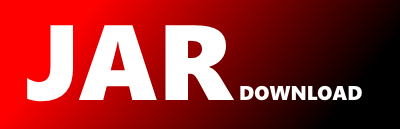
org.zbus.client.ha.CommonsClientPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zbus-client Show documentation
Show all versions of zbus-client Show documentation
lightweight message queue, service bus
The newest version!
package org.zbus.client.ha;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
import org.apache.commons.pool2.BasePooledObjectFactory;
import org.apache.commons.pool2.ObjectPool;
import org.apache.commons.pool2.PooledObject;
import org.apache.commons.pool2.impl.DefaultPooledObject;
import org.apache.commons.pool2.impl.GenericObjectPool;
import org.apache.commons.pool2.impl.GenericObjectPoolConfig;
import org.zbus.client.ClientPool;
import org.zbus.remoting.ClientDispachterManager;
import org.zbus.remoting.RemotingClient;
public class CommonsClientPool implements ClientPool{
private final ObjectPool pool;
private final GenericObjectPoolConfig config;
private final String broker;
public CommonsClientPool(GenericObjectPoolConfig config, String broker, ClientDispachterManager clientMgr){
this.config = config;
this.broker = broker;
RemotingClientFactory factory = new RemotingClientFactory(clientMgr, this.broker);
this.pool = new GenericObjectPool(factory, this.config);
}
public CommonsClientPool(GenericObjectPoolConfig config, String broker) throws IOException {
this(config, broker, defaultClientDispachterManager());
}
public CommonsClientPool(PoolConfig config, String broker) throws IOException {
this(config, broker, null);
}
public CommonsClientPool(PoolConfig config, String broker, ClientDispachterManager clientMgr){
this(toObjectPoolConfig(config), broker, clientMgr);
}
private static ClientDispachterManager defaultClientDispachterManager() throws IOException{
ClientDispachterManager clientMgr = new ClientDispachterManager();
clientMgr.start();
return clientMgr;
}
private static GenericObjectPoolConfig toObjectPoolConfig(PoolConfig config){
//TODO
return new GenericObjectPoolConfig();
}
public RemotingClient borrowClient(String mq) throws Exception {
return pool.borrowObject();
}
public List borrowEachClient(String mq) throws Exception {
return Arrays.asList(borrowClient(mq));
}
public void invalidateClient(RemotingClient client) throws Exception{
pool.invalidateObject(client);
}
public void returnClient(RemotingClient client) throws Exception {
pool.returnObject(client);
}
public void returnClient(List clients) throws Exception {
for(RemotingClient client : clients){
returnClient(client);
}
}
public void destroy() {
pool.close();
}
}
class RemotingClientFactory extends BasePooledObjectFactory {
private final ClientDispachterManager cliengMgr;
private final String broker;
public RemotingClientFactory(final ClientDispachterManager clientMgr, final String broker){
this.cliengMgr = clientMgr;
this.broker = broker;
}
@Override
public RemotingClient create() throws Exception {
return new RemotingClient(broker, cliengMgr);
}
@Override
public PooledObject wrap(RemotingClient obj) {
return new DefaultPooledObject(obj);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy