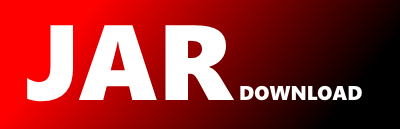
yamcs.protobuf.links.links.proto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yamcs-api Show documentation
Show all versions of yamcs-api Show documentation
Used by external clients to communicate with Yamcs
syntax="proto2";
package yamcs.protobuf.links;
option java_package = "org.yamcs.protobuf.links";
option java_outer_classname = "LinksServiceProto";
option java_multiple_files = true;
import "yamcs/api/annotations.proto";
import "yamcs/protobuf/actions/actions.proto";
import "google/protobuf/struct.proto";
service LinksApi {
// List links
rpc ListLinks(ListLinksRequest) returns (ListLinksResponse) {
option (yamcs.api.route) = {
get: "/api/links/{instance?}"
};
}
// Get a link
rpc GetLink(GetLinkRequest) returns (LinkInfo) {
option (yamcs.api.route) = {
get: "/api/links/{instance}/{link}"
};
}
// Enable a link
rpc EnableLink(EnableLinkRequest) returns (LinkInfo) {
option (yamcs.api.route) = {
post: "/api/links/{instance}/{link}:enable"
log: "Link '{link}' enabled"
};
}
// Disable a link
rpc DisableLink(DisableLinkRequest) returns (LinkInfo) {
option (yamcs.api.route) = {
post: "/api/links/{instance}/{link}:disable"
log: "Link '{link}' disabled"
};
}
// Reset link counters
rpc ResetLinkCounters(ResetLinkCountersRequest) returns (LinkInfo) {
option (yamcs.api.route) = {
post: "/api/links/{instance}/{link}:resetCounters"
};
}
// Receive link updates
rpc SubscribeLinks(SubscribeLinksRequest) returns (stream LinkEvent) {
option (yamcs.api.websocket) = {
topic: "links"
};
}
// Run a link-specific action.
//
// It is up to the link implementation to register and handle
// any link actions.
rpc RunAction(RunActionRequest) returns (google.protobuf.Struct) {
option (yamcs.api.route) = {
post: "/api/links/{instance}/{link}/actions/{action}"
body: "message"
log: "Action '{action}' performed on link '{link}'"
};
}
}
message ListLinksRequest {
// Yamcs instance name
optional string instance = 1;
}
message ListLinksResponse {
repeated LinkInfo links = 1;
}
message GetLinkRequest {
// Yamcs instance name
optional string instance = 1;
// Link name
optional string link = 2;
}
message EnableLinkRequest {
// Yamcs instance name
optional string instance = 1;
// Link name
optional string link = 2;
}
message DisableLinkRequest {
// Yamcs instance name
optional string instance = 1;
// Link name
optional string link = 2;
}
message ResetLinkCountersRequest {
// Yamcs instance name
optional string instance = 1;
// Link name
optional string link = 2;
}
message RunActionRequest {
// Yamcs instance name
optional string instance = 1;
// Link name
optional string link = 2;
// Action name
optional string action = 3;
// Action message
optional google.protobuf.Struct message = 4;
}
message LinkEvent {
// Detail for all links
repeated LinkInfo links = 3;
}
message SubscribeLinksRequest {
// Yamcs instance name
optional string instance = 1;
}
message LinkInfo {
reserved 5,8;
// Yamcs instance name
optional string instance = 1;
// Link name
optional string name = 2;
// Java class name
optional string type = 3;
// Configuration
optional string spec = 4;
// Whether this link is currently disabled.
optional bool disabled = 6;
optional string status = 7;
// Counter of inbound (received) data.
// The unit of this is specific to each link.
optional int64 dataInCount = 10;
// Counter of outbound (transmitted) data.
// The unit of this is specific to each link.
optional int64 dataOutCount = 11;
// Short status information
optional string detailedStatus = 9;
//if this is a sublink of an aggregated data link, this is the name of the parent
optional string parentName = 12;
// Custom actions
repeated yamcs.protobuf.actions.ActionInfo actions = 13;
// Custom info fields
optional google.protobuf.Struct extra = 14;
// System parameters generated by this link
repeated string parameters = 15;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy