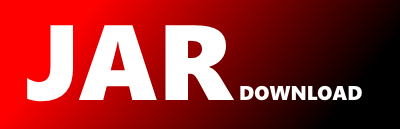
org.zbus.server.mq.PullSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zbus-client Show documentation
Show all versions of zbus-client Show documentation
lightweight message queue, service bus
The newest version!
package org.zbus.server.mq;
import java.util.HashSet;
import java.util.Set;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.locks.ReentrantLock;
import org.zbus.remoting.Message;
import org.zbus.remoting.znet.Session;
public class PullSession {
public static final int HIGHT_WATER_MARK = 100;
Session session;
Message pullMsg;
final ReentrantLock pullMsgLock = new ReentrantLock();
final Set topicSet = new HashSet();
final BlockingQueue msgQ = new LinkedBlockingQueue();
public PullSession(Session sess, Message msg) {
this.session = sess;
this.setPullMsg(msg);
}
public void subscribeTopics(String topicString){
if(topicString == null) return;
String[] ts = topicString.split("[,]");
for(String t : ts){
if(t.trim().isEmpty()) continue;
topicSet.add(t.trim());
}
}
public boolean isTopicMatched(String topic){
if(topic == null) return false;
if(topicSet.contains("*")) return true;
return topicSet.contains(topic);
}
public Session getSession() {
return session;
}
public void setSession(Session session) {
this.session = session;
}
public Message getPullMsg() {
return this.pullMsg;
}
public void setPullMsg(Message msg) {
this.pullMsg = msg;
if(msg == null) return;
String topic = this.pullMsg.getTopic();
if(topic != null){
this.subscribeTopics(topic);
}
}
public Set getTopics(){
return this.topicSet;
}
public BlockingQueue getMsgQ() {
return msgQ;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy