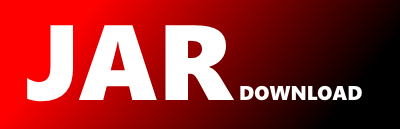
org.yestech.lib.io.AutoFlushingPrintWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yeslib Show documentation
Show all versions of yeslib Show documentation
A collection of classes that can be used across yestech artifacts/components, but must not be dependant
on any yestech component. Most of the code is utility type code. When more than a few classes are
found to be in a package or the package start to handle more that a few reposibilities then a new
independant component is created and the existing code in yeslib is ported to the new component.
The newest version!
/*
* Copyright LGPL3
* YES Technology Association
* http://yestech.org
*
* http://www.opensource.org/licenses/lgpl-3.0.html
*/
package org.yestech.lib.io;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.PrintWriter;
import java.io.OutputStream;
/**
* A PrintWriter that auto flush all calls after delegating to parent class.
*
*/
public class AutoFlushingPrintWriter extends PrintWriter {
final private static Logger logger = LoggerFactory.getLogger(AutoFlushingPrintWriter.class);
/**
* Creates a new PrintWriter, without automatic line flushing, from an
* existing OutputStream. This convenience constructor creates the
* necessary intermediate OutputStreamWriter, which will convert characters
* into bytes using the default character encoding.
*
* @param out An output stream
* @see java.io.OutputStreamWriter#OutputStreamWriter(java.io.OutputStream)
*/
public AutoFlushingPrintWriter(OutputStream out) {
super(out);
}
/**
* Writes A Portion of an array of characters.
*
* @param buf Array of characters
* @param off Offset from which to start writing characters
* @param len Number of characters to write
*/
@Override
public void write(char[] buf, int off, int len) {
super.write(buf, off, len);
super.flush();
}
/**
* Writes a single character.
*
* @param c int specifying a character to be written.
*/
@Override
public void write(int c) {
super.write(c);
super.flush();
}
/**
* Writes an array of characters. This method cannot be inherited from the
* Writer class because it must suppress I/O exceptions.
*
* @param buf Array of characters to be written
*/
@Override
public void write(char[] buf) {
super.write(buf);
super.flush();
}
/**
* Writes a portion of a string.
*
* @param s A String
* @param off Offset from which to start writing characters
* @param len Number of characters to write
*/
@Override
public void write(String s, int off, int len) {
super.write(s, off, len);
super.flush();
}
/**
* Writes a string. This method cannot be inherited from the Writer class
* because it must suppress I/O exceptions.
*
* @param s String to be written
*/
@Override
public void write(String s) {
super.write(s);
super.flush();
}
/**
* Prints a boolean value. The string produced by {@link
* String#valueOf(boolean)}
is translated into bytes
* according to the platform's default character encoding, and these bytes
* are written in exactly the manner of the {@link
* #write(int)}
method.
*
* @param b The boolean
to be printed
*/
@Override
public void print(boolean b) {
super.print(b);
super.flush();
}
/**
* Prints a character. The character is translated into one or more bytes
* according to the platform's default character encoding, and these bytes
* are written in exactly the manner of the {@link
* #write(int)}
method.
*
* @param c The char
to be printed
*/
@Override
public void print(char c) {
super.print(c);
super.flush();
}
/**
* Prints an integer. The string produced by {@link
* String#valueOf(int)}
is translated into bytes according
* to the platform's default character encoding, and these bytes are
* written in exactly the manner of the {@link #write(int)}
* method.
*
* @param i The int
to be printed
* @see Integer#toString(int)
*/
@Override
public void print(int i) {
super.print(i);
super.flush();
}
/**
* Prints a long integer. The string produced by {@link
* String#valueOf(long)}
is translated into bytes
* according to the platform's default character encoding, and these bytes
* are written in exactly the manner of the {@link #write(int)}
* method.
*
* @param l The long
to be printed
* @see Long#toString(long)
*/
@Override
public void print(long l) {
super.print(l);
super.flush();
}
/**
* Prints a floating-point number. The string produced by {@link
* String#valueOf(float)}
is translated into bytes
* according to the platform's default character encoding, and these bytes
* are written in exactly the manner of the {@link #write(int)}
* method.
*
* @param f The float
to be printed
* @see Float#toString(float)
*/
@Override
public void print(float f) {
super.print(f);
super.flush();
}
/**
* Prints a double-precision floating-point number. The string produced by
* {@link String#valueOf(double)}
is translated into
* bytes according to the platform's default character encoding, and these
* bytes are written in exactly the manner of the {@link
* #write(int)}
method.
*
* @param d The double
to be printed
* @see Double#toString(double)
*/
@Override
public void print(double d) {
super.print(d);
super.flush();
}
/**
* Prints an array of characters. The characters are converted into bytes
* according to the platform's default character encoding, and these bytes
* are written in exactly the manner of the {@link #write(int)}
* method.
*
* @param s The array of chars to be printed
* @throws NullPointerException If s
is null
*/
@Override
public void print(char[] s) {
super.print(s);
super.flush();
}
/**
* Prints a string. If the argument is null
then the string
* "null"
is printed. Otherwise, the string's characters are
* converted into bytes according to the platform's default character
* encoding, and these bytes are written in exactly the manner of the
* {@link #write(int)}
method.
*
* @param s The String
to be printed
*/
@Override
public void print(String s) {
super.print(s);
super.flush();
}
/**
* Prints an object. The string produced by the {@link
* String#valueOf(Object)}
method is translated into bytes
* according to the platform's default character encoding, and these bytes
* are written in exactly the manner of the {@link #write(int)}
* method.
*
* @param obj The Object
to be printed
* @see Object#toString()
*/
@Override
public void print(Object obj) {
super.print(obj);
super.flush();
}
/**
* Terminates the current line by writing the line separator string. The
* line separator string is defined by the system property
* line.separator
, and is not necessarily a single newline
* character ('\n'
).
*/
@Override
public void println() {
super.println();
super.flush();
}
/**
* Prints a boolean value and then terminates the line. This method behaves
* as though it invokes {@link #print(boolean)}
and then
* {@link #println()}
.
*
* @param x the boolean
value to be printed
*/
@Override
public void println(boolean x) {
super.println(x);
super.flush();
}
/**
* Prints a character and then terminates the line. This method behaves as
* though it invokes {@link #print(char)}
and then {@link
* #println()}
.
*
* @param x the char
value to be printed
*/
@Override
public void println(char x) {
super.println(x);
super.flush();
}
/**
* Prints an integer and then terminates the line. This method behaves as
* though it invokes {@link #print(int)}
and then {@link
* #println()}
.
*
* @param x the int
value to be printed
*/
@Override
public void println(int x) {
super.println(x);
super.flush();
}
/**
* Prints a long integer and then terminates the line. This method behaves
* as though it invokes {@link #print(long)}
and then
* {@link #println()}
.
*
* @param x the long
value to be printed
*/
@Override
public void println(long x) {
super.println(x);
super.flush();
}
/**
* Prints a floating-point number and then terminates the line. This method
* behaves as though it invokes {@link #print(float)}
and then
* {@link #println()}
.
*
* @param x the float
value to be printed
*/
@Override
public void println(float x) {
super.println(x);
super.flush();
}
/**
* Prints a double-precision floating-point number and then terminates the
* line. This method behaves as though it invokes {@link
* #print(double)}
and then {@link #println()}
.
*
* @param x the double
value to be printed
*/
@Override
public void println(double x) {
super.println(x);
super.flush();
}
/**
* Prints an array of characters and then terminates the line. This method
* behaves as though it invokes {@link #print(char[])}
and then
* {@link #println()}
.
*
* @param x the array of char
values to be printed
*/
@Override
public void println(char[] x) {
super.println(x);
super.flush();
}
/**
* Prints a String and then terminates the line. This method behaves as
* though it invokes {@link #print(String)}
and then
* {@link #println()}
.
*
* @param x the String
value to be printed
*/
@Override
public void println(String x) {
super.println(x);
super.flush();
}
/**
* Prints an Object and then terminates the line. This method calls
* at first String.valueOf(x) to get the printed object's string value,
* then behaves as
* though it invokes {@link #print(String)}
and then
* {@link #println()}
.
*
* @param x The Object
to be printed.
*/
@Override
public void println(Object x) {
super.println(x);
super.flush();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy