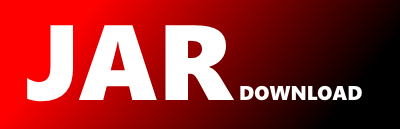
org.yamcs.protobuf.activities.AbstractActivitiesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
package org.yamcs.protobuf.activities;
import com.google.protobuf.Descriptors.MethodDescriptor;
import com.google.protobuf.Descriptors.ServiceDescriptor;
import com.google.protobuf.Message;
import org.yamcs.api.Api;
import org.yamcs.api.Observer;
@javax.annotation.processing.Generated(value = "org.yamcs.maven.ServiceGenerator", date = "2025-02-13T11:12:59.913209251Z")
@SuppressWarnings("unchecked")
public abstract class AbstractActivitiesApi implements Api {
/**
*
* List activities
*
*/
public abstract void listActivities(T ctx, ListActivitiesRequest request, Observer observer);
/**
*
* Get an activity
*
*/
public abstract void getActivity(T ctx, GetActivityRequest request, Observer observer);
/**
*
* Get the activity log
*
*/
public abstract void getActivityLog(T ctx, GetActivityLogRequest request, Observer observer);
/**
*
* Start an activity
*
* The request body allows for the execution of arbitrary activities.
* The following activity types are included in the core Yamcs module:
*
* .. rubric:: Command
*
* Execute a single command
*
* ``type`` (string)
* Set to ``COMMAND``.
*
* ``args`` (map)
* Map accepting the following options:
*
* ``command``
* **Required.** Qualified name of a command
*
* ``args`` (map)
* Named arguments, if the command requires any
*
* ``extra`` (map)
* Extra command options
*
* ``processor`` (string)
* Optional processor name. If not provided, Yamcs defaults
* to any processor it can find with commanding enabled.
*
* Example:
*
* .. code-block:: json
*
* {
* "type": "COMMAND",
* "args": {
* "command": "/YSS/SIMULATOR/SWITCH_VOLTAGE_ON",
* "args": {
* "voltage_num": 3
* },
* "processor": "realtime"
* }
* }
*
* .. rubric:: Command Stack
*
* Execute a command stack
*
* ``type`` (string)
* Set to ``COMMAND_STACK``.
*
* ``args`` (map)
* Map accepting the following options:
*
* ``bucket``
* **Required.** The name of the bucket containg the stack
*
* ``stack``
* **Required.** The name of the stack object inside the bucket
*
* ``processor`` (string)
* Optional processor name. If not provided, Yamcs defaults
* to any processor it can find with commanding enabled.
*
* Example:
*
* .. code-block:: json
*
* {
* "type": "COMMAND_STACK",
* "args": {
* "bucket": "mybucket",
* "stack": "mystack.ycs",
* "processor": "realtime"
* }
* }
*
* .. rubric:: Script
*
* Run a script
*
* ``type`` (string)
* Set to ``SCRIPT``.
* ``args`` (map)
* Map accepting the following options:
*
* ``script``
* **Required.** Script identifier.
*
* This should be the relative path to an executable file in one of the
* search locations. When unconfigured, the default search location is
* :file:`etc/scripts/` relative to the Yamcs working directory.
*
* ``args`` (string or string[])
* Script arguments
*
* ``processor`` (string)
* If provided, this information is passed to the script in an environment
* variable ``YAMCS_PROCESSOR``.
*
* Example:
*
* .. code-block:: json
*
* {
* "type": "SCRIPT",
* "args": {
* "script": "simulate_los.py",
* "args": "--duration 60",
* "processor": "realtime"
* }
* }
*
*/
public abstract void startActivity(T ctx, StartActivityRequest request, Observer observer);
/**
*
* Cancel an ongoing activity
*
*/
public abstract void cancelActivity(T ctx, CancelActivityRequest request, Observer observer);
/**
*
* Mark an ongoing activity as completed.
*
* This method may only be used with manual activities.
*
*/
public abstract void completeManualActivity(T ctx, CompleteManualActivityRequest request, Observer observer);
/**
*
* Receive activity status updates
*
*/
public abstract void subscribeGlobalStatus(T ctx, SubscribeGlobalStatusRequest request, Observer observer);
/**
*
* Receive activity updates
*
*/
public abstract void subscribeActivities(T ctx, SubscribeActivitiesRequest request, Observer observer);
/**
*
* Receive activity log updates
*
*/
public abstract void subscribeActivityLog(T ctx, SubscribeActivityLogRequest request, Observer observer);
/**
*
* List available executors
*
*/
public abstract void listExecutors(T ctx, ListExecutorsRequest request, Observer observer);
/**
*
* List scripts available for activities of type SCRIPT
*
*/
public abstract void listScripts(T ctx, ListScriptsRequest request, Observer observer);
@Override
public final ServiceDescriptor getDescriptorForType() {
return ActivitiesServiceProto.getDescriptor().getServices().get(0);
}
@Override
public final Message getRequestPrototype(MethodDescriptor method) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
return ListActivitiesRequest.getDefaultInstance();
case 1:
return GetActivityRequest.getDefaultInstance();
case 2:
return GetActivityLogRequest.getDefaultInstance();
case 3:
return StartActivityRequest.getDefaultInstance();
case 4:
return CancelActivityRequest.getDefaultInstance();
case 5:
return CompleteManualActivityRequest.getDefaultInstance();
case 6:
return SubscribeGlobalStatusRequest.getDefaultInstance();
case 7:
return SubscribeActivitiesRequest.getDefaultInstance();
case 8:
return SubscribeActivityLogRequest.getDefaultInstance();
case 9:
return ListExecutorsRequest.getDefaultInstance();
case 10:
return ListScriptsRequest.getDefaultInstance();
default:
throw new IllegalStateException();
}
}
@Override
public final Message getResponsePrototype(MethodDescriptor method) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
return ListActivitiesResponse.getDefaultInstance();
case 1:
return ActivityInfo.getDefaultInstance();
case 2:
return GetActivityLogResponse.getDefaultInstance();
case 3:
return ActivityInfo.getDefaultInstance();
case 4:
return ActivityInfo.getDefaultInstance();
case 5:
return ActivityInfo.getDefaultInstance();
case 6:
return GlobalActivityStatus.getDefaultInstance();
case 7:
return ActivityInfo.getDefaultInstance();
case 8:
return ActivityLogInfo.getDefaultInstance();
case 9:
return ListExecutorsResponse.getDefaultInstance();
case 10:
return ListScriptsResponse.getDefaultInstance();
default:
throw new IllegalStateException();
}
}
@Override
public final void callMethod(MethodDescriptor method, T ctx, Message request, Observer future) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
case 0:
listActivities(ctx, (ListActivitiesRequest) request, (Observer)(Object) future);
return;
case 1:
getActivity(ctx, (GetActivityRequest) request, (Observer)(Object) future);
return;
case 2:
getActivityLog(ctx, (GetActivityLogRequest) request, (Observer)(Object) future);
return;
case 3:
startActivity(ctx, (StartActivityRequest) request, (Observer)(Object) future);
return;
case 4:
cancelActivity(ctx, (CancelActivityRequest) request, (Observer)(Object) future);
return;
case 5:
completeManualActivity(ctx, (CompleteManualActivityRequest) request, (Observer)(Object) future);
return;
case 6:
subscribeGlobalStatus(ctx, (SubscribeGlobalStatusRequest) request, (Observer)(Object) future);
return;
case 7:
subscribeActivities(ctx, (SubscribeActivitiesRequest) request, (Observer)(Object) future);
return;
case 8:
subscribeActivityLog(ctx, (SubscribeActivityLogRequest) request, (Observer)(Object) future);
return;
case 9:
listExecutors(ctx, (ListExecutorsRequest) request, (Observer)(Object) future);
return;
case 10:
listScripts(ctx, (ListScriptsRequest) request, (Observer)(Object) future);
return;
default:
throw new IllegalStateException();
}
}
@Override
public final Observer callMethod(MethodDescriptor method, T ctx, Observer future) {
if (method.getService() != getDescriptorForType()) {
throw new IllegalArgumentException("Method not contained by this service.");
}
switch (method.getIndex()) {
default:
throw new IllegalStateException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy