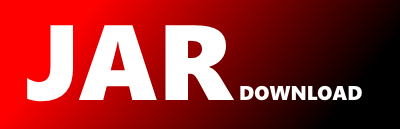
party.iroiro.luajava.Lua51 Maven / Gradle / Ivy
/*
* Copyright (C) 2022 the original author or authors.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package party.iroiro.luajava;
import java.util.concurrent.atomic.AtomicReference;
import static party.iroiro.luajava.Lua51Consts.*;
/**
* A thin wrapper around a Lua 5.1 Lua state
*/
public class Lua51 extends AbstractLua {
private final static AtomicReference natives = new AtomicReference<>();
/**
* Creates a new Lua state
*
* @throws LinkageError if Lua 5.1 natives unavailable
*/
public Lua51() throws LinkageError {
super(getNatives());
}
protected Lua51(long L, int id, AbstractLua main) {
super(main.getLuaNative(), L, id, main);
}
private static LuaNative getNatives() throws LinkageError {
synchronized (natives) {
if (natives.get() == null) {
try {
natives.set(new Lua51Natives());
} catch (IllegalStateException e) {
throw new LinkageError("Unable to find natives or init", e);
}
}
return natives.get();
}
}
@Override
protected AbstractLua newThread(long L, int id, AbstractLua mainThread) {
return new Lua51(L, id, mainThread);
}
@Override
public LuaError convertError(int code) {
switch (code) {
case 0:
return LuaError.OK;
case LUA_YIELD:
return LuaError.YIELD;
case LUA_ERRRUN:
return LuaError.RUNTIME;
case LUA_ERRSYNTAX:
return LuaError.SYNTAX;
case LUA_ERRMEM:
return LuaError.MEMORY;
case LUA_ERRERR:
return LuaError.HANDLER;
default:
return null;
}
}
@Override
public LuaType convertType(int code) {
switch (code) {
case LUA_TBOOLEAN:
return LuaType.BOOLEAN;
case LUA_TFUNCTION:
return LuaType.FUNCTION;
case LUA_TLIGHTUSERDATA:
return LuaType.LIGHTUSERDATA;
case LUA_TNIL:
return LuaType.NIL;
case LUA_TNONE:
return LuaType.NONE;
case LUA_TNUMBER:
return LuaType.NUMBER;
case LUA_TSTRING:
return LuaType.STRING;
case LUA_TTABLE:
return LuaType.TABLE;
case LUA_TTHREAD:
return LuaType.THREAD;
case LUA_TUSERDATA:
return LuaType.USERDATA;
default:
return null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy