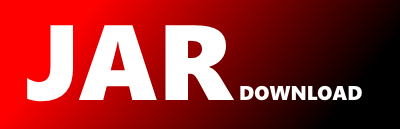
org.zeroturnaround.javarebel.integration.streambase.StreamBaseJarClassLoaderCBP Maven / Gradle / Ivy
The newest version!
package org.zeroturnaround.javarebel.integration.streambase;
import org.zeroturnaround.bundled.javassist.CannotCompileException;
import org.zeroturnaround.bundled.javassist.ClassPool;
import org.zeroturnaround.bundled.javassist.CtClass;
import org.zeroturnaround.bundled.javassist.CtConstructor;
import org.zeroturnaround.bundled.javassist.CtNewMethod;
import org.zeroturnaround.bundled.javassist.NotFoundException;
import org.zeroturnaround.javarebel.LoggerFactory;
import org.zeroturnaround.javarebel.integration.support.JavassistClassBytecodeProcessor;
/**
* Transforms com.streambase.sb.runtime.JarClassLoader
.
*
* @author Rein Raudjärv
*/
public class StreamBaseJarClassLoaderCBP extends JavassistClassBytecodeProcessor {
public void process(ClassPool cp, ClassLoader cl, CtClass ctClass) throws Exception {
cp.importPackage("org.zeroturnaround.javarebel");
cp.importPackage("org.zeroturnaround.javarebel.integration.generic");
initializeClassLoader(ctClass);
reinitializeClassLoader(cp, ctClass);
baseIntegration(cp, ctClass);
resourceStreamProviding(cp, ctClass);
}
private void initializeClassLoader(CtClass ctClass) throws CannotCompileException {
CtConstructor[] constructors = ctClass.getConstructors();
for (int i = 0; i < constructors.length; i++)
constructors[i].insertAfter(
"{" +
" IntegrationFactory.getInstance().registerClassLoader($0, new RestrictedClassResourceSource($0));" +
"}");
}
private void reinitializeClassLoader(ClassPool cp, CtClass ctClass) throws CannotCompileException {
cp.importPackage("org.zeroturnaround.javarebel");
/*
* Reinitialize ClassLoader if new JAR is added or an existing is removed
*/
try {
ctClass.getDeclaredMethod("addJar").insertAfter(
"{" +
" IntegrationFactory.getInstance().reinitializeClassLoader($0);" +
"}");
} catch (NotFoundException e) {
LoggerFactory.getInstance().error(e);
}
try {
ctClass.getDeclaredMethod("removeJar").insertAfter(
"{" +
" IntegrationFactory.getInstance().reinitializeClassLoader($0);" +
"}");
} catch (NotFoundException e) {
LoggerFactory.getInstance().error(e);
}
}
private void baseIntegration(ClassPool cp, CtClass ctClass) throws NotFoundException, CannotCompileException {
cp.importPackage("org.zeroturnaround.javarebel");
ctClass.getMethod("findClass", "(Ljava/lang/String;)Ljava/lang/Class;").insertBefore(
"{ synchronized ($0) {" +
" Class result =" +
" findLoadedClass($1);" +
" if (result != null)" +
" return result;" +
" result = " +
" IntegrationFactory.getInstance().findReloadableClass($0, $1);" +
" if (result != null)" +
" return result;" +
"}}");
try {
ctClass.getDeclaredMethod("findResource", cp.get(new String[] {String.class.getName()})).insertBefore(
"{" +
" Integration integration = IntegrationFactory.getInstance();" +
" if (integration.isResourceReplaced($0, $1))" +
" return integration.findResource($0, $1);" +
"}");
}
catch (NotFoundException e) {
// skip
}
try {
ctClass.getDeclaredMethod("findResources", cp.get(new String[] {String.class.getName()})).insertBefore(
"{" +
" Integration integration = IntegrationFactory.getInstance();" +
" if (integration.isResourceReplaced($0, $1))" +
" return integration.findResources($0, $1);" +
"}");
}
catch (NotFoundException e) {
// skip
}
}
private void resourceStreamProviding(ClassPool cp, CtClass ctClass) throws CannotCompileException {
cp.importPackage("java.io");
cp.importPackage("java.net");
cp.importPackage("java.util");
cp.importPackage("java.util.jar");
cp.importPackage("org.zeroturnaround.javarebel");
cp.importPackage("org.zeroturnaround.javarebel.integration.streambase");
cp.importPackage("com.streambase.sb.util");
boolean findResourceMissing = false;
try {
ctClass.getDeclaredMethod("findResource");
} catch (NotFoundException e) {
findResourceMissing = true;
}
if (findResourceMissing) {
try {
ctClass.removeMethod(ctClass.getDeclaredMethod("getResourceAsStream"));
} catch (NotFoundException e) {
LoggerFactory.getInstance().error(e);
}
ctClass.addMethod(CtNewMethod.make(
"protected URL findResource(String name) {" +
" Integration integration = IntegrationFactory.getInstance();" +
" if (integration.isResourceReplaced($0, $1))" +
" return integration.findResource($0, $1);" +
" Msg.debug2(\"find findResource: {0}\", new Object[] { name });" +
" Iterator i = this.jars.values().iterator();" +
" if (i.hasNext()) {" +
" JarFile jf = (JarFile)i.next();" +
" JarEntry je = jf.getJarEntry(name);" +
" if (je != null) {" +
" return JarUtil.toURL(jf, je);" +
" }" +
" }" +
" return null;" +
"}", ctClass));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy