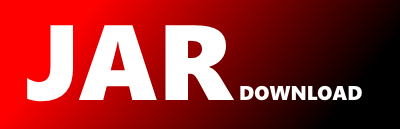
org.zwobble.mammoth.internal.util.Maps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mammoth Show documentation
Show all versions of mammoth Show documentation
Convert Word documents to simple and clean HTML
package org.zwobble.mammoth.internal.util;
import java.util.*;
import java.util.function.Function;
public class Maps {
public static Map map() {
return Collections.emptyMap();
}
public static Map map(K key1, V value1) {
return Collections.singletonMap(key1, value1);
}
public static Map map(K key1, V value1, K key2, V value2) {
Map map = new HashMap<>();
map.put(key1, value1);
map.put(key2, value2);
return map;
}
public static Map map(K key1, V value1, K key2, V value2, K key3, V value3) {
Map map = new HashMap<>();
map.put(key1, value1);
map.put(key2, value2);
map.put(key3, value3);
return map;
}
public static Map.Entry entry(K key, V value) {
return new AbstractMap.SimpleImmutableEntry(key, value);
}
public static Optional lookup(Map map, K key) {
return Optional.ofNullable(map.get(key));
}
public static Map toMapWithKey(Iterable iterable, Function function) {
return toMap(iterable, element -> entry(function.apply(element), element));
}
public static Map toMap(Iterable iterable, Function> function) {
Map map = new HashMap<>();
for (T element : iterable) {
Map.Entry entry = function.apply(element);
map.put(entry.getKey(), entry.getValue());
}
return map;
}
public static Map> toMultiMapWithKey(Iterable iterable, Function function) {
return toMultiMap(iterable, element -> entry(function.apply(element), element));
}
public static Map> toMultiMap(Iterable iterable, Function> function) {
Map> map = new HashMap<>();
for (T element : iterable) {
Map.Entry pair = function.apply(element);
map.computeIfAbsent(pair.getKey(), key -> new ArrayList())
.add(pair.getValue());
}
return map;
}
public static Map eagerMapKeys(Map map, Function function) {
Map result = new HashMap<>();
for (Map.Entry element : map.entrySet()) {
result.put(function.apply(element.getKey()), element.getValue());
}
return result;
}
public static Map eagerMapValues(Map map, Function function) {
Map result = new HashMap<>();
for (Map.Entry element : map.entrySet()) {
result.put(element.getKey(), function.apply(element.getValue()));
}
return result;
}
public static Builder builder() {
return new Builder<>();
}
public static class Builder {
private final Map values = new HashMap<>();
public Builder put(K key, V value) {
values.put(key, value);
return this;
}
public Map build() {
return values;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy