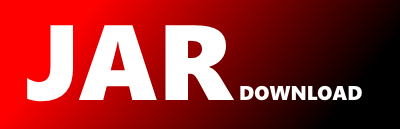
org.zkoss.zkex.zul.Borderlayout Maven / Gradle / Ivy
/* Borderlayout.java
{{IS_NOTE
Purpose:
Description:
History:
Aug 27, 2007 3:34:53 PM , Created by jumperchen
}}IS_NOTE
Copyright (C) 2007 Potix Corporation. All Rights Reserved.
{{IS_RIGHT
This program is distributed under GPL Version 2.0 in the hope that
it will be useful, but WITHOUT ANY WARRANTY.
}}IS_RIGHT
*/
package org.zkoss.zkex.zul;
import java.util.Iterator;
import org.zkoss.zk.ui.Component;
import org.zkoss.zk.ui.HtmlBasedComponent;
import org.zkoss.zk.ui.UiException;
/**
* A border layout lays out a container, arranging and resizing its components
* to fit in five regions: north, south, east, west, and center. Each region may
* contain no more than one component, and is identified by a corresponding
* constant: NORTH
, SOUTH
, EAST
,
* WEST
, and CENTER
. When adding a component to
* a container with a border layout, use one of these five constants, for
* example:
*
*
* <borderlayout>
* <north margins="1,5,1,1" size="20%" splittable="true" collapsible="true" minsize="100" maxsize="400">
* <div>
* North
* </div>
* </north>
* <west size="25%" splittable="true" autoscroll="true">
* <div>
* West
* </div>
* </west>
* <center flex="true">
* <div>
* Center
* </div>
* </center>
* <east size="25%" collapsible="true" onOpen='alert(self.id + " is open :" +event.open)'>
* <div>
* East
* </div>
* </east>
* <south size="50%" splittable="true">
* <div>
* south
* </div>
* </south>
* </borderlayout>
*
*
*
* Default {@link #getZclass}: z-border-layout. (since 3.5.0)
*
* @author jumperchen
* @since 3.0.0
*/
public class Borderlayout extends HtmlBasedComponent {
/**
* The north layout constraint (top of container).
*/
public static final String NORTH = "north";
/**
* The south layout constraint (bottom of container).
*/
public static final String SOUTH = "south";
/**
* The east layout constraint (right side of container).
*/
public static final String EAST = "east";
/**
* The west layout constraint (left side of container).
*/
public static final String WEST = "west";
/**
* The center layout constraint (middle of container).
*/
public static final String CENTER = "center";
private transient North _north;
private transient South _south;
private transient West _west;
private transient East _east;
private transient Center _center;
public Borderlayout() {
}
public North getNorth() {
return _north;
}
public South getSouth() {
return _south;
}
public West getWest() {
return _west;
}
public East getEast() {
return _east;
}
public Center getCenter() {
return _center;
}
/**
* Re-size this layout component.
*/
public void resize() {
smartUpdate("z.resize", "");
}
public boolean insertBefore(Component child, Component insertBefore) {
if (!(child instanceof LayoutRegion))
throw new UiException("Unsupported child for Borderlayout: "
+ child);
if (child instanceof North) {
if (_north != null && child != _north)
throw new UiException("Only one north child is allowed: "
+ this);
_north = (North) child;
} else if (child instanceof South) {
if (_south != null && child != _south)
throw new UiException("Only one south child is allowed: "
+ this);
_south = (South) child;
} else if (child instanceof West) {
if (_west != null && child != _west)
throw new UiException("Only one west child is allowed: " + this);
_west = (West) child;
} else if (child instanceof East) {
if (_east != null && child != _east)
throw new UiException("Only one east child is allowed: " + this);
_east = (East) child;
} else if (child instanceof Center) {
if (_center != null && child != _center)
throw new UiException("Only one center child is allowed: "
+ this);
_center = (Center) child;
}
smartUpdate("z.chchg", true);
return super.insertBefore(child, insertBefore);
}
public void onChildRemoved(Component child) {
super.onChildRemoved(child);
if (_north == child) _north = null;
else if (_south == child) _south = null;
else if (_west == child) _west = null;
else if (_east == child) _east = null;
else if (_center == child) _center = null;
}
public String getZclass() {
return _zclass == null ? "z-border-layout" : super.getZclass();
}
//Cloneable//
public Object clone() {
final Borderlayout clone = (Borderlayout) super.clone();
clone.afterUnmarshal();
return clone;
}
private void afterUnmarshal() {
for (Iterator it = getChildren().iterator(); it.hasNext();) {
final Object child = it.next();
if (child instanceof North) {
_north = (North) child;
} else if (child instanceof South) {
_south = (South) child;
} else if (child instanceof Center) {
_center = (Center) child;
} else if (child instanceof West) {
_west = (West) child;
} else if (child instanceof East) {
_east = (East) child;
}
}
}
//-- Serializable --//
private synchronized void readObject(java.io.ObjectInputStream s)
throws java.io.IOException, ClassNotFoundException {
s.defaultReadObject();
afterUnmarshal();
}
}