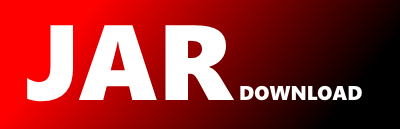
org.zeroturnaround.javarebel.integration.resin.ResinPatchHelper Maven / Gradle / Ivy
The newest version!
package org.zeroturnaround.javarebel.integration.resin;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.jar.JarOutputStream;
import java.util.zip.ZipEntry;
import org.zeroturnaround.javarebel.ClassBytecodeProcessor;
import org.zeroturnaround.javarebel.Logger;
import org.zeroturnaround.javarebel.LoggerFactory;
import org.zeroturnaround.javarebel.integration.util.ClassUtil;
import org.zeroturnaround.javarebel.integration.util.IoUtil;
/**
* JavaRebel Resin patch related methods.
*
* Used since Resin 3.1.x.
*
* @author Rein Raudjärv
*/
public class ResinPatchHelper {
/**
* Whether the Resin patch has been applied in this JVM.
*/
private static boolean enabled;
/**
* If the patch is applied this method is called by the SystemClassLoader
.
*/
public static void setEnabled() {
enabled = true;
}
/**
* Show error if Resin patch is not applied.
*/
public static void checkEnabled() {
if (!isEnabled())
error();
}
/**
* @return true
if the Resin patch is applied.
*/
private static boolean isEnabled() {
return enabled;
}
private static void error() {
System.out.println();
System.out.println("#############################################################");
System.out.println();
System.out.println(" JavaRebel Resin installation problem!");
System.out.println();
System.out.println(" See http://www.zeroturnaround.com/resin-problem/");
System.out.println(" or consult with the installation manual for correct");
System.out.println(" JavaRebel installation with Resin server.");
System.out.println();
System.out.println("#############################################################");
System.out.println();
}
/**
* Create a temporary JAR containing transformed Resin boot classes.
*
* @param cl class loader containing the original class resources.
* @return temporary JAR file.
*/
public static File createResinPatch(ClassLoader cl) throws IOException {
File jar = File.createTempFile("javarebel-resin-patch", ".jar");
JarOutputStream out = new JarOutputStream(new FileOutputStream(jar));
transformAndZip(out, cl,
"com.caucho.loader.DynamicClassLoader",
new ResinDynamicClassLoaderCBP());
transformAndZip(out, cl,
"com.caucho.loader.SystemClassLoader",
new ResinSystemClassLoaderCBP());
transformAndZip(out, cl,
"com.caucho.server.webapp.WebApp",
new ResinWebAppCBP());
out.close();
Logger log = LoggerFactory.getInstance();
if (log.isEnabled())
log.log("Resin patch created: " + jar);
return jar;
}
private static void transformAndZip(JarOutputStream out, ClassLoader cl, String classname, ClassBytecodeProcessor cbp) throws IOException {
// Get the byte code of the class
byte[] bytecode = ClassUtil.getBytecode(cl, classname);
// Transform the byte code
byte[] transformed = cbp.process(cl, classname, bytecode);
Logger log = LoggerFactory.getInstance();
if (log.isEnabled())
log.log("Class '" + classname + "' processed by '" + cbp.getClass().getName() + "' into JAR.");
// Add a ZIP entry
out.putNextEntry(new ZipEntry(ClassUtil.getResourecName(classname)));
IoUtil.write(transformed, out);
out.closeEntry();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy