(); i++) {
if (!getNameFilter(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < nameFilter_.size(); i++) {
output.writeMessage(1, nameFilter_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBool(2, sendRaw_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(3, performMonitoring_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < nameFilter_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, nameFilter_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, sendRaw_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, performMonitoring_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.ParameterReplayRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.ParameterReplayRequest other = (org.yamcs.protobuf.Yamcs.ParameterReplayRequest) obj;
if (!getNameFilterList()
.equals(other.getNameFilterList())) return false;
if (hasSendRaw() != other.hasSendRaw()) return false;
if (hasSendRaw()) {
if (getSendRaw()
!= other.getSendRaw()) return false;
}
if (hasPerformMonitoring() != other.hasPerformMonitoring()) return false;
if (hasPerformMonitoring()) {
if (getPerformMonitoring()
!= other.getPerformMonitoring()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getNameFilterCount() > 0) {
hash = (37 * hash) + NAMEFILTER_FIELD_NUMBER;
hash = (53 * hash) + getNameFilterList().hashCode();
}
if (hasSendRaw()) {
hash = (37 * hash) + SENDRAW_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSendRaw());
}
if (hasPerformMonitoring()) {
hash = (37 * hash) + PERFORMMONITORING_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPerformMonitoring());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.ParameterReplayRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.ParameterReplayRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.ParameterReplayRequest)
org.yamcs.protobuf.Yamcs.ParameterReplayRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ParameterReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ParameterReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ParameterReplayRequest.class, org.yamcs.protobuf.Yamcs.ParameterReplayRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.ParameterReplayRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getNameFilterFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (nameFilterBuilder_ == null) {
nameFilter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
nameFilterBuilder_.clear();
}
sendRaw_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
performMonitoring_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ParameterReplayRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ParameterReplayRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.ParameterReplayRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ParameterReplayRequest build() {
org.yamcs.protobuf.Yamcs.ParameterReplayRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ParameterReplayRequest buildPartial() {
org.yamcs.protobuf.Yamcs.ParameterReplayRequest result = new org.yamcs.protobuf.Yamcs.ParameterReplayRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (nameFilterBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
nameFilter_ = java.util.Collections.unmodifiableList(nameFilter_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.nameFilter_ = nameFilter_;
} else {
result.nameFilter_ = nameFilterBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.sendRaw_ = sendRaw_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.performMonitoring_ = performMonitoring_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.ParameterReplayRequest) {
return mergeFrom((org.yamcs.protobuf.Yamcs.ParameterReplayRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.ParameterReplayRequest other) {
if (other == org.yamcs.protobuf.Yamcs.ParameterReplayRequest.getDefaultInstance()) return this;
if (nameFilterBuilder_ == null) {
if (!other.nameFilter_.isEmpty()) {
if (nameFilter_.isEmpty()) {
nameFilter_ = other.nameFilter_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNameFilterIsMutable();
nameFilter_.addAll(other.nameFilter_);
}
onChanged();
}
} else {
if (!other.nameFilter_.isEmpty()) {
if (nameFilterBuilder_.isEmpty()) {
nameFilterBuilder_.dispose();
nameFilterBuilder_ = null;
nameFilter_ = other.nameFilter_;
bitField0_ = (bitField0_ & ~0x00000001);
nameFilterBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNameFilterFieldBuilder() : null;
} else {
nameFilterBuilder_.addAllMessages(other.nameFilter_);
}
}
}
if (other.hasSendRaw()) {
setSendRaw(other.getSendRaw());
}
if (other.hasPerformMonitoring()) {
setPerformMonitoring(other.getPerformMonitoring());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getNameFilterCount(); i++) {
if (!getNameFilter(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.ParameterReplayRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.ParameterReplayRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List nameFilter_ =
java.util.Collections.emptyList();
private void ensureNameFilterIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
nameFilter_ = new java.util.ArrayList(nameFilter_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder> nameFilterBuilder_;
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public java.util.List getNameFilterList() {
if (nameFilterBuilder_ == null) {
return java.util.Collections.unmodifiableList(nameFilter_);
} else {
return nameFilterBuilder_.getMessageList();
}
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public int getNameFilterCount() {
if (nameFilterBuilder_ == null) {
return nameFilter_.size();
} else {
return nameFilterBuilder_.getCount();
}
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId getNameFilter(int index) {
if (nameFilterBuilder_ == null) {
return nameFilter_.get(index);
} else {
return nameFilterBuilder_.getMessage(index);
}
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder setNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (nameFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameFilterIsMutable();
nameFilter_.set(index, value);
onChanged();
} else {
nameFilterBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder setNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.set(index, builderForValue.build());
onChanged();
} else {
nameFilterBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (nameFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameFilterIsMutable();
nameFilter_.add(value);
onChanged();
} else {
nameFilterBuilder_.addMessage(value);
}
return this;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (nameFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameFilterIsMutable();
nameFilter_.add(index, value);
onChanged();
} else {
nameFilterBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(
org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.add(builderForValue.build());
onChanged();
} else {
nameFilterBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.add(index, builderForValue.build());
onChanged();
} else {
nameFilterBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addAllNameFilter(
java.lang.Iterable extends org.yamcs.protobuf.Yamcs.NamedObjectId> values) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nameFilter_);
onChanged();
} else {
nameFilterBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder clearNameFilter() {
if (nameFilterBuilder_ == null) {
nameFilter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
nameFilterBuilder_.clear();
}
return this;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder removeNameFilter(int index) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.remove(index);
onChanged();
} else {
nameFilterBuilder_.remove(index);
}
return this;
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder getNameFilterBuilder(
int index) {
return getNameFilterFieldBuilder().getBuilder(index);
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getNameFilterOrBuilder(
int index) {
if (nameFilterBuilder_ == null) {
return nameFilter_.get(index); } else {
return nameFilterBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterOrBuilderList() {
if (nameFilterBuilder_ != null) {
return nameFilterBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nameFilter_);
}
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder addNameFilterBuilder() {
return getNameFilterFieldBuilder().addBuilder(
org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance());
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder addNameFilterBuilder(
int index) {
return getNameFilterFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance());
}
/**
*
* At least 1 filter is required
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public java.util.List
getNameFilterBuilderList() {
return getNameFilterFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterFieldBuilder() {
if (nameFilterBuilder_ == null) {
nameFilterBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>(
nameFilter_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
nameFilter_ = null;
}
return nameFilterBuilder_;
}
private boolean sendRaw_ ;
/**
* optional bool sendRaw = 2 [default = false];
* @return Whether the sendRaw field is set.
*/
@java.lang.Override
public boolean hasSendRaw() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bool sendRaw = 2 [default = false];
* @return The sendRaw.
*/
@java.lang.Override
public boolean getSendRaw() {
return sendRaw_;
}
/**
* optional bool sendRaw = 2 [default = false];
* @param value The sendRaw to set.
* @return This builder for chaining.
*/
public Builder setSendRaw(boolean value) {
bitField0_ |= 0x00000002;
sendRaw_ = value;
onChanged();
return this;
}
/**
* optional bool sendRaw = 2 [default = false];
* @return This builder for chaining.
*/
public Builder clearSendRaw() {
bitField0_ = (bitField0_ & ~0x00000002);
sendRaw_ = false;
onChanged();
return this;
}
private boolean performMonitoring_ ;
/**
*
*i.e. out of limit checking
*
*
* optional bool performMonitoring = 3 [default = false];
* @return Whether the performMonitoring field is set.
*/
@java.lang.Override
public boolean hasPerformMonitoring() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*i.e. out of limit checking
*
*
* optional bool performMonitoring = 3 [default = false];
* @return The performMonitoring.
*/
@java.lang.Override
public boolean getPerformMonitoring() {
return performMonitoring_;
}
/**
*
*i.e. out of limit checking
*
*
* optional bool performMonitoring = 3 [default = false];
* @param value The performMonitoring to set.
* @return This builder for chaining.
*/
public Builder setPerformMonitoring(boolean value) {
bitField0_ |= 0x00000004;
performMonitoring_ = value;
onChanged();
return this;
}
/**
*
*i.e. out of limit checking
*
*
* optional bool performMonitoring = 3 [default = false];
* @return This builder for chaining.
*/
public Builder clearPerformMonitoring() {
bitField0_ = (bitField0_ & ~0x00000004);
performMonitoring_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.ParameterReplayRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.ParameterReplayRequest)
private static final org.yamcs.protobuf.Yamcs.ParameterReplayRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.ParameterReplayRequest();
}
public static org.yamcs.protobuf.Yamcs.ParameterReplayRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ParameterReplayRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ParameterReplayRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ParameterReplayRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PacketReplayRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.PacketReplayRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
java.util.List
getNameFilterList();
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
org.yamcs.protobuf.Yamcs.NamedObjectId getNameFilter(int index);
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
int getNameFilterCount();
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterOrBuilderList();
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getNameFilterOrBuilder(
int index);
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @return A list containing the tmLinks.
*/
java.util.List
getTmLinksList();
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @return The count of tmLinks.
*/
int getTmLinksCount();
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param index The index of the element to return.
* @return The tmLinks at the given index.
*/
java.lang.String getTmLinks(int index);
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param index The index of the value to return.
* @return The bytes of the tmLinks at the given index.
*/
com.google.protobuf.ByteString
getTmLinksBytes(int index);
}
/**
* Protobuf type {@code yamcs.protobuf.PacketReplayRequest}
*/
public static final class PacketReplayRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.PacketReplayRequest)
PacketReplayRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use PacketReplayRequest.newBuilder() to construct.
private PacketReplayRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PacketReplayRequest() {
nameFilter_ = java.util.Collections.emptyList();
tmLinks_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PacketReplayRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PacketReplayRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
nameFilter_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
nameFilter_.add(
input.readMessage(org.yamcs.protobuf.Yamcs.NamedObjectId.PARSER, extensionRegistry));
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
tmLinks_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
tmLinks_.add(bs);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
nameFilter_ = java.util.Collections.unmodifiableList(nameFilter_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
tmLinks_ = tmLinks_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PacketReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PacketReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.PacketReplayRequest.class, org.yamcs.protobuf.Yamcs.PacketReplayRequest.Builder.class);
}
public static final int NAMEFILTER_FIELD_NUMBER = 1;
private java.util.List nameFilter_;
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public java.util.List getNameFilterList() {
return nameFilter_;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterOrBuilderList() {
return nameFilter_;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public int getNameFilterCount() {
return nameFilter_.size();
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectId getNameFilter(int index) {
return nameFilter_.get(index);
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getNameFilterOrBuilder(
int index) {
return nameFilter_.get(index);
}
public static final int TMLINKS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList tmLinks_;
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @return A list containing the tmLinks.
*/
public com.google.protobuf.ProtocolStringList
getTmLinksList() {
return tmLinks_;
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @return The count of tmLinks.
*/
public int getTmLinksCount() {
return tmLinks_.size();
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param index The index of the element to return.
* @return The tmLinks at the given index.
*/
public java.lang.String getTmLinks(int index) {
return tmLinks_.get(index);
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param index The index of the value to return.
* @return The bytes of the tmLinks at the given index.
*/
public com.google.protobuf.ByteString
getTmLinksBytes(int index) {
return tmLinks_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getNameFilterCount(); i++) {
if (!getNameFilter(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < nameFilter_.size(); i++) {
output.writeMessage(1, nameFilter_.get(i));
}
for (int i = 0; i < tmLinks_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, tmLinks_.getRaw(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < nameFilter_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, nameFilter_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < tmLinks_.size(); i++) {
dataSize += computeStringSizeNoTag(tmLinks_.getRaw(i));
}
size += dataSize;
size += 1 * getTmLinksList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.PacketReplayRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.PacketReplayRequest other = (org.yamcs.protobuf.Yamcs.PacketReplayRequest) obj;
if (!getNameFilterList()
.equals(other.getNameFilterList())) return false;
if (!getTmLinksList()
.equals(other.getTmLinksList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getNameFilterCount() > 0) {
hash = (37 * hash) + NAMEFILTER_FIELD_NUMBER;
hash = (53 * hash) + getNameFilterList().hashCode();
}
if (getTmLinksCount() > 0) {
hash = (37 * hash) + TMLINKS_FIELD_NUMBER;
hash = (53 * hash) + getTmLinksList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.PacketReplayRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.PacketReplayRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.PacketReplayRequest)
org.yamcs.protobuf.Yamcs.PacketReplayRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PacketReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PacketReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.PacketReplayRequest.class, org.yamcs.protobuf.Yamcs.PacketReplayRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.PacketReplayRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getNameFilterFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (nameFilterBuilder_ == null) {
nameFilter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
nameFilterBuilder_.clear();
}
tmLinks_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PacketReplayRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PacketReplayRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.PacketReplayRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PacketReplayRequest build() {
org.yamcs.protobuf.Yamcs.PacketReplayRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PacketReplayRequest buildPartial() {
org.yamcs.protobuf.Yamcs.PacketReplayRequest result = new org.yamcs.protobuf.Yamcs.PacketReplayRequest(this);
int from_bitField0_ = bitField0_;
if (nameFilterBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
nameFilter_ = java.util.Collections.unmodifiableList(nameFilter_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.nameFilter_ = nameFilter_;
} else {
result.nameFilter_ = nameFilterBuilder_.build();
}
if (((bitField0_ & 0x00000002) != 0)) {
tmLinks_ = tmLinks_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.tmLinks_ = tmLinks_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.PacketReplayRequest) {
return mergeFrom((org.yamcs.protobuf.Yamcs.PacketReplayRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.PacketReplayRequest other) {
if (other == org.yamcs.protobuf.Yamcs.PacketReplayRequest.getDefaultInstance()) return this;
if (nameFilterBuilder_ == null) {
if (!other.nameFilter_.isEmpty()) {
if (nameFilter_.isEmpty()) {
nameFilter_ = other.nameFilter_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNameFilterIsMutable();
nameFilter_.addAll(other.nameFilter_);
}
onChanged();
}
} else {
if (!other.nameFilter_.isEmpty()) {
if (nameFilterBuilder_.isEmpty()) {
nameFilterBuilder_.dispose();
nameFilterBuilder_ = null;
nameFilter_ = other.nameFilter_;
bitField0_ = (bitField0_ & ~0x00000001);
nameFilterBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNameFilterFieldBuilder() : null;
} else {
nameFilterBuilder_.addAllMessages(other.nameFilter_);
}
}
}
if (!other.tmLinks_.isEmpty()) {
if (tmLinks_.isEmpty()) {
tmLinks_ = other.tmLinks_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureTmLinksIsMutable();
tmLinks_.addAll(other.tmLinks_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getNameFilterCount(); i++) {
if (!getNameFilter(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.PacketReplayRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.PacketReplayRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List nameFilter_ =
java.util.Collections.emptyList();
private void ensureNameFilterIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
nameFilter_ = new java.util.ArrayList(nameFilter_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder> nameFilterBuilder_;
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public java.util.List getNameFilterList() {
if (nameFilterBuilder_ == null) {
return java.util.Collections.unmodifiableList(nameFilter_);
} else {
return nameFilterBuilder_.getMessageList();
}
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public int getNameFilterCount() {
if (nameFilterBuilder_ == null) {
return nameFilter_.size();
} else {
return nameFilterBuilder_.getCount();
}
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId getNameFilter(int index) {
if (nameFilterBuilder_ == null) {
return nameFilter_.get(index);
} else {
return nameFilterBuilder_.getMessage(index);
}
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder setNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (nameFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameFilterIsMutable();
nameFilter_.set(index, value);
onChanged();
} else {
nameFilterBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder setNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.set(index, builderForValue.build());
onChanged();
} else {
nameFilterBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (nameFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameFilterIsMutable();
nameFilter_.add(value);
onChanged();
} else {
nameFilterBuilder_.addMessage(value);
}
return this;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (nameFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameFilterIsMutable();
nameFilter_.add(index, value);
onChanged();
} else {
nameFilterBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(
org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.add(builderForValue.build());
onChanged();
} else {
nameFilterBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.add(index, builderForValue.build());
onChanged();
} else {
nameFilterBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addAllNameFilter(
java.lang.Iterable extends org.yamcs.protobuf.Yamcs.NamedObjectId> values) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nameFilter_);
onChanged();
} else {
nameFilterBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder clearNameFilter() {
if (nameFilterBuilder_ == null) {
nameFilter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
nameFilterBuilder_.clear();
}
return this;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder removeNameFilter(int index) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.remove(index);
onChanged();
} else {
nameFilterBuilder_.remove(index);
}
return this;
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder getNameFilterBuilder(
int index) {
return getNameFilterFieldBuilder().getBuilder(index);
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getNameFilterOrBuilder(
int index) {
if (nameFilterBuilder_ == null) {
return nameFilter_.get(index); } else {
return nameFilterBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterOrBuilderList() {
if (nameFilterBuilder_ != null) {
return nameFilterBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nameFilter_);
}
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder addNameFilterBuilder() {
return getNameFilterFieldBuilder().addBuilder(
org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance());
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder addNameFilterBuilder(
int index) {
return getNameFilterFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance());
}
/**
*
* No filter, means all packets for which privileges exist, are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public java.util.List
getNameFilterBuilderList() {
return getNameFilterFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterFieldBuilder() {
if (nameFilterBuilder_ == null) {
nameFilterBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>(
nameFilter_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
nameFilter_ = null;
}
return nameFilterBuilder_;
}
private com.google.protobuf.LazyStringList tmLinks_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTmLinksIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
tmLinks_ = new com.google.protobuf.LazyStringArrayList(tmLinks_);
bitField0_ |= 0x00000002;
}
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @return A list containing the tmLinks.
*/
public com.google.protobuf.ProtocolStringList
getTmLinksList() {
return tmLinks_.getUnmodifiableView();
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @return The count of tmLinks.
*/
public int getTmLinksCount() {
return tmLinks_.size();
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param index The index of the element to return.
* @return The tmLinks at the given index.
*/
public java.lang.String getTmLinks(int index) {
return tmLinks_.get(index);
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param index The index of the value to return.
* @return The bytes of the tmLinks at the given index.
*/
public com.google.protobuf.ByteString
getTmLinksBytes(int index) {
return tmLinks_.getByteString(index);
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param index The index to set the value at.
* @param value The tmLinks to set.
* @return This builder for chaining.
*/
public Builder setTmLinks(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTmLinksIsMutable();
tmLinks_.set(index, value);
onChanged();
return this;
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param value The tmLinks to add.
* @return This builder for chaining.
*/
public Builder addTmLinks(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTmLinksIsMutable();
tmLinks_.add(value);
onChanged();
return this;
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param values The tmLinks to add.
* @return This builder for chaining.
*/
public Builder addAllTmLinks(
java.lang.Iterable values) {
ensureTmLinksIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tmLinks_);
onChanged();
return this;
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @return This builder for chaining.
*/
public Builder clearTmLinks() {
tmLinks_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*if specified, only replay packets originally received on one of those links
*
*
* repeated string tmLinks = 2;
* @param value The bytes of the tmLinks to add.
* @return This builder for chaining.
*/
public Builder addTmLinksBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTmLinksIsMutable();
tmLinks_.add(value);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.PacketReplayRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.PacketReplayRequest)
private static final org.yamcs.protobuf.Yamcs.PacketReplayRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.PacketReplayRequest();
}
public static org.yamcs.protobuf.Yamcs.PacketReplayRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PacketReplayRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PacketReplayRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PacketReplayRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EventReplayRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.EventReplayRequest)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code yamcs.protobuf.EventReplayRequest}
*/
public static final class EventReplayRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.EventReplayRequest)
EventReplayRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use EventReplayRequest.newBuilder() to construct.
private EventReplayRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EventReplayRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new EventReplayRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EventReplayRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_EventReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_EventReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.EventReplayRequest.class, org.yamcs.protobuf.Yamcs.EventReplayRequest.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.EventReplayRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.EventReplayRequest other = (org.yamcs.protobuf.Yamcs.EventReplayRequest) obj;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.EventReplayRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.EventReplayRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.EventReplayRequest)
org.yamcs.protobuf.Yamcs.EventReplayRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_EventReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_EventReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.EventReplayRequest.class, org.yamcs.protobuf.Yamcs.EventReplayRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.EventReplayRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_EventReplayRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.EventReplayRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.EventReplayRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.EventReplayRequest build() {
org.yamcs.protobuf.Yamcs.EventReplayRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.EventReplayRequest buildPartial() {
org.yamcs.protobuf.Yamcs.EventReplayRequest result = new org.yamcs.protobuf.Yamcs.EventReplayRequest(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.EventReplayRequest) {
return mergeFrom((org.yamcs.protobuf.Yamcs.EventReplayRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.EventReplayRequest other) {
if (other == org.yamcs.protobuf.Yamcs.EventReplayRequest.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.EventReplayRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.EventReplayRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.EventReplayRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.EventReplayRequest)
private static final org.yamcs.protobuf.Yamcs.EventReplayRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.EventReplayRequest();
}
public static org.yamcs.protobuf.Yamcs.EventReplayRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EventReplayRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EventReplayRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.EventReplayRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommandHistoryReplayRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.CommandHistoryReplayRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
java.util.List
getNameFilterList();
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
org.yamcs.protobuf.Yamcs.NamedObjectId getNameFilter(int index);
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
int getNameFilterCount();
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterOrBuilderList();
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getNameFilterOrBuilder(
int index);
}
/**
* Protobuf type {@code yamcs.protobuf.CommandHistoryReplayRequest}
*/
public static final class CommandHistoryReplayRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.CommandHistoryReplayRequest)
CommandHistoryReplayRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommandHistoryReplayRequest.newBuilder() to construct.
private CommandHistoryReplayRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommandHistoryReplayRequest() {
nameFilter_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommandHistoryReplayRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommandHistoryReplayRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
nameFilter_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
nameFilter_.add(
input.readMessage(org.yamcs.protobuf.Yamcs.NamedObjectId.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
nameFilter_ = java.util.Collections.unmodifiableList(nameFilter_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_CommandHistoryReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_CommandHistoryReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.class, org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.Builder.class);
}
public static final int NAMEFILTER_FIELD_NUMBER = 1;
private java.util.List nameFilter_;
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public java.util.List getNameFilterList() {
return nameFilter_;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterOrBuilderList() {
return nameFilter_;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public int getNameFilterCount() {
return nameFilter_.size();
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectId getNameFilter(int index) {
return nameFilter_.get(index);
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getNameFilterOrBuilder(
int index) {
return nameFilter_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getNameFilterCount(); i++) {
if (!getNameFilter(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < nameFilter_.size(); i++) {
output.writeMessage(1, nameFilter_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < nameFilter_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, nameFilter_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest other = (org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest) obj;
if (!getNameFilterList()
.equals(other.getNameFilterList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getNameFilterCount() > 0) {
hash = (37 * hash) + NAMEFILTER_FIELD_NUMBER;
hash = (53 * hash) + getNameFilterList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.CommandHistoryReplayRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.CommandHistoryReplayRequest)
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_CommandHistoryReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_CommandHistoryReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.class, org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getNameFilterFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (nameFilterBuilder_ == null) {
nameFilter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
nameFilterBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_CommandHistoryReplayRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest build() {
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest buildPartial() {
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest result = new org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest(this);
int from_bitField0_ = bitField0_;
if (nameFilterBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
nameFilter_ = java.util.Collections.unmodifiableList(nameFilter_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.nameFilter_ = nameFilter_;
} else {
result.nameFilter_ = nameFilterBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest) {
return mergeFrom((org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest other) {
if (other == org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest.getDefaultInstance()) return this;
if (nameFilterBuilder_ == null) {
if (!other.nameFilter_.isEmpty()) {
if (nameFilter_.isEmpty()) {
nameFilter_ = other.nameFilter_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNameFilterIsMutable();
nameFilter_.addAll(other.nameFilter_);
}
onChanged();
}
} else {
if (!other.nameFilter_.isEmpty()) {
if (nameFilterBuilder_.isEmpty()) {
nameFilterBuilder_.dispose();
nameFilterBuilder_ = null;
nameFilter_ = other.nameFilter_;
bitField0_ = (bitField0_ & ~0x00000001);
nameFilterBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNameFilterFieldBuilder() : null;
} else {
nameFilterBuilder_.addAllMessages(other.nameFilter_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getNameFilterCount(); i++) {
if (!getNameFilter(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List nameFilter_ =
java.util.Collections.emptyList();
private void ensureNameFilterIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
nameFilter_ = new java.util.ArrayList(nameFilter_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder> nameFilterBuilder_;
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public java.util.List getNameFilterList() {
if (nameFilterBuilder_ == null) {
return java.util.Collections.unmodifiableList(nameFilter_);
} else {
return nameFilterBuilder_.getMessageList();
}
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public int getNameFilterCount() {
if (nameFilterBuilder_ == null) {
return nameFilter_.size();
} else {
return nameFilterBuilder_.getCount();
}
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId getNameFilter(int index) {
if (nameFilterBuilder_ == null) {
return nameFilter_.get(index);
} else {
return nameFilterBuilder_.getMessage(index);
}
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder setNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (nameFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameFilterIsMutable();
nameFilter_.set(index, value);
onChanged();
} else {
nameFilterBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder setNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.set(index, builderForValue.build());
onChanged();
} else {
nameFilterBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (nameFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameFilterIsMutable();
nameFilter_.add(value);
onChanged();
} else {
nameFilterBuilder_.addMessage(value);
}
return this;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId value) {
if (nameFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameFilterIsMutable();
nameFilter_.add(index, value);
onChanged();
} else {
nameFilterBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(
org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.add(builderForValue.build());
onChanged();
} else {
nameFilterBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addNameFilter(
int index, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder builderForValue) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.add(index, builderForValue.build());
onChanged();
} else {
nameFilterBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder addAllNameFilter(
java.lang.Iterable extends org.yamcs.protobuf.Yamcs.NamedObjectId> values) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nameFilter_);
onChanged();
} else {
nameFilterBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder clearNameFilter() {
if (nameFilterBuilder_ == null) {
nameFilter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
nameFilterBuilder_.clear();
}
return this;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public Builder removeNameFilter(int index) {
if (nameFilterBuilder_ == null) {
ensureNameFilterIsMutable();
nameFilter_.remove(index);
onChanged();
} else {
nameFilterBuilder_.remove(index);
}
return this;
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder getNameFilterBuilder(
int index) {
return getNameFilterFieldBuilder().getBuilder(index);
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder getNameFilterOrBuilder(
int index) {
if (nameFilterBuilder_ == null) {
return nameFilter_.get(index); } else {
return nameFilterBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public java.util.List extends org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterOrBuilderList() {
if (nameFilterBuilder_ != null) {
return nameFilterBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nameFilter_);
}
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder addNameFilterBuilder() {
return getNameFilterFieldBuilder().addBuilder(
org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance());
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public org.yamcs.protobuf.Yamcs.NamedObjectId.Builder addNameFilterBuilder(
int index) {
return getNameFilterFieldBuilder().addBuilder(
index, org.yamcs.protobuf.Yamcs.NamedObjectId.getDefaultInstance());
}
/**
*
* No filter, means all command history entries are sent
*
*
* repeated .yamcs.protobuf.NamedObjectId nameFilter = 1;
*/
public java.util.List
getNameFilterBuilderList() {
return getNameFilterFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>
getNameFilterFieldBuilder() {
if (nameFilterBuilder_ == null) {
nameFilterBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.yamcs.protobuf.Yamcs.NamedObjectId, org.yamcs.protobuf.Yamcs.NamedObjectId.Builder, org.yamcs.protobuf.Yamcs.NamedObjectIdOrBuilder>(
nameFilter_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
nameFilter_ = null;
}
return nameFilterBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.CommandHistoryReplayRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.CommandHistoryReplayRequest)
private static final org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest();
}
public static org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommandHistoryReplayRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommandHistoryReplayRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.CommandHistoryReplayRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PpReplayRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.PpReplayRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @return A list containing the groupNameFilter.
*/
java.util.List
getGroupNameFilterList();
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @return The count of groupNameFilter.
*/
int getGroupNameFilterCount();
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param index The index of the element to return.
* @return The groupNameFilter at the given index.
*/
java.lang.String getGroupNameFilter(int index);
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param index The index of the value to return.
* @return The bytes of the groupNameFilter at the given index.
*/
com.google.protobuf.ByteString
getGroupNameFilterBytes(int index);
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @return A list containing the groupNameExclude.
*/
java.util.List
getGroupNameExcludeList();
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @return The count of groupNameExclude.
*/
int getGroupNameExcludeCount();
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param index The index of the element to return.
* @return The groupNameExclude at the given index.
*/
java.lang.String getGroupNameExclude(int index);
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param index The index of the value to return.
* @return The bytes of the groupNameExclude at the given index.
*/
com.google.protobuf.ByteString
getGroupNameExcludeBytes(int index);
}
/**
*
*Request to replay parameters - they can be filtered by the parameter group
*
*
* Protobuf type {@code yamcs.protobuf.PpReplayRequest}
*/
public static final class PpReplayRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.PpReplayRequest)
PpReplayRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use PpReplayRequest.newBuilder() to construct.
private PpReplayRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PpReplayRequest() {
groupNameFilter_ = com.google.protobuf.LazyStringArrayList.EMPTY;
groupNameExclude_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PpReplayRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PpReplayRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
groupNameFilter_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
groupNameFilter_.add(bs);
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
groupNameExclude_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
groupNameExclude_.add(bs);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
groupNameFilter_ = groupNameFilter_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
groupNameExclude_ = groupNameExclude_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PpReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PpReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.PpReplayRequest.class, org.yamcs.protobuf.Yamcs.PpReplayRequest.Builder.class);
}
public static final int GROUPNAMEFILTER_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList groupNameFilter_;
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @return A list containing the groupNameFilter.
*/
public com.google.protobuf.ProtocolStringList
getGroupNameFilterList() {
return groupNameFilter_;
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @return The count of groupNameFilter.
*/
public int getGroupNameFilterCount() {
return groupNameFilter_.size();
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param index The index of the element to return.
* @return The groupNameFilter at the given index.
*/
public java.lang.String getGroupNameFilter(int index) {
return groupNameFilter_.get(index);
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param index The index of the value to return.
* @return The bytes of the groupNameFilter at the given index.
*/
public com.google.protobuf.ByteString
getGroupNameFilterBytes(int index) {
return groupNameFilter_.getByteString(index);
}
public static final int GROUPNAMEEXCLUDE_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList groupNameExclude_;
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @return A list containing the groupNameExclude.
*/
public com.google.protobuf.ProtocolStringList
getGroupNameExcludeList() {
return groupNameExclude_;
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @return The count of groupNameExclude.
*/
public int getGroupNameExcludeCount() {
return groupNameExclude_.size();
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param index The index of the element to return.
* @return The groupNameExclude at the given index.
*/
public java.lang.String getGroupNameExclude(int index) {
return groupNameExclude_.get(index);
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param index The index of the value to return.
* @return The bytes of the groupNameExclude at the given index.
*/
public com.google.protobuf.ByteString
getGroupNameExcludeBytes(int index) {
return groupNameExclude_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < groupNameFilter_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, groupNameFilter_.getRaw(i));
}
for (int i = 0; i < groupNameExclude_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, groupNameExclude_.getRaw(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < groupNameFilter_.size(); i++) {
dataSize += computeStringSizeNoTag(groupNameFilter_.getRaw(i));
}
size += dataSize;
size += 1 * getGroupNameFilterList().size();
}
{
int dataSize = 0;
for (int i = 0; i < groupNameExclude_.size(); i++) {
dataSize += computeStringSizeNoTag(groupNameExclude_.getRaw(i));
}
size += dataSize;
size += 1 * getGroupNameExcludeList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.PpReplayRequest)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.PpReplayRequest other = (org.yamcs.protobuf.Yamcs.PpReplayRequest) obj;
if (!getGroupNameFilterList()
.equals(other.getGroupNameFilterList())) return false;
if (!getGroupNameExcludeList()
.equals(other.getGroupNameExcludeList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getGroupNameFilterCount() > 0) {
hash = (37 * hash) + GROUPNAMEFILTER_FIELD_NUMBER;
hash = (53 * hash) + getGroupNameFilterList().hashCode();
}
if (getGroupNameExcludeCount() > 0) {
hash = (37 * hash) + GROUPNAMEEXCLUDE_FIELD_NUMBER;
hash = (53 * hash) + getGroupNameExcludeList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.PpReplayRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*Request to replay parameters - they can be filtered by the parameter group
*
*
* Protobuf type {@code yamcs.protobuf.PpReplayRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.PpReplayRequest)
org.yamcs.protobuf.Yamcs.PpReplayRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PpReplayRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PpReplayRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.PpReplayRequest.class, org.yamcs.protobuf.Yamcs.PpReplayRequest.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.PpReplayRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
groupNameFilter_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
groupNameExclude_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_PpReplayRequest_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PpReplayRequest getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.PpReplayRequest.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PpReplayRequest build() {
org.yamcs.protobuf.Yamcs.PpReplayRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PpReplayRequest buildPartial() {
org.yamcs.protobuf.Yamcs.PpReplayRequest result = new org.yamcs.protobuf.Yamcs.PpReplayRequest(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
groupNameFilter_ = groupNameFilter_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.groupNameFilter_ = groupNameFilter_;
if (((bitField0_ & 0x00000002) != 0)) {
groupNameExclude_ = groupNameExclude_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.groupNameExclude_ = groupNameExclude_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.PpReplayRequest) {
return mergeFrom((org.yamcs.protobuf.Yamcs.PpReplayRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.PpReplayRequest other) {
if (other == org.yamcs.protobuf.Yamcs.PpReplayRequest.getDefaultInstance()) return this;
if (!other.groupNameFilter_.isEmpty()) {
if (groupNameFilter_.isEmpty()) {
groupNameFilter_ = other.groupNameFilter_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureGroupNameFilterIsMutable();
groupNameFilter_.addAll(other.groupNameFilter_);
}
onChanged();
}
if (!other.groupNameExclude_.isEmpty()) {
if (groupNameExclude_.isEmpty()) {
groupNameExclude_ = other.groupNameExclude_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureGroupNameExcludeIsMutable();
groupNameExclude_.addAll(other.groupNameExclude_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.PpReplayRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.PpReplayRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList groupNameFilter_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureGroupNameFilterIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
groupNameFilter_ = new com.google.protobuf.LazyStringArrayList(groupNameFilter_);
bitField0_ |= 0x00000001;
}
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @return A list containing the groupNameFilter.
*/
public com.google.protobuf.ProtocolStringList
getGroupNameFilterList() {
return groupNameFilter_.getUnmodifiableView();
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @return The count of groupNameFilter.
*/
public int getGroupNameFilterCount() {
return groupNameFilter_.size();
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param index The index of the element to return.
* @return The groupNameFilter at the given index.
*/
public java.lang.String getGroupNameFilter(int index) {
return groupNameFilter_.get(index);
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param index The index of the value to return.
* @return The bytes of the groupNameFilter at the given index.
*/
public com.google.protobuf.ByteString
getGroupNameFilterBytes(int index) {
return groupNameFilter_.getByteString(index);
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param index The index to set the value at.
* @param value The groupNameFilter to set.
* @return This builder for chaining.
*/
public Builder setGroupNameFilter(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupNameFilterIsMutable();
groupNameFilter_.set(index, value);
onChanged();
return this;
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param value The groupNameFilter to add.
* @return This builder for chaining.
*/
public Builder addGroupNameFilter(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupNameFilterIsMutable();
groupNameFilter_.add(value);
onChanged();
return this;
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param values The groupNameFilter to add.
* @return This builder for chaining.
*/
public Builder addAllGroupNameFilter(
java.lang.Iterable values) {
ensureGroupNameFilterIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, groupNameFilter_);
onChanged();
return this;
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @return This builder for chaining.
*/
public Builder clearGroupNameFilter() {
groupNameFilter_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* No filter, means all pp groups are sent
*
*
* repeated string groupNameFilter = 1;
* @param value The bytes of the groupNameFilter to add.
* @return This builder for chaining.
*/
public Builder addGroupNameFilterBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupNameFilterIsMutable();
groupNameFilter_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList groupNameExclude_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureGroupNameExcludeIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
groupNameExclude_ = new com.google.protobuf.LazyStringArrayList(groupNameExclude_);
bitField0_ |= 0x00000002;
}
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @return A list containing the groupNameExclude.
*/
public com.google.protobuf.ProtocolStringList
getGroupNameExcludeList() {
return groupNameExclude_.getUnmodifiableView();
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @return The count of groupNameExclude.
*/
public int getGroupNameExcludeCount() {
return groupNameExclude_.size();
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param index The index of the element to return.
* @return The groupNameExclude at the given index.
*/
public java.lang.String getGroupNameExclude(int index) {
return groupNameExclude_.get(index);
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param index The index of the value to return.
* @return The bytes of the groupNameExclude at the given index.
*/
public com.google.protobuf.ByteString
getGroupNameExcludeBytes(int index) {
return groupNameExclude_.getByteString(index);
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param index The index to set the value at.
* @param value The groupNameExclude to set.
* @return This builder for chaining.
*/
public Builder setGroupNameExclude(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupNameExcludeIsMutable();
groupNameExclude_.set(index, value);
onChanged();
return this;
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param value The groupNameExclude to add.
* @return This builder for chaining.
*/
public Builder addGroupNameExclude(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupNameExcludeIsMutable();
groupNameExclude_.add(value);
onChanged();
return this;
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param values The groupNameExclude to add.
* @return This builder for chaining.
*/
public Builder addAllGroupNameExclude(
java.lang.Iterable values) {
ensureGroupNameExcludeIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, groupNameExclude_);
onChanged();
return this;
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @return This builder for chaining.
*/
public Builder clearGroupNameExclude() {
groupNameExclude_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* exclude the parameters from these groups
* this takes precedence over the filter above (i.e. if a group is part of both, it will be excluded)
*
*
* repeated string groupNameExclude = 2;
* @param value The bytes of the groupNameExclude to add.
* @return This builder for chaining.
*/
public Builder addGroupNameExcludeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupNameExcludeIsMutable();
groupNameExclude_.add(value);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.PpReplayRequest)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.PpReplayRequest)
private static final org.yamcs.protobuf.Yamcs.PpReplayRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.PpReplayRequest();
}
public static org.yamcs.protobuf.Yamcs.PpReplayRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PpReplayRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PpReplayRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.PpReplayRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReplayStatusOrBuilder extends
// @@protoc_insertion_point(interface_extends:yamcs.protobuf.ReplayStatus)
com.google.protobuf.MessageOrBuilder {
/**
* required .yamcs.protobuf.ReplayStatus.ReplayState state = 1;
* @return Whether the state field is set.
*/
boolean hasState();
/**
* required .yamcs.protobuf.ReplayStatus.ReplayState state = 1;
* @return The state.
*/
org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState getState();
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
* @return Whether the request field is set.
*/
boolean hasRequest();
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
* @return The request.
*/
org.yamcs.protobuf.Yamcs.ReplayRequest getRequest();
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
*/
org.yamcs.protobuf.Yamcs.ReplayRequestOrBuilder getRequestOrBuilder();
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return Whether the errorMessage field is set.
*/
boolean hasErrorMessage();
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return The errorMessage.
*/
java.lang.String getErrorMessage();
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return The bytes for errorMessage.
*/
com.google.protobuf.ByteString
getErrorMessageBytes();
}
/**
* Protobuf type {@code yamcs.protobuf.ReplayStatus}
*/
public static final class ReplayStatus extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:yamcs.protobuf.ReplayStatus)
ReplayStatusOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReplayStatus.newBuilder() to construct.
private ReplayStatus(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReplayStatus() {
state_ = 0;
errorMessage_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ReplayStatus();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ReplayStatus(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState value = org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
state_ = rawValue;
}
break;
}
case 18: {
org.yamcs.protobuf.Yamcs.ReplayRequest.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = request_.toBuilder();
}
request_ = input.readMessage(org.yamcs.protobuf.Yamcs.ReplayRequest.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(request_);
request_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
errorMessage_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ReplayStatus.class, org.yamcs.protobuf.Yamcs.ReplayStatus.Builder.class);
}
/**
* Protobuf enum {@code yamcs.protobuf.ReplayStatus.ReplayState}
*/
public enum ReplayState
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* just at the beginning or when the replay request (start, stop or packet selection) changes
*
*
* INITIALIZATION = 0;
*/
INITIALIZATION(0),
/**
* RUNNING = 1;
*/
RUNNING(1),
/**
*
* The replay has reached the end with the endaction stop
*
*
* STOPPED = 2;
*/
STOPPED(2),
/**
*
* The replay stopped due to an error.
*
*
* ERROR = 3;
*/
ERROR(3),
/**
* PAUSED = 4;
*/
PAUSED(4),
/**
*
* The replay is finished and closed
*
*
* CLOSED = 5;
*/
CLOSED(5),
;
/**
*
* just at the beginning or when the replay request (start, stop or packet selection) changes
*
*
* INITIALIZATION = 0;
*/
public static final int INITIALIZATION_VALUE = 0;
/**
* RUNNING = 1;
*/
public static final int RUNNING_VALUE = 1;
/**
*
* The replay has reached the end with the endaction stop
*
*
* STOPPED = 2;
*/
public static final int STOPPED_VALUE = 2;
/**
*
* The replay stopped due to an error.
*
*
* ERROR = 3;
*/
public static final int ERROR_VALUE = 3;
/**
* PAUSED = 4;
*/
public static final int PAUSED_VALUE = 4;
/**
*
* The replay is finished and closed
*
*
* CLOSED = 5;
*/
public static final int CLOSED_VALUE = 5;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ReplayState valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ReplayState forNumber(int value) {
switch (value) {
case 0: return INITIALIZATION;
case 1: return RUNNING;
case 2: return STOPPED;
case 3: return ERROR;
case 4: return PAUSED;
case 5: return CLOSED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ReplayState> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ReplayState findValueByNumber(int number) {
return ReplayState.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.ReplayStatus.getDescriptor().getEnumTypes().get(0);
}
private static final ReplayState[] VALUES = values();
public static ReplayState valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ReplayState(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:yamcs.protobuf.ReplayStatus.ReplayState)
}
private int bitField0_;
public static final int STATE_FIELD_NUMBER = 1;
private int state_;
/**
* required .yamcs.protobuf.ReplayStatus.ReplayState state = 1;
* @return Whether the state field is set.
*/
@java.lang.Override public boolean hasState() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required .yamcs.protobuf.ReplayStatus.ReplayState state = 1;
* @return The state.
*/
@java.lang.Override public org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState getState() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState result = org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState.valueOf(state_);
return result == null ? org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState.INITIALIZATION : result;
}
public static final int REQUEST_FIELD_NUMBER = 2;
private org.yamcs.protobuf.Yamcs.ReplayRequest request_;
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
* @return Whether the request field is set.
*/
@java.lang.Override
public boolean hasRequest() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
* @return The request.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayRequest getRequest() {
return request_ == null ? org.yamcs.protobuf.Yamcs.ReplayRequest.getDefaultInstance() : request_;
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayRequestOrBuilder getRequestOrBuilder() {
return request_ == null ? org.yamcs.protobuf.Yamcs.ReplayRequest.getDefaultInstance() : request_;
}
public static final int ERRORMESSAGE_FIELD_NUMBER = 3;
private volatile java.lang.Object errorMessage_;
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return Whether the errorMessage field is set.
*/
@java.lang.Override
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return The errorMessage.
*/
@java.lang.Override
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
errorMessage_ = s;
}
return s;
}
}
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return The bytes for errorMessage.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasState()) {
memoizedIsInitialized = 0;
return false;
}
if (hasRequest()) {
if (!getRequest().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, state_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getRequest());
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, errorMessage_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, state_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRequest());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, errorMessage_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.yamcs.protobuf.Yamcs.ReplayStatus)) {
return super.equals(obj);
}
org.yamcs.protobuf.Yamcs.ReplayStatus other = (org.yamcs.protobuf.Yamcs.ReplayStatus) obj;
if (hasState() != other.hasState()) return false;
if (hasState()) {
if (state_ != other.state_) return false;
}
if (hasRequest() != other.hasRequest()) return false;
if (hasRequest()) {
if (!getRequest()
.equals(other.getRequest())) return false;
}
if (hasErrorMessage() != other.hasErrorMessage()) return false;
if (hasErrorMessage()) {
if (!getErrorMessage()
.equals(other.getErrorMessage())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasState()) {
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + state_;
}
if (hasRequest()) {
hash = (37 * hash) + REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getRequest().hashCode();
}
if (hasErrorMessage()) {
hash = (37 * hash) + ERRORMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getErrorMessage().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.yamcs.protobuf.Yamcs.ReplayStatus prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code yamcs.protobuf.ReplayStatus}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:yamcs.protobuf.ReplayStatus)
org.yamcs.protobuf.Yamcs.ReplayStatusOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.yamcs.protobuf.Yamcs.ReplayStatus.class, org.yamcs.protobuf.Yamcs.ReplayStatus.Builder.class);
}
// Construct using org.yamcs.protobuf.Yamcs.ReplayStatus.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRequestFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
state_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
if (requestBuilder_ == null) {
request_ = null;
} else {
requestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
errorMessage_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.yamcs.protobuf.Yamcs.internal_static_yamcs_protobuf_ReplayStatus_descriptor;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayStatus getDefaultInstanceForType() {
return org.yamcs.protobuf.Yamcs.ReplayStatus.getDefaultInstance();
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayStatus build() {
org.yamcs.protobuf.Yamcs.ReplayStatus result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayStatus buildPartial() {
org.yamcs.protobuf.Yamcs.ReplayStatus result = new org.yamcs.protobuf.Yamcs.ReplayStatus(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.state_ = state_;
if (((from_bitField0_ & 0x00000002) != 0)) {
if (requestBuilder_ == null) {
result.request_ = request_;
} else {
result.request_ = requestBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.errorMessage_ = errorMessage_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.yamcs.protobuf.Yamcs.ReplayStatus) {
return mergeFrom((org.yamcs.protobuf.Yamcs.ReplayStatus)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.yamcs.protobuf.Yamcs.ReplayStatus other) {
if (other == org.yamcs.protobuf.Yamcs.ReplayStatus.getDefaultInstance()) return this;
if (other.hasState()) {
setState(other.getState());
}
if (other.hasRequest()) {
mergeRequest(other.getRequest());
}
if (other.hasErrorMessage()) {
bitField0_ |= 0x00000004;
errorMessage_ = other.errorMessage_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasState()) {
return false;
}
if (hasRequest()) {
if (!getRequest().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.yamcs.protobuf.Yamcs.ReplayStatus parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.yamcs.protobuf.Yamcs.ReplayStatus) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int state_ = 0;
/**
* required .yamcs.protobuf.ReplayStatus.ReplayState state = 1;
* @return Whether the state field is set.
