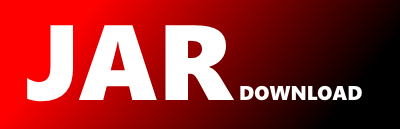
org.zalando.logbook.BaseHttpMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of logbook-api Show documentation
Show all versions of logbook-api Show documentation
HTTP request and response logging
package org.zalando.logbook;
import javax.annotation.Nullable;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
import static java.lang.String.CASE_INSENSITIVE_ORDER;
public interface BaseHttpMessage {
String getProtocolVersion();
Origin getOrigin();
Map> getHeaders();
@Nullable
String getContentType();
Charset getCharset();
class HeadersBuilder {
private Map> headers;
public HeadersBuilder() {
// package private so we can trick code coverage
headers = new TreeMap<>(CASE_INSENSITIVE_ORDER);
}
public HeadersBuilder put(final String key, final String value) {
final List values = headers.get(key);
if (values != null) {
values.add(value);
} else {
final ArrayList list = new ArrayList<>();
list.add(value);
headers.put(key, list);
}
return this;
}
public HeadersBuilder put(final String key, final Iterable values) {
for (final String value : values) {
put(key, value);
}
return this;
}
public Map> build() {
for (final Map.Entry> e : headers.entrySet()) {
e.setValue(Collections.unmodifiableList(e.getValue()));
}
headers = Collections.unmodifiableMap(headers);
return headers;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy