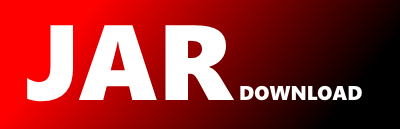
org.zalando.fauxpas.TryWith Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of faux-pas Show documentation
Show all versions of faux-pas Show documentation
Error handling in Functional Programming
The newest version!
package org.zalando.fauxpas;
import lombok.SneakyThrows;
import org.apiguardian.api.API;
import javax.annotation.Nullable;
import static org.apiguardian.api.API.Status.MAINTAINED;
import static org.apiguardian.api.API.Status.STABLE;
public final class TryWith {
private TryWith() {
}
@API(status = MAINTAINED)
public static void tryWith(
@Nullable final O outer, @Nullable final I inner, final ThrowingBiConsumer consumer) throws X {
tryWith(outer, a -> {
tryWith(inner, b -> {
consumer.tryAccept(a, b);
});
});
}
@API(status = STABLE)
public static void tryWith(@Nullable final R resource,
final ThrowingConsumer consumer) throws X {
try {
consumer.tryAccept(resource);
} catch (final Throwable e) {
throw tryClose(resource, TryWith.cast(e));
}
tryClose(resource);
}
@API(status = MAINTAINED)
public static T tryWith(
@Nullable final O outer, @Nullable final I inner, final ThrowingBiFunction function) throws X {
// not exactly sure why those explicit type parameters are needed
return TryWith.tryWith(outer, a ->
tryWith(inner, b -> {
return function.tryApply(a, b);
}));
}
@API(status = STABLE)
public static T tryWith(@Nullable final R resource,
final ThrowingFunction supplier) throws X {
final T value;
try {
value = supplier.tryApply(resource);
} catch (final Throwable e) {
throw tryClose(resource, TryWith.cast(e));
}
tryClose(resource);
return value;
}
@SneakyThrows
private static void tryClose(@Nullable final AutoCloseable resource) {
if (resource == null) {
return;
}
resource.close();
}
@SuppressWarnings("unchecked")
private static X cast(final Throwable e) {
return (X) e;
}
private static X tryClose(@Nullable final AutoCloseable closeable, final X e) {
if (closeable == null) {
return e;
}
try {
closeable.close();
} catch (final Throwable inner) {
e.addSuppressed(inner);
}
return e;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy