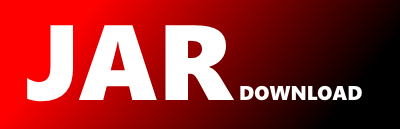
org.zanata.maven.HelpMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zanata-maven-plugin Show documentation
Show all versions of zanata-maven-plugin Show documentation
Zanata client for managing projects, publishing
source text and retrieving translations.
package org.zanata.maven;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
/**
* Display help information on zanata-maven-plugin.
Call mvn zanata:help -Ddetail=true -Dgoal=<goal-name>
to display parameter details.
*
* @version generated on Tue Sep 03 09:21:15 EST 2013
* @author org.apache.maven.tools.plugin.generator.PluginHelpGenerator (version 2.9)
* @goal help
* @requiresProject false
* @threadSafe
*/
@SuppressWarnings( "all" )
public class HelpMojo
extends AbstractMojo
{
/**
* If true
, display all settable properties for each goal.
*
* @parameter expression="${detail}" default-value="false"
*/
private boolean detail;
/**
* The name of the goal for which to show help. If unspecified, all goals will be displayed.
*
* @parameter expression="${goal}"
*/
private java.lang.String goal;
/**
* The maximum length of a display line, should be positive.
*
* @parameter expression="${lineLength}" default-value="80"
*/
private int lineLength;
/**
* The number of spaces per indentation level, should be positive.
*
* @parameter expression="${indentSize}" default-value="2"
*/
private int indentSize;
/** {@inheritDoc} */
public void execute()
throws MojoExecutionException
{
if ( lineLength <= 0 )
{
getLog().warn( "The parameter 'lineLength' should be positive, using '80' as default." );
lineLength = 80;
}
if ( indentSize <= 0 )
{
getLog().warn( "The parameter 'indentSize' should be positive, using '2' as default." );
indentSize = 2;
}
StringBuffer sb = new StringBuffer();
append( sb, "org.zanata:zanata-maven-plugin:3.1.2", 0 );
append( sb, "", 0 );
append( sb, "Zanata Maven Plugin", 0 );
append( sb, "Zanata client for managing projects, publishing source text and retrieving translations.", 1 );
append( sb, "", 0 );
if ( goal == null || goal.length() <= 0 )
{
append( sb, "This plugin has 14 goals:", 0 );
append( sb, "", 0 );
}
if ( goal == null || goal.length() <= 0 || "glossary-delete".equals( goal ) )
{
append( sb, "zanata:glossary-delete", 0 );
append( sb, "Delete glossary entry from Zanata.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "allGlossary (Default: false)", 2 );
append( sb, "Delete entire glossaries", 3 );
append( sb, "Expression: ${zanata.allGlossary}", 3 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "lang", 2 );
append( sb, "Locale of glossary to delete", 3 );
append( sb, "Expression: ${zanata.lang}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "Project slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.project}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "glossary-push".equals( goal ) )
{
append( sb, "zanata:glossary-push", 0 );
append( sb, "Pushes glossary file into Zanata.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "batchSize (Default: 50)", 2 );
append( sb, "Batch size for large glossary file", 3 );
append( sb, "Expression: ${zanata.batchSize}", 3 );
append( sb, "", 0 );
append( sb, "commentCols (Default: pos,description)", 2 );
append( sb, "Customized comment column headers for csv file format. Format of CSV: {source locale},{locale},{locale}...,pos,description OR {source locale},{locale},{locale}...,description1,description2...", 3 );
append( sb, "Expression: ${zanata.commentCols}", 3 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "glossaryFile", 2 );
append( sb, "Location path for the glossary file", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.glossaryFile}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "Project slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.project}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "sourceLang (Default: en-US)", 2 );
append( sb, "Source language of document", 3 );
append( sb, "Expression: ${zanata.sourceLang}", 3 );
append( sb, "", 0 );
append( sb, "transLang", 2 );
append( sb, "Translation language of document. Not required for csv file", 3 );
append( sb, "Expression: ${zanata.transLang}", 3 );
append( sb, "", 0 );
append( sb, "treatSourceCommentsAsTarget (Default: false)", 2 );
append( sb, "Treat source comments and references as target comments in glossary file as translation comment", 3 );
append( sb, "Expression: ${zanata.treatSourceCommentsAsTarget}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "help".equals( goal ) )
{
append( sb, "zanata:help", 0 );
append( sb, "Display help information on zanata-maven-plugin.\nCall\n\u00a0\u00a0mvn\u00a0zanata:help\u00a0-Ddetail=true\u00a0-Dgoal=\nto display parameter details.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "detail (Default: false)", 2 );
append( sb, "If true, display all settable properties for each goal.", 3 );
append( sb, "Expression: ${detail}", 3 );
append( sb, "", 0 );
append( sb, "goal", 2 );
append( sb, "The name of the goal for which to show help. If unspecified, all goals will be displayed.", 3 );
append( sb, "Expression: ${goal}", 3 );
append( sb, "", 0 );
append( sb, "indentSize (Default: 2)", 2 );
append( sb, "The number of spaces per indentation level, should be positive.", 3 );
append( sb, "Expression: ${indentSize}", 3 );
append( sb, "", 0 );
append( sb, "lineLength (Default: 80)", 2 );
append( sb, "The maximum length of a display line, should be positive.", 3 );
append( sb, "Expression: ${lineLength}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "list-remote".equals( goal ) )
{
append( sb, "zanata:list-remote", 0 );
append( sb, "Lists all remote documents in the configured Zanata project version.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "Project slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.project}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "publican-pull".equals( goal ) )
{
append( sb, "zanata:publican-pull", 0 );
append( sb, "Pulls translated text from Zanata. DEPRECATED: use \'pull\' with projectType \'podir\' goal.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "dstDir", 2 );
append( sb, "Base directory for publican files (with subdirectory \'pot\' and optional locale directories), although the location of \'pot\' can be overridden with the dstDirPot option.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.dstDir}", 3 );
append( sb, "", 0 );
append( sb, "dstDirPot (Default: ${zanata.dstDir}/pot)", 2 );
append( sb, "Base directory for pot files.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.dstDirPot}", 3 );
append( sb, "", 0 );
append( sb, "exportPot", 2 );
append( sb, "Export source text from Zanata to local POT files, overwriting or erasing existing POT files (DANGER!)", 3 );
append( sb, "Expression: ${zanata.exportPot}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "Project slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.project}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "publican-push".equals( goal ) )
{
append( sb, "zanata:publican-push", 0 );
append( sb, "Pushes publican source text to a Zanata project version so that it can be translated. DEPRECATED: use \'push\' with projectType \'podir\'.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "copyTrans (Default: true)", 2 );
append( sb, "Whether the server should copy latest translations from equivalent messages/documents in the database.", 3 );
append( sb, "Expression: ${zanata.copyTrans}", 3 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "importPo", 2 );
append( sb, "Import/merge translations from local PO files to the server, overwriting or erasing existing translations (DANGER!)", 3 );
append( sb, "Expression: ${zanata.importPo}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "merge (Default: auto)", 2 );
append( sb, "Merge type: \'auto\' (default) or \'import\' (DANGER!).", 3 );
append( sb, "Expression: ${zanata.merge}", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "Project slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.project}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "sourceLang", 2 );
append( sb, "Language of source (defaults to en-US)", 3 );
append( sb, "Expression: ${zanata.sourceLang}", 3 );
append( sb, "", 0 );
append( sb, "srcDir", 2 );
append( sb, "Base directory for publican files (with subdirectory \'pot\' and optional locale directories), although the location of \'pot\' can be overridden with the srcDirPot option.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.srcDir}", 3 );
append( sb, "", 0 );
append( sb, "srcDirPot (Default: ${zanata.srcDir}/pot)", 2 );
append( sb, "Base directory for pot files.", 3 );
append( sb, "Expression: ${zanata.srcDirPot}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
append( sb, "validate", 2 );
append( sb, "Should the client validate XML before sending request to server (debugging).", 3 );
append( sb, "Expression: ${zanata.validate}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "pull".equals( goal ) )
{
append( sb, "zanata:pull", 0 );
append( sb, "Pulls translated text from Zanata.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "continueAfterError (Default: false)", 2 );
append( sb, "When there is an error, whether try to workaround it and continue to next text flow or fail the process. i.e. when encounter a mismatch plural form, it will try to use singular form. Note: This option may not work on all circumstances.", 3 );
append( sb, "Expression: ${zanata.continueAfterError}", 3 );
append( sb, "", 0 );
append( sb, "createSkeletons", 2 );
append( sb, "Whether to create skeleton entries for strings/files which have not been translated yet", 3 );
append( sb, "Expression: ${zanata.createSkeletons}", 3 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "dryRun", 2 );
append( sb, "Dry run: don\'t change any data, on the server or on the filesystem.", 3 );
append( sb, "Expression: ${dryRun}", 3 );
append( sb, "", 0 );
append( sb, "enableModules", 2 );
append( sb, "Whether module processing should be enabled. This option is obsolete. Please use pull-module instead.", 3 );
append( sb, "Expression: ${zanata.enableModules}", 3 );
append( sb, "", 0 );
append( sb, "encodeTabs (Default: true)", 2 );
append( sb, "Whether tabs should be encoded as \\t (true) or left as tabs (false).", 3 );
append( sb, "Expression: ${zanata.encodeTabs}", 3 );
append( sb, "", 0 );
append( sb, "fromDoc", 2 );
append( sb, "Specifies a document from which to begin the push operation. Documents before this document (sorted alphabetically) will not be pushed. For multi-module projects, the full document path including module id is required. Use this option to resume a failed push operation.", 3 );
append( sb, "Expression: ${zanata.fromDoc}", 3 );
append( sb, "", 0 );
append( sb, "includeFuzzy (Default: false)", 2 );
append( sb, "Whether to include fuzzy translations in translation files when using project type \'file\'. If this option is false, source text will be used for any string that does not have an approved translation.", 3 );
append( sb, "Expression: ${zanata.includeFuzzy}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "locales", 2 );
append( sb, "Locales to push to/pull from the server. By default all locales in zanata.xml will be pushed/pulled. Usage: -Dzanata.locales=locale1,locale2,locale3", 3 );
append( sb, "Expression: ${zanata.locales}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "pullSrc", 2 );
append( sb, "Export source-language text from Zanata to local files, overwriting or erasing existing files (DANGER!). This option is deprecated, replaced by pullType.", 3 );
append( sb, "Expression: ${zanata.pullSrc}", 3 );
append( sb, "", 0 );
append( sb, "pullType (Default: trans)", 2 );
append( sb, "Type of pull to perform from the server: \'source\' pulls source documents only. \'trans\' pulls translation documents only. \'both\' pulls both source and translation documents.", 3 );
append( sb, "Expression: ${zanata.pullType}", 3 );
append( sb, "", 0 );
append( sb, "purgeCache (Default: false)", 2 );
append( sb, "Whether to purge the cache before performing the pull operation. This means that all documents will be fetched from the server anew.", 3 );
append( sb, "Expression: ${zanata.purgeCache}", 3 );
append( sb, "", 0 );
append( sb, "skip", 2 );
append( sb, "(no description available)", 3 );
append( sb, "Expression: ${zanata.skip}", 3 );
append( sb, "", 0 );
append( sb, "srcDir (Default: .)", 2 );
append( sb, "Base directory for source-language files", 3 );
append( sb, "Expression: ${zanata.srcDir}", 3 );
append( sb, "", 0 );
append( sb, "transDir (Default: .)", 2 );
append( sb, "Base directory for target-language files (translations)", 3 );
append( sb, "Expression: ${zanata.transDir}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "useCache (Default: true)", 2 );
append( sb, "Whether to use an Entity cache when fetching documents. When using the cache, documents that have been retrieved previously and have not changed since then will not be retrieved again.", 3 );
append( sb, "Expression: ${zanata.useCache}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "pull-module".equals( goal ) )
{
append( sb, "zanata:pull-module", 0 );
append( sb, "Pulls translated text from Zanata.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "continueAfterError (Default: false)", 2 );
append( sb, "When there is an error, whether try to workaround it and continue to next text flow or fail the process. i.e. when encounter a mismatch plural form, it will try to use singular form. Note: This option may not work on all circumstances.", 3 );
append( sb, "Expression: ${zanata.continueAfterError}", 3 );
append( sb, "", 0 );
append( sb, "createSkeletons", 2 );
append( sb, "Whether to create skeleton entries for strings/files which have not been translated yet", 3 );
append( sb, "Expression: ${zanata.createSkeletons}", 3 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "dryRun", 2 );
append( sb, "Dry run: don\'t change any data, on the server or on the filesystem.", 3 );
append( sb, "Expression: ${dryRun}", 3 );
append( sb, "", 0 );
append( sb, "encodeTabs (Default: true)", 2 );
append( sb, "Whether tabs should be encoded as \\t (true) or left as tabs (false).", 3 );
append( sb, "Expression: ${zanata.encodeTabs}", 3 );
append( sb, "", 0 );
append( sb, "fromDoc", 2 );
append( sb, "Specifies a document from which to begin the push operation. Documents before this document (sorted alphabetically) will not be pushed. For multi-module projects, the full document path including module id is required. Use this option to resume a failed push operation.", 3 );
append( sb, "Expression: ${zanata.fromDoc}", 3 );
append( sb, "", 0 );
append( sb, "includeFuzzy (Default: false)", 2 );
append( sb, "Whether to include fuzzy translations in translation files when using project type \'file\'. If this option is false, source text will be used for any string that does not have an approved translation.", 3 );
append( sb, "Expression: ${zanata.includeFuzzy}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "locales", 2 );
append( sb, "Locales to push to/pull from the server. By default all locales in zanata.xml will be pushed/pulled. Usage: -Dzanata.locales=locale1,locale2,locale3", 3 );
append( sb, "Expression: ${zanata.locales}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "pullSrc", 2 );
append( sb, "Export source-language text from Zanata to local files, overwriting or erasing existing files (DANGER!). This option is deprecated, replaced by pullType.", 3 );
append( sb, "Expression: ${zanata.pullSrc}", 3 );
append( sb, "", 0 );
append( sb, "pullType (Default: trans)", 2 );
append( sb, "Type of pull to perform from the server: \'source\' pulls source documents only. \'trans\' pulls translation documents only. \'both\' pulls both source and translation documents.", 3 );
append( sb, "Expression: ${zanata.pullType}", 3 );
append( sb, "", 0 );
append( sb, "purgeCache (Default: false)", 2 );
append( sb, "Whether to purge the cache before performing the pull operation. This means that all documents will be fetched from the server anew.", 3 );
append( sb, "Expression: ${zanata.purgeCache}", 3 );
append( sb, "", 0 );
append( sb, "skip", 2 );
append( sb, "(no description available)", 3 );
append( sb, "Expression: ${zanata.skip}", 3 );
append( sb, "", 0 );
append( sb, "srcDir (Default: .)", 2 );
append( sb, "Base directory for source-language files", 3 );
append( sb, "Expression: ${zanata.srcDir}", 3 );
append( sb, "", 0 );
append( sb, "transDir (Default: .)", 2 );
append( sb, "Base directory for target-language files (translations)", 3 );
append( sb, "Expression: ${zanata.transDir}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "useCache (Default: true)", 2 );
append( sb, "Whether to use an Entity cache when fetching documents. When using the cache, documents that have been retrieved previously and have not changed since then will not be retrieved again.", 3 );
append( sb, "Expression: ${zanata.useCache}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "push".equals( goal ) )
{
append( sb, "zanata:push", 0 );
append( sb, "Pushes source text to a Zanata project version so that it can be translated, and optionally push translated text as well. NB: Any documents which exist on the server but not locally will be deleted as obsolete.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "caseSensitive (Default: true)", 2 );
append( sb, "Case sensitive for includes and excludes options.", 3 );
append( sb, "Expression: ${zanata.caseSensitive}", 3 );
append( sb, "", 0 );
append( sb, "copyTrans (Default: true)", 2 );
append( sb, "Whether the server should copy latest translations from equivalent messages/documents in the database", 3 );
append( sb, "Expression: ${zanata.copyTrans}", 3 );
append( sb, "", 0 );
append( sb, "defaultExcludes (Default: true)", 2 );
append( sb, "Add default excludes (.svn, .git, etc) to the exclude filters.", 3 );
append( sb, "Expression: ${zanata.defaultExcludes}", 3 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "dryRun", 2 );
append( sb, "Dry run: don\'t change any data, on the server or on the filesystem.", 3 );
append( sb, "Expression: ${dryRun}", 3 );
append( sb, "", 0 );
append( sb, "enableModules", 2 );
append( sb, "Whether module processing should be enabled. This option is obsolete. Please use push-module instead.", 3 );
append( sb, "Expression: ${zanata.enableModules}", 3 );
append( sb, "", 0 );
append( sb, "excludeLocaleFilenames (Default: true)", 2 );
append( sb, "Exclude filenames which match locales in zanata.xml (other than the source locale). For instance, if zanata.xml includes de and fr, then the files messages_de.properties and messages_fr.properties will not be treated as source files.\nNB: This parameter will be ignored for some project types which use different file naming conventions (eg podir, gettext).\n", 3 );
append( sb, "Expression: ${zanata.excludeLocaleFilenames}", 3 );
append( sb, "", 0 );
append( sb, "excludes", 2 );
append( sb, "Wildcard pattern to exclude files and directories. Usage -Dzanata.excludes=\'Pattern1,Pattern2,Pattern3\'", 3 );
append( sb, "Expression: ${zanata.excludes}", 3 );
append( sb, "", 0 );
append( sb, "fileTypes (Default: txt,dtd,odt,fodt,odp,fodp,ods,fods,odg,fodg,odf,odb)", 2 );
append( sb, "File types to locate and transmit to the server when using project type \'file\'.", 3 );
append( sb, "Expression: ${zanata.fileTypes}", 3 );
append( sb, "", 0 );
append( sb, "fromDoc", 2 );
append( sb, "Specifies a document from which to begin the push operation. Documents before this document (sorted alphabetically) will not be pushed. For multi-module projects, the full document path including module id is required. Use this option to resume a failed push operation.", 3 );
append( sb, "Expression: ${zanata.fromDoc}", 3 );
append( sb, "", 0 );
append( sb, "includes", 2 );
append( sb, "Wildcard pattern to include files and directories. This parameter is only needed for some project types, eg XLIFF, Properties. Usage -Dzanata.includes=\'src/myfile*.xml,**/*.xliff.xml\'", 3 );
append( sb, "Expression: ${zanata.includes}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "locales", 2 );
append( sb, "Locales to push to/pull from the server. By default all locales in zanata.xml will be pushed/pulled. Usage: -Dzanata.locales=locale1,locale2,locale3", 3 );
append( sb, "Expression: ${zanata.locales}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "maxChunkSize (Default: 1048576)", 2 );
append( sb, "Maximum size, in bytes, of document chunks to transmit when using project type \'file\'. Documents smaller than this size will be transmitted in a single request, larger documents will be sent over multiple requests. Usage -Dzanata.maxChunkSize=12345", 3 );
append( sb, "Expression: ${zanata.maxChunkSize}", 3 );
append( sb, "", 0 );
append( sb, "merge (Default: auto)", 2 );
append( sb, "Merge type: \'auto\' (default) or \'import\' (DANGER!).", 3 );
append( sb, "Expression: ${zanata.merge}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "pushTrans", 2 );
append( sb, "Push translations from local files to the server (merge or import: see mergeType). This option is deprecated, replaced by pushType.", 3 );
append( sb, "Expression: ${zanata.pushTrans}", 3 );
append( sb, "", 0 );
append( sb, "pushType (Default: source)", 2 );
append( sb, "Type of push to perform on the server: \'source\' pushes source documents only. \'trans\' pushes translation documents only. \'both\' pushes both source and translation documents.", 3 );
append( sb, "Expression: ${zanata.pushType}", 3 );
append( sb, "", 0 );
append( sb, "skip", 2 );
append( sb, "(no description available)", 3 );
append( sb, "Expression: ${zanata.skip}", 3 );
append( sb, "", 0 );
append( sb, "sourceLang (Default: en-US)", 2 );
append( sb, "Language of source documents", 3 );
append( sb, "Expression: ${zanata.sourceLang}", 3 );
append( sb, "", 0 );
append( sb, "srcDir (Default: .)", 2 );
append( sb, "Base directory for source-language files", 3 );
append( sb, "Expression: ${zanata.srcDir}", 3 );
append( sb, "", 0 );
append( sb, "transDir (Default: .)", 2 );
append( sb, "Base directory for target-language files (translations)", 3 );
append( sb, "Expression: ${zanata.transDir}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
append( sb, "useSrcOrder (Default: false)", 2 );
append( sb, "Obsolete option, only for backwards compatibility", 3 );
append( sb, "Expression: ${zanata.useSrcOrder}", 3 );
append( sb, "", 0 );
append( sb, "validate (Default: content)", 2 );
append( sb, "Run validation check against file. Only applies to XLIFF project type. \'CONTENT\' - content validation check (quick). \'XSD\' - validation check against xliff 1.1 schema - http://www.oasis-open.org/committees/xliff/documents/xliff-core-1.1.xsd.", 3 );
append( sb, "Expression: ${zanata.validate}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "push-module".equals( goal ) )
{
append( sb, "zanata:push-module", 0 );
append( sb, "Pushes source text to a Zanata project version so that it can be translated, and optionally push translated text as well. NB: Any documents which exist on the server but not locally will be deleted as obsolete. If deleteObsoleteModules is true, documents belonging to unknown/obsolete modules will be deleted as well.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "caseSensitive (Default: true)", 2 );
append( sb, "Case sensitive for includes and excludes options.", 3 );
append( sb, "Expression: ${zanata.caseSensitive}", 3 );
append( sb, "", 0 );
append( sb, "copyTrans (Default: true)", 2 );
append( sb, "Whether the server should copy latest translations from equivalent messages/documents in the database", 3 );
append( sb, "Expression: ${zanata.copyTrans}", 3 );
append( sb, "", 0 );
append( sb, "defaultExcludes (Default: true)", 2 );
append( sb, "Add default excludes (.svn, .git, etc) to the exclude filters.", 3 );
append( sb, "Expression: ${zanata.defaultExcludes}", 3 );
append( sb, "", 0 );
append( sb, "deleteObsoleteModules (Default: false)", 2 );
append( sb, "Remove modules that are found in the server but not locally.", 3 );
append( sb, "Expression: ${zanata.deleteObsoleteModules}", 3 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "dryRun", 2 );
append( sb, "Dry run: don\'t change any data, on the server or on the filesystem.", 3 );
append( sb, "Expression: ${dryRun}", 3 );
append( sb, "", 0 );
append( sb, "excludeLocaleFilenames (Default: true)", 2 );
append( sb, "Exclude filenames which match locales in zanata.xml (other than the source locale). For instance, if zanata.xml includes de and fr, then the files messages_de.properties and messages_fr.properties will not be treated as source files.\nNB: This parameter will be ignored for some project types which use different file naming conventions (eg podir, gettext).\n", 3 );
append( sb, "Expression: ${zanata.excludeLocaleFilenames}", 3 );
append( sb, "", 0 );
append( sb, "excludes", 2 );
append( sb, "Wildcard pattern to exclude files and directories. Usage -Dzanata.excludes=\'Pattern1,Pattern2,Pattern3\'", 3 );
append( sb, "Expression: ${zanata.excludes}", 3 );
append( sb, "", 0 );
append( sb, "fileTypes (Default: txt,dtd,odt,fodt,odp,fodp,ods,fods,odg,fodg,odf,odb)", 2 );
append( sb, "File types to locate and transmit to the server when using project type \'file\'.", 3 );
append( sb, "Expression: ${zanata.fileTypes}", 3 );
append( sb, "", 0 );
append( sb, "fromDoc", 2 );
append( sb, "Specifies a document from which to begin the push operation. Documents before this document (sorted alphabetically) will not be pushed. For multi-module projects, the full document path including module id is required. Use this option to resume a failed push operation.", 3 );
append( sb, "Expression: ${zanata.fromDoc}", 3 );
append( sb, "", 0 );
append( sb, "includes", 2 );
append( sb, "Wildcard pattern to include files and directories. This parameter is only needed for some project types, eg XLIFF, Properties. Usage -Dzanata.includes=\'src/myfile*.xml,**/*.xliff.xml\'", 3 );
append( sb, "Expression: ${zanata.includes}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "locales", 2 );
append( sb, "Locales to push to/pull from the server. By default all locales in zanata.xml will be pushed/pulled. Usage: -Dzanata.locales=locale1,locale2,locale3", 3 );
append( sb, "Expression: ${zanata.locales}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "maxChunkSize (Default: 1048576)", 2 );
append( sb, "Maximum size, in bytes, of document chunks to transmit when using project type \'file\'. Documents smaller than this size will be transmitted in a single request, larger documents will be sent over multiple requests. Usage -Dzanata.maxChunkSize=12345", 3 );
append( sb, "Expression: ${zanata.maxChunkSize}", 3 );
append( sb, "", 0 );
append( sb, "merge (Default: auto)", 2 );
append( sb, "Merge type: \'auto\' (default) or \'import\' (DANGER!).", 3 );
append( sb, "Expression: ${zanata.merge}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "pushTrans", 2 );
append( sb, "Push translations from local files to the server (merge or import: see mergeType). This option is deprecated, replaced by pushType.", 3 );
append( sb, "Expression: ${zanata.pushTrans}", 3 );
append( sb, "", 0 );
append( sb, "pushType (Default: source)", 2 );
append( sb, "Type of push to perform on the server: \'source\' pushes source documents only. \'trans\' pushes translation documents only. \'both\' pushes both source and translation documents.", 3 );
append( sb, "Expression: ${zanata.pushType}", 3 );
append( sb, "", 0 );
append( sb, "skip", 2 );
append( sb, "(no description available)", 3 );
append( sb, "Expression: ${zanata.skip}", 3 );
append( sb, "", 0 );
append( sb, "sourceLang (Default: en-US)", 2 );
append( sb, "Language of source documents", 3 );
append( sb, "Expression: ${zanata.sourceLang}", 3 );
append( sb, "", 0 );
append( sb, "srcDir (Default: .)", 2 );
append( sb, "Base directory for source-language files", 3 );
append( sb, "Expression: ${zanata.srcDir}", 3 );
append( sb, "", 0 );
append( sb, "transDir (Default: .)", 2 );
append( sb, "Base directory for target-language files (translations)", 3 );
append( sb, "Expression: ${zanata.transDir}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
append( sb, "validate (Default: content)", 2 );
append( sb, "Run validation check against file. Only applies to XLIFF project type. \'CONTENT\' - content validation check (quick). \'XSD\' - validation check against xliff 1.1 schema - http://www.oasis-open.org/committees/xliff/documents/xliff-core-1.1.xsd.", 3 );
append( sb, "Expression: ${zanata.validate}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "put-project".equals( goal ) )
{
append( sb, "zanata:put-project", 0 );
append( sb, "Creates or updates a Zanata project.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "defaultProjectType", 2 );
append( sb, "Default Project type. Versions under this project that do not specify a project type will use this default. Valid values are {utf8properties, properties, gettext, podir, xliff, xml, file}. See https://github.com/zanata/zanata/wiki/Project-Types", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.defaultProjectType}", 3 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "projectDesc", 2 );
append( sb, "Project description", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.projectDesc}", 3 );
append( sb, "", 0 );
append( sb, "projectName", 2 );
append( sb, "Project name", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.projectName}", 3 );
append( sb, "", 0 );
append( sb, "projectSlug", 2 );
append( sb, "Project slug/ID", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.projectSlug}", 3 );
append( sb, "", 0 );
append( sb, "sourceCheckoutUrl", 2 );
append( sb, "URL for original source in a machine-readable format, e.g. [email protected]:zanata/zanata.git", 3 );
append( sb, "Expression: ${zanata.sourceCheckoutUrl}", 3 );
append( sb, "", 0 );
append( sb, "sourceViewUrl", 2 );
append( sb, "URL for original source in a human-readable format, e.g. https://github.com/zanata/zanata", 3 );
append( sb, "Expression: ${zanata.sourceViewUrl}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "put-user".equals( goal ) )
{
append( sb, "zanata:put-user", 0 );
append( sb, "Creates or updates a Zanata user.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "userDisabled", 2 );
append( sb, "Whether the account should be disabled", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.userDisabled}", 3 );
append( sb, "", 0 );
append( sb, "userEmail", 2 );
append( sb, "Email address of the user", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.userEmail}", 3 );
append( sb, "", 0 );
append( sb, "userKey", 2 );
append( sb, "User\'s api key (empty for none)", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.userKey}", 3 );
append( sb, "", 0 );
append( sb, "userLangs", 2 );
append( sb, "Language teams for the user", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.userLangs}", 3 );
append( sb, "", 0 );
append( sb, "userName", 2 );
append( sb, "Full name of the user", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.userName}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
append( sb, "userPasswordHash", 2 );
append( sb, "User password hash", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.userPasswordHash}", 3 );
append( sb, "", 0 );
append( sb, "userRoles", 2 );
append( sb, "Security roles for the user", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.userRoles}", 3 );
append( sb, "", 0 );
append( sb, "userUsername", 2 );
append( sb, "Login/username of the user", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.userUsername}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "put-version".equals( goal ) )
{
append( sb, "zanata:put-version", 0 );
append( sb, "Creates or updates a Zanata project version.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Project type {utf8properties, properties, gettext, podir, xliff, xml, file} Leave blank to inherit default project type from parent project", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
append( sb, "versionProject", 2 );
append( sb, "ID of Zanata project", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.versionProject}", 3 );
append( sb, "", 0 );
append( sb, "versionSlug", 2 );
append( sb, "Project version ID", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${zanata.versionSlug}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "stats".equals( goal ) )
{
append( sb, "zanata:stats", 0 );
append( sb, "Get translation statistics from Zanata.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "disableSSLCert (Default: false)", 2 );
append( sb, "Disable SSL certificate verification when connecting to Zanata host by https.", 3 );
append( sb, "Expression: ${zanata.disableSSLCert}", 3 );
append( sb, "", 0 );
append( sb, "documentId", 2 );
append( sb, "Document id to fetch statistics for.", 3 );
append( sb, "Expression: ${zanata.docId}", 3 );
append( sb, "", 0 );
append( sb, "format (Default: console)", 2 );
append( sb, "Output format for the statistics. Valid options are: csv - For a csv format (via the console). console (default) - For regular console printing.", 3 );
append( sb, "Expression: ${zanata.format}", 3 );
append( sb, "", 0 );
append( sb, "includeDetails (Default: false)", 2 );
append( sb, "Include detailed statistics.", 3 );
append( sb, "Expression: ${zanata.details}", 3 );
append( sb, "", 0 );
append( sb, "includeWordLevelStats (Default: false)", 2 );
append( sb, "Include word-level stats. By default, only message-level statistics will be fetched.", 3 );
append( sb, "Expression: ${zanata.word}", 3 );
append( sb, "", 0 );
append( sb, "interactiveMode (Default: ${settings.interactiveMode})", 2 );
append( sb, "Interactive mode is enabled by default, but can be disabled using Maven\'s -B/--batch-mode option.", 3 );
append( sb, "", 0 );
append( sb, "key", 2 );
append( sb, "API key for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.key}", 3 );
append( sb, "", 0 );
append( sb, "logHttp (Default: false)", 2 );
append( sb, "Enable HTTP message logging.", 3 );
append( sb, "Expression: ${zanata.logHttp}", 3 );
append( sb, "", 0 );
append( sb, "project", 2 );
append( sb, "Project slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.project}", 3 );
append( sb, "", 0 );
append( sb, "projectConfig (Default: ${basedir}/zanata.xml)", 2 );
append( sb, "Zanata project configuration file.", 3 );
append( sb, "Expression: ${zanata.projectConfig}", 3 );
append( sb, "", 0 );
append( sb, "projectType", 2 );
append( sb, "Type of project ( \'properties\' = Java .properties, \'podir\' = publican-style gettext directories, \'utf8properties\' = UTF-8 .properties files, \'gettext\' = gettext PO files, \'file\' = EXPERIMENTAL document files of various types). If \'file\' is used, transDir must not be the same as or nested within srcDir, and vice versa.", 3 );
append( sb, "Expression: ${zanata.projectType}", 3 );
append( sb, "", 0 );
append( sb, "projectVersion", 2 );
append( sb, "Project version slug (id) within Zanata server.", 3 );
append( sb, "Expression: ${zanata.projectVersion}", 3 );
append( sb, "", 0 );
append( sb, "url", 2 );
append( sb, "Base URL for the server. Defaults to the value in zanata.xml (if present).", 3 );
append( sb, "Expression: ${zanata.url}", 3 );
append( sb, "", 0 );
append( sb, "userConfig (Default: ${user.home}/.config/zanata.ini)", 2 );
append( sb, "Client configuration file for Zanata.", 3 );
append( sb, "Expression: ${zanata.userConfig}", 3 );
append( sb, "", 0 );
append( sb, "username", 2 );
append( sb, "Username for accessing the REST API. Defaults to the value in zanata.ini.", 3 );
append( sb, "Expression: ${zanata.username}", 3 );
append( sb, "", 0 );
}
}
if ( getLog().isInfoEnabled() )
{
getLog().info( sb.toString() );
}
}
/**
* Repeat a String n
times to form a new string.
*
* @param str String to repeat
* @param repeat number of times to repeat str
* @return String with repeated String
* @throws NegativeArraySizeException if repeat < 0
* @throws NullPointerException if str is null
*/
private static String repeat( String str, int repeat )
{
StringBuffer buffer = new StringBuffer( repeat * str.length() );
for ( int i = 0; i < repeat; i++ )
{
buffer.append( str );
}
return buffer.toString();
}
/**
* Append a description to the buffer by respecting the indentSize and lineLength parameters.
* Note: The last character is always a new line.
*
* @param sb The buffer to append the description, not null
.
* @param description The description, not null
.
* @param indent The base indentation level of each line, must not be negative.
*/
private void append( StringBuffer sb, String description, int indent )
{
for ( Iterator it = toLines( description, indent, indentSize, lineLength ).iterator(); it.hasNext(); )
{
sb.append( it.next().toString() ).append( '\n' );
}
}
/**
* Splits the specified text into lines of convenient display length.
*
* @param text The text to split into lines, must not be null
.
* @param indent The base indentation level of each line, must not be negative.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
* @return The sequence of display lines, never null
.
* @throws NegativeArraySizeException if indent < 0
*/
private static List toLines( String text, int indent, int indentSize, int lineLength )
{
List lines = new ArrayList();
String ind = repeat( "\t", indent );
String[] plainLines = text.split( "(\r\n)|(\r)|(\n)" );
for ( int i = 0; i < plainLines.length; i++ )
{
toLines( lines, ind + plainLines[i], indentSize, lineLength );
}
return lines;
}
/**
* Adds the specified line to the output sequence, performing line wrapping if necessary.
*
* @param lines The sequence of display lines, must not be null
.
* @param line The line to add, must not be null
.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
*/
private static void toLines( List lines, String line, int indentSize, int lineLength )
{
int lineIndent = getIndentLevel( line );
StringBuffer buf = new StringBuffer( 256 );
String[] tokens = line.split( " +" );
for ( int i = 0; i < tokens.length; i++ )
{
String token = tokens[i];
if ( i > 0 )
{
if ( buf.length() + token.length() >= lineLength )
{
lines.add( buf.toString() );
buf.setLength( 0 );
buf.append( repeat( " ", lineIndent * indentSize ) );
}
else
{
buf.append( ' ' );
}
}
for ( int j = 0; j < token.length(); j++ )
{
char c = token.charAt( j );
if ( c == '\t' )
{
buf.append( repeat( " ", indentSize - buf.length() % indentSize ) );
}
else if ( c == '\u00A0' )
{
buf.append( ' ' );
}
else
{
buf.append( c );
}
}
}
lines.add( buf.toString() );
}
/**
* Gets the indentation level of the specified line.
*
* @param line The line whose indentation level should be retrieved, must not be null
.
* @return The indentation level of the line.
*/
private static int getIndentLevel( String line )
{
int level = 0;
for ( int i = 0; i < line.length() && line.charAt( i ) == '\t'; i++ )
{
level++;
}
for ( int i = level + 1; i <= level + 4 && i < line.length(); i++ )
{
if ( line.charAt( i ) == '\t' )
{
level++;
break;
}
}
return level;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy