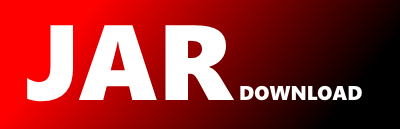
org.yestech.notify.service.MemoryQueueNotificationProducer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yesnotify Show documentation
Show all versions of yesnotify Show documentation
Framework to sent emails using templates.
Currently xsl and velocity template engines are supported.
/*
* Copyright LGPL3
* YES Technology Association
* http://yestech.org
*
* http://www.opensource.org/licenses/lgpl-3.0.html
*/
/*
*
* Original Author: Artie Copeland
* Last Modified Date: $DateTime: $
*/
package org.yestech.notify.service;
import org.yestech.notify.objectmodel.INotificationJob;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.concurrent.LinkedBlockingQueue;
/**
* @author $Author: $
* @version $Revision: $
*/
public class MemoryQueueNotificationProducer implements INotificationProducer {
final private static Logger logger = LoggerFactory.getLogger(MemoryQueueNotificationProducer.class);
private LinkedBlockingQueue queue;
public LinkedBlockingQueue getQueue() {
return queue;
}
public void setQueue(LinkedBlockingQueue queue) {
this.queue = queue;
}
@Override
public void send(INotificationJob notificationJob) {
if (notificationJob != null) {
try {
queue.put(notificationJob);
} catch (InterruptedException e) {
logger.error("error enqueue", e);
}
}
}
}