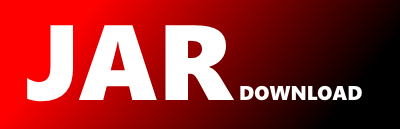
org.zbus.client.rpc.json.JsonServiceHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zbus-client Show documentation
Show all versions of zbus-client Show documentation
lightweight message queue, service bus
The newest version!
/************************************************************************
* Copyright (c) 2011-2012 HONG LEIMING.
*
* Permission is hereby granted, free of charge, to any person obtaining a
* copy of this software and associated documentation files (the
* "Software"), to deal in the Software without restriction, including
* without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to permit
* persons to whom the Software is furnished to do so, subject to the
* following conditions:
*
* The above copyright notice and this permission notice shall be included
* in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
* OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN
* NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
* DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
* OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
* USE OR OTHER DEALINGS IN THE SOFTWARE.
***************************************************************************/
package org.zbus.client.rpc.json;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
import org.zbus.client.rpc.Remote;
import org.zbus.client.rpc.ServiceHandler;
import org.zbus.logging.Logger;
import org.zbus.logging.LoggerFactory;
import org.zbus.remoting.Message;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.alibaba.fastjson.serializer.SerializerFeature;
class MethodInstance{
public Method method;
public Object instance;
public MethodInstance(Method method, Object instance){
this.method = method;
this.instance = instance;
}
@Override
public String toString() {
return "MethodInstance [method=" + method + ", instance=" + instance + "]";
}
}
public class JsonServiceHandler implements ServiceHandler {
private static final Logger log = LoggerFactory.getLogger(JsonServiceHandler.class);
private static final String DEFAULT_ENCODING = "UTF-8";
private Map methods = new HashMap();
public void addModule(Class> clazz, Object... services){
addModule(clazz.getSimpleName(), services);
}
public void addModule(String module, Object... services){
for(Object service: services){
this.initCommandTable(module, service);
}
}
private void initCommandTable(String module, Object service){
Class>[] classes = new Class>[service.getClass().getInterfaces().length+1];
classes[0] = service.getClass();
for(int i=1; i clazz : classes){
Method [] methods = clazz.getMethods();
for (Method m : methods) {
Remote cmd = m.getAnnotation(Remote.class);
if(cmd != null){
String paramMD5 = "";
Class>[] paramTypes = m.getParameterTypes();
StringBuilder sb = new StringBuilder();
for(int i=0;i returnType=null;
if(error == null){
try {
Class>[] paramTypeList = target.method.getParameterTypes();
returnType = target.method.getReturnType();
//添加返回值类型,判断是否为数组
if(returnType != null && returnType.isArray()){
returnType = returnType.getComponentType();
}
if(args == null){ //FIX of none parameters
args = new JSONArray();
}
if(paramTypeList.length == args.size()){
Object[] params = new Object[paramTypeList.length];
for(int i=0; i paramType = paramTypeList[i];
requiredParamTypeString += paramType.getName();
if(i returnType, String encoding){
Message res = new Message();
JSONObject data = new JSONObject();
res.setStatus("200");
data.put("result", result);
if(returnType != null){
data.put("returnType", returnType);
}
byte[] resBytes = null;
resBytes = JsonHelper.toJSONBytes(data, encoding, SerializerFeature.WriteMapNullValue, SerializerFeature.WriteClassName);
res.setJsonBody(resBytes);
return res;
}
private Message errorMessage(Throwable error, String encoding, String status){
Message res = new Message();
res.setStatus(status);
JSONObject data = new JSONObject();
StringWriter sw = new StringWriter();
PrintWriter pw = new PrintWriter(sw);
if(error.getCause() != null){
error = error.getCause();
}
error.printStackTrace(pw);
data.put("error", error);
data.put("stack_trace", sw.toString());
byte[] resBytes = null;
resBytes = JsonHelper.toJSONBytes(data, encoding, SerializerFeature.WriteMapNullValue, SerializerFeature.WriteClassName);
res.setJsonBody(resBytes);
return res;
}
@Override
public Message handleRequest(Message request) {
String encoding = request.getEncoding();
if(encoding == null){
encoding = DEFAULT_ENCODING;
}
try {
byte[] jsonBytes = request.getBody();
return this.handleJsonRequest(jsonBytes, encoding);
} catch (Throwable error) {
return errorMessage(error, encoding, "500");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy