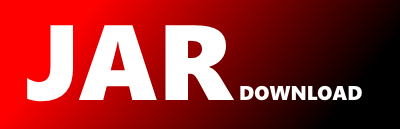
org.zalando.logbook.HttpHeaders Maven / Gradle / Ivy
Show all versions of logbook-api Show documentation
package org.zalando.logbook;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.concurrent.Immutable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.BiFunction;
import java.util.function.BiPredicate;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.UnaryOperator;
import static org.zalando.logbook.DefaultHttpHeaders.EMPTY;
/**
* An immutable multi-map representing HTTP headers. It offers three kinds of
* operations:
*
* {@code update} is essentially {@link Map#put(Object, Object)}, but returns
* a new instance with the updated entries.
*
* {@code delete} is essentially {@link Map#remove(Object)}, but returns a new
* instance without the deleted headers.
*
* {@code apply} applies a function to all entries or a subset of them and
* applies the changes. If the given operator returns a null value the entry
* will be deleted otherwise updated.
*/
@Immutable
public interface HttpHeaders extends Map> {
@CheckReturnValue
HttpHeaders update(String name, String... values);
@CheckReturnValue
HttpHeaders update(String name, Collection value);
@CheckReturnValue
HttpHeaders update(Map> headers);
@CheckReturnValue
HttpHeaders apply(String name, UnaryOperator> operator);
@CheckReturnValue
HttpHeaders apply(
Collection names,
BiFunction, Collection> operator);
@CheckReturnValue
HttpHeaders apply(
BiPredicate> predicate,
BiFunction, Collection> operator);
@CheckReturnValue
HttpHeaders apply(
BiFunction, Collection> operator);
@CheckReturnValue
HttpHeaders delete(String... names);
@CheckReturnValue
HttpHeaders delete(Collection names);
@CheckReturnValue
HttpHeaders delete(BiPredicate> predicate);
@Nullable
default String getFirst(String name) {
return Optional
.ofNullable(get(name))
.map(it -> it.isEmpty() ? null : it.get(0))
.orElse(null);
}
static HttpHeaders empty() {
return EMPTY;
}
static HttpHeaders of(final String name, final String... values) {
return empty().update(name, values);
}
static HttpHeaders of(final Map> headers) {
return empty().update(headers);
}
static BiPredicate predicate(final Predicate predicate) {
return (t, u) -> predicate.test(t);
}
// deprecated stuff from here on till the end
@Deprecated
@Override
List put(String key, List value);
@Deprecated
@Override
List remove(Object key);
@Deprecated
@Override
void putAll(Map extends String, ? extends List> m);
@Deprecated
@Override
void clear();
@Deprecated
@Override
void replaceAll(BiFunction super String, ? super List, ? extends List> function);
@Deprecated
@Override
List putIfAbsent(String key, List value);
@Deprecated
@Override
boolean remove(Object key, Object value);
@Deprecated
@Override
boolean replace(String key, List oldValue, List newValue);
@Deprecated
@Override
List replace(String key, List value);
@Deprecated
@Override
List computeIfAbsent(String key, Function super String, ? extends List> mappingFunction);
@Deprecated
@Override
List computeIfPresent(String key,
BiFunction super String, ? super List, ? extends List> remappingFunction);
@Deprecated
@Override
List compute(String key,
BiFunction super String, ? super List, ? extends List> remappingFunction);
@Deprecated
@Override
List merge(String key, List value,
BiFunction super List, ? super List, ? extends List> remappingFunction);
}