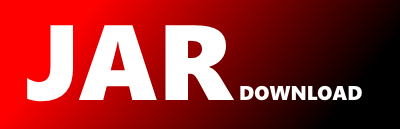
org.yaml.snakeyaml.Yaml Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snakeyaml Show documentation
Show all versions of snakeyaml Show documentation
YAML 1.1 parser and emitter for Java
/**
* Copyright (c) 2008-2011, http://www.snakeyaml.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.yaml.snakeyaml;
import java.io.InputStream;
import java.io.Reader;
import java.io.StringReader;
import java.io.StringWriter;
import java.io.Writer;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.regex.Pattern;
import org.yaml.snakeyaml.composer.Composer;
import org.yaml.snakeyaml.constructor.BaseConstructor;
import org.yaml.snakeyaml.constructor.Constructor;
import org.yaml.snakeyaml.emitter.Emitter;
import org.yaml.snakeyaml.error.YAMLException;
import org.yaml.snakeyaml.events.Event;
import org.yaml.snakeyaml.introspector.BeanAccess;
import org.yaml.snakeyaml.nodes.Node;
import org.yaml.snakeyaml.nodes.Tag;
import org.yaml.snakeyaml.parser.Parser;
import org.yaml.snakeyaml.parser.ParserImpl;
import org.yaml.snakeyaml.reader.StreamReader;
import org.yaml.snakeyaml.reader.UnicodeReader;
import org.yaml.snakeyaml.representer.Representer;
import org.yaml.snakeyaml.resolver.Resolver;
import org.yaml.snakeyaml.serializer.Serializer;
/**
* Public YAML interface. Each Thread must have its own instance.
*/
public class Yaml {
protected final Resolver resolver;
private String name;
protected BaseConstructor constructor;
protected Representer representer;
protected DumperOptions dumperOptions;
protected LoaderOptions loaderOptions;
/**
* Create Yaml instance. It is safe to create a few instances and use them
* in different Threads.
*/
public Yaml() {
this(new Constructor(), new LoaderOptions(), new Representer(), new DumperOptions(),
new Resolver());
}
public Yaml(LoaderOptions loaderOptions) {
this(new Constructor(), loaderOptions, new Representer(), new DumperOptions(),
new Resolver());
}
/**
* Create Yaml instance.
*
* @param dumperOptions
* DumperOptions to configure outgoing objects
*/
public Yaml(DumperOptions dumperOptions) {
this(new Constructor(), new Representer(), dumperOptions);
}
/**
* Create Yaml instance. It is safe to create a few instances and use them
* in different Threads.
*
* @param representer
* Representer to emit outgoing objects
*/
public Yaml(Representer representer) {
this(new Constructor(), representer);
}
/**
* Create Yaml instance. It is safe to create a few instances and use them
* in different Threads.
*
* @param constructor
* BaseConstructor to construct incoming documents
*/
public Yaml(BaseConstructor constructor) {
this(constructor, new Representer());
}
/**
* Create Yaml instance. It is safe to create a few instances and use them
* in different Threads.
*
* @param constructor
* BaseConstructor to construct incoming documents
* @param representer
* Representer to emit outgoing objects
*/
public Yaml(BaseConstructor constructor, Representer representer) {
this(constructor, representer, new DumperOptions());
}
/**
* Create Yaml instance. It is safe to create a few instances and use them
* in different Threads.
*
* @param representer
* Representer to emit outgoing objects
* @param dumperOptions
* DumperOptions to configure outgoing objects
*/
public Yaml(Representer representer, DumperOptions dumperOptions) {
this(new Constructor(), representer, dumperOptions, new Resolver());
}
/**
* Create Yaml instance. It is safe to create a few instances and use them
* in different Threads.
*
* @param constructor
* BaseConstructor to construct incoming documents
* @param representer
* Representer to emit outgoing objects
* @param dumperOptions
* DumperOptions to configure outgoing objects
*/
public Yaml(BaseConstructor constructor, Representer representer, DumperOptions dumperOptions) {
this(constructor, representer, dumperOptions, new Resolver());
}
/**
* Create Yaml instance. It is safe to create a few instances and use them
* in different Threads.
*
* @param constructor
* BaseConstructor to construct incoming documents
* @param representer
* Representer to emit outgoing objects
* @param dumperOptions
* DumperOptions to configure outgoing objects
* @param resolver
* Resolver to detect implicit type
*/
public Yaml(BaseConstructor constructor, Representer representer, DumperOptions dumperOptions,
Resolver resolver) {
this(constructor, new LoaderOptions(), representer, dumperOptions, resolver);
}
/**
* Create Yaml instance. It is safe to create a few instances and use them
* in different Threads.
*
* @param constructor
* BaseConstructor to construct incoming documents
* @param loaderOptions
* LoaderOptions to control construction process
* @param representer
* Representer to emit outgoing objects
* @param dumperOptions
* DumperOptions to configure outgoing objects
* @param resolver
* Resolver to detect implicit type
*/
public Yaml(BaseConstructor constructor, LoaderOptions loaderOptions, Representer representer,
DumperOptions dumperOptions, Resolver resolver) {
if (!constructor.isExplicitPropertyUtils()) {
constructor.setPropertyUtils(representer.getPropertyUtils());
} else if (!representer.isExplicitPropertyUtils()) {
representer.setPropertyUtils(constructor.getPropertyUtils());
}
this.constructor = constructor;
this.loaderOptions = loaderOptions;
representer.setDefaultFlowStyle(dumperOptions.getDefaultFlowStyle());
representer.setDefaultScalarStyle(dumperOptions.getDefaultScalarStyle());
representer.getPropertyUtils().setAllowReadOnlyProperties(
dumperOptions.isAllowReadOnlyProperties());
this.representer = representer;
this.dumperOptions = dumperOptions;
this.resolver = resolver;
this.name = "Yaml:" + System.identityHashCode(this);
}
/**
* Serialize a Java object into a YAML String.
*
* @param data
* Java object to be Serialized to YAML
* @return YAML String
*/
public String dump(Object data) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy