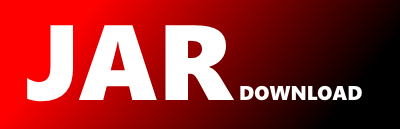
org.zwobble.mammoth.internal.docx.InMemoryDocxFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mammoth Show documentation
Show all versions of mammoth Show documentation
Convert Word documents to simple and clean HTML
package org.zwobble.mammoth.internal.docx;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
import static org.zwobble.mammoth.internal.util.Maps.eagerMapValues;
import static org.zwobble.mammoth.internal.util.Maps.lookup;
import static org.zwobble.mammoth.internal.util.Streams.toByteArray;
public class InMemoryDocxFile implements DocxFile {
public static DocxFile fromStream(InputStream stream) throws IOException {
ZipInputStream zipStream = new ZipInputStream(stream);
Map entries = new HashMap<>();
ZipEntry entry;
while ((entry = zipStream.getNextEntry()) != null) {
entries.put(entry.getName(), toByteArray(zipStream));
}
return new InMemoryDocxFile(entries);
}
public static DocxFile fromStrings(Map entries) {
return new InMemoryDocxFile(eagerMapValues(entries, value -> value.getBytes(StandardCharsets.UTF_8)));
}
private final Map entries;
public InMemoryDocxFile(Map entries) {
this.entries = entries;
}
@Override
public Optional tryGetInputStream(String name) throws IOException {
return lookup(entries, name)
.map(ByteArrayInputStream::new);
}
@Override
public void close() throws IOException {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy