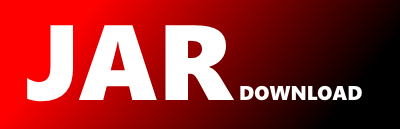
party.iroiro.luajava.Lua51Natives Maven / Gradle / Ivy
/*
* Copyright (C) 2022 the original author or authors.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package party.iroiro.luajava;
import java.util.concurrent.atomic.AtomicBoolean;
import java.nio.Buffer;
import com.badlogic.gdx.utils.SharedLibraryLoader;
/**
* Lua C API wrappers
*
*
* This file is programmatically generated from the Lua 5.1 Reference Manual.
*
*
* The following functions are excluded:
*
* luaL_addchar
* luaL_addlstring
* luaL_addsize
* luaL_addstring
* luaL_addvalue
* luaL_argcheck
* luaL_argerror
* luaL_buffinit
* luaL_checkany
* luaL_checkint
* luaL_checkinteger
* luaL_checklong
* luaL_checklstring
* luaL_checknumber
* luaL_checkoption
* luaL_checkstack
* luaL_checkstring
* luaL_checktype
* luaL_checkudata
* luaL_dofile
* luaL_error
* luaL_loadbuffer
* luaL_loadfile
* luaL_optint
* luaL_optinteger
* luaL_optlong
* luaL_optlstring
* luaL_optnumber
* luaL_optstring
* luaL_prepbuffer
* luaL_pushresult
* luaL_register
* lua_atpanic
* lua_call
* lua_cpcall
* lua_dump
* lua_getallocf
* lua_gethook
* lua_getinfo
* lua_getlocal
* lua_getstack
* lua_load
* lua_newstate
* lua_pushcclosure
* lua_pushcfunction
* lua_pushfstring
* lua_pushliteral
* lua_pushlstring
* lua_pushvfstring
* lua_register
* lua_setallocf
* lua_sethook
* lua_setlocal
* lua_tocfunction
* lua_tolstring
*
*/
@SuppressWarnings({"unused", "rawtypes"})
public class Lua51Natives extends LuaNative {
/*JNI
#include "luacustomamalg.h"
#include "lua.hpp"
#include "jni.h"
#include "jua.h"
#include "luacomp.h"
#include "juaapi.h"
#include "jualib.h"
#include "juaamalg.h"
#include "luacustom.h"
*/
private final static AtomicBoolean loaded = new AtomicBoolean(false);
protected Lua51Natives() throws IllegalStateException {
synchronized (loaded) {
if (loaded.get()) { return; }
try {
new SharedLibraryLoader().load("lua51");
if (initBindings() != 0) {
throw new RuntimeException("Unable to init bindings");
}
loaded.set(true);
} catch (Throwable e) {
throw new IllegalStateException(e);
}
}
}
private native static int initBindings() throws Exception; /*
return (jint) initLua51Bindings(env);
*/
/**
* Get LUA_REGISTRYINDEX
, which is a computed compile time constant
*/
protected native int getRegistryIndex(); /*
return LUA_REGISTRYINDEX;
*/
/**
* Wrapper of lua_checkstack
*
*
* [-0, +0, m]
*
*
*
* int lua_checkstack (lua_State *L, int extra);
*
*
*
* Ensures that there are at least extra
free stack slots in the stack.
* It returns false if it cannot grow the stack to that size.
* This function never shrinks the stack;
* if the stack is already larger than the new size,
* it is left unchanged.
*
*
* @param ptr the lua_State*
pointer
* @param extra extra slots
* @return see description
*/
protected native int lua_checkstack(long ptr, int extra); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_checkstack((lua_State *) L, (int) extra);
return returnValueReceiver;
*/
/**
* Wrapper of lua_close
*
*
* [-0, +0, -]
*
*
*
* void lua_close (lua_State *L);
*
*
*
* Destroys all objects in the given Lua state
* (calling the corresponding garbage-collection metamethods, if any)
* and frees all dynamic memory used by this state.
* On several platforms, you may not need to call this function,
* because all resources are naturally released when the host program ends.
* On the other hand, long-running programs,
* such as a daemon or a web server,
* might need to release states as soon as they are not needed,
* to avoid growing too large.
*
*
* @param ptr the lua_State*
pointer
*/
protected native void lua_close(long ptr); /*
lua_State * L = (lua_State *) ptr;
lua_close((lua_State *) L);
*/
/**
* Wrapper of lua_concat
*
*
* [-n, +1, e]
*
*
*
* void lua_concat (lua_State *L, int n);
*
*
*
* Concatenates the n
values at the top of the stack,
* pops them, and leaves the result at the top.
* If n
is 1, the result is the single value on the stack
* (that is, the function does nothing);
* if n
is 0, the result is the empty string.
* Concatenation is performed following the usual semantics of Lua
* (see §2.5.4).
*
*
* @param ptr the lua_State*
pointer
* @param n the number of elements
*/
protected native void lua_concat(long ptr, int n); /*
lua_State * L = (lua_State *) ptr;
lua_concat((lua_State *) L, (int) n);
*/
/**
* Wrapper of lua_createtable
*
*
* [-0, +1, m]
*
*
*
* void lua_createtable (lua_State *L, int narr, int nrec);
*
*
*
* Creates a new empty table and pushes it onto the stack.
* The new table has space pre-allocated
* for narr
array elements and nrec
non-array elements.
* This pre-allocation is useful when you know exactly how many elements
* the table will have.
* Otherwise you can use the function lua_newtable
.
*
*
* @param ptr the lua_State*
pointer
* @param narr the number of pre-allocated array elements
* @param nrec the number of pre-allocated non-array elements
*/
protected native void lua_createtable(long ptr, int narr, int nrec); /*
lua_State * L = (lua_State *) ptr;
lua_createtable((lua_State *) L, (int) narr, (int) nrec);
*/
/**
* Wrapper of lua_equal
*
*
* [-0, +0, e]
*
*
*
* int lua_equal (lua_State *L, int index1, int index2);
*
*
*
* Returns 1 if the two values in acceptable indices index1
and
* index2
are equal,
* following the semantics of the Lua ==
operator
* (that is, may call metamethods).
* Otherwise returns 0.
* Also returns 0 if any of the indices is non valid.
*
*
* @param ptr the lua_State*
pointer
* @param index1 the stack position of the first element
* @param index2 the stack position of the second element
* @return see description
*/
protected native int lua_equal(long ptr, int index1, int index2); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_equal((lua_State *) L, (int) index1, (int) index2);
return returnValueReceiver;
*/
/**
* Wrapper of lua_error
*
*
* [-1, +0, v]
*
*
*
* int lua_error (lua_State *L);
*
*
*
* Generates a Lua error.
* The error message (which can actually be a Lua value of any type)
* must be on the stack top.
* This function does a long jump,
* and therefore never returns.
* (see luaL_error
).
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_error(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_error((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_gc
*
*
* [-0, +0, e]
*
*
*
* int lua_gc (lua_State *L, int what, int data);
*
*
*
* Controls the garbage collector.
*
*
*
* This function performs several tasks,
* according to the value of the parameter what
:
*
*
*
*
*
* -
*
LUA_GCSTOP
:
* stops the garbage collector.
*
*
* -
*
LUA_GCRESTART
:
* restarts the garbage collector.
*
*
* -
*
LUA_GCCOLLECT
:
* performs a full garbage-collection cycle.
*
*
* -
*
LUA_GCCOUNT
:
* returns the current amount of memory (in Kbytes) in use by Lua.
*
*
* -
*
LUA_GCCOUNTB
:
* returns the remainder of dividing the current amount of bytes of
* memory in use by Lua by 1024.
*
*
* -
*
LUA_GCSTEP
:
* performs an incremental step of garbage collection.
* The step "size" is controlled by data
* (larger values mean more steps) in a non-specified way.
* If you want to control the step size
* you must experimentally tune the value of data
.
* The function returns 1 if the step finished a
* garbage-collection cycle.
*
*
* -
*
LUA_GCSETPAUSE
:
* sets data
as the new value
* for the pause of the collector (see §2.10).
* The function returns the previous value of the pause.
*
*
* -
*
LUA_GCSETSTEPMUL
:
* sets data
as the new value for the step multiplier of
* the collector (see §2.10).
* The function returns the previous value of the step multiplier.
*
*
*
*
* @param ptr the lua_State*
pointer
* @param what what
* @param data data
* @return see description
*/
protected native int lua_gc(long ptr, int what, int data); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_gc((lua_State *) L, (int) what, (int) data);
return returnValueReceiver;
*/
/**
* Wrapper of lua_getfenv
*
*
* [-0, +1, -]
*
*
*
* void lua_getfenv (lua_State *L, int index);
*
*
*
* Pushes onto the stack the environment table of
* the value at the given index.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_getfenv(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_getfenv((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_getfield
*
*
* [-0, +1, e]
*
*
*
* void lua_getfield (lua_State *L, int index, const char *k);
*
*
*
* Pushes onto the stack the value t[k]
,
* where t
is the value at the given valid index.
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.8).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param k the field name
*/
protected native void lua_getfield(long ptr, int index, String k); /*
lua_State * L = (lua_State *) ptr;
lua_getfield((lua_State *) L, (int) index, (const char *) k);
*/
/**
* Wrapper of lua_getfield
*
*
* [-0, +1, e]
*
*
*
* void lua_getfield (lua_State *L, int index, const char *k);
*
*
*
* Pushes onto the stack the value t[k]
,
* where t
is the value at the given valid index.
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.8).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param k the field name
*/
protected native void luaJ_getfield(long ptr, int index, String k); /*
lua_State * L = (lua_State *) ptr;
lua_getfield((lua_State *) L, (int) index, (const char *) k);
*/
/**
* Wrapper of lua_getglobal
*
*
* [-0, +1, e]
*
*
*
* void lua_getglobal (lua_State *L, const char *name);
*
*
*
* Pushes onto the stack the value of the global name
.
* It is defined as a macro:
*
*
*
*
* #define lua_getglobal(L,s) lua_getfield(L, LUA_GLOBALSINDEX, s)
*
*
* @param ptr the lua_State*
pointer
* @param name the name
*/
protected native void lua_getglobal(long ptr, String name); /*
lua_State * L = (lua_State *) ptr;
lua_getglobal((lua_State *) L, (const char *) name);
*/
/**
* Wrapper of lua_getglobal
*
*
* [-0, +1, e]
*
*
*
* void lua_getglobal (lua_State *L, const char *name);
*
*
*
* Pushes onto the stack the value of the global name
.
* It is defined as a macro:
*
*
*
*
* #define lua_getglobal(L,s) lua_getfield(L, LUA_GLOBALSINDEX, s)
*
*
* @param ptr the lua_State*
pointer
* @param name the name
*/
protected native void luaJ_getglobal(long ptr, String name); /*
lua_State * L = (lua_State *) ptr;
lua_getglobal((lua_State *) L, (const char *) name);
*/
/**
* Wrapper of lua_getmetatable
*
*
* [-0, +(0|1), -]
*
*
*
* int lua_getmetatable (lua_State *L, int index);
*
*
*
* Pushes onto the stack the metatable of the value at the given
* acceptable index.
* If the index is not valid,
* or if the value does not have a metatable,
* the function returns 0 and pushes nothing on the stack.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_getmetatable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_getmetatable((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_gettable
*
*
* [-1, +1, e]
*
*
*
* void lua_gettable (lua_State *L, int index);
*
*
*
* Pushes onto the stack the value t[k]
,
* where t
is the value at the given valid index
* and k
is the value at the top of the stack.
*
*
*
* This function pops the key from the stack
* (putting the resulting value in its place).
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.8).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_gettable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_gettable((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_gettable
*
*
* [-1, +1, e]
*
*
*
* void lua_gettable (lua_State *L, int index);
*
*
*
* Pushes onto the stack the value t[k]
,
* where t
is the value at the given valid index
* and k
is the value at the top of the stack.
*
*
*
* This function pops the key from the stack
* (putting the resulting value in its place).
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.8).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void luaJ_gettable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_gettable((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_gettop
*
*
* [-0, +0, -]
*
*
*
* int lua_gettop (lua_State *L);
*
*
*
* Returns the index of the top element in the stack.
* Because indices start at 1,
* this result is equal to the number of elements in the stack
* (and so 0 means an empty stack).
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_gettop(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_gettop((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_insert
*
*
* [-1, +1, -]
*
*
*
* void lua_insert (lua_State *L, int index);
*
*
*
* Moves the top element into the given valid index,
* shifting up the elements above this index to open space.
* Cannot be called with a pseudo-index,
* because a pseudo-index is not an actual stack position.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_insert(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_insert((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_isboolean
*
*
* [-0, +0, -]
*
*
*
* int lua_isboolean (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index has type boolean,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isboolean(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isboolean((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_iscfunction
*
*
* [-0, +0, -]
*
*
*
* int lua_iscfunction (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index is a C function,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_iscfunction(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_iscfunction((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isfunction
*
*
* [-0, +0, -]
*
*
*
* int lua_isfunction (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index is a function
* (either C or Lua), and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isfunction(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isfunction((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_islightuserdata
*
*
* [-0, +0, -]
*
*
*
* int lua_islightuserdata (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index is a light userdata,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_islightuserdata(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_islightuserdata((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isnil
*
*
* [-0, +0, -]
*
*
*
* int lua_isnil (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index is nil,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isnil(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isnil((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isnone
*
*
* [-0, +0, -]
*
*
*
* int lua_isnone (lua_State *L, int index);
*
*
*
* Returns 1 if the given acceptable index is not valid
* (that is, it refers to an element outside the current stack),
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isnone(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isnone((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isnoneornil
*
*
* [-0, +0, -]
*
*
*
* int lua_isnoneornil (lua_State *L, int index);
*
*
*
* Returns 1 if the given acceptable index is not valid
* (that is, it refers to an element outside the current stack)
* or if the value at this index is nil,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isnoneornil(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isnoneornil((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isnumber
*
*
* [-0, +0, -]
*
*
*
* int lua_isnumber (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index is a number
* or a string convertible to a number,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isnumber(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isnumber((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isstring
*
*
* [-0, +0, -]
*
*
*
* int lua_isstring (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index is a string
* or a number (which is always convertible to a string),
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isstring(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isstring((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_istable
*
*
* [-0, +0, -]
*
*
*
* int lua_istable (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index is a table,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_istable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_istable((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isthread
*
*
* [-0, +0, -]
*
*
*
* int lua_isthread (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index is a thread,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isthread(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isthread((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isuserdata
*
*
* [-0, +0, -]
*
*
*
* int lua_isuserdata (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given acceptable index is a userdata
* (either full or light), and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isuserdata(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isuserdata((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_lessthan
*
*
* [-0, +0, e]
*
*
*
* int lua_lessthan (lua_State *L, int index1, int index2);
*
*
*
* Returns 1 if the value at acceptable index index1
is smaller
* than the value at acceptable index index2
,
* following the semantics of the Lua <
operator
* (that is, may call metamethods).
* Otherwise returns 0.
* Also returns 0 if any of the indices is non valid.
*
*
* @param ptr the lua_State*
pointer
* @param index1 the stack position of the first element
* @param index2 the stack position of the second element
* @return see description
*/
protected native int lua_lessthan(long ptr, int index1, int index2); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_lessthan((lua_State *) L, (int) index1, (int) index2);
return returnValueReceiver;
*/
/**
* Wrapper of lua_newtable
*
*
* [-0, +1, m]
*
*
*
* void lua_newtable (lua_State *L);
*
*
*
* Creates a new empty table and pushes it onto the stack.
* It is equivalent to lua_createtable(L, 0, 0)
.
*
*
* @param ptr the lua_State*
pointer
*/
protected native void lua_newtable(long ptr); /*
lua_State * L = (lua_State *) ptr;
lua_newtable((lua_State *) L);
*/
/**
* Wrapper of lua_newthread
*
*
* [-0, +1, m]
*
*
*
* lua_State *lua_newthread (lua_State *L);
*
*
*
* Creates a new thread, pushes it on the stack,
* and returns a pointer to a lua_State
that represents this new thread.
* The new state returned by this function shares with the original state
* all global objects (such as tables),
* but has an independent execution stack.
*
*
*
* There is no explicit function to close or to destroy a thread.
* Threads are subject to garbage collection,
* like any Lua object.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native long lua_newthread(long ptr); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_newthread((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_newuserdata
*
*
* [-0, +1, m]
*
*
*
* void *lua_newuserdata (lua_State *L, size_t size);
*
*
*
* This function allocates a new block of memory with the given size,
* pushes onto the stack a new full userdata with the block address,
* and returns this address.
*
*
*
* Userdata represent C values in Lua.
* A full userdata represents a block of memory.
* It is an object (like a table):
* you must create it, it can have its own metatable,
* and you can detect when it is being collected.
* A full userdata is only equal to itself (under raw equality).
*
*
*
* When Lua collects a full userdata with a gc
metamethod,
* Lua calls the metamethod and marks the userdata as finalized.
* When this userdata is collected again then
* Lua frees its corresponding memory.
*
*
* @param ptr the lua_State*
pointer
* @param size size
* @return see description
*/
protected native long lua_newuserdata(long ptr, int size); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_newuserdata((lua_State *) L, (size_t) size);
return returnValueReceiver;
*/
/**
* Wrapper of lua_next
*
*
* [-1, +(2|0), e]
*
*
*
* int lua_next (lua_State *L, int index);
*
*
*
* Pops a key from the stack,
* and pushes a key-value pair from the table at the given index
* (the "next" pair after the given key).
* If there are no more elements in the table,
* then lua_next
returns 0 (and pushes nothing).
*
*
*
* A typical traversal looks like this:
*
*
*
*
* /* table is in the stack at index 't' */
* lua_pushnil(L); /* first key */
* while (lua_next(L, t) != 0) {
* /* uses 'key' (at index -2) and 'value' (at index -1) */
* printf("%s - %s\n",
* lua_typename(L, lua_type(L, -2)),
* lua_typename(L, lua_type(L, -1)));
* /* removes 'value'; keeps 'key' for next iteration */
* lua_pop(L, 1);
* }
*
*
*
* While traversing a table,
* do not call lua_tolstring
directly on a key,
* unless you know that the key is actually a string.
* Recall that lua_tolstring
changes
* the value at the given index;
* this confuses the next call to lua_next
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_next(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_next((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_objlen
*
*
* [-0, +0, -]
*
*
*
* size_t lua_objlen (lua_State *L, int index);
*
*
*
* Returns the "length" of the value at the given acceptable index:
* for strings, this is the string length;
* for tables, this is the result of the length operator ('#
');
* for userdata, this is the size of the block of memory allocated
* for the userdata;
* for other values, it is 0.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_objlen(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_objlen((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_pcall
*
*
* [-(nargs + 1), +(nresults|1), -]
*
*
*
* int lua_pcall (lua_State *L, int nargs, int nresults, int errfunc);
*
*
*
* Calls a function in protected mode.
*
*
*
* Both nargs
and nresults
have the same meaning as
* in lua_call
.
* If there are no errors during the call,
* lua_pcall
behaves exactly like lua_call
.
* However, if there is any error,
* lua_pcall
catches it,
* pushes a single value on the stack (the error message),
* and returns an error code.
* Like lua_call
,
* lua_pcall
always removes the function
* and its arguments from the stack.
*
*
*
* If errfunc
is 0,
* then the error message returned on the stack
* is exactly the original error message.
* Otherwise, errfunc
is the stack index of an
* error handler function.
* (In the current implementation, this index cannot be a pseudo-index.)
* In case of runtime errors,
* this function will be called with the error message
* and its return value will be the message returned on the stack by lua_pcall
.
*
*
*
* Typically, the error handler function is used to add more debug
* information to the error message, such as a stack traceback.
* Such information cannot be gathered after the return of lua_pcall
,
* since by then the stack has unwound.
*
*
*
* The lua_pcall
function returns 0 in case of success
* or one of the following error codes
* (defined in lua.h
):
*
*
*
*
*
* -
*
LUA_ERRRUN
:
* a runtime error.
*
*
* -
*
LUA_ERRMEM
:
* memory allocation error.
* For such errors, Lua does not call the error handler function.
*
*
* -
*
LUA_ERRERR
:
* error while running the error handler function.
*
*
*
*
* @param ptr the lua_State*
pointer
* @param nargs the number of arguments that you pushed onto the stack
* @param nresults the number of results, or LUA_MULTRET
* @param errfunc 0 or the stack index of an error handler function
* @return see description
*/
protected native int lua_pcall(long ptr, int nargs, int nresults, int errfunc); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_pcall((lua_State *) L, (int) nargs, (int) nresults, (int) errfunc);
return returnValueReceiver;
*/
/**
* Wrapper of lua_pcall
*
*
* [-(nargs + 1), +(nresults|1), -]
*
*
*
* int lua_pcall (lua_State *L, int nargs, int nresults, int errfunc);
*
*
*
* Calls a function in protected mode.
*
*
*
* Both nargs
and nresults
have the same meaning as
* in lua_call
.
* If there are no errors during the call,
* lua_pcall
behaves exactly like lua_call
.
* However, if there is any error,
* lua_pcall
catches it,
* pushes a single value on the stack (the error message),
* and returns an error code.
* Like lua_call
,
* lua_pcall
always removes the function
* and its arguments from the stack.
*
*
*
* If errfunc
is 0,
* then the error message returned on the stack
* is exactly the original error message.
* Otherwise, errfunc
is the stack index of an
* error handler function.
* (In the current implementation, this index cannot be a pseudo-index.)
* In case of runtime errors,
* this function will be called with the error message
* and its return value will be the message returned on the stack by lua_pcall
.
*
*
*
* Typically, the error handler function is used to add more debug
* information to the error message, such as a stack traceback.
* Such information cannot be gathered after the return of lua_pcall
,
* since by then the stack has unwound.
*
*
*
* The lua_pcall
function returns 0 in case of success
* or one of the following error codes
* (defined in lua.h
):
*
*
*
*
*
* -
*
LUA_ERRRUN
:
* a runtime error.
*
*
* -
*
LUA_ERRMEM
:
* memory allocation error.
* For such errors, Lua does not call the error handler function.
*
*
* -
*
LUA_ERRERR
:
* error while running the error handler function.
*
*
*
*
* @param ptr the lua_State*
pointer
* @param nargs the number of arguments that you pushed onto the stack
* @param nresults the number of results, or LUA_MULTRET
* @param errfunc 0 or the stack index of an error handler function
*/
protected native void luaJ_pcall(long ptr, int nargs, int nresults, int errfunc); /*
lua_State * L = (lua_State *) ptr;
lua_pcall((lua_State *) L, (int) nargs, (int) nresults, (int) errfunc);
*/
/**
* Wrapper of lua_pop
*
*
* [-n, +0, -]
*
*
*
* void lua_pop (lua_State *L, int n);
*
*
*
* Pops n
elements from the stack.
*
*
* @param ptr the lua_State*
pointer
* @param n the number of elements
*/
protected native void lua_pop(long ptr, int n); /*
lua_State * L = (lua_State *) ptr;
lua_pop((lua_State *) L, (int) n);
*/
/**
* Wrapper of lua_pushboolean
*
*
* [-0, +1, -]
*
*
*
* void lua_pushboolean (lua_State *L, int b);
*
*
*
* Pushes a boolean value with value b
onto the stack.
*
*
* @param ptr the lua_State*
pointer
* @param b boolean
*/
protected native void lua_pushboolean(long ptr, int b); /*
lua_State * L = (lua_State *) ptr;
lua_pushboolean((lua_State *) L, (int) b);
*/
/**
* Wrapper of lua_pushinteger
*
*
* [-0, +1, -]
*
*
*
* void lua_pushinteger (lua_State *L, lua_Integer n);
*
*
*
* Pushes a number with value n
onto the stack.
*
*
* @param ptr the lua_State*
pointer
* @param n the number of elements
*/
protected native void lua_pushinteger(long ptr, int n); /*
lua_State * L = (lua_State *) ptr;
lua_pushinteger((lua_State *) L, (lua_Integer) n);
*/
/**
* Wrapper of lua_pushlightuserdata
*
*
* [-0, +1, -]
*
*
*
* void lua_pushlightuserdata (lua_State *L, void *p);
*
*
*
* Pushes a light userdata onto the stack.
*
*
*
* Userdata represent C values in Lua.
* A light userdata represents a pointer.
* It is a value (like a number):
* you do not create it, it has no individual metatable,
* and it is not collected (as it was never created).
* A light userdata is equal to "any"
* light userdata with the same C address.
*
*
* @param ptr the lua_State*
pointer
* @param p the pointer
*/
protected native void lua_pushlightuserdata(long ptr, long p); /*
lua_State * L = (lua_State *) ptr;
lua_pushlightuserdata((lua_State *) L, (void *) p);
*/
/**
* Wrapper of lua_pushnil
*
*
* [-0, +1, -]
*
*
*
* void lua_pushnil (lua_State *L);
*
*
*
* Pushes a nil value onto the stack.
*
*
* @param ptr the lua_State*
pointer
*/
protected native void lua_pushnil(long ptr); /*
lua_State * L = (lua_State *) ptr;
lua_pushnil((lua_State *) L);
*/
/**
* Wrapper of lua_pushnumber
*
*
* [-0, +1, -]
*
*
*
* void lua_pushnumber (lua_State *L, lua_Number n);
*
*
*
* Pushes a number with value n
onto the stack.
*
*
* @param ptr the lua_State*
pointer
* @param n the number of elements
*/
protected native void lua_pushnumber(long ptr, double n); /*
lua_State * L = (lua_State *) ptr;
lua_pushnumber((lua_State *) L, (lua_Number) n);
*/
/**
* Wrapper of lua_pushstring
*
*
* [-0, +1, m]
*
*
*
* void lua_pushstring (lua_State *L, const char *s);
*
*
*
* Pushes the zero-terminated string pointed to by s
* onto the stack.
* Lua makes (or reuses) an internal copy of the given string,
* so the memory at s
can be freed or reused immediately after
* the function returns.
* The string cannot contain embedded zeros;
* it is assumed to end at the first zero.
*
*
* @param ptr the lua_State*
pointer
* @param s the string
*/
protected native void lua_pushstring(long ptr, String s); /*
lua_State * L = (lua_State *) ptr;
lua_pushstring((lua_State *) L, (const char *) s);
*/
/**
* Wrapper of lua_pushstring
*
*
* [-0, +1, m]
*
*
*
* void lua_pushstring (lua_State *L, const char *s);
*
*
*
* Pushes the zero-terminated string pointed to by s
* onto the stack.
* Lua makes (or reuses) an internal copy of the given string,
* so the memory at s
can be freed or reused immediately after
* the function returns.
* The string cannot contain embedded zeros;
* it is assumed to end at the first zero.
*
*
* @param ptr the lua_State*
pointer
* @param s the string
*/
protected native void luaJ_pushstring(long ptr, String s); /*
lua_State * L = (lua_State *) ptr;
lua_pushstring((lua_State *) L, (const char *) s);
*/
/**
* Wrapper of lua_pushthread
*
*
* [-0, +1, -]
*
*
*
* int lua_pushthread (lua_State *L);
*
*
*
* Pushes the thread represented by L
onto the stack.
* Returns 1 if this thread is the main thread of its state.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_pushthread(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_pushthread((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_pushvalue
*
*
* [-0, +1, -]
*
*
*
* void lua_pushvalue (lua_State *L, int index);
*
*
*
* Pushes a copy of the element at the given valid index
* onto the stack.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_pushvalue(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_pushvalue((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_rawequal
*
*
* [-0, +0, -]
*
*
*
* int lua_rawequal (lua_State *L, int index1, int index2);
*
*
*
* Returns 1 if the two values in acceptable indices index1
and
* index2
are primitively equal
* (that is, without calling metamethods).
* Otherwise returns 0.
* Also returns 0 if any of the indices are non valid.
*
*
* @param ptr the lua_State*
pointer
* @param index1 the stack position of the first element
* @param index2 the stack position of the second element
* @return see description
*/
protected native int lua_rawequal(long ptr, int index1, int index2); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_rawequal((lua_State *) L, (int) index1, (int) index2);
return returnValueReceiver;
*/
/**
* Wrapper of lua_rawget
*
*
* [-1, +1, -]
*
*
*
* void lua_rawget (lua_State *L, int index);
*
*
*
* Similar to lua_gettable
, but does a raw access
* (i.e., without metamethods).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_rawget(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_rawget((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_rawget
*
*
* [-1, +1, -]
*
*
*
* void lua_rawget (lua_State *L, int index);
*
*
*
* Similar to lua_gettable
, but does a raw access
* (i.e., without metamethods).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void luaJ_rawget(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_rawget((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_rawgeti
*
*
* [-0, +1, -]
*
*
*
* void lua_rawgeti (lua_State *L, int index, int n);
*
*
*
* Pushes onto the stack the value t[n]
,
* where t
is the value at the given valid index.
* The access is raw;
* that is, it does not invoke metamethods.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param n the number of elements
*/
protected native void lua_rawgeti(long ptr, int index, int n); /*
lua_State * L = (lua_State *) ptr;
lua_rawgeti((lua_State *) L, (int) index, (int) n);
*/
/**
* Wrapper of lua_rawgeti
*
*
* [-0, +1, -]
*
*
*
* void lua_rawgeti (lua_State *L, int index, int n);
*
*
*
* Pushes onto the stack the value t[n]
,
* where t
is the value at the given valid index.
* The access is raw;
* that is, it does not invoke metamethods.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param n the number of elements
*/
protected native void luaJ_rawgeti(long ptr, int index, int n); /*
lua_State * L = (lua_State *) ptr;
lua_rawgeti((lua_State *) L, (int) index, (int) n);
*/
/**
* Wrapper of lua_rawset
*
*
* [-2, +0, m]
*
*
*
* void lua_rawset (lua_State *L, int index);
*
*
*
* Similar to lua_settable
, but does a raw assignment
* (i.e., without metamethods).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_rawset(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_rawset((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_rawseti
*
*
* [-1, +0, m]
*
*
*
* void lua_rawseti (lua_State *L, int index, int n);
*
*
*
* Does the equivalent of t[n] = v
,
* where t
is the value at the given valid index
* and v
is the value at the top of the stack.
*
*
*
* This function pops the value from the stack.
* The assignment is raw;
* that is, it does not invoke metamethods.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param n the number of elements
*/
protected native void lua_rawseti(long ptr, int index, int n); /*
lua_State * L = (lua_State *) ptr;
lua_rawseti((lua_State *) L, (int) index, (int) n);
*/
/**
* Wrapper of lua_remove
*
*
* [-1, +0, -]
*
*
*
* void lua_remove (lua_State *L, int index);
*
*
*
* Removes the element at the given valid index,
* shifting down the elements above this index to fill the gap.
* Cannot be called with a pseudo-index,
* because a pseudo-index is not an actual stack position.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_remove(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_remove((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_replace
*
*
* [-1, +0, -]
*
*
*
* void lua_replace (lua_State *L, int index);
*
*
*
* Moves the top element into the given position (and pops it),
* without shifting any element
* (therefore replacing the value at the given position).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_replace(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_replace((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_resume
*
*
* [-?, +?, -]
*
*
*
* int lua_resume (lua_State *L, int narg);
*
*
*
* Starts and resumes a coroutine in a given thread.
*
*
*
* To start a coroutine, you first create a new thread
* (see lua_newthread
);
* then you push onto its stack the main function plus any arguments;
* then you call lua_resume
,
* with narg
being the number of arguments.
* This call returns when the coroutine suspends or finishes its execution.
* When it returns, the stack contains all values passed to lua_yield
,
* or all values returned by the body function.
* lua_resume
returns
* LUA_YIELD
if the coroutine yields,
* 0 if the coroutine finishes its execution
* without errors,
* or an error code in case of errors (see lua_pcall
).
* In case of errors,
* the stack is not unwound,
* so you can use the debug API over it.
* The error message is on the top of the stack.
* To restart a coroutine, you put on its stack only the values to
* be passed as results from yield
,
* and then call lua_resume
.
*
*
* @param ptr the lua_State*
pointer
* @param narg the number of arguments
* @return see description
*/
protected native int lua_resume(long ptr, int narg); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_resume((lua_State *) L, (int) narg);
return returnValueReceiver;
*/
/**
* Wrapper of lua_setfenv
*
*
* [-1, +0, -]
*
*
*
* int lua_setfenv (lua_State *L, int index);
*
*
*
* Pops a table from the stack and sets it as
* the new environment for the value at the given index.
* If the value at the given index is
* neither a function nor a thread nor a userdata,
* lua_setfenv
returns 0.
* Otherwise it returns 1.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_setfenv(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_setfenv((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_setfield
*
*
* [-1, +0, e]
*
*
*
* void lua_setfield (lua_State *L, int index, const char *k);
*
*
*
* Does the equivalent to t[k] = v
,
* where t
is the value at the given valid index
* and v
is the value at the top of the stack.
*
*
*
* This function pops the value from the stack.
* As in Lua, this function may trigger a metamethod
* for the "newindex" event (see §2.8).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param k the field name
*/
protected native void lua_setfield(long ptr, int index, String k); /*
lua_State * L = (lua_State *) ptr;
lua_setfield((lua_State *) L, (int) index, (const char *) k);
*/
/**
* Wrapper of lua_setglobal
*
*
* [-1, +0, e]
*
*
*
* void lua_setglobal (lua_State *L, const char *name);
*
*
*
* Pops a value from the stack and
* sets it as the new value of global name
.
* It is defined as a macro:
*
*
*
*
* #define lua_setglobal(L,s) lua_setfield(L, LUA_GLOBALSINDEX, s)
*
*
* @param ptr the lua_State*
pointer
* @param name the name
*/
protected native void lua_setglobal(long ptr, String name); /*
lua_State * L = (lua_State *) ptr;
lua_setglobal((lua_State *) L, (const char *) name);
*/
/**
* Wrapper of lua_setmetatable
*
*
* [-1, +0, -]
*
*
*
* int lua_setmetatable (lua_State *L, int index);
*
*
*
* Pops a table from the stack and
* sets it as the new metatable for the value at the given
* acceptable index.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_setmetatable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_setmetatable((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_setmetatable
*
*
* [-1, +0, -]
*
*
*
* int lua_setmetatable (lua_State *L, int index);
*
*
*
* Pops a table from the stack and
* sets it as the new metatable for the value at the given
* acceptable index.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void luaJ_setmetatable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_setmetatable((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_settable
*
*
* [-2, +0, e]
*
*
*
* void lua_settable (lua_State *L, int index);
*
*
*
* Does the equivalent to t[k] = v
,
* where t
is the value at the given valid index,
* v
is the value at the top of the stack,
* and k
is the value just below the top.
*
*
*
* This function pops both the key and the value from the stack.
* As in Lua, this function may trigger a metamethod
* for the "newindex" event (see §2.8).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_settable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_settable((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_settop
*
*
* [-?, +?, -]
*
*
*
* void lua_settop (lua_State *L, int index);
*
*
*
* Accepts any acceptable index, or 0,
* and sets the stack top to this index.
* If the new top is larger than the old one,
* then the new elements are filled with nil.
* If index
is 0, then all stack elements are removed.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_settop(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_settop((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_status
*
*
* [-0, +0, -]
*
*
*
* int lua_status (lua_State *L);
*
*
*
* Returns the status of the thread L
.
*
*
*
* The status can be 0 for a normal thread,
* an error code if the thread finished its execution with an error,
* or LUA_YIELD
if the thread is suspended.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_status(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_status((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_toboolean
*
*
* [-0, +0, -]
*
*
*
* int lua_toboolean (lua_State *L, int index);
*
*
*
* Converts the Lua value at the given acceptable index to a C boolean
* value (0 or 1).
* Like all tests in Lua,
* lua_toboolean
returns 1 for any Lua value
* different from false and nil;
* otherwise it returns 0.
* It also returns 0 when called with a non-valid index.
* (If you want to accept only actual boolean values,
* use lua_isboolean
to test the value's type.)
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_toboolean(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_toboolean((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_tointeger
*
*
* [-0, +0, -]
*
*
*
* lua_Integer lua_tointeger (lua_State *L, int index);
*
*
*
* Converts the Lua value at the given acceptable index
* to the signed integral type lua_Integer
.
* The Lua value must be a number or a string convertible to a number
* (see §2.2.1);
* otherwise, lua_tointeger
returns 0.
*
*
*
* If the number is not an integer,
* it is truncated in some non-specified way.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_tointeger(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_tointeger((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_tonumber
*
*
* [-0, +0, -]
*
*
*
* lua_Number lua_tonumber (lua_State *L, int index);
*
*
*
* Converts the Lua value at the given acceptable index
* to the C type lua_Number
(see lua_Number
).
* The Lua value must be a number or a string convertible to a number
* (see §2.2.1);
* otherwise, lua_tonumber
returns 0.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native double lua_tonumber(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jdouble returnValueReceiver = (jdouble) lua_tonumber((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_topointer
*
*
* [-0, +0, -]
*
*
*
* const void *lua_topointer (lua_State *L, int index);
*
*
*
* Converts the value at the given acceptable index to a generic
* C pointer (void*
).
* The value can be a userdata, a table, a thread, or a function;
* otherwise, lua_topointer
returns NULL
.
* Different objects will give different pointers.
* There is no way to convert the pointer back to its original value.
*
*
*
* Typically this function is used only for debug information.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native long lua_topointer(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_topointer((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_tostring
*
*
* [-0, +0, m]
*
*
*
* const char *lua_tostring (lua_State *L, int index);
*
*
*
* Equivalent to lua_tolstring
with len
equal to NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native String lua_tostring(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) lua_tostring((lua_State *) L, (int) index);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of lua_tothread
*
*
* [-0, +0, -]
*
*
*
* lua_State *lua_tothread (lua_State *L, int index);
*
*
*
* Converts the value at the given acceptable index to a Lua thread
* (represented as lua_State*
).
* This value must be a thread;
* otherwise, the function returns NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native long lua_tothread(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_tothread((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_touserdata
*
*
* [-0, +0, -]
*
*
*
* void *lua_touserdata (lua_State *L, int index);
*
*
*
* If the value at the given acceptable index is a full userdata,
* returns its block address.
* If the value is a light userdata,
* returns its pointer.
* Otherwise, returns NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native long lua_touserdata(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_touserdata((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_type
*
*
* [-0, +0, -]
*
*
*
* int lua_type (lua_State *L, int index);
*
*
*
* Returns the type of the value in the given acceptable index,
* or LUA_TNONE
for a non-valid index
* (that is, an index to an "empty" stack position).
* The types returned by lua_type
are coded by the following constants
* defined in lua.h
:
* LUA_TNIL
,
* LUA_TNUMBER
,
* LUA_TBOOLEAN
,
* LUA_TSTRING
,
* LUA_TTABLE
,
* LUA_TFUNCTION
,
* LUA_TUSERDATA
,
* LUA_TTHREAD
,
* and
* LUA_TLIGHTUSERDATA
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_type(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_type((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_typename
*
*
* [-0, +0, -]
*
*
*
* const char *lua_typename (lua_State *L, int tp);
*
*
*
* Returns the name of the type encoded by the value tp
,
* which must be one the values returned by lua_type
.
*
*
* @param ptr the lua_State*
pointer
* @param tp type id
* @return see description
*/
protected native String lua_typename(long ptr, int tp); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) lua_typename((lua_State *) L, (int) tp);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of lua_xmove
*
*
* [-?, +?, -]
*
*
*
* void lua_xmove (lua_State *from, lua_State *to, int n);
*
*
*
* Exchange values between different threads of the same global state.
*
*
*
* This function pops n
values from the stack from
,
* and pushes them onto the stack to
.
*
*
* @param from a thread
* @param to another thread
* @param n the number of elements
*/
protected native void lua_xmove(long from, long to, int n); /*
lua_xmove((lua_State *) from, (lua_State *) to, (int) n);
*/
/**
* Wrapper of lua_yield
*
*
* [-?, +?, -]
*
*
*
* int lua_yield (lua_State *L, int nresults);
*
*
*
* Yields a coroutine.
*
*
*
* This function should only be called as the
* return expression of a C function, as follows:
*
*
*
*
* return lua_yield (L, nresults);
*
*
*
* When a C function calls lua_yield
in that way,
* the running coroutine suspends its execution,
* and the call to lua_resume
that started this coroutine returns.
* The parameter nresults
is the number of values from the stack
* that are passed as results to lua_resume
.
*
*
* 3.8 – The Debug Interface
*
*
*
* Lua has no built-in debugging facilities.
* Instead, it offers a special interface
* by means of functions and hooks.
* This interface allows the construction of different
* kinds of debuggers, profilers, and other tools
* that need "inside information" from the interpreter.
*
*
* @param ptr the lua_State*
pointer
* @param nresults the number of results, or LUA_MULTRET
* @return see description
*/
protected native int lua_yield(long ptr, int nresults); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_yield((lua_State *) L, (int) nresults);
return returnValueReceiver;
*/
/**
* Wrapper of lua_gethookcount
*
*
* [-0, +0, -]
*
*
*
* int lua_gethookcount (lua_State *L);
*
*
*
* Returns the current hook count.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_gethookcount(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_gethookcount((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_gethookmask
*
*
* [-0, +0, -]
*
*
*
* int lua_gethookmask (lua_State *L);
*
*
*
* Returns the current hook mask.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_gethookmask(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_gethookmask((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_getupvalue
*
*
* [-0, +(0|1), -]
*
*
*
* const char *lua_getupvalue (lua_State *L, int funcindex, int n);
*
*
*
* Gets information about a closure's upvalue.
* (For Lua functions,
* upvalues are the external local variables that the function uses,
* and that are consequently included in its closure.)
* lua_getupvalue
gets the index n
of an upvalue,
* pushes the upvalue's value onto the stack,
* and returns its name.
* funcindex
points to the closure in the stack.
* (Upvalues have no particular order,
* as they are active through the whole function.
* So, they are numbered in an arbitrary order.)
*
*
*
* Returns NULL
(and pushes nothing)
* when the index is greater than the number of upvalues.
* For C functions, this function uses the empty string ""
* as a name for all upvalues.
*
*
* @param ptr the lua_State*
pointer
* @param funcindex the stack position of the closure
* @param n the index in the upvalue
* @return see description
*/
protected native String lua_getupvalue(long ptr, int funcindex, int n); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) lua_getupvalue((lua_State *) L, (int) funcindex, (int) n);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of lua_setupvalue
*
*
* [-(0|1), +0, -]
*
*
*
* const char *lua_setupvalue (lua_State *L, int funcindex, int n);
*
*
*
* Sets the value of a closure's upvalue.
* It assigns the value at the top of the stack
* to the upvalue and returns its name.
* It also pops the value from the stack.
* Parameters funcindex
and n
are as in the lua_getupvalue
* (see lua_getupvalue
).
*
*
*
* Returns NULL
(and pops nothing)
* when the index is greater than the number of upvalues.
*
*
* @param ptr the lua_State*
pointer
* @param funcindex the stack position of the closure
* @param n the index in the upvalue
* @return see description
*/
protected native String lua_setupvalue(long ptr, int funcindex, int n); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) lua_setupvalue((lua_State *) L, (int) funcindex, (int) n);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of luaL_callmeta
*
*
* [-0, +(0|1), e]
*
*
*
* int luaL_callmeta (lua_State *L, int obj, const char *e);
*
*
*
* Calls a metamethod.
*
*
*
* If the object at index obj
has a metatable and this
* metatable has a field e
,
* this function calls this field and passes the object as its only argument.
* In this case this function returns 1 and pushes onto the
* stack the value returned by the call.
* If there is no metatable or no metamethod,
* this function returns 0 (without pushing any value on the stack).
*
*
* @param ptr the lua_State*
pointer
* @param obj the stack position of the object
* @param e field name
* @return see description
*/
protected native int luaL_callmeta(long ptr, int obj, String e); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_callmeta((lua_State *) L, (int) obj, (const char *) e);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_dostring
*
*
* [-0, +?, m]
*
*
*
* int luaL_dostring (lua_State *L, const char *str);
*
*
*
* Loads and runs the given string.
* It is defined as the following macro:
*
*
*
*
* (luaL_loadstring(L, str) || lua_pcall(L, 0, LUA_MULTRET, 0))
*
*
*
* It returns 0 if there are no errors
* or 1 in case of errors.
*
*
* @param ptr the lua_State*
pointer
* @param str string
* @return see description
*/
protected native int luaL_dostring(long ptr, String str); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_dostring((lua_State *) L, (const char *) str);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_getmetafield
*
*
* [-0, +(0|1), m]
*
*
*
* int luaL_getmetafield (lua_State *L, int obj, const char *e);
*
*
*
* Pushes onto the stack the field e
from the metatable
* of the object at index obj
.
* If the object does not have a metatable,
* or if the metatable does not have this field,
* returns 0 and pushes nothing.
*
*
* @param ptr the lua_State*
pointer
* @param obj the stack position of the object
* @param e field name
* @return see description
*/
protected native int luaL_getmetafield(long ptr, int obj, String e); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_getmetafield((lua_State *) L, (int) obj, (const char *) e);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_getmetatable
*
*
* [-0, +1, -]
*
*
*
* void luaL_getmetatable (lua_State *L, const char *tname);
*
*
*
* Pushes onto the stack the metatable associated with name tname
* in the registry (see luaL_newmetatable
).
*
*
* @param ptr the lua_State*
pointer
* @param tname type name
*/
protected native void luaL_getmetatable(long ptr, String tname); /*
lua_State * L = (lua_State *) ptr;
luaL_getmetatable((lua_State *) L, (const char *) tname);
*/
/**
* Wrapper of luaL_getmetatable
*
*
* [-0, +1, -]
*
*
*
* void luaL_getmetatable (lua_State *L, const char *tname);
*
*
*
* Pushes onto the stack the metatable associated with name tname
* in the registry (see luaL_newmetatable
).
*
*
* @param ptr the lua_State*
pointer
* @param tname type name
*/
protected native void luaJ_getmetatable(long ptr, String tname); /*
lua_State * L = (lua_State *) ptr;
luaL_getmetatable((lua_State *) L, (const char *) tname);
*/
/**
* Wrapper of luaL_gsub
*
*
* [-0, +1, m]
*
*
*
* const char *luaL_gsub (lua_State *L,
* const char *s,
* const char *p,
* const char *r);
*
*
*
* Creates a copy of string s
by replacing
* any occurrence of the string p
* with the string r
.
* Pushes the resulting string on the stack and returns it.
*
*
* @param ptr the lua_State*
pointer
* @param s the string
* @param p the replaced sequence
* @param r the replacing string
* @return see description
*/
protected native String luaL_gsub(long ptr, String s, String p, String r); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) luaL_gsub((lua_State *) L, (const char *) s, (const char *) p, (const char *) r);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of luaL_loadstring
*
*
* [-0, +1, m]
*
*
*
* int luaL_loadstring (lua_State *L, const char *s);
*
*
*
* Loads a string as a Lua chunk.
* This function uses lua_load
to load the chunk in
* the zero-terminated string s
.
*
*
*
* This function returns the same results as lua_load
.
*
*
*
* Also as lua_load
, this function only loads the chunk;
* it does not run it.
*
*
* @param ptr the lua_State*
pointer
* @param s the string
* @return see description
*/
protected native int luaL_loadstring(long ptr, String s); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_loadstring((lua_State *) L, (const char *) s);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_newmetatable
*
*
* [-0, +1, m]
*
*
*
* int luaL_newmetatable (lua_State *L, const char *tname);
*
*
*
* If the registry already has the key tname
,
* returns 0.
* Otherwise,
* creates a new table to be used as a metatable for userdata,
* adds it to the registry with key tname
,
* and returns 1.
*
*
*
* In both cases pushes onto the stack the final value associated
* with tname
in the registry.
*
*
* @param ptr the lua_State*
pointer
* @param tname type name
* @return see description
*/
protected native int luaL_newmetatable(long ptr, String tname); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_newmetatable((lua_State *) L, (const char *) tname);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_newmetatable
*
*
* [-0, +1, m]
*
*
*
* int luaL_newmetatable (lua_State *L, const char *tname);
*
*
*
* If the registry already has the key tname
,
* returns 0.
* Otherwise,
* creates a new table to be used as a metatable for userdata,
* adds it to the registry with key tname
,
* and returns 1.
*
*
*
* In both cases pushes onto the stack the final value associated
* with tname
in the registry.
*
*
* @param ptr the lua_State*
pointer
* @param tname type name
*/
protected native void luaJ_newmetatable(long ptr, String tname); /*
lua_State * L = (lua_State *) ptr;
luaL_newmetatable((lua_State *) L, (const char *) tname);
*/
/**
* Wrapper of luaL_newstate
*
*
* [-0, +0, -]
*
*
*
* lua_State *luaL_newstate (void);
*
*
*
* Creates a new Lua state.
* It calls lua_newstate
with an
* allocator based on the standard C realloc
function
* and then sets a panic function (see lua_atpanic
) that prints
* an error message to the standard error output in case of fatal
* errors.
*
*
*
* Returns the new state,
* or NULL
if there is a memory allocation error.
*
*
* @param lid the id of the Lua state, to be used to identify between Java and Lua
* @return see description
*/
protected native long luaL_newstate(int lid); /*
lua_State* L = luaL_newstate();
luaJavaSetup(L, env, lid);
return (jlong) L;
*/
/**
* Wrapper of luaL_openlibs
*
*
* [-0, +0, m]
*
*
*
* void luaL_openlibs (lua_State *L);
*
*
*
* Opens all standard Lua libraries into the given state.
*
*
* @param ptr the lua_State*
pointer
*/
protected native void luaL_openlibs(long ptr); /*
lua_State * L = (lua_State *) ptr;
luaL_openlibs((lua_State *) L);
*/
/**
* Wrapper of luaL_ref
*
*
* [-1, +0, m]
*
*
*
* int luaL_ref (lua_State *L, int t);
*
*
*
* Creates and returns a reference,
* in the table at index t
,
* for the object at the top of the stack (and pops the object).
*
*
*
* A reference is a unique integer key.
* As long as you do not manually add integer keys into table t
,
* luaL_ref
ensures the uniqueness of the key it returns.
* You can retrieve an object referred by reference r
* by calling lua_rawgeti(L, t, r)
.
* Function luaL_unref
frees a reference and its associated object.
*
*
*
* If the object at the top of the stack is nil,
* luaL_ref
returns the constant LUA_REFNIL
.
* The constant LUA_NOREF
is guaranteed to be different
* from any reference returned by luaL_ref
.
*
*
* @param ptr the lua_State*
pointer
* @param t the stack index
* @return see description
*/
protected native int luaL_ref(long ptr, int t); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_ref((lua_State *) L, (int) t);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_typename
*
*
* [-0, +0, -]
*
*
*
* const char *luaL_typename (lua_State *L, int index);
*
*
*
* Returns the name of the type of the value at the given index.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native String luaL_typename(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) luaL_typename((lua_State *) L, (int) index);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of luaL_typerror
*
*
* [-0, +0, v]
*
*
*
* int luaL_typerror (lua_State *L, int narg, const char *tname);
*
*
*
* Generates an error with a message like the following:
*
*
*
*
* location: bad argument narg to 'func' (tname expected, got rt)
*
*
*
* where location
is produced by luaL_where
,
* func
is the name of the current function,
* and rt
is the type name of the actual argument.
*
*
* @param ptr the lua_State*
pointer
* @param narg the number of arguments
* @param tname type name
* @return see description
*/
protected native int luaL_typerror(long ptr, int narg, String tname); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_typerror((lua_State *) L, (int) narg, (const char *) tname);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_unref
*
*
* [-0, +0, -]
*
*
*
* void luaL_unref (lua_State *L, int t, int ref);
*
*
*
* Releases reference ref
from the table at index t
* (see luaL_ref
).
* The entry is removed from the table,
* so that the referred object can be collected.
* The reference ref
is also freed to be used again.
*
*
*
* If ref
is LUA_NOREF
or LUA_REFNIL
,
* luaL_unref
does nothing.
*
*
* @param ptr the lua_State*
pointer
* @param t the stack index
* @param ref the reference
*/
protected native void luaL_unref(long ptr, int t, int ref); /*
lua_State * L = (lua_State *) ptr;
luaL_unref((lua_State *) L, (int) t, (int) ref);
*/
/**
* Wrapper of luaL_where
*
*
* [-0, +1, m]
*
*
*
* void luaL_where (lua_State *L, int lvl);
*
*
*
* Pushes onto the stack a string identifying the current position
* of the control at level lvl
in the call stack.
* Typically this string has the following format:
*
*
*
*
* chunkname:currentline:
*
*
*
* Level 0 is the running function,
* level 1 is the function that called the running function,
* etc.
*
*
*
* This function is used to build a prefix for error messages.
*
*
* @param ptr the lua_State*
pointer
* @param lvl the running level
*/
protected native void luaL_where(long ptr, int lvl); /*
lua_State * L = (lua_State *) ptr;
luaL_where((lua_State *) L, (int) lvl);
*/
/**
* A wrapper function
*
*
* Open a library indivisually, alternative to luaL_openlibs
*
*
* @param ptr the lua_State*
pointer
* @param lib library name
*/
protected native void luaJ_openlib(long ptr, String lib); /*
lua_State * L = (lua_State *) ptr;
luaJ_openlib((lua_State *) L, (const char *) lib);
*/
/**
* A wrapper function
*
*
* See lua_compare
*
*
* @param ptr the lua_State*
pointer
* @param index1 the stack position of the first element
* @param index2 the stack position of the second element
* @param op the operator
* @return see description
*/
protected native int luaJ_compare(long ptr, int index1, int index2, int op); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_compare((lua_State *) L, (int) index1, (int) index2, (int) op);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Wrapper of lua_(obj)len
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int luaJ_len(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_len((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Load a direct buffer
*
*
* @param ptr the lua_State*
pointer
* @param buffer the buffer (expecting direct)
* @param size size
* @param name the name
* @return see description
*/
protected native int luaJ_loadbuffer(long ptr, Buffer buffer, int size, String name); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_loadbuffer((lua_State *) L, (unsigned char *) buffer, (int) size, (const char *) name);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Run a direct buffer
*
*
* @param ptr the lua_State*
pointer
* @param buffer the buffer (expecting direct)
* @param size size
* @param name the name
* @return see description
*/
protected native int luaJ_dobuffer(long ptr, Buffer buffer, int size, String name); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_dobuffer((lua_State *) L, (unsigned char *) buffer, (int) size, (const char *) name);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Protected call
*
*
* @param ptr the lua_State*
pointer
* @param nargs the number of arguments that you pushed onto the stack
* @param nresults the number of results, or LUA_MULTRET
* @return see description
*/
protected native int luaJ_pcall(long ptr, int nargs, int nresults); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_pcall((lua_State *) L, (int) nargs, (int) nresults);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Resume a coroutine
*
*
* @param ptr the lua_State*
pointer
* @param nargs the number of arguments that you pushed onto the stack
* @return see description
*/
protected native int luaJ_resume(long ptr, int nargs); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_resume((lua_State *) L, (int) nargs);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Push a Java object
*
*
* @param ptr the lua_State*
pointer
* @param obj the Java object
*/
protected native void luaJ_pushobject(long ptr, Object obj); /*
lua_State * L = (lua_State *) ptr;
luaJ_pushobject((JNIEnv *) env, (lua_State *) L, (jobject) obj);
*/
/**
* A wrapper function
*
*
* Push a Java class
*
*
* @param ptr the lua_State*
pointer
* @param clazz the Java class
*/
protected native void luaJ_pushclass(long ptr, Object clazz); /*
lua_State * L = (lua_State *) ptr;
luaJ_pushclass((JNIEnv *) env, (lua_State *) L, (jobject) clazz);
*/
/**
* A wrapper function
*
*
* Push a Java array
*
*
* @param ptr the lua_State*
pointer
* @param array the Java array
*/
protected native void luaJ_pusharray(long ptr, Object array); /*
lua_State * L = (lua_State *) ptr;
luaJ_pusharray((JNIEnv *) env, (lua_State *) L, (jobject) array);
*/
/**
* A wrapper function
*
*
* Push a JFunction
*
*
* @param ptr the lua_State*
pointer
* @param func the function object
*/
protected native void luaJ_pushfunction(long ptr, Object func); /*
lua_State * L = (lua_State *) ptr;
luaJ_pushfunction((JNIEnv *) env, (lua_State *) L, (jobject) func);
*/
/**
* A wrapper function
*
*
* Is a Java object (including object, array or class)
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int luaJ_isobject(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_isobject((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Convert to Java object if it is one
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native Object luaJ_toobject(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jobject returnValueReceiver = (jobject) luaJ_toobject((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Create a new thread
*
*
* @param ptr the lua_State*
pointer
* @param lid the id of the Lua state, to be used to identify between Java and Lua
* @return see description
*/
protected native long luaJ_newthread(long ptr, int lid); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) luaJ_newthread((lua_State *) L, (int) lid);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Append a searcher loading from Java side into package.searchers / loaders
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int luaJ_initloader(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_initloader((lua_State *) L);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Runs {@code CallNonvirtualMethodA}. See AbstractLua for usages.
* Parameters should be boxed and pushed on stack.
*
*
* @param ptr the lua_State*
pointer
* @param clazz the Java class
* @param method the method name
* @param sig the method signature used in {@code GetMethodID}
* @param obj the Java object
* @param params encoded parameter types
* @return see description
*/
protected native int luaJ_invokespecial(long ptr, Class clazz, String method, String sig, Object obj, String params); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_invokespecial((JNIEnv *) env, (lua_State *) L, (jclass) clazz, (const char *) method, (const char *) sig, (jobject) obj, (const char *) params);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Removes the thread from the global registry, thus allowing it to get garbage collected
*
*
* @param ptr the lua_State*
pointer
*/
protected native void luaJ_removestateindex(long ptr); /*
lua_State * L = (lua_State *) ptr;
luaJ_removestateindex((lua_State *) L);
*/
/**
* A wrapper function
*
*
* Performs a full garbage-collection cycle
*
*
* @param ptr the lua_State*
pointer
*/
protected native void luaJ_gc(long ptr); /*
lua_State * L = (lua_State *) ptr;
luaJ_gc((lua_State *) L);
*/
}