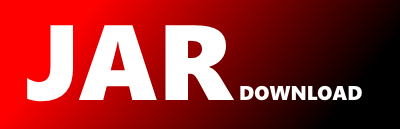
org.yestech.lib.camel.TerracottaEndpoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yeslib Show documentation
Show all versions of yeslib Show documentation
A collection of classes that can be used across yestech artifacts/components, but must not be dependant
on any yestech component. Most of the code is utility type code. When more than a few classes are
found to be in a package or the package start to handle more that a few reposibilities then a new
independant component is created and the existing code in yeslib is ported to the new component.
The newest version!
/*
* Copyright LGPL3
* YES Technology Association
* http://yestech.org
*
* http://www.opensource.org/licenses/lgpl-3.0.html
*/
package org.yestech.lib.camel;
import java.util.HashSet;
import java.util.Set;
import java.util.concurrent.CopyOnWriteArraySet;
import org.apache.camel.Component;
import org.apache.camel.Consumer;
import org.apache.camel.Processor;
import org.apache.camel.Producer;
import org.apache.camel.impl.DefaultEndpoint;
import org.terracotta.message.pipe.Pipe;
import org.terracotta.modules.annotations.Root;
/**
* An implementation of the asynchronous Pipe exchanges on a {@link org.terracotta.message.pipe.Pipe} within a CamelContext
*
* A component based on camel SEDA component.
*/
public class TerracottaEndpoint extends DefaultEndpoint {
@Root
private Pipe
© 2015 - 2025 Weber Informatics LLC | Privacy Policy