*/
@java.lang.Override public boolean hasState() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required .yamcs.protobuf.ReplayStatus.ReplayState state = 1;
* @return The state.
*/
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState getState() {
@SuppressWarnings("deprecation")
org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState result = org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState.valueOf(state_);
return result == null ? org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState.INITIALIZATION : result;
}
/**
* required .yamcs.protobuf.ReplayStatus.ReplayState state = 1;
* @param value The state to set.
* @return This builder for chaining.
*/
public Builder setState(org.yamcs.protobuf.Yamcs.ReplayStatus.ReplayState value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
state_ = value.getNumber();
onChanged();
return this;
}
/**
* required .yamcs.protobuf.ReplayStatus.ReplayState state = 1;
* @return This builder for chaining.
*/
public Builder clearState() {
bitField0_ = (bitField0_ & ~0x00000001);
state_ = 0;
onChanged();
return this;
}
private org.yamcs.protobuf.Yamcs.ReplayRequest request_;
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.ReplayRequest, org.yamcs.protobuf.Yamcs.ReplayRequest.Builder, org.yamcs.protobuf.Yamcs.ReplayRequestOrBuilder> requestBuilder_;
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
* @return Whether the request field is set.
*/
public boolean hasRequest() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
* @return The request.
*/
public org.yamcs.protobuf.Yamcs.ReplayRequest getRequest() {
if (requestBuilder_ == null) {
return request_ == null ? org.yamcs.protobuf.Yamcs.ReplayRequest.getDefaultInstance() : request_;
} else {
return requestBuilder_.getMessage();
}
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
*/
public Builder setRequest(org.yamcs.protobuf.Yamcs.ReplayRequest value) {
if (requestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
request_ = value;
onChanged();
} else {
requestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
*/
public Builder setRequest(
org.yamcs.protobuf.Yamcs.ReplayRequest.Builder builderForValue) {
if (requestBuilder_ == null) {
request_ = builderForValue.build();
onChanged();
} else {
requestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
*/
public Builder mergeRequest(org.yamcs.protobuf.Yamcs.ReplayRequest value) {
if (requestBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
request_ != null &&
request_ != org.yamcs.protobuf.Yamcs.ReplayRequest.getDefaultInstance()) {
request_ =
org.yamcs.protobuf.Yamcs.ReplayRequest.newBuilder(request_).mergeFrom(value).buildPartial();
} else {
request_ = value;
}
onChanged();
} else {
requestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
*/
public Builder clearRequest() {
if (requestBuilder_ == null) {
request_ = null;
onChanged();
} else {
requestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
*/
public org.yamcs.protobuf.Yamcs.ReplayRequest.Builder getRequestBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getRequestFieldBuilder().getBuilder();
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
*/
public org.yamcs.protobuf.Yamcs.ReplayRequestOrBuilder getRequestOrBuilder() {
if (requestBuilder_ != null) {
return requestBuilder_.getMessageOrBuilder();
} else {
return request_ == null ?
org.yamcs.protobuf.Yamcs.ReplayRequest.getDefaultInstance() : request_;
}
}
/**
* optional .yamcs.protobuf.ReplayRequest request = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.ReplayRequest, org.yamcs.protobuf.Yamcs.ReplayRequest.Builder, org.yamcs.protobuf.Yamcs.ReplayRequestOrBuilder>
getRequestFieldBuilder() {
if (requestBuilder_ == null) {
requestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.yamcs.protobuf.Yamcs.ReplayRequest, org.yamcs.protobuf.Yamcs.ReplayRequest.Builder, org.yamcs.protobuf.Yamcs.ReplayRequestOrBuilder>(
getRequest(),
getParentForChildren(),
isClean());
request_ = null;
}
return requestBuilder_;
}
private java.lang.Object errorMessage_ = "";
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return Whether the errorMessage field is set.
*/
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return The errorMessage.
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
errorMessage_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return The bytes for errorMessage.
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @param value The errorMessage to set.
* @return This builder for chaining.
*/
public Builder setErrorMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
errorMessage_ = value;
onChanged();
return this;
}
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @return This builder for chaining.
*/
public Builder clearErrorMessage() {
bitField0_ = (bitField0_ & ~0x00000004);
errorMessage_ = getDefaultInstance().getErrorMessage();
onChanged();
return this;
}
/**
*
* In case state is ERROR
*
*
* optional string errorMessage = 3;
* @param value The bytes for errorMessage to set.
* @return This builder for chaining.
*/
public Builder setErrorMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
errorMessage_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:yamcs.protobuf.ReplayStatus)
}
// @@protoc_insertion_point(class_scope:yamcs.protobuf.ReplayStatus)
private static final org.yamcs.protobuf.Yamcs.ReplayStatus DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.yamcs.protobuf.Yamcs.ReplayStatus();
}
public static org.yamcs.protobuf.Yamcs.ReplayStatus getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ReplayStatus parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ReplayStatus(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.yamcs.protobuf.Yamcs.ReplayStatus getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_Value_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_Value_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_AggregateValue_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_AggregateValue_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_NamedObjectId_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_NamedObjectId_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_NamedObjectList_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_NamedObjectList_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_ArchiveRecord_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_ArchiveRecord_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_ArchiveRecord_ExtraEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_ArchiveRecord_ExtraEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_ReplaySpeed_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_ReplaySpeed_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_ReplayRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_ReplayRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_ParameterReplayRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_ParameterReplayRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_PacketReplayRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_PacketReplayRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_EventReplayRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_EventReplayRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_CommandHistoryReplayRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_CommandHistoryReplayRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_PpReplayRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_PpReplayRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_yamcs_protobuf_ReplayStatus_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_yamcs_protobuf_ReplayStatus_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\032yamcs/protobuf/yamcs.proto\022\016yamcs.prot" +
"obuf\032\037google/protobuf/timestamp.proto\"\241\004" +
"\n\005Value\022(\n\004type\030\001 \002(\0162\032.yamcs.protobuf.V" +
"alue.Type\022\022\n\nfloatValue\030\002 \001(\002\022\023\n\013doubleV" +
"alue\030\003 \001(\001\022\023\n\013sint32Value\030\004 \001(\021\022\023\n\013uint3" +
"2Value\030\005 \001(\r\022\023\n\013binaryValue\030\006 \001(\014\022\023\n\013str" +
"ingValue\030\007 \001(\t\022\026\n\016timestampValue\030\010 \001(\003\022\023" +
"\n\013uint64Value\030\t \001(\004\022\023\n\013sint64Value\030\n \001(\022" +
"\022\024\n\014booleanValue\030\013 \001(\010\0226\n\016aggregateValue" +
"\030\014 \001(\0132\036.yamcs.protobuf.AggregateValue\022)" +
"\n\narrayValue\030\r \003(\0132\025.yamcs.protobuf.Valu" +
"e\"\265\001\n\004Type\022\t\n\005FLOAT\020\000\022\n\n\006DOUBLE\020\001\022\n\n\006UIN" +
"T32\020\002\022\n\n\006SINT32\020\003\022\n\n\006BINARY\020\004\022\n\n\006STRING\020" +
"\005\022\r\n\tTIMESTAMP\020\006\022\n\n\006UINT64\020\007\022\n\n\006SINT64\020\010" +
"\022\013\n\007BOOLEAN\020\t\022\r\n\tAGGREGATE\020\n\022\t\n\005ARRAY\020\013\022" +
"\016\n\nENUMERATED\020\014\022\010\n\004NONE\020\r\"D\n\016AggregateVa" +
"lue\022\014\n\004name\030\001 \003(\t\022$\n\005value\030\002 \003(\0132\025.yamcs" +
".protobuf.Value\"0\n\rNamedObjectId\022\014\n\004name" +
"\030\001 \002(\t\022\021\n\tnamespace\030\002 \001(\t\">\n\017NamedObject" +
"List\022+\n\004list\030\001 \003(\0132\035.yamcs.protobuf.Name" +
"dObjectId\"\246\002\n\rArchiveRecord\022)\n\002id\030\001 \001(\0132" +
"\035.yamcs.protobuf.NamedObjectId\022\013\n\003num\030\004 " +
"\001(\005\022\020\n\010seqFirst\030\006 \001(\003\022\017\n\007seqLast\030\007 \001(\003\022)" +
"\n\005first\030\010 \001(\0132\032.google.protobuf.Timestam" +
"p\022(\n\004last\030\t \001(\0132\032.google.protobuf.Timest" +
"amp\0227\n\005extra\030\n \003(\0132(.yamcs.protobuf.Arch" +
"iveRecord.ExtraEntry\032,\n\nExtraEntry\022\013\n\003ke" +
"y\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:\0028\001\"\245\001\n\013ReplaySpe" +
"ed\0229\n\004type\030\001 \002(\0162+.yamcs.protobuf.Replay" +
"Speed.ReplaySpeedType\022\r\n\005param\030\002 \001(\002\"L\n\017" +
"ReplaySpeedType\022\010\n\004AFAP\020\001\022\017\n\013FIXED_DELAY" +
"\020\002\022\014\n\010REALTIME\020\003\022\020\n\014STEP_BY_STEP\020\004\"\246\004\n\rR" +
"eplayRequest\022)\n\005start\030\r \001(\0132\032.google.pro" +
"tobuf.Timestamp\022(\n\004stop\030\016 \001(\0132\032.google.p" +
"rotobuf.Timestamp\0222\n\tendAction\030\003 \001(\0162\031.y" +
"amcs.protobuf.EndAction:\004QUIT\022*\n\005speed\030\004" +
" \001(\0132\033.yamcs.protobuf.ReplaySpeed\022\017\n\007rev" +
"erse\030\017 \001(\010\022@\n\020parameterRequest\030\010 \001(\0132&.y" +
"amcs.protobuf.ParameterReplayRequest\022:\n\r" +
"packetRequest\030\t \001(\0132#.yamcs.protobuf.Pac" +
"ketReplayRequest\0228\n\014eventRequest\030\n \001(\0132\"" +
".yamcs.protobuf.EventReplayRequest\022J\n\025co" +
"mmandHistoryRequest\030\013 \001(\0132+.yamcs.protob" +
"uf.CommandHistoryReplayRequest\0222\n\tppRequ" +
"est\030\014 \001(\0132\037.yamcs.protobuf.PpReplayReque" +
"st\022\027\n\tautostart\030\020 \001(\010:\004true\"\205\001\n\026Paramete" +
"rReplayRequest\0221\n\nnameFilter\030\001 \003(\0132\035.yam" +
"cs.protobuf.NamedObjectId\022\026\n\007sendRaw\030\002 \001" +
"(\010:\005false\022 \n\021performMonitoring\030\003 \001(\010:\005fa" +
"lse\"Y\n\023PacketReplayRequest\0221\n\nnameFilter" +
"\030\001 \003(\0132\035.yamcs.protobuf.NamedObjectId\022\017\n" +
"\007tmLinks\030\002 \003(\t\"\024\n\022EventReplayRequest\"P\n\033" +
"CommandHistoryReplayRequest\0221\n\nnameFilte" +
"r\030\001 \003(\0132\035.yamcs.protobuf.NamedObjectId\"D" +
"\n\017PpReplayRequest\022\027\n\017groupNameFilter\030\001 \003" +
"(\t\022\030\n\020groupNameExclude\030\002 \003(\t\"\355\001\n\014ReplayS" +
"tatus\0227\n\005state\030\001 \002(\0162(.yamcs.protobuf.Re" +
"playStatus.ReplayState\022.\n\007request\030\002 \001(\0132" +
"\035.yamcs.protobuf.ReplayRequest\022\024\n\014errorM" +
"essage\030\003 \001(\t\"^\n\013ReplayState\022\022\n\016INITIALIZ" +
"ATION\020\000\022\013\n\007RUNNING\020\001\022\013\n\007STOPPED\020\002\022\t\n\005ERR" +
"OR\020\003\022\n\n\006PAUSED\020\004\022\n\n\006CLOSED\020\005*)\n\tEndActio" +
"n\022\010\n\004LOOP\020\001\022\010\n\004QUIT\020\002\022\010\n\004STOP\020\003B\024\n\022org.y" +
"amcs.protobuf"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.TimestampProto.getDescriptor(),
});
internal_static_yamcs_protobuf_Value_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_yamcs_protobuf_Value_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_Value_descriptor,
new java.lang.String[] { "Type", "FloatValue", "DoubleValue", "Sint32Value", "Uint32Value", "BinaryValue", "StringValue", "TimestampValue", "Uint64Value", "Sint64Value", "BooleanValue", "AggregateValue", "ArrayValue", });
internal_static_yamcs_protobuf_AggregateValue_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_yamcs_protobuf_AggregateValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_AggregateValue_descriptor,
new java.lang.String[] { "Name", "Value", });
internal_static_yamcs_protobuf_NamedObjectId_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_yamcs_protobuf_NamedObjectId_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_NamedObjectId_descriptor,
new java.lang.String[] { "Name", "Namespace", });
internal_static_yamcs_protobuf_NamedObjectList_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_yamcs_protobuf_NamedObjectList_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_NamedObjectList_descriptor,
new java.lang.String[] { "List", });
internal_static_yamcs_protobuf_ArchiveRecord_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_yamcs_protobuf_ArchiveRecord_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_ArchiveRecord_descriptor,
new java.lang.String[] { "Id", "Num", "SeqFirst", "SeqLast", "First", "Last", "Extra", });
internal_static_yamcs_protobuf_ArchiveRecord_ExtraEntry_descriptor =
internal_static_yamcs_protobuf_ArchiveRecord_descriptor.getNestedTypes().get(0);
internal_static_yamcs_protobuf_ArchiveRecord_ExtraEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_ArchiveRecord_ExtraEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_yamcs_protobuf_ReplaySpeed_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_yamcs_protobuf_ReplaySpeed_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_ReplaySpeed_descriptor,
new java.lang.String[] { "Type", "Param", });
internal_static_yamcs_protobuf_ReplayRequest_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_yamcs_protobuf_ReplayRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_ReplayRequest_descriptor,
new java.lang.String[] { "Start", "Stop", "EndAction", "Speed", "Reverse", "ParameterRequest", "PacketRequest", "EventRequest", "CommandHistoryRequest", "PpRequest", "Autostart", });
internal_static_yamcs_protobuf_ParameterReplayRequest_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_yamcs_protobuf_ParameterReplayRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_ParameterReplayRequest_descriptor,
new java.lang.String[] { "NameFilter", "SendRaw", "PerformMonitoring", });
internal_static_yamcs_protobuf_PacketReplayRequest_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_yamcs_protobuf_PacketReplayRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_PacketReplayRequest_descriptor,
new java.lang.String[] { "NameFilter", "TmLinks", });
internal_static_yamcs_protobuf_EventReplayRequest_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_yamcs_protobuf_EventReplayRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_EventReplayRequest_descriptor,
new java.lang.String[] { });
internal_static_yamcs_protobuf_CommandHistoryReplayRequest_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_yamcs_protobuf_CommandHistoryReplayRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_CommandHistoryReplayRequest_descriptor,
new java.lang.String[] { "NameFilter", });
internal_static_yamcs_protobuf_PpReplayRequest_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_yamcs_protobuf_PpReplayRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_PpReplayRequest_descriptor,
new java.lang.String[] { "GroupNameFilter", "GroupNameExclude", });
internal_static_yamcs_protobuf_ReplayStatus_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_yamcs_protobuf_ReplayStatus_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_yamcs_protobuf_ReplayStatus_descriptor,
new java.lang.String[] { "State", "Request", "ErrorMessage", });
com.google.protobuf.TimestampProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy