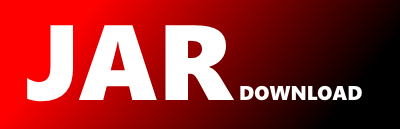
zmq.io.Metadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jeromq Show documentation
Show all versions of jeromq Show documentation
Pure Java implementation of libzmq
package zmq.io;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.ByteBuffer;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.Set;
import zmq.Msg;
import zmq.ZError;
import zmq.ZMQ;
import zmq.util.Wire;
public class Metadata
{
/**
* Call backs during parsing process
*/
public interface ParseListener
{
/**
* Called when a property has been parsed.
* @param name the name of the property.
* @param value the value of the property.
* @param valueAsString the value in a string representation.
* @return 0 to continue the parsing process, any other value to interrupt it.
*/
int parsed(String name, byte[] value, String valueAsString);
}
public static final String IDENTITY = "Identity";
public static final String SOCKET_TYPE = "Socket-Type";
public static final String USER_ID = "User-Id";
public static final String PEER_ADDRESS = "Peer-Address";
// Dictionary holding metadata.
private final Properties dictionary = new Properties();
public Metadata()
{
super();
}
public Metadata(Properties dictionary)
{
this.dictionary.putAll(dictionary);
}
public final Set keySet()
{
return dictionary.stringPropertyNames();
}
public final void remove(String key)
{
dictionary.remove(key);
}
// Returns property value or NULL if
// property is not found.
public final String get(String key)
{
return dictionary.getProperty(key);
}
public final void set(String key, String value)
{
dictionary.setProperty(key, value);
}
@Override
public int hashCode()
{
return dictionary.hashCode();
}
@Override
public boolean equals(Object other)
{
if (this == other) {
return true;
}
if (other == null) {
return false;
}
if (!(other instanceof Metadata)) {
return false;
}
Metadata that = (Metadata) other;
return this.dictionary.equals(that.dictionary);
}
public final void set(Metadata zapProperties)
{
dictionary.putAll(zapProperties.dictionary);
}
public final boolean isEmpty()
{
return dictionary.isEmpty();
}
@Override
public String toString()
{
return "Metadata=" + dictionary;
}
public final byte[] bytes()
{
ByteArrayOutputStream stream = new ByteArrayOutputStream(size());
try {
write(stream);
return stream.toByteArray();
}
catch (IOException e) {
return new byte[0];
}
finally {
try {
stream.close();
}
catch (IOException e) {
}
}
}
private int size()
{
int size = 0;
for (Entry
© 2015 - 2024 Weber Informatics LLC | Privacy Policy