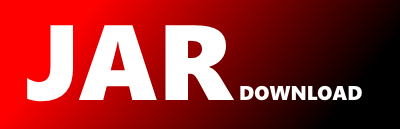
org.zeroturnaround.javarebel.integration.websphere.CompoundClassLoaderClassBytecodeProcessor Maven / Gradle / Ivy
The newest version!
package org.zeroturnaround.javarebel.integration.websphere;
import org.zeroturnaround.bundled.javassist.CannotCompileException;
import org.zeroturnaround.bundled.javassist.ClassPool;
import org.zeroturnaround.bundled.javassist.CtClass;
import org.zeroturnaround.bundled.javassist.CtConstructor;
import org.zeroturnaround.bundled.javassist.CtMethod;
import org.zeroturnaround.bundled.javassist.NotFoundException;
import org.zeroturnaround.bundled.javassist.expr.ExprEditor;
import org.zeroturnaround.bundled.javassist.expr.MethodCall;
import org.zeroturnaround.javarebel.integration.support.JavassistClassBytecodeProcessor;
public class CompoundClassLoaderClassBytecodeProcessor extends
JavassistClassBytecodeProcessor {
public void process(ClassPool cp, ClassLoader cl, CtClass ctClass) throws Exception {
CtConstructor[] constructors = ctClass.getConstructors();
for (int i = 0; i < constructors.length; i++) {
CtConstructor constructor = constructors[i];
constructor.insertAfter(
"org.zeroturnaround.javarebel.IntegrationFactory.getInstance()" +
" .registerClassLoader($0, " +
" new org.zeroturnaround.javarebel.integration.generic.FindResourceClassResourceSource($0));");
}
CtMethod m = ctClass.getMethod("findClass", "(Ljava/lang/String;Z)Ljava/lang/Class;");
m.insertBefore(
"{ synchronized ($0) {" +
" Class result =" +
" findLoadedClass($1);" +
" if (result != null)" +
" return result;" +
" result = " +
" org.zeroturnaround.javarebel.IntegrationFactory.getInstance()" +
" .findReloadableClass($0, $1);" +
" if (result != null)" +
" return result;" +
" }}");
try {
m = ctClass.getDeclaredMethod("getResource");
m.instrument(new ExprEditor() {
public void edit(MethodCall m) throws CannotCompileException {
if (m.getClassName().equals("java.util.HashSet") && m.getMethodName().equals("contains"))
m.replace("{ $_ = false; }");
}
});
} catch (NotFoundException e) {
}
try {
m = ctClass.getDeclaredMethod("getResourceAsStream");
m.instrument(new ExprEditor() {
public void edit(MethodCall m) throws CannotCompileException {
if (m.getClassName().equals("java.util.HashSet") && m.getMethodName().equals("contains"))
m.replace("{ $_ = false; }");
}
});
} catch (NotFoundException e) {
}
try {
m = ctClass.getDeclaredMethod("findResource", cp.get(new String[] {String.class.getName()}));
m.insertBefore(
"{ " +
" org.zeroturnaround.javarebel.Integration integration = org.zeroturnaround.javarebel.IntegrationFactory.getInstance();" +
" if (integration.isResourceReplaced($0, $1))" +
" return integration.findResource($0, $1);" +
" }");
} catch (NotFoundException e) {
}
try {
m = ctClass.getDeclaredMethod("findResources", cp.get(new String[] {String.class.getName()}));
m.insertBefore(
"{ " +
" org.zeroturnaround.javarebel.Integration integration = org.zeroturnaround.javarebel.IntegrationFactory.getInstance();" +
" if (integration.isResourceReplaced($0, $1))" +
" return integration.findResources($0, $1);" +
" }");
} catch (NotFoundException e) {
}
try {
m = ctClass.getDeclaredMethod("localGetResourceAsStream");
m.insertBefore(
"{ " +
" org.zeroturnaround.javarebel.Integration integration = org.zeroturnaround.javarebel.IntegrationFactory.getInstance();" +
" if (integration.isResourceReplaced($0, $1)) {" +
" java.net.URL url = integration.findResource($0, $1);" +
" try {" +
" return url != null ? url.openStream() : null;" +
" } catch (java.io.IOException e) {" +
" return null;" +
" }" +
" }" +
"}");
} catch (NotFoundException e) {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy