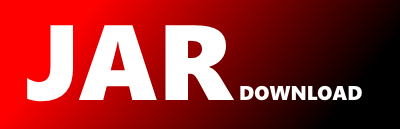
org.yatech.jedis.utils.lua.JedisCommands Maven / Gradle / Ivy
Show all versions of jedis-utils Show documentation
package org.yatech.jedis.utils.lua;
import java.util.Map;
/**
* Created by Yinon Avraham on 01/09/2015.
*/
public interface JedisCommands {
// +----+
// | DB |
// +----+
// SELECT
/**
* Select the DB with having the specified zero-based numeric index.
* @param index
* @return
*/
VoidReturnType select(int index);
/**
* Select the DB with having the specified zero-based numeric index.
* @param index
* @return
*/
VoidReturnType select(LuaValue index);
// +------+
// | KEYS |
// +------+
// DEL
/**
* Removes the specified key. A key is ignored if it does not exist.
* @param key
* @return
*/
VoidReturnType del(String key);
/**
* Removes the specified key. A key is ignored if it does not exist.
* @param key
* @return
*/
VoidReturnType del(LuaValue key);
// TODO [YA] EXISTS
// EXPIRE
/**
* Set a key's time to live in seconds
* @param key
* @param seconds
* @return
*/
VoidReturnType expire(String key, int seconds);
/**
* Set a key's time to live in seconds
* @param key
* @param seconds
* @return
*/
VoidReturnType expire(String key, LuaValue seconds);
/**
* Set a key's time to live in seconds
* @param key
* @param seconds
* @return
*/
VoidReturnType expire(LuaValue key, int seconds);
/**
* Set a key's time to live in seconds
* @param key
* @param seconds
* @return
*/
VoidReturnType expire(LuaValue key, LuaValue seconds);
// EXPIREAT
/**
* Set the expiration for a key as a UNIX timestamp
* @param key
* @param timestamp
* @return
*/
VoidReturnType expireAt(String key, long timestamp);
/**
* Set the expiration for a key as a UNIX timestamp
* @param key
* @param timestamp
* @return
*/
VoidReturnType expireAt(String key, LuaValue timestamp);
/**
* Set the expiration for a key as a UNIX timestamp
* @param key
* @param timestamp
* @return
*/
VoidReturnType expireAt(LuaValue key, long timestamp);
/**
* Set the expiration for a key as a UNIX timestamp
* @param key
* @param timestamp
* @return
*/
VoidReturnType expireAt(LuaValue key, LuaValue timestamp);
// KEYS
/**
* Find all keys matching the pattern
* @param pattern
* @return
*/
LuaLocalArray keys(String pattern);
/**
* Find all keys matching the pattern
* @param pattern
* @return
*/
LuaLocalArray keys(LuaValue pattern);
// MOVE
/**
* Move a key to another database
* @param key
* @param db
* @return
*/
VoidReturnType move(String key, int db);
/**
* Move a key to another database
* @param key
* @param db
* @return
*/
VoidReturnType move(String key, LuaValue db);
/**
* Move a key to another database
* @param key
* @param db
* @return
*/
VoidReturnType move(LuaValue key, int db);
/**
* Move a key to another database
* @param key
* @param db
* @return
*/
VoidReturnType move(LuaValue key, LuaValue db);
// DEL
/**
* Remove the expiration from a key
* @param key
* @return
*/
VoidReturnType persist(String key);
/**
* Remove the expiration from a key
* @param key
* @return
*/
VoidReturnType persist(LuaValue key);
// PEXPIRE
/**
* Set a key's time to live in milliseconds
* @param key
* @param milliseconds
* @return
*/
VoidReturnType pexpire(String key, long milliseconds);
/**
* Set a key's time to live in milliseconds
* @param key
* @param milliseconds
* @return
*/
VoidReturnType pexpire(String key, LuaValue milliseconds);
/**
* Set a key's time to live in milliseconds
* @param key
* @param milliseconds
* @return
*/
VoidReturnType pexpire(LuaValue key, long milliseconds);
/**
* Set a key's time to live in milliseconds
* @param key
* @param milliseconds
* @return
*/
VoidReturnType pexpire(LuaValue key, LuaValue milliseconds);
// PEXPIREAT
/**
* Set the expiration for a key as a UNIX timestamp specified in milliseconds
* @param key
* @param timestamp
* @return
*/
VoidReturnType pexpireAt(String key, long timestamp);
/**
* Set the expiration for a key as a UNIX timestamp specified in milliseconds
* @param key
* @param timestamp
* @return
*/
VoidReturnType pexpireAt(String key, LuaValue timestamp);
/**
* Set the expiration for a key as a UNIX timestamp specified in milliseconds
* @param key
* @param timestamp
* @return
*/
VoidReturnType pexpireAt(LuaValue key, long timestamp);
/**
* Set the expiration for a key as a UNIX timestamp specified in milliseconds
* @param key
* @param timestamp
* @return
*/
VoidReturnType pexpireAt(LuaValue key, LuaValue timestamp);
// PTTL
/**
* Get the time to live for a key in milliseconds
* @param key
* @return
*/
LuaLocalValue pttl(String key);
/**
* Get the time to live for a key in milliseconds
* @param key
* @return
*/
LuaLocalValue pttl(LuaValue key);
// RANDOMKEY
/**
* Return a random key from the keyspace
* @return
*/
LuaLocalValue randomKey();
// RENAME
/**
* Rename a key
* @param key
* @param newKey
* @return
*/
VoidReturnType rename(String key, String newKey);
/**
* Rename a key
* @param key
* @param newKey
* @return
*/
VoidReturnType rename(String key, LuaValue newKey);
/**
* Rename a key
* @param key
* @param newKey
* @return
*/
VoidReturnType rename(LuaValue key, String newKey);
/**
* Rename a key
* @param key
* @param newKey
* @return
*/
VoidReturnType rename(LuaValue key, LuaValue newKey);
// RENAMENX
/**
* Rename a key, only if the new key does not exist
* @param key
* @param newKey
* @return
*/
VoidReturnType renamenx(String key, String newKey);
/**
* Rename a key, only if the new key does not exist
* @param key
* @param newKey
* @return
*/
VoidReturnType renamenx(String key, LuaValue newKey);
/**
* Rename a key, only if the new key does not exist
* @param key
* @param newKey
* @return
*/
VoidReturnType renamenx(LuaValue key, String newKey);
/**
* Rename a key, only if the new key does not exist
* @param key
* @param newKey
* @return
*/
VoidReturnType renamenx(LuaValue key, LuaValue newKey);
// PTTL
/**
* Get the time to live for a key
* @param key
* @return
*/
LuaLocalValue ttl(String key);
/**
* Get the time to live for a key
* @param key
* @return
*/
LuaLocalValue ttl(LuaValue key);
// TYPE
/**
* Determine the type stored at key
* @param key
* @return
*/
LuaLocalValue type(String key);
/**
* Determine the type stored at key
* @param key
* @return
*/
LuaLocalValue type(LuaValue key);
// +------+
// | HASH |
// +------+
// HDEL
/**
* Delete one or more hash fields
* @param key
* @param field
* @param moreFields
* @return
*/
VoidReturnType hdel(String key, String field, String... moreFields);
/**
* Delete one or more hash fields
* @param key
* @param field
* @param moreFields
* @return
*/
VoidReturnType hdel(String key, LuaValue field, LuaValue... moreFields);
/**
* Delete one or more hash fields
* @param key
* @param field
* @param moreFields
* @return
*/
VoidReturnType hdel(LuaValue key, String field, String... moreFields);
/**
* Delete one or more hash fields
* @param key
* @param field
* @param moreFields
* @return
*/
VoidReturnType hdel(LuaValue key, LuaValue field, LuaValue... moreFields);
// TODO [YA] HEXISTS
// HGET
/**
* Get the value of a hash field
* @param key
* @param field
* @return
*/
LuaLocalValue hget(String key, String field);
/**
* Get the value of a hash field
* @param key
* @param field
* @return
*/
LuaLocalValue hget(String key, LuaValue field);
/**
* Get the value of a hash field
* @param key
* @param field
* @return
*/
LuaLocalValue hget(LuaValue key, String field);
/**
* Get the value of a hash field
* @param key
* @param field
* @return
*/
LuaLocalValue hget(LuaValue key, LuaValue field);
// HGETALL
/**
* Returns all fields and values of the hash stored at key.
* @param key
* @return
*/
LuaLocalArray hgetAll(String key);
/**
* Returns all fields and values of the hash stored at key.
* @param key
* @return
*/
LuaLocalArray hgetAll(LuaValue key);
// HINCRBY
/**
* Increment the numeric value of a hash field by the given number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrBy(String key, String field, long increment);
/**
* Increment the numeric value of a hash field by the given number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrBy(String key, String field, LuaValue increment);
/**
* Increment the numeric value of a hash field by the given number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrBy(String key, LuaValue field, long increment);
/**
* Increment the numeric value of a hash field by the given number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrBy(String key, LuaValue field, LuaValue increment);
/**
* Increment the numeric value of a hash field by the given number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrBy(LuaValue key, String field, long increment);
/**
* Increment the numeric value of a hash field by the given number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrBy(LuaValue key, String field, LuaValue increment);
/**
* Increment the numeric value of a hash field by the given number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrBy(LuaValue key, LuaValue field, long increment);
/**
* Increment the numeric value of a hash field by the given number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrBy(LuaValue key, LuaValue field, LuaValue increment);
// HINCRBYFLOAT
/**
* Increment the numeric value of a hash field by the given double precision floating point number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrByFloat(String key, String field, double increment);
/**
* Increment the numeric value of a hash field by the given double precision floating point number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrByFloat(String key, String field, LuaValue increment);
/**
* Increment the numeric value of a hash field by the given double precision floating point number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrByFloat(String key, LuaValue field, double increment);
/**
* Increment the numeric value of a hash field by the given double precision floating point number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrByFloat(String key, LuaValue field, LuaValue increment);
/**
* Increment the numeric value of a hash field by the given double precision floating point number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrByFloat(LuaValue key, String field, double increment);
/**
* Increment the numeric value of a hash field by the given double precision floating point number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrByFloat(LuaValue key, String field, LuaValue increment);
/**
* Increment the numeric value of a hash field by the given double precision floating point number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrByFloat(LuaValue key, LuaValue field, double increment);
/**
* Increment the numeric value of a hash field by the given double precision floating point number
* @param key
* @param field
* @param increment
* @return
*/
VoidReturnType hincrByFloat(LuaValue key, LuaValue field, LuaValue increment);
// HMSET
/**
* Sets the specified fields to their respective values in the hash stored at key.
* This command overwrites any existing fields in the hash.
* If key does not exist, a new key holding a hash is created.
* @param key
* @param hash
* @return
*/
VoidReturnType hmset(String key, Map hash);
/**
* Sets the specified fields to their respective values in the hash stored at key.
* This command overwrites any existing fields in the hash.
* If key does not exist, a new key holding a hash is created.
* @param key
* @param hash
* @return
*/
VoidReturnType hmset(LuaValue key, Map hash);
/**
* Sets the specified fields to their respective values in the hash stored at key.
* This command overwrites any existing fields in the hash.
* If key does not exist, a new key holding a hash is created.
* @param key
* @param hash
* @return
*/
VoidReturnType hmset(String key, LuaLocalArray hash);
/**
* Sets the specified fields to their respective values in the hash stored at key.
* This command overwrites any existing fields in the hash.
* If key does not exist, a new key holding a hash is created.
* @param key
* @param hash
* @return
*/
VoidReturnType hmset(LuaValue key, LuaLocalArray hash);
// +---------+
// | STRINGS |
// +---------+
// SET
/**
* Set the string value of a key
* @param key
* @param value
* @return
*/
VoidReturnType set(String key, String value);
/**
* Set the string value of a key
* @param key
* @param value
* @return
*/
VoidReturnType set(String key, LuaValue value);
/**
* Set the string value of a key
* @param key
* @param value
* @return
*/
VoidReturnType set(LuaValue key, String value);
/**
* Set the string value of a key
* @param key
* @param value
* @return
*/
VoidReturnType set(LuaValue key, LuaValue value);
// GET
/**
* Get the value of a key
* @param key
* @return
*/
LuaLocalValue get(String key);
/**
* Get the value of a key
* @param key
* @return
*/
LuaLocalValue get(LuaValue key);
// +------+
// | ZSET |
// +------+
// ZADD
/**
* Add the specified member having the specifeid score to the sorted set stored at key. If member
* is already a member of the sorted set the score is updated, and the element reinserted in the
* right position to ensure sorting. If key does not exist a new sorted set with the specified
* member as sole member is crated. If the key exists but does not hold a sorted set value an
* error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
*
* @param key
* @param score
* @param member
* @return
*/
VoidReturnType zadd(String key, double score, String member);
/**
* Add the specified member having the specifeid score to the sorted set stored at key. If member
* is already a member of the sorted set the score is updated, and the element reinserted in the
* right position to ensure sorting. If key does not exist a new sorted set with the specified
* member as sole member is crated. If the key exists but does not hold a sorted set value an
* error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
*
* @param key
* @param score
* @param member
* @return
*/
VoidReturnType zadd(String key, double score, LuaValue member);
/**
* Add the specified member having the specifeid score to the sorted set stored at key. If member
* is already a member of the sorted set the score is updated, and the element reinserted in the
* right position to ensure sorting. If key does not exist a new sorted set with the specified
* member as sole member is crated. If the key exists but does not hold a sorted set value an
* error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
*
* @param key
* @param score
* @param member
* @return
*/
VoidReturnType zadd(String key, LuaValue score, String member);
/**
* Add the specified member having the specifeid score to the sorted set stored at key. If member
* is already a member of the sorted set the score is updated, and the element reinserted in the
* right position to ensure sorting. If key does not exist a new sorted set with the specified
* member as sole member is crated. If the key exists but does not hold a sorted set value an
* error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
*
* @param key
* @param score
* @param member
* @return
*/
VoidReturnType zadd(String key, LuaValue score, LuaValue member);
/**
* Add the specified member having the specifeid score to the sorted set stored at key. If member
* is already a member of the sorted set the score is updated, and the element reinserted in the
* right position to ensure sorting. If key does not exist a new sorted set with the specified
* member as sole member is crated. If the key exists but does not hold a sorted set value an
* error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
*
* @param key
* @param score
* @param member
* @return
*/
VoidReturnType zadd(LuaValue key, double score, String member);
/**
* Add the specified member having the specifeid score to the sorted set stored at key. If member
* is already a member of the sorted set the score is updated, and the element reinserted in the
* right position to ensure sorting. If key does not exist a new sorted set with the specified
* member as sole member is crated. If the key exists but does not hold a sorted set value an
* error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
*
* @param key
* @param score
* @param member
* @return
*/
VoidReturnType zadd(LuaValue key, double score, LuaValue member);
/**
* Add the specified member having the specifeid score to the sorted set stored at key. If member
* is already a member of the sorted set the score is updated, and the element reinserted in the
* right position to ensure sorting. If key does not exist a new sorted set with the specified
* member as sole member is crated. If the key exists but does not hold a sorted set value an
* error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
*
* @param key
* @param score
* @param member
* @return
*/
VoidReturnType zadd(LuaValue key, LuaValue score, String member);
/**
* Add the specified member having the specifeid score to the sorted set stored at key. If member
* is already a member of the sorted set the score is updated, and the element reinserted in the
* right position to ensure sorting. If key does not exist a new sorted set with the specified
* member as sole member is crated. If the key exists but does not hold a sorted set value an
* error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
*
* @param key
* @param score
* @param member
* @return
*/
VoidReturnType zadd(LuaValue key, LuaValue score, LuaValue member);
/**
* Adds all the specified members with the specified scores to the sorted set stored at key.
* It is possible to specify multiple score / member pairs. If a specified member is already
* a member of the sorted set, the score is updated and the element reinserted at the right
* position to ensure the correct ordering.
*
* If key does not exist, a new sorted set with the specified members as sole members is created,
* like if the sorted set was empty. If the key exists but does not hold a sorted set, an error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
* @param key
* @param scoreMembers
* @return
*/
VoidReturnType zadd(String key, Map scoreMembers);
/**
* Adds all the specified members with the specified scores to the sorted set stored at key.
* It is possible to specify multiple score / member pairs. If a specified member is already
* a member of the sorted set, the score is updated and the element reinserted at the right
* position to ensure the correct ordering.
*
* If key does not exist, a new sorted set with the specified members as sole members is created,
* like if the sorted set was empty. If the key exists but does not hold a sorted set, an error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
* @param key
* @param scoreMembers
* @return
*/
VoidReturnType zadd(LuaValue key, Map scoreMembers);
/**
* Adds all the specified members with the specified scores to the sorted set stored at key.
* It is possible to specify multiple score / member pairs. If a specified member is already
* a member of the sorted set, the score is updated and the element reinserted at the right
* position to ensure the correct ordering.
*
* If key does not exist, a new sorted set with the specified members as sole members is created,
* like if the sorted set was empty. If the key exists but does not hold a sorted set, an error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
* @param key
* @param scoreMembers
* @return
*/
VoidReturnType zadd(String key, LuaLocalArray scoreMembers);
/**
* Adds all the specified members with the specified scores to the sorted set stored at key.
* It is possible to specify multiple score / member pairs. If a specified member is already
* a member of the sorted set, the score is updated and the element reinserted at the right
* position to ensure the correct ordering.
*
* If key does not exist, a new sorted set with the specified members as sole members is created,
* like if the sorted set was empty. If the key exists but does not hold a sorted set, an error is returned.
*
* Time complexity O(log(N)) with N being the number of elements in the sorted set
* @param key
* @param scoreMembers
* @return
*/
VoidReturnType zadd(LuaValue key, LuaLocalArray scoreMembers);
// ZSCORE
/**
* Returns the score of member in the sorted set at key.
* @param key
* @param member
* @return A local representing the score value.
* If member does not exist in the sorted set, or key does not exist, nil
is returned.
*/
LuaLocalValue zscore(String key, String member);
/**
* Returns the score of member in the sorted set at key.
* @param key
* @param member
* @return A local representing the score value.
* If member does not exist in the sorted set, or key does not exist, nil
is returned.
*/
LuaLocalValue zscore(LuaValue key, String member);
/**
* Returns the score of member in the sorted set at key.
* @param key
* @param member
* @return A local representing the score value.
* If member does not exist in the sorted set, or key does not exist, nil
is returned.
*/
LuaLocalValue zscore(String key, LuaValue member);
/**
* Returns the score of member in the sorted set at key.
* @param key
* @param member
* @return A local representing the score value.
* If member does not exist in the sorted set, or key does not exist, nil
is returned.
*/
LuaLocalValue zscore(LuaValue key, LuaValue member);
}