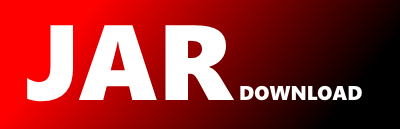
org.zeroturnaround.javarebel.integration.jboss5.BaseClassLoaderDomainCBP Maven / Gradle / Ivy
The newest version!
package org.zeroturnaround.javarebel.integration.jboss5;
import org.zeroturnaround.bundled.javassist.ClassPool;
import org.zeroturnaround.bundled.javassist.CtClass;
import org.zeroturnaround.bundled.javassist.CtMethod;
import org.zeroturnaround.bundled.javassist.NotFoundException;
import org.zeroturnaround.javarebel.integration.support.JavassistClassBytecodeProcessor;
/**
* Transforms class org.jboss.classloader.spi.base.BaseClassLoaderDomain
* to integrate JBoss 5.x class loader with JavaRebel.
*
* @author Jevgeni Kabanov
* @author Rein Raudjärv
*/
public class BaseClassLoaderDomainCBP extends JavassistClassBytecodeProcessor {
public void process(ClassPool cp, ClassLoader cl, CtClass ctClass) throws Exception {
cp.importPackage("org.jboss.classloader.spi");
cp.importPackage("org.jboss.classloader.spi.base");
cp.importPackage("org.jboss.classloader.plugins");
cp.importPackage("java.util");
cp.importPackage("java.net");
/*
* Since JBoss 5.1 globalClassCache uses ClassCacheItem entries.
* Prior that Loader entries were used.
*/
boolean useClassCacheItem;
try {
cp.get("org.jboss.classloader.spi.base.BaseClassLoaderDomain$ClassCacheItem");
useClassCacheItem = true;
} catch (NotFoundException e) {
useClassCacheItem = false;
}
CtMethod findLoaderInExportsMethod = ctClass.getDeclaredMethod("findLoaderInExports", cp.get(new String[]{"org.jboss.classloader.spi.base.BaseClassLoader", String.class.getName(), boolean.class.getName()}));
findLoaderInExportsMethod.setBody(
"{" +
(useClassCacheItem ?
" BaseClassLoaderDomain$ClassCacheItem item = (BaseClassLoaderDomain$ClassCacheItem) globalClassCache.get($2);" +
" if (item != null) {" +
" Loader loader = item.loader;" +
" if (loader != null) {" +
" return loader;" +
" }" +
" }"
:
" Loader loader = (Loader) globalClassCache.get($2);" +
" if (loader != null) {" +
" return loader;" +
" }"
) +
" if (globalClassBlackList.containsKey($2)) {" +
" return null;" +
" }" +
" boolean canCache = true;" +
" boolean canBlackList = true;" +
" for (Iterator i = classLoaders.iterator(); i.hasNext();) {" +
" ClassLoaderInformation info = (ClassLoaderInformation) i.next();" +
" BaseDelegateLoader exported = info.getExported();" +
// See whether the policies allow caching/blacklisting
" BaseClassLoaderPolicy loaderPolicy = exported.getPolicy();" +
" if (loaderPolicy == null || loaderPolicy.isCacheable() == false)" +
" canCache = false;" +
" if (loaderPolicy == null || loaderPolicy.isBlackListable() == false)" +
" canBlackList = false;" +
" if (exported.getResource($2) != null) {" +
" if (canCache) {" +
" globalClassCache.put($2, " + (useClassCacheItem ? "new BaseClassLoaderDomain$ClassCacheItem($0, (Loader) exported)" : "exported") + ");" +
" }" +
" return exported;" +
" }" +
" }" +
// Here is not found in the exports so can we blacklist it?
" if (canBlackList) {" +
" globalClassBlackList.put($2, $2);" +
" }" +
" return null;" +
"}");
CtMethod getResourceFromExportsMethod = ctClass.getDeclaredMethod(
"getResourceFromExports",
cp.get(new String[]{"org.jboss.classloader.spi.base.BaseClassLoader", String.class.getName(), boolean.class.getName()}));
getResourceFromExportsMethod.setBody(
"{" +
" URL result = globalResourceCache.get($2);" +
" if (globalResourceBlackList.containsKey($2)) {" +
" return null;" +
" }" +
" boolean canCache = true;" +
" boolean canBlackList = true;" +
" for (Iterator i = classLoaders.iterator(); i.hasNext();) {" +
" ClassLoaderInformation info = (ClassLoaderInformation) i.next();" +
" BaseDelegateLoader loader = info.getExported();" +
// See whether the policies allow caching/blacklisting
" BaseClassLoaderPolicy loaderPolicy = loader.getPolicy();" +
" if (loaderPolicy == null || loaderPolicy.isCacheable() == false)" +
" canCache = false;" +
" if (loaderPolicy == null || loaderPolicy.isBlackListable() == false)" +
" canBlackList = false;" +
" result = loader.getResource($2);" +
" if (result != null) {" +
" if (canCache) {" +
" globalResourceCache.put($2, result);" +
" }" +
" return result;" +
" }" +
" }" +
// Here is not found in the exports so can we blacklist it?
" if (canBlackList) {" +
" globalResourceBlackList.put($2, $2);" +
" }" +
" return null;" +
"}");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy