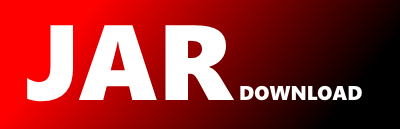
org.znerd.util.test.TestUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of znerd-util Show documentation
Show all versions of znerd-util Show documentation
Set of utility classes (with associated unit tests) used by other znerd.org-projects
The newest version!
// See the COPYRIGHT file for copyright and license information
package org.znerd.util.test;
import java.lang.reflect.Constructor;
import java.lang.reflect.Modifier;
import org.znerd.util.ArrayUtils;
import org.znerd.util.text.TextUtils;
public class TestUtils {
/**
* Tests if the specified utility class has a matching constructor. The following is checked:
*
* - There must be exactly 1 constructor.
*
- This constructor must be marked
private.
* - Invoking the constructor should not throw any exception.
*
*
* @param clazz The class to check, should not be null
.
* @throws IllegalArgumentException if clazz == null
.
* @throws TestFailedException In case an issue is found.
*/
public static final void testUtilityClassConstructor(Class> clazz) throws IllegalArgumentException, TestFailedException {
if (clazz == null) {
throw new IllegalArgumentException("clazz == null");
}
Constructor> con = assertOneConstructor(clazz);
assertPrivateConstructor(con);
assertNoExceptionFromConstructor(con);
}
private static Constructor> assertOneConstructor(Class> clazz) throws TestFailedException {
Constructor>[] cons = clazz.getDeclaredConstructors();
int constructorCount = ArrayUtils.countElements(cons);
if (constructorCount != 1) {
throw new TestFailedException("Expected exactly 1 constructor in class " + clazz.getName() + ". However, " + constructorCount + " were found.");
}
return cons[0];
}
private static void assertPrivateConstructor(Constructor> con) throws TestFailedException {
int modifiers = con.getModifiers();
if (Modifier.PRIVATE != modifiers) {
String className = con.getDeclaringClass().getName();
throw new TestFailedException("Expected " + className + " constructor to be private (modifiers==" + Modifier.PRIVATE + "). Instead: modifiers==" + modifiers + '.');
}
}
private static void assertNoExceptionFromConstructor(Constructor> con) throws TestFailedException {
try {
con.setAccessible(true);
con.newInstance((Object[]) null);
} catch (Throwable cause) {
String className = con.getDeclaringClass().getName();
throw new TestFailedException("Calling the " + className + " constructor resulted in an exception of class " + cause.getClass().getName() + ". The exception message is: " + TextUtils.quote(cause.getMessage()) + '.', cause);
}
}
private TestUtils() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy