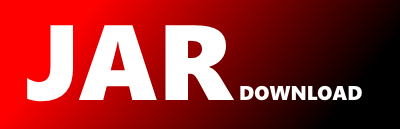
org.zbus.remoting.MessageCodec Maven / Gradle / Ivy
package org.zbus.remoting;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import org.zbus.remoting.nio.Codec;
import org.zbus.remoting.nio.IoBuffer;
public class MessageCodec implements Codec{
public IoBuffer encode(Object obj) {
if(!(obj instanceof Message)){
throw new RuntimeException("Message unknown");
}
Message msg = (Message)obj;
IoBuffer buf = IoBuffer.allocate(msg.estimatedSize()+256);
buf.put(msg.getMetaString()+"\r\n");
Map headers = msg.getHead();
int contentLength = 0;
if(msg.getBody() != null){
contentLength = msg.getBody().length;
}
String lenKey = Message.HEADER_CONTENT_LENGTH;
Iterator> iter = headers.entrySet().iterator();
while(iter.hasNext()){
Entry e = iter.next();
buf.put(e.getKey() + ": " + e.getValue() + "\r\n");
}
if(!headers.containsKey(lenKey)){
buf.put(lenKey + ": " + contentLength + "\r\n");
}
buf.put("\r\n");
if(msg.getBody() != null){
buf.put(msg.getBody());
}
buf.flip();
return buf;
}
public Object decode(IoBuffer buf) {
int headerIdx = findHeaderEnd(buf);
if(headerIdx == -1) return null;
int headerLen = headerIdx+1-buf.position();
buf.mark();
Message msg = new Message();
msg.decodeHeaders(buf.array(), buf.position(), headerLen);
buf.position(buf.position()+headerLen);
String contentLength = msg.getHeadOrParam(Message.HEADER_CONTENT_LENGTH);
if(contentLength == null){ //just head
return msg;
}
int bodyLen = Integer.valueOf(contentLength);
if(buf.remaining()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy