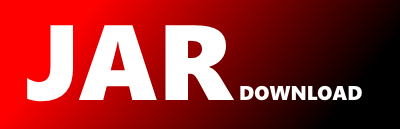
yamcs.protobuf.alarms.alarms_service.proto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
syntax="proto2";
package yamcs.protobuf.alarms;
option java_package = "org.yamcs.protobuf.alarms";
option java_outer_classname = "AlarmsServiceProto";
option java_multiple_files = true;
import "google/protobuf/empty.proto";
import "google/protobuf/timestamp.proto";
import "yamcs/api/annotations.proto";
import "yamcs/protobuf/alarms/alarms.proto";
service AlarmsApi {
// List alarms
rpc ListAlarms(ListAlarmsRequest) returns (ListAlarmsResponse) {
option (yamcs.api.route) = {
get: "/api/archive/{instance}/alarms/{name**}"
};
}
// List alarms
rpc ListProcessorAlarms(ListProcessorAlarmsRequest) returns (ListProcessorAlarmsResponse) {
option (yamcs.api.route) = {
get: "/api/processors/{instance}/{processor}/alarms"
};
}
// Update an alarm
rpc EditAlarm(EditAlarmRequest) returns (google.protobuf.Empty) {
option (yamcs.api.route) = {
patch: "/api/processors/{instance}/{processor}/alarms/{name*}/{seqnum}"
body: "*"
deprecated: true
};
}
// Acknowledge an alarm
rpc AcknowledgeAlarm(AcknowledgeAlarmRequest) returns (google.protobuf.Empty) {
option (yamcs.api.route) = {
post: "/api/processors/{instance}/{processor}/alarms/{alarm*}/{seqnum}:acknowledge"
body: "*"
};
}
// Shelve an alarm
rpc ShelveAlarm(ShelveAlarmRequest) returns (google.protobuf.Empty) {
option (yamcs.api.route) = {
post: "/api/processors/{instance}/{processor}/alarms/{alarm*}/{seqnum}:shelve"
body: "*"
};
}
// Unshelve an alarm
rpc UnshelveAlarm(UnshelveAlarmRequest) returns (google.protobuf.Empty) {
option (yamcs.api.route) = {
post: "/api/processors/{instance}/{processor}/alarms/{alarm*}/{seqnum}:unshelve"
};
}
// Clear an alarm
rpc ClearAlarm(ClearAlarmRequest) returns (google.protobuf.Empty) {
option (yamcs.api.route) = {
post: "/api/processors/{instance}/{processor}/alarms/{alarm*}/{seqnum}:clear"
body: "*"
};
}
// Receive alarm status updates
rpc SubscribeGlobalStatus(SubscribeGlobalStatusRequest) returns (stream GlobalAlarmStatus) {
option (yamcs.api.websocket) = {
topic: "global-alarm-status"
};
}
// Receive alarm updates
rpc SubscribeAlarms(SubscribeAlarmsRequest) returns (stream AlarmData) {
option (yamcs.api.websocket) = {
topic: "alarms"
};
}
}
message ListAlarmsRequest {
// Yamcs instance name
optional string instance = 1;
// The zero-based row number at which to start outputting results.
// Default: ``0``
optional int64 pos = 2;
// The maximum number of returned records per page. Choose this value
// too high and you risk hitting the maximum response size limit
// enforced by the server. Default: ``100``
optional int32 limit = 3;
// Filter the lower bound of the alarm's trigger time. Specify a date
// string in ISO 8601 format. This bound is inclusive.
optional google.protobuf.Timestamp start = 4;
// Filter the upper bound of the alarm's trigger time. Specify a date
// string in ISO 8601 format. This bound is exclusive.
optional google.protobuf.Timestamp stop = 5;
// The order of the returned results. Can be either ``asc`` or
// ``desc``. The sorting is always by trigger time (i.e. the
// generation time of the trigger value). Default: ``desc``
optional string order = 6;
// Filter alarm instances on a specific alarm name (for example:
// parameter name)
optional string name = 7;
// Continuation token returned by a previous page response.
optional string next = 8;
}
message ListAlarmsResponse {
repeated AlarmData alarms = 1;
// Token indicating the response is only partial. More results can then
// be obtained by performing the same request (including all original
// query parameters) and setting the ``next`` parameter to this token.
optional string continuationToken = 2;
}
message ListProcessorAlarmsRequest {
optional string instance = 1;
optional string processor = 2;
// pending alarms are those for which the minimum number of violations has not been reached
optional bool includePending = 3;
}
message ListProcessorAlarmsResponse {
repeated AlarmData alarms = 1;
}
message SubscribeAlarmsRequest {
optional string instance = 1;
optional string processor = 2;
// pending alarms are those for which the minimum number of violations has not been reached
optional bool includePending = 3;
}
message EditAlarmRequest {
// Yamcs instance name
optional string instance = 1;
// Processor name
optional string processor = 2;
// Alarm name
optional string name = 3;
optional uint32 seqnum = 4;
// **Required.** The state of the alarm.
// Either ``acknowledged``, ``shelved``, ``unshelved`` or ``cleared``.
optional string state = 5;
// Message documenting the alarm change.
optional string comment = 6;
//shelve time in milliseconds (if the state = shelved)
//can be left out which means it is shelved indefinitely
optional uint64 shelveDuration = 7;
}
message AcknowledgeAlarmRequest {
// Yamcs instance name
optional string instance = 1;
// Processor name
optional string processor = 2;
// Alarm name
optional string alarm = 3;
optional uint32 seqnum = 4;
// Message documenting the alarm change.
optional string comment = 5;
}
message ShelveAlarmRequest {
// Yamcs instance name
optional string instance = 1;
// Processor name
optional string processor = 2;
// Alarm name
optional string alarm = 3;
optional uint32 seqnum = 4;
// Message documenting the alarm change.
optional string comment = 5;
//shelve time in milliseconds (if the state = shelved)
//can be left out which means it is shelved indefinitely
optional uint64 shelveDuration = 6;
}
message UnshelveAlarmRequest {
// Yamcs instance name
optional string instance = 1;
// Processor name
optional string processor = 2;
// Alarm name
optional string alarm = 3;
optional uint32 seqnum = 4;
}
message ClearAlarmRequest {
// Yamcs instance name
optional string instance = 1;
// Processor name
optional string processor = 2;
// Alarm name
optional string alarm = 3;
optional uint32 seqnum = 4;
// Message documenting the alarm change
optional string comment = 5;
}
message SubscribeGlobalStatusRequest {
// Yamcs instance name
optional string instance = 1;
// Processor name
optional string processor = 2;
}
message GlobalAlarmStatus {
// The number of active unacknowledged alarms
optional int32 unacknowledgedCount = 1;
// True if there is at least one unacknowledged alarm not OK
// (i.e. latest value of parameter still out of limits)
optional bool unacknowledgedActive = 2;
// Highest severity among all unacknowledged alarms
optional AlarmSeverity unacknowledgedSeverity = 7;
// The number of active acknowledged alarms
optional int32 acknowledgedCount = 3;
// True if there is at least one acknowledged alarm not OK
// (i.e. latest value of parameter still out of limits)
optional bool acknowledgedActive = 4;
// Highest severity among all acknowledged alarms
optional AlarmSeverity acknowledgedSeverity = 8;
// The number of shelved alarms
optional int32 shelvedCount = 5;
// True if there is at least one shelved alarm not OK (i.e. latest value of parameter still out of limits)
optional bool shelvedActive = 6;
// Highest severity among all shelved alarms
optional AlarmSeverity shelvedSeverity = 9;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy