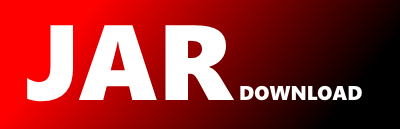
org.zalando.logbook.ApplyHttpHeaders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of logbook-api Show documentation
Show all versions of logbook-api Show documentation
HTTP request and response logging
package org.zalando.logbook;
import com.google.gag.annotation.remark.ThisWouldBeOneLineIn;
import javax.annotation.Nullable;
import java.util.Collection;
import java.util.List;
import java.util.function.BiFunction;
import java.util.function.BiPredicate;
import java.util.function.UnaryOperator;
import static java.util.Collections.singleton;
import static org.zalando.logbook.Fold.fold;
interface ApplyHttpHeaders extends HttpHeaders {
@Override
default HttpHeaders apply(
final String name,
final UnaryOperator> operator) {
return apply(singleton(name), (ignored, previous) ->
operator.apply(previous));
}
@Override
default HttpHeaders apply(
final Collection names,
final BiFunction, Collection> operator) {
final HttpHeaders self = this;
return fold(names, self, (result, name) -> {
final List previous = get(name);
return applyTo(operator, name, previous, result);
});
}
@Override
default HttpHeaders apply(
final BiPredicate> predicate,
final BiFunction, Collection> operator) {
return apply((name, previous) -> {
if (predicate.test(name, previous)) {
return operator.apply(name, previous);
}
return previous;
});
}
@Override
default HttpHeaders apply(
final BiFunction, Collection> operator) {
final HttpHeaders self = this;
return fold(entrySet(), self, (result, entry) -> {
final String name = entry.getKey();
final List previous = entry.getValue();
return applyTo(operator, name, previous, result);
});
}
// Effectively package-private because this interface is and so are all
// implementations of it. Ideally it would be private
@ThisWouldBeOneLineIn(language = "Java 9", toWit = "private")
default HttpHeaders applyTo(
final BiFunction, Collection> operator,
final String name,
final List previous,
final HttpHeaders headers) {
@Nullable final Collection next =
operator.apply(name, previous);
return next == null ?
headers.delete(name) :
headers.update(name, next);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy