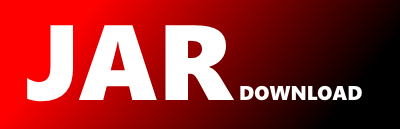
org.zkoss.zk.ui.util.Composer Maven / Gradle / Ivy
/* Composer.java
Purpose:
Description:
History:
Thu Oct 25 18:24:27 2007, Created by tomyeh
Copyright (C) 2007 Potix Corporation. All Rights Reserved.
{{IS_RIGHT
This program is distributed under LGPL Version 2.1 in the hope that
it will be useful, but WITHOUT ANY WARRANTY.
}}IS_RIGHT
*/
package org.zkoss.zk.ui.util;
import org.zkoss.zk.ui.Component;
/**
* Represents a composer to initialize a component (or a component of tree)
* when ZK loader is composing a component.
* It is the controller in the MVC pattern, while the component is the view.
*
* To initialize a component, you can implement this interface
* and then specify the class or an instance of it with the apply attribute
* as follows.
*
*
<window apply="my.MyComposer"/>
*<window apply="${a_composer}"/>
*
* Then, ZK loader will
*
* - Invoke {@link ComposerExt#doBeforeCompose}, if the composer
* also implements {@link ComposerExt}.
* - Create the component (by use of
* {@link org.zkoss.zk.ui.sys.UiFactory#newComponent}, which creates
* and initializes the component accordingly).
*
- Invokes {@link ComposerExt#doBeforeComposeChildren}, if
* {@link ComposerExt} is also implemented.
* - Composes all children, if any, of this component defined in
* the ZUML page.
* - Invokes {@link #doAfterCompose} after all children are, if any,
* composed.
* - Posts the onCreate event if necessary.
*
*
* To intercept the lifecycle of the creation of a page,
* implement {@link Initiator} and specify the class with the init directive.
*
*
Note: {@link org.zkoss.zk.ui.ext.AfterCompose} has to be implemented
* as part of a component, while {@link Composer} is a controller used
* to initialize a component (that might or might not implement
* {@link org.zkoss.zk.ui.ext.AfterCompose}).
*
*
Alternatives: in most cases, you don't implement {@link Composer} directly.
* Rather, you can extend from one of the following skeletons.
*
* - {@link org.zkoss.zk.ui.select.SelectorComposer}
* - It supports the autowiring based on Java annotation and a CSS3-based selector.
* If you don't know which one to use, use {@link org.zkoss.zk.ui.select.SelectorComposer}.
* - {@link GenericForwardComposer}
* - It supports the autowiring based on naming convention.
* You don't need to specify annotations explicitly, but it is error-prone if
* it is used properly.
*
*
* @author tomyeh
* @since 3.0.0
* @see org.zkoss.zk.ui.ext.AfterCompose
* @see ComposerExt
* @see FullComposer
* @see Initiator
*/
public interface Composer {
/** Invokes after ZK loader creates this component,
* initializes it and composes all its children, if any.
* @param comp the component has been composed
*/
public void doAfterCompose(T comp) throws Exception;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy