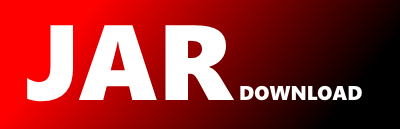
org.zkoss.bind.xel.zel.BindELResolver Maven / Gradle / Ivy
/* BindELResolver.java
Purpose:
Description:
History:
Aug 10, 2011 4:31:51 PM, Created by henrichen
Copyright (C) 2011 Potix Corporation. All Rights Reserved.
*/
package org.zkoss.bind.xel.zel;
import java.lang.reflect.Method;
import java.util.Set;
import org.zkoss.bind.BindContext;
import org.zkoss.bind.Binder;
import org.zkoss.bind.Form;
import org.zkoss.bind.FormExt;
import org.zkoss.bind.impl.BinderImpl;
import org.zkoss.bind.impl.LoadFormBindingImpl;
import org.zkoss.bind.impl.Path;
import org.zkoss.bind.sys.BinderCtrl;
import org.zkoss.bind.sys.Binding;
import org.zkoss.bind.sys.LoadBinding;
import org.zkoss.bind.sys.ReferenceBinding;
import org.zkoss.bind.sys.SaveBinding;
import org.zkoss.bind.tracker.impl.TrackerImpl;
import org.zkoss.xel.XelContext;
import org.zkoss.xel.zel.XelELResolver;
import org.zkoss.zel.CompositeELResolver;
import org.zkoss.zel.ELContext;
import org.zkoss.zel.ELException;
import org.zkoss.zel.ELResolver;
import org.zkoss.zel.PropertyNotFoundException;
import org.zkoss.zel.PropertyNotWritableException;
import org.zkoss.zel.impl.lang.EvaluationContext;
import org.zkoss.zk.ui.Component;
/**
* ELResolver for Binding; handle Form bean.
* @author henrichen
* @since 6.0.0
*/
public class BindELResolver extends XelELResolver {
private final CompositeELResolver _resolver;
public BindELResolver(XelContext ctx) {
super(ctx);
_resolver = new CompositeELResolver();
_resolver.add(new PathELResolver()); //must be the first
_resolver.add(new FormELResolver());
_resolver.add(new ListModelELResolver());
_resolver.add(new TreeModelELResolver());
_resolver.add(new ValidationMessagesELResolver());
_resolver.add(new ImplicitObjectELResolver());//ZK-1032 Able to wire Event to command method
_resolver.add(new DynamicPropertiedELResolver());//ZK-1472 Bind Include Arg
_resolver.add(super.getELResolver());
}
protected ELResolver getELResolver() {
return _resolver;
}
//ELResolver//
public Object getValue(ELContext ctx, Object base, Object property)
throws PropertyNotFoundException, ELException {
Object value = super.getValue(ctx, base, property);
final BindELContext bctx = (BindELContext)((EvaluationContext)ctx).getELContext();
Object ignoreRefVal = bctx.getAttribute(BinderImpl.IGNORE_REF_VALUE);
//ZK-950: The expression reference doesn't update while change the instant of the reference
final ReferenceBinding rbinding = value instanceof ReferenceBinding ? (ReferenceBinding)value : null;
if (rbinding != null) {
//ZK-1299 Use @ref and save after will cause null point exception
if(Boolean.TRUE.equals(ignoreRefVal)){
return rbinding;
}
value = rbinding.getValue((BindELContext) ((EvaluationContext)ctx).getELContext());
}
//If value evaluated to a ReferenceBinding, always tie the ReferenceBinding itself as the
//evaluated bean, @see TrackerImpl#getLoadBindings0() and TrackerImpl#getAllTrackerNodesByBeanNodes()
tieValue(ctx, base, property, rbinding != null ? rbinding : value, false);
return value;
}
public void setValue(ELContext ctx, Object base, Object property, Object value)
throws PropertyNotFoundException, PropertyNotWritableException, ELException {
if(base ==null){
//ZK-1085 PropertyNotWritableException when using reference binding
//for setting value to a reference-binding and simple-node (base = null), we let reference-binding handle it
Object val = super.getValue(ctx, base, property);//property resolved sets true when getValue
if(val instanceof ReferenceBinding){
((ReferenceBinding)val).setValue((BindELContext)((EvaluationContext)ctx).getELContext(),value);
return;
}
}else if (base instanceof ReferenceBinding) {
base = ((ReferenceBinding)base).getValue((BindELContext)((EvaluationContext)ctx).getELContext());
}
super.setValue(ctx, base, property, value);
tieValue(ctx, base, property, value, true);
}
private static Path getPathList(BindELContext ctx){
return (Path)ctx.getContext(Path.class);//get path, see #PathResolver
}
//save value into equal beans
private void saveEqualBeans(ELContext elCtx, Object base, String prop, Object value) {
final BindELContext ctx = (BindELContext)((EvaluationContext)elCtx).getELContext();
final BindContext bctx = (BindContext) ctx.getAttribute(BinderImpl.BINDCTX);
if (bctx.getAttribute(BinderImpl.SAVE_BASE) != null) { //recursive back, return
return;
}
ctx.setAttribute(BinderImpl.SAVE_BASE, Boolean.TRUE);
try {
final Binder binder = bctx.getBinder();
final TrackerImpl tracker = (TrackerImpl) ((BinderCtrl)binder).getTracker();
final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy