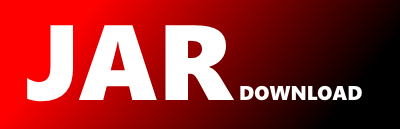
party.iroiro.luajava.Lua53Natives Maven / Gradle / Ivy
/*
* Copyright (C) 2022 the original author or authors.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package party.iroiro.luajava;
import java.util.concurrent.atomic.AtomicBoolean;
import java.nio.Buffer;
import com.badlogic.gdx.utils.SharedLibraryLoader;
/**
* Lua C API wrappers
*
*
* This file is programmatically generated from the Lua 5.3 Reference Manual.
*
*
* The following functions are excluded:
*
* luaL_addchar
* luaL_addlstring
* luaL_addsize
* luaL_addstring
* luaL_addvalue
* luaL_argcheck
* luaL_argerror
* luaL_buffinit
* luaL_buffinitsize
* luaL_checkany
* luaL_checkinteger
* luaL_checklstring
* luaL_checknumber
* luaL_checkoption
* luaL_checkstack
* luaL_checkstring
* luaL_checktype
* luaL_checkudata
* luaL_checkversion
* luaL_dofile
* luaL_error
* luaL_loadbuffer
* luaL_loadbufferx
* luaL_loadfile
* luaL_loadfilex
* luaL_newlib
* luaL_newlibtable
* luaL_opt
* luaL_optinteger
* luaL_optlstring
* luaL_optnumber
* luaL_optstring
* luaL_prepbuffer
* luaL_prepbuffsize
* luaL_pushresult
* luaL_pushresultsize
* luaL_requiref
* luaL_setfuncs
* lua_atpanic
* lua_call
* lua_callk
* lua_dump
* lua_getallocf
* lua_gethook
* lua_getinfo
* lua_getlocal
* lua_getstack
* lua_load
* lua_newstate
* lua_pcallk
* lua_pushcclosure
* lua_pushcfunction
* lua_pushfstring
* lua_pushliteral
* lua_pushlstring
* lua_pushvfstring
* lua_register
* lua_setallocf
* lua_sethook
* lua_setlocal
* lua_tocfunction
* lua_tolstring
* lua_yieldk
*
*/
@SuppressWarnings({"unused", "rawtypes"})
public class Lua53Natives extends LuaNative {
/*JNI
#include "luacustomamalg.h"
#include "lua.hpp"
#include "jni.h"
#include "jua.h"
#include "luacomp.h"
#include "juaapi.h"
#include "jualib.h"
#include "juaamalg.h"
#include "luacustom.h"
*/
private final static AtomicBoolean loaded = new AtomicBoolean(false);
protected Lua53Natives() throws IllegalStateException {
synchronized (loaded) {
if (loaded.get()) { return; }
try {
new SharedLibraryLoader().load("lua53");
if (initBindings() != 0) {
throw new RuntimeException("Unable to init bindings");
}
loaded.set(true);
} catch (Throwable e) {
throw new IllegalStateException(e);
}
}
}
private native static int initBindings() throws Exception; /*
return (jint) initLua53Bindings(env);
*/
/**
* Get LUA_REGISTRYINDEX
, which is a computed compile time constant
*/
protected native int getRegistryIndex(); /*
return LUA_REGISTRYINDEX;
*/
/**
* Wrapper of lua_absindex
*
*
* [-0, +0, –]
*
*
*
* int lua_absindex (lua_State *L, int idx);
*
*
*
* Converts the acceptable index idx
* into an equivalent absolute index
* (that is, one that does not depend on the stack top).
*
*
* @param ptr the lua_State*
pointer
* @param idx the stack position
* @return see description
*/
protected native int lua_absindex(long ptr, int idx); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_absindex((lua_State *) L, (int) idx);
return returnValueReceiver;
*/
/**
* Wrapper of lua_arith
*
*
* [-(2|1), +1, e]
*
*
*
* void lua_arith (lua_State *L, int op);
*
*
*
* Performs an arithmetic or bitwise operation over the two values
* (or one, in the case of negations)
* at the top of the stack,
* with the value at the top being the second operand,
* pops these values, and pushes the result of the operation.
* The function follows the semantics of the corresponding Lua operator
* (that is, it may call metamethods).
*
*
*
* The value of op
must be one of the following constants:
*
*
*
*
*
* -
*
LUA_OPADD
: performs addition (+
)
* -
*
LUA_OPSUB
: performs subtraction (-
)
* -
*
LUA_OPMUL
: performs multiplication (*
)
* -
*
LUA_OPDIV
: performs float division (/
)
* -
*
LUA_OPIDIV
: performs floor division (//
)
* -
*
LUA_OPMOD
: performs modulo (%
)
* -
*
LUA_OPPOW
: performs exponentiation (^
)
* -
*
LUA_OPUNM
: performs mathematical negation (unary -
)
* -
*
LUA_OPBNOT
: performs bitwise NOT (~
)
* -
*
LUA_OPBAND
: performs bitwise AND (&
)
* -
*
LUA_OPBOR
: performs bitwise OR (|
)
* -
*
LUA_OPBXOR
: performs bitwise exclusive OR (~
)
* -
*
LUA_OPSHL
: performs left shift (<<
)
* -
*
LUA_OPSHR
: performs right shift (>>
)
*
*
*
* @param ptr the lua_State*
pointer
* @param op the operator
*/
protected native void lua_arith(long ptr, int op); /*
lua_State * L = (lua_State *) ptr;
lua_arith((lua_State *) L, (int) op);
*/
/**
* Wrapper of lua_checkstack
*
*
* [-0, +0, –]
*
*
*
* int lua_checkstack (lua_State *L, int n);
*
*
*
* Ensures that the stack has space for at least n
extra slots
* (that is, that you can safely push up to n
values into it).
* It returns false if it cannot fulfill the request,
* either because it would cause the stack
* to be larger than a fixed maximum size
* (typically at least several thousand elements) or
* because it cannot allocate memory for the extra space.
* This function never shrinks the stack;
* if the stack already has space for the extra slots,
* it is left unchanged.
*
*
* @param ptr the lua_State*
pointer
* @param n the number of elements
* @return see description
*/
protected native int lua_checkstack(long ptr, int n); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_checkstack((lua_State *) L, (int) n);
return returnValueReceiver;
*/
/**
* Wrapper of lua_close
*
*
* [-0, +0, –]
*
*
*
* void lua_close (lua_State *L);
*
*
*
* Destroys all objects in the given Lua state
* (calling the corresponding garbage-collection metamethods, if any)
* and frees all dynamic memory used by this state.
* In several platforms, you may not need to call this function,
* because all resources are naturally released when the host program ends.
* On the other hand, long-running programs that create multiple states,
* such as daemons or web servers,
* will probably need to close states as soon as they are not needed.
*
*
* @param ptr the lua_State*
pointer
*/
protected native void lua_close(long ptr); /*
lua_State * L = (lua_State *) ptr;
lua_close((lua_State *) L);
*/
/**
* Wrapper of lua_compare
*
*
* [-0, +0, e]
*
*
*
* int lua_compare (lua_State *L, int index1, int index2, int op);
*
*
*
* Compares two Lua values.
* Returns 1 if the value at index index1
satisfies op
* when compared with the value at index index2
,
* following the semantics of the corresponding Lua operator
* (that is, it may call metamethods).
* Otherwise returns 0.
* Also returns 0 if any of the indices is not valid.
*
*
*
* The value of op
must be one of the following constants:
*
*
*
*
*
* -
*
LUA_OPEQ
: compares for equality (==
)
* -
*
LUA_OPLT
: compares for less than (<
)
* -
*
LUA_OPLE
: compares for less or equal (<=
)
*
*
*
* @param ptr the lua_State*
pointer
* @param index1 the stack position of the first element
* @param index2 the stack position of the second element
* @param op the operator
* @return see description
*/
protected native int lua_compare(long ptr, int index1, int index2, int op); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_compare((lua_State *) L, (int) index1, (int) index2, (int) op);
return returnValueReceiver;
*/
/**
* Wrapper of lua_concat
*
*
* [-n, +1, e]
*
*
*
* void lua_concat (lua_State *L, int n);
*
*
*
* Concatenates the n
values at the top of the stack,
* pops them, and leaves the result at the top.
* If n
is 1, the result is the single value on the stack
* (that is, the function does nothing);
* if n
is 0, the result is the empty string.
* Concatenation is performed following the usual semantics of Lua
* (see §3.4.6).
*
*
* @param ptr the lua_State*
pointer
* @param n the number of elements
*/
protected native void lua_concat(long ptr, int n); /*
lua_State * L = (lua_State *) ptr;
lua_concat((lua_State *) L, (int) n);
*/
/**
* Wrapper of lua_copy
*
*
* [-0, +0, –]
*
*
*
* void lua_copy (lua_State *L, int fromidx, int toidx);
*
*
*
* Copies the element at index fromidx
* into the valid index toidx
,
* replacing the value at that position.
* Values at other positions are not affected.
*
*
* @param ptr the lua_State*
pointer
* @param fromidx a stack position
* @param toidx another stack position
*/
protected native void lua_copy(long ptr, int fromidx, int toidx); /*
lua_State * L = (lua_State *) ptr;
lua_copy((lua_State *) L, (int) fromidx, (int) toidx);
*/
/**
* Wrapper of lua_createtable
*
*
* [-0, +1, m]
*
*
*
* void lua_createtable (lua_State *L, int narr, int nrec);
*
*
*
* Creates a new empty table and pushes it onto the stack.
* Parameter narr
is a hint for how many elements the table
* will have as a sequence;
* parameter nrec
is a hint for how many other elements
* the table will have.
* Lua may use these hints to preallocate memory for the new table.
* This preallocation is useful for performance when you know in advance
* how many elements the table will have.
* Otherwise you can use the function lua_newtable
.
*
*
* @param ptr the lua_State*
pointer
* @param narr the number of pre-allocated array elements
* @param nrec the number of pre-allocated non-array elements
*/
protected native void lua_createtable(long ptr, int narr, int nrec); /*
lua_State * L = (lua_State *) ptr;
lua_createtable((lua_State *) L, (int) narr, (int) nrec);
*/
/**
* Wrapper of lua_error
*
*
* [-1, +0, v]
*
*
*
* int lua_error (lua_State *L);
*
*
*
* Generates a Lua error,
* using the value at the top of the stack as the error object.
* This function does a long jump,
* and therefore never returns
* (see luaL_error
).
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_error(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_error((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_gc
*
*
* [-0, +0, m]
*
*
*
* int lua_gc (lua_State *L, int what, int data);
*
*
*
* Controls the garbage collector.
*
*
*
* This function performs several tasks,
* according to the value of the parameter what
:
*
*
*
*
*
* -
*
LUA_GCSTOP
:
* stops the garbage collector.
*
*
* -
*
LUA_GCRESTART
:
* restarts the garbage collector.
*
*
* -
*
LUA_GCCOLLECT
:
* performs a full garbage-collection cycle.
*
*
* -
*
LUA_GCCOUNT
:
* returns the current amount of memory (in Kbytes) in use by Lua.
*
*
* -
*
LUA_GCCOUNTB
:
* returns the remainder of dividing the current amount of bytes of
* memory in use by Lua by 1024.
*
*
* -
*
LUA_GCSTEP
:
* performs an incremental step of garbage collection.
*
*
* -
*
LUA_GCSETPAUSE
:
* sets data
as the new value
* for the pause of the collector (see §2.5)
* and returns the previous value of the pause.
*
*
* -
*
LUA_GCSETSTEPMUL
:
* sets data
as the new value for the step multiplier of
* the collector (see §2.5)
* and returns the previous value of the step multiplier.
*
*
* -
*
LUA_GCISRUNNING
:
* returns a boolean that tells whether the collector is running
* (i.e., not stopped).
*
*
*
*
*
* For more details about these options,
* see collectgarbage
.
*
*
* @param ptr the lua_State*
pointer
* @param what what
* @param data data
* @return see description
*/
protected native int lua_gc(long ptr, int what, int data); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_gc((lua_State *) L, (int) what, (int) data);
return returnValueReceiver;
*/
/**
* Wrapper of lua_getfield
*
*
* [-0, +1, e]
*
*
*
* int lua_getfield (lua_State *L, int index, const char *k);
*
*
*
* Pushes onto the stack the value t[k]
,
* where t
is the value at the given index.
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.4).
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param k the field name
* @return see description
*/
protected native int lua_getfield(long ptr, int index, String k); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_getfield((lua_State *) L, (int) index, (const char *) k);
return returnValueReceiver;
*/
/**
* Wrapper of lua_getfield
*
*
* [-0, +1, e]
*
*
*
* int lua_getfield (lua_State *L, int index, const char *k);
*
*
*
* Pushes onto the stack the value t[k]
,
* where t
is the value at the given index.
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.4).
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param k the field name
*/
protected native void luaJ_getfield(long ptr, int index, String k); /*
lua_State * L = (lua_State *) ptr;
lua_getfield((lua_State *) L, (int) index, (const char *) k);
*/
/**
* Wrapper of lua_getextraspace
*
*
* [-0, +0, –]
*
*
*
* void *lua_getextraspace (lua_State *L);
*
*
*
* Returns a pointer to a raw memory area associated with the
* given Lua state.
* The application can use this area for any purpose;
* Lua does not use it for anything.
*
*
*
* Each new thread has this area initialized with a copy
* of the area of the main thread.
*
*
*
* By default, this area has the size of a pointer to void,
* but you can recompile Lua with a different size for this area.
* (See LUA_EXTRASPACE
in luaconf.h
.)
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native long lua_getextraspace(long ptr); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_getextraspace((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_getglobal
*
*
* [-0, +1, e]
*
*
*
* int lua_getglobal (lua_State *L, const char *name);
*
*
*
* Pushes onto the stack the value of the global name
.
* Returns the type of that value.
*
*
* @param ptr the lua_State*
pointer
* @param name the name
* @return see description
*/
protected native int lua_getglobal(long ptr, String name); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_getglobal((lua_State *) L, (const char *) name);
return returnValueReceiver;
*/
/**
* Wrapper of lua_getglobal
*
*
* [-0, +1, e]
*
*
*
* int lua_getglobal (lua_State *L, const char *name);
*
*
*
* Pushes onto the stack the value of the global name
.
* Returns the type of that value.
*
*
* @param ptr the lua_State*
pointer
* @param name the name
*/
protected native void luaJ_getglobal(long ptr, String name); /*
lua_State * L = (lua_State *) ptr;
lua_getglobal((lua_State *) L, (const char *) name);
*/
/**
* Wrapper of lua_geti
*
*
* [-0, +1, e]
*
*
*
* int lua_geti (lua_State *L, int index, lua_Integer i);
*
*
*
* Pushes onto the stack the value t[i]
,
* where t
is the value at the given index.
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.4).
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param i i
* @return see description
*/
protected native int lua_geti(long ptr, int index, int i); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_geti((lua_State *) L, (int) index, (lua_Integer) i);
return returnValueReceiver;
*/
/**
* Wrapper of lua_geti
*
*
* [-0, +1, e]
*
*
*
* int lua_geti (lua_State *L, int index, lua_Integer i);
*
*
*
* Pushes onto the stack the value t[i]
,
* where t
is the value at the given index.
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.4).
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param i i
*/
protected native void luaJ_geti(long ptr, int index, int i); /*
lua_State * L = (lua_State *) ptr;
lua_geti((lua_State *) L, (int) index, (lua_Integer) i);
*/
/**
* Wrapper of lua_getmetatable
*
*
* [-0, +(0|1), –]
*
*
*
* int lua_getmetatable (lua_State *L, int index);
*
*
*
* If the value at the given index has a metatable,
* the function pushes that metatable onto the stack and returns 1.
* Otherwise,
* the function returns 0 and pushes nothing on the stack.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_getmetatable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_getmetatable((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_gettable
*
*
* [-1, +1, e]
*
*
*
* int lua_gettable (lua_State *L, int index);
*
*
*
* Pushes onto the stack the value t[k]
,
* where t
is the value at the given index
* and k
is the value at the top of the stack.
*
*
*
* This function pops the key from the stack,
* pushing the resulting value in its place.
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.4).
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_gettable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_gettable((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_gettable
*
*
* [-1, +1, e]
*
*
*
* int lua_gettable (lua_State *L, int index);
*
*
*
* Pushes onto the stack the value t[k]
,
* where t
is the value at the given index
* and k
is the value at the top of the stack.
*
*
*
* This function pops the key from the stack,
* pushing the resulting value in its place.
* As in Lua, this function may trigger a metamethod
* for the "index" event (see §2.4).
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void luaJ_gettable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_gettable((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_gettop
*
*
* [-0, +0, –]
*
*
*
* int lua_gettop (lua_State *L);
*
*
*
* Returns the index of the top element in the stack.
* Because indices start at 1,
* this result is equal to the number of elements in the stack;
* in particular, 0 means an empty stack.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_gettop(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_gettop((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_getuservalue
*
*
* [-0, +1, –]
*
*
*
* int lua_getuservalue (lua_State *L, int index);
*
*
*
* Pushes onto the stack the Lua value associated with the full userdata
* at the given index.
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_getuservalue(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_getuservalue((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_insert
*
*
* [-1, +1, –]
*
*
*
* void lua_insert (lua_State *L, int index);
*
*
*
* Moves the top element into the given valid index,
* shifting up the elements above this index to open space.
* This function cannot be called with a pseudo-index,
* because a pseudo-index is not an actual stack position.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_insert(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_insert((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_isboolean
*
*
* [-0, +0, –]
*
*
*
* int lua_isboolean (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is a boolean,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isboolean(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isboolean((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_iscfunction
*
*
* [-0, +0, –]
*
*
*
* int lua_iscfunction (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is a C function,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_iscfunction(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_iscfunction((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isfunction
*
*
* [-0, +0, –]
*
*
*
* int lua_isfunction (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is a function
* (either C or Lua), and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isfunction(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isfunction((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isinteger
*
*
* [-0, +0, –]
*
*
*
* int lua_isinteger (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is an integer
* (that is, the value is a number and is represented as an integer),
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isinteger(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isinteger((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_islightuserdata
*
*
* [-0, +0, –]
*
*
*
* int lua_islightuserdata (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is a light userdata,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_islightuserdata(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_islightuserdata((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isnil
*
*
* [-0, +0, –]
*
*
*
* int lua_isnil (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is nil,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isnil(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isnil((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isnone
*
*
* [-0, +0, –]
*
*
*
* int lua_isnone (lua_State *L, int index);
*
*
*
* Returns 1 if the given index is not valid,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isnone(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isnone((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isnoneornil
*
*
* [-0, +0, –]
*
*
*
* int lua_isnoneornil (lua_State *L, int index);
*
*
*
* Returns 1 if the given index is not valid
* or if the value at this index is nil,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isnoneornil(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isnoneornil((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isnumber
*
*
* [-0, +0, –]
*
*
*
* int lua_isnumber (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is a number
* or a string convertible to a number,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isnumber(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isnumber((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isstring
*
*
* [-0, +0, –]
*
*
*
* int lua_isstring (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is a string
* or a number (which is always convertible to a string),
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isstring(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isstring((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_istable
*
*
* [-0, +0, –]
*
*
*
* int lua_istable (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is a table,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_istable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_istable((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isthread
*
*
* [-0, +0, –]
*
*
*
* int lua_isthread (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is a thread,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isthread(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isthread((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isuserdata
*
*
* [-0, +0, –]
*
*
*
* int lua_isuserdata (lua_State *L, int index);
*
*
*
* Returns 1 if the value at the given index is a userdata
* (either full or light), and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_isuserdata(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isuserdata((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_isyieldable
*
*
* [-0, +0, –]
*
*
*
* int lua_isyieldable (lua_State *L);
*
*
*
* Returns 1 if the given coroutine can yield,
* and 0 otherwise.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_isyieldable(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_isyieldable((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_len
*
*
* [-0, +1, e]
*
*
*
* void lua_len (lua_State *L, int index);
*
*
*
* Returns the length of the value at the given index.
* It is equivalent to the '#
' operator in Lua (see §3.4.7) and
* may trigger a metamethod for the "length" event (see §2.4).
* The result is pushed on the stack.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_len(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_len((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_newtable
*
*
* [-0, +1, m]
*
*
*
* void lua_newtable (lua_State *L);
*
*
*
* Creates a new empty table and pushes it onto the stack.
* It is equivalent to lua_createtable(L, 0, 0)
.
*
*
* @param ptr the lua_State*
pointer
*/
protected native void lua_newtable(long ptr); /*
lua_State * L = (lua_State *) ptr;
lua_newtable((lua_State *) L);
*/
/**
* Wrapper of lua_newthread
*
*
* [-0, +1, m]
*
*
*
* lua_State *lua_newthread (lua_State *L);
*
*
*
* Creates a new thread, pushes it on the stack,
* and returns a pointer to a lua_State
that represents this new thread.
* The new thread returned by this function shares with the original thread
* its global environment,
* but has an independent execution stack.
*
*
*
* There is no explicit function to close or to destroy a thread.
* Threads are subject to garbage collection,
* like any Lua object.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native long lua_newthread(long ptr); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_newthread((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_newuserdata
*
*
* [-0, +1, m]
*
*
*
* void *lua_newuserdata (lua_State *L, size_t size);
*
*
*
* This function allocates a new block of memory with the given size,
* pushes onto the stack a new full userdata with the block address,
* and returns this address.
* The host program can freely use this memory.
*
*
* @param ptr the lua_State*
pointer
* @param size size
* @return see description
*/
protected native long lua_newuserdata(long ptr, int size); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_newuserdata((lua_State *) L, (size_t) size);
return returnValueReceiver;
*/
/**
* Wrapper of lua_next
*
*
* [-1, +(2|0), e]
*
*
*
* int lua_next (lua_State *L, int index);
*
*
*
* Pops a key from the stack,
* and pushes a key–value pair from the table at the given index
* (the "next" pair after the given key).
* If there are no more elements in the table,
* then lua_next
returns 0 (and pushes nothing).
*
*
*
* A typical traversal looks like this:
*
*
*
*
* /* table is in the stack at index 't' */
* lua_pushnil(L); /* first key */
* while (lua_next(L, t) != 0) {
* /* uses 'key' (at index -2) and 'value' (at index -1) */
* printf("%s - %s\n",
* lua_typename(L, lua_type(L, -2)),
* lua_typename(L, lua_type(L, -1)));
* /* removes 'value'; keeps 'key' for next iteration */
* lua_pop(L, 1);
* }
*
*
*
* While traversing a table,
* do not call lua_tolstring
directly on a key,
* unless you know that the key is actually a string.
* Recall that lua_tolstring
may change
* the value at the given index;
* this confuses the next call to lua_next
.
*
*
*
* See function next
for the caveats of modifying
* the table during its traversal.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_next(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_next((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_pcall
*
*
* [-(nargs + 1), +(nresults|1), –]
*
*
*
* int lua_pcall (lua_State *L, int nargs, int nresults, int msgh);
*
*
*
* Calls a function in protected mode.
*
*
*
* Both nargs
and nresults
have the same meaning as
* in lua_call
.
* If there are no errors during the call,
* lua_pcall
behaves exactly like lua_call
.
* However, if there is any error,
* lua_pcall
catches it,
* pushes a single value on the stack (the error object),
* and returns an error code.
* Like lua_call
,
* lua_pcall
always removes the function
* and its arguments from the stack.
*
*
*
* If msgh
is 0,
* then the error object returned on the stack
* is exactly the original error object.
* Otherwise, msgh
is the stack index of a
* message handler.
* (This index cannot be a pseudo-index.)
* In case of runtime errors,
* this function will be called with the error object
* and its return value will be the object
* returned on the stack by lua_pcall
.
*
*
*
* Typically, the message handler is used to add more debug
* information to the error object, such as a stack traceback.
* Such information cannot be gathered after the return of lua_pcall
,
* since by then the stack has unwound.
*
*
*
* The lua_pcall
function returns one of the following constants
* (defined in lua.h
):
*
*
*
*
*
* -
*
LUA_OK
(0):
* success.
*
* -
*
LUA_ERRRUN
:
* a runtime error.
*
*
* -
*
LUA_ERRMEM
:
* memory allocation error.
* For such errors, Lua does not call the message handler.
*
*
* -
*
LUA_ERRERR
:
* error while running the message handler.
*
*
* -
*
LUA_ERRGCMM
:
* error while running a __gc
metamethod.
* For such errors, Lua does not call the message handler
* (as this kind of error typically has no relation
* with the function being called).
*
*
*
*
* @param ptr the lua_State*
pointer
* @param nargs the number of arguments that you pushed onto the stack
* @param nresults the number of results, or LUA_MULTRET
* @param msgh stack position of message handler
* @return see description
*/
protected native int lua_pcall(long ptr, int nargs, int nresults, int msgh); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_pcall((lua_State *) L, (int) nargs, (int) nresults, (int) msgh);
return returnValueReceiver;
*/
/**
* Wrapper of lua_pcall
*
*
* [-(nargs + 1), +(nresults|1), –]
*
*
*
* int lua_pcall (lua_State *L, int nargs, int nresults, int msgh);
*
*
*
* Calls a function in protected mode.
*
*
*
* Both nargs
and nresults
have the same meaning as
* in lua_call
.
* If there are no errors during the call,
* lua_pcall
behaves exactly like lua_call
.
* However, if there is any error,
* lua_pcall
catches it,
* pushes a single value on the stack (the error object),
* and returns an error code.
* Like lua_call
,
* lua_pcall
always removes the function
* and its arguments from the stack.
*
*
*
* If msgh
is 0,
* then the error object returned on the stack
* is exactly the original error object.
* Otherwise, msgh
is the stack index of a
* message handler.
* (This index cannot be a pseudo-index.)
* In case of runtime errors,
* this function will be called with the error object
* and its return value will be the object
* returned on the stack by lua_pcall
.
*
*
*
* Typically, the message handler is used to add more debug
* information to the error object, such as a stack traceback.
* Such information cannot be gathered after the return of lua_pcall
,
* since by then the stack has unwound.
*
*
*
* The lua_pcall
function returns one of the following constants
* (defined in lua.h
):
*
*
*
*
*
* -
*
LUA_OK
(0):
* success.
*
* -
*
LUA_ERRRUN
:
* a runtime error.
*
*
* -
*
LUA_ERRMEM
:
* memory allocation error.
* For such errors, Lua does not call the message handler.
*
*
* -
*
LUA_ERRERR
:
* error while running the message handler.
*
*
* -
*
LUA_ERRGCMM
:
* error while running a __gc
metamethod.
* For such errors, Lua does not call the message handler
* (as this kind of error typically has no relation
* with the function being called).
*
*
*
*
* @param ptr the lua_State*
pointer
* @param nargs the number of arguments that you pushed onto the stack
* @param nresults the number of results, or LUA_MULTRET
* @param msgh stack position of message handler
*/
protected native void luaJ_pcall(long ptr, int nargs, int nresults, int msgh); /*
lua_State * L = (lua_State *) ptr;
lua_pcall((lua_State *) L, (int) nargs, (int) nresults, (int) msgh);
*/
/**
* Wrapper of lua_pop
*
*
* [-n, +0, –]
*
*
*
* void lua_pop (lua_State *L, int n);
*
*
*
* Pops n
elements from the stack.
*
*
* @param ptr the lua_State*
pointer
* @param n the number of elements
*/
protected native void lua_pop(long ptr, int n); /*
lua_State * L = (lua_State *) ptr;
lua_pop((lua_State *) L, (int) n);
*/
/**
* Wrapper of lua_pushboolean
*
*
* [-0, +1, –]
*
*
*
* void lua_pushboolean (lua_State *L, int b);
*
*
*
* Pushes a boolean value with value b
onto the stack.
*
*
* @param ptr the lua_State*
pointer
* @param b boolean
*/
protected native void lua_pushboolean(long ptr, int b); /*
lua_State * L = (lua_State *) ptr;
lua_pushboolean((lua_State *) L, (int) b);
*/
/**
* Wrapper of lua_pushglobaltable
*
*
* [-0, +1, –]
*
*
*
* void lua_pushglobaltable (lua_State *L);
*
*
*
* Pushes the global environment onto the stack.
*
*
* @param ptr the lua_State*
pointer
*/
protected native void lua_pushglobaltable(long ptr); /*
lua_State * L = (lua_State *) ptr;
lua_pushglobaltable((lua_State *) L);
*/
/**
* Wrapper of lua_pushinteger
*
*
* [-0, +1, –]
*
*
*
* void lua_pushinteger (lua_State *L, lua_Integer n);
*
*
*
* Pushes an integer with value n
onto the stack.
*
*
* @param ptr the lua_State*
pointer
* @param n the number of elements
*/
protected native void lua_pushinteger(long ptr, int n); /*
lua_State * L = (lua_State *) ptr;
lua_pushinteger((lua_State *) L, (lua_Integer) n);
*/
/**
* Wrapper of lua_pushlightuserdata
*
*
* [-0, +1, –]
*
*
*
* void lua_pushlightuserdata (lua_State *L, void *p);
*
*
*
* Pushes a light userdata onto the stack.
*
*
*
* Userdata represent C values in Lua.
* A light userdata represents a pointer, a void*
.
* It is a value (like a number):
* you do not create it, it has no individual metatable,
* and it is not collected (as it was never created).
* A light userdata is equal to "any"
* light userdata with the same C address.
*
*
* @param ptr the lua_State*
pointer
* @param p the pointer
*/
protected native void lua_pushlightuserdata(long ptr, long p); /*
lua_State * L = (lua_State *) ptr;
lua_pushlightuserdata((lua_State *) L, (void *) p);
*/
/**
* Wrapper of lua_pushnil
*
*
* [-0, +1, –]
*
*
*
* void lua_pushnil (lua_State *L);
*
*
*
* Pushes a nil value onto the stack.
*
*
* @param ptr the lua_State*
pointer
*/
protected native void lua_pushnil(long ptr); /*
lua_State * L = (lua_State *) ptr;
lua_pushnil((lua_State *) L);
*/
/**
* Wrapper of lua_pushnumber
*
*
* [-0, +1, –]
*
*
*
* void lua_pushnumber (lua_State *L, lua_Number n);
*
*
*
* Pushes a float with value n
onto the stack.
*
*
* @param ptr the lua_State*
pointer
* @param n the number of elements
*/
protected native void lua_pushnumber(long ptr, double n); /*
lua_State * L = (lua_State *) ptr;
lua_pushnumber((lua_State *) L, (lua_Number) n);
*/
/**
* Wrapper of lua_pushstring
*
*
* [-0, +1, m]
*
*
*
* const char *lua_pushstring (lua_State *L, const char *s);
*
*
*
* Pushes the zero-terminated string pointed to by s
* onto the stack.
* Lua makes (or reuses) an internal copy of the given string,
* so the memory at s
can be freed or reused immediately after
* the function returns.
*
*
*
* Returns a pointer to the internal copy of the string.
*
*
*
* If s
is NULL
, pushes nil and returns NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param s the string
* @return see description
*/
protected native String lua_pushstring(long ptr, String s); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) lua_pushstring((lua_State *) L, (const char *) s);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of lua_pushstring
*
*
* [-0, +1, m]
*
*
*
* const char *lua_pushstring (lua_State *L, const char *s);
*
*
*
* Pushes the zero-terminated string pointed to by s
* onto the stack.
* Lua makes (or reuses) an internal copy of the given string,
* so the memory at s
can be freed or reused immediately after
* the function returns.
*
*
*
* Returns a pointer to the internal copy of the string.
*
*
*
* If s
is NULL
, pushes nil and returns NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param s the string
*/
protected native void luaJ_pushstring(long ptr, String s); /*
lua_State * L = (lua_State *) ptr;
lua_pushstring((lua_State *) L, (const char *) s);
*/
/**
* Wrapper of lua_pushthread
*
*
* [-0, +1, –]
*
*
*
* int lua_pushthread (lua_State *L);
*
*
*
* Pushes the thread represented by L
onto the stack.
* Returns 1 if this thread is the main thread of its state.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_pushthread(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_pushthread((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_pushvalue
*
*
* [-0, +1, –]
*
*
*
* void lua_pushvalue (lua_State *L, int index);
*
*
*
* Pushes a copy of the element at the given index
* onto the stack.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_pushvalue(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_pushvalue((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_rawequal
*
*
* [-0, +0, –]
*
*
*
* int lua_rawequal (lua_State *L, int index1, int index2);
*
*
*
* Returns 1 if the two values in indices index1
and
* index2
are primitively equal
* (that is, without calling the __eq
metamethod).
* Otherwise returns 0.
* Also returns 0 if any of the indices are not valid.
*
*
* @param ptr the lua_State*
pointer
* @param index1 the stack position of the first element
* @param index2 the stack position of the second element
* @return see description
*/
protected native int lua_rawequal(long ptr, int index1, int index2); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_rawequal((lua_State *) L, (int) index1, (int) index2);
return returnValueReceiver;
*/
/**
* Wrapper of lua_rawget
*
*
* [-1, +1, –]
*
*
*
* int lua_rawget (lua_State *L, int index);
*
*
*
* Similar to lua_gettable
, but does a raw access
* (i.e., without metamethods).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_rawget(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_rawget((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_rawget
*
*
* [-1, +1, –]
*
*
*
* int lua_rawget (lua_State *L, int index);
*
*
*
* Similar to lua_gettable
, but does a raw access
* (i.e., without metamethods).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void luaJ_rawget(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_rawget((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_rawgeti
*
*
* [-0, +1, –]
*
*
*
* int lua_rawgeti (lua_State *L, int index, lua_Integer n);
*
*
*
* Pushes onto the stack the value t[n]
,
* where t
is the table at the given index.
* The access is raw,
* that is, it does not invoke the __index
metamethod.
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param n the number of elements
* @return see description
*/
protected native int lua_rawgeti(long ptr, int index, int n); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_rawgeti((lua_State *) L, (int) index, (lua_Integer) n);
return returnValueReceiver;
*/
/**
* Wrapper of lua_rawgeti
*
*
* [-0, +1, –]
*
*
*
* int lua_rawgeti (lua_State *L, int index, lua_Integer n);
*
*
*
* Pushes onto the stack the value t[n]
,
* where t
is the table at the given index.
* The access is raw,
* that is, it does not invoke the __index
metamethod.
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param n the number of elements
*/
protected native void luaJ_rawgeti(long ptr, int index, int n); /*
lua_State * L = (lua_State *) ptr;
lua_rawgeti((lua_State *) L, (int) index, (lua_Integer) n);
*/
/**
* Wrapper of lua_rawgetp
*
*
* [-0, +1, –]
*
*
*
* int lua_rawgetp (lua_State *L, int index, const void *p);
*
*
*
* Pushes onto the stack the value t[k]
,
* where t
is the table at the given index and
* k
is the pointer p
represented as a light userdata.
* The access is raw;
* that is, it does not invoke the __index
metamethod.
*
*
*
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param p the lightuserdata
* @return see description
*/
protected native int lua_rawgetp(long ptr, int index, long p); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_rawgetp((lua_State *) L, (int) index, (const void *) p);
return returnValueReceiver;
*/
/**
* Wrapper of lua_rawlen
*
*
* [-0, +0, –]
*
*
*
* size_t lua_rawlen (lua_State *L, int index);
*
*
*
* Returns the raw "length" of the value at the given index:
* for strings, this is the string length;
* for tables, this is the result of the length operator ('#
')
* with no metamethods;
* for userdata, this is the size of the block of memory allocated
* for the userdata;
* for other values, it is 0.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_rawlen(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_rawlen((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_rawset
*
*
* [-2, +0, m]
*
*
*
* void lua_rawset (lua_State *L, int index);
*
*
*
* Similar to lua_settable
, but does a raw assignment
* (i.e., without metamethods).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_rawset(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_rawset((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_rawseti
*
*
* [-1, +0, m]
*
*
*
* void lua_rawseti (lua_State *L, int index, lua_Integer i);
*
*
*
* Does the equivalent of t[i] = v
,
* where t
is the table at the given index
* and v
is the value at the top of the stack.
*
*
*
* This function pops the value from the stack.
* The assignment is raw,
* that is, it does not invoke the __newindex
metamethod.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param i i
*/
protected native void lua_rawseti(long ptr, int index, int i); /*
lua_State * L = (lua_State *) ptr;
lua_rawseti((lua_State *) L, (int) index, (lua_Integer) i);
*/
/**
* Wrapper of lua_rawsetp
*
*
* [-1, +0, m]
*
*
*
* void lua_rawsetp (lua_State *L, int index, const void *p);
*
*
*
* Does the equivalent of t[p] = v
,
* where t
is the table at the given index,
* p
is encoded as a light userdata,
* and v
is the value at the top of the stack.
*
*
*
* This function pops the value from the stack.
* The assignment is raw,
* that is, it does not invoke __newindex
metamethod.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param p the lightuserdata
*/
protected native void lua_rawsetp(long ptr, int index, long p); /*
lua_State * L = (lua_State *) ptr;
lua_rawsetp((lua_State *) L, (int) index, (const void *) p);
*/
/**
* Wrapper of lua_remove
*
*
* [-1, +0, –]
*
*
*
* void lua_remove (lua_State *L, int index);
*
*
*
* Removes the element at the given valid index,
* shifting down the elements above this index to fill the gap.
* This function cannot be called with a pseudo-index,
* because a pseudo-index is not an actual stack position.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_remove(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_remove((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_replace
*
*
* [-1, +0, –]
*
*
*
* void lua_replace (lua_State *L, int index);
*
*
*
* Moves the top element into the given valid index
* without shifting any element
* (therefore replacing the value at that given index),
* and then pops the top element.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_replace(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_replace((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_resume
*
*
* [-?, +?, –]
*
*
*
* int lua_resume (lua_State *L, lua_State *from, int nargs);
*
*
*
* Starts and resumes a coroutine in the given thread L
.
*
*
*
* To start a coroutine,
* you push onto the thread stack the main function plus any arguments;
* then you call lua_resume
,
* with nargs
being the number of arguments.
* This call returns when the coroutine suspends or finishes its execution.
* When it returns, the stack contains all values passed to lua_yield
,
* or all values returned by the body function.
* lua_resume
returns
* LUA_YIELD
if the coroutine yields,
* LUA_OK
if the coroutine finishes its execution
* without errors,
* or an error code in case of errors (see lua_pcall
).
*
*
*
* In case of errors,
* the stack is not unwound,
* so you can use the debug API over it.
* The error object is on the top of the stack.
*
*
*
* To resume a coroutine,
* you remove any results from the last lua_yield
,
* put on its stack only the values to
* be passed as results from yield
,
* and then call lua_resume
.
*
*
*
* The parameter from
represents the coroutine that is resuming L
.
* If there is no such coroutine,
* this parameter can be NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param from a thread
* @param nargs the number of arguments that you pushed onto the stack
* @return see description
*/
protected native int lua_resume(long ptr, long from, int nargs); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_resume((lua_State *) L, (lua_State *) from, (int) nargs);
return returnValueReceiver;
*/
/**
* Wrapper of lua_rotate
*
*
* [-0, +0, –]
*
*
*
* void lua_rotate (lua_State *L, int idx, int n);
*
*
*
* Rotates the stack elements between the valid index idx
* and the top of the stack.
* The elements are rotated n
positions in the direction of the top,
* for a positive n
,
* or -n
positions in the direction of the bottom,
* for a negative n
.
* The absolute value of n
must not be greater than the size
* of the slice being rotated.
* This function cannot be called with a pseudo-index,
* because a pseudo-index is not an actual stack position.
*
*
* @param ptr the lua_State*
pointer
* @param idx the stack position
* @param n the number of elements
*/
protected native void lua_rotate(long ptr, int idx, int n); /*
lua_State * L = (lua_State *) ptr;
lua_rotate((lua_State *) L, (int) idx, (int) n);
*/
/**
* Wrapper of lua_setfield
*
*
* [-1, +0, e]
*
*
*
* void lua_setfield (lua_State *L, int index, const char *k);
*
*
*
* Does the equivalent to t[k] = v
,
* where t
is the value at the given index
* and v
is the value at the top of the stack.
*
*
*
* This function pops the value from the stack.
* As in Lua, this function may trigger a metamethod
* for the "newindex" event (see §2.4).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param k the field name
*/
protected native void lua_setfield(long ptr, int index, String k); /*
lua_State * L = (lua_State *) ptr;
lua_setfield((lua_State *) L, (int) index, (const char *) k);
*/
/**
* Wrapper of lua_setglobal
*
*
* [-1, +0, e]
*
*
*
* void lua_setglobal (lua_State *L, const char *name);
*
*
*
* Pops a value from the stack and
* sets it as the new value of global name
.
*
*
* @param ptr the lua_State*
pointer
* @param name the name
*/
protected native void lua_setglobal(long ptr, String name); /*
lua_State * L = (lua_State *) ptr;
lua_setglobal((lua_State *) L, (const char *) name);
*/
/**
* Wrapper of lua_seti
*
*
* [-1, +0, e]
*
*
*
* void lua_seti (lua_State *L, int index, lua_Integer n);
*
*
*
* Does the equivalent to t[n] = v
,
* where t
is the value at the given index
* and v
is the value at the top of the stack.
*
*
*
* This function pops the value from the stack.
* As in Lua, this function may trigger a metamethod
* for the "newindex" event (see §2.4).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param n the number of elements
*/
protected native void lua_seti(long ptr, int index, int n); /*
lua_State * L = (lua_State *) ptr;
lua_seti((lua_State *) L, (int) index, (lua_Integer) n);
*/
/**
* Wrapper of lua_setmetatable
*
*
* [-1, +0, –]
*
*
*
* void lua_setmetatable (lua_State *L, int index);
*
*
*
* Pops a table from the stack and
* sets it as the new metatable for the value at the given index.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_setmetatable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_setmetatable((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_setmetatable
*
*
* [-1, +0, –]
*
*
*
* void lua_setmetatable (lua_State *L, int index);
*
*
*
* Pops a table from the stack and
* sets it as the new metatable for the value at the given index.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void luaJ_setmetatable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_setmetatable((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_settable
*
*
* [-2, +0, e]
*
*
*
* void lua_settable (lua_State *L, int index);
*
*
*
* Does the equivalent to t[k] = v
,
* where t
is the value at the given index,
* v
is the value at the top of the stack,
* and k
is the value just below the top.
*
*
*
* This function pops both the key and the value from the stack.
* As in Lua, this function may trigger a metamethod
* for the "newindex" event (see §2.4).
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_settable(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_settable((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_settop
*
*
* [-?, +?, –]
*
*
*
* void lua_settop (lua_State *L, int index);
*
*
*
* Accepts any index, or 0,
* and sets the stack top to this index.
* If the new top is larger than the old one,
* then the new elements are filled with nil.
* If index
is 0, then all stack elements are removed.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_settop(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_settop((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_setuservalue
*
*
* [-1, +0, –]
*
*
*
* void lua_setuservalue (lua_State *L, int index);
*
*
*
* Pops a value from the stack and sets it as
* the new value associated to the full userdata at the given index.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
*/
protected native void lua_setuservalue(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
lua_setuservalue((lua_State *) L, (int) index);
*/
/**
* Wrapper of lua_status
*
*
* [-0, +0, –]
*
*
*
* int lua_status (lua_State *L);
*
*
*
* Returns the status of the thread L
.
*
*
*
* The status can be 0 (LUA_OK
) for a normal thread,
* an error code if the thread finished the execution
* of a lua_resume
with an error,
* or LUA_YIELD
if the thread is suspended.
*
*
*
* You can only call functions in threads with status LUA_OK
.
* You can resume threads with status LUA_OK
* (to start a new coroutine) or LUA_YIELD
* (to resume a coroutine).
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_status(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_status((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_stringtonumber
*
*
* [-0, +1, –]
*
*
*
* size_t lua_stringtonumber (lua_State *L, const char *s);
*
*
*
* Converts the zero-terminated string s
to a number,
* pushes that number into the stack,
* and returns the total size of the string,
* that is, its length plus one.
* The conversion can result in an integer or a float,
* according to the lexical conventions of Lua (see §3.1).
* The string may have leading and trailing spaces and a sign.
* If the string is not a valid numeral,
* returns 0 and pushes nothing.
* (Note that the result can be used as a boolean,
* true if the conversion succeeds.)
*
*
* @param ptr the lua_State*
pointer
* @param s the string
* @return see description
*/
protected native int lua_stringtonumber(long ptr, String s); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_stringtonumber((lua_State *) L, (const char *) s);
return returnValueReceiver;
*/
/**
* Wrapper of lua_toboolean
*
*
* [-0, +0, –]
*
*
*
* int lua_toboolean (lua_State *L, int index);
*
*
*
* Converts the Lua value at the given index to a C boolean
* value (0 or 1).
* Like all tests in Lua,
* lua_toboolean
returns true for any Lua value
* different from false and nil;
* otherwise it returns false.
* (If you want to accept only actual boolean values,
* use lua_isboolean
to test the value's type.)
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_toboolean(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_toboolean((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_tointeger
*
*
* [-0, +0, –]
*
*
*
* lua_Integer lua_tointeger (lua_State *L, int index);
*
*
*
* Equivalent to lua_tointegerx
with isnum
equal to NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_tointeger(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_tointeger((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_tointegerx
*
*
* [-0, +0, –]
*
*
*
* lua_Integer lua_tointegerx (lua_State *L, int index, int *isnum);
*
*
*
* Converts the Lua value at the given index
* to the signed integral type lua_Integer
.
* The Lua value must be an integer,
* or a number or string convertible to an integer (see §3.4.3);
* otherwise, lua_tointegerx
returns 0.
*
*
*
* If isnum
is not NULL
,
* its referent is assigned a boolean value that
* indicates whether the operation succeeded.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param isnum pointer to a boolean to be assigned
* @return see description
*/
protected native int lua_tointegerx(long ptr, int index, long isnum); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_tointegerx((lua_State *) L, (int) index, (int *) isnum);
return returnValueReceiver;
*/
/**
* Wrapper of lua_tonumber
*
*
* [-0, +0, –]
*
*
*
* lua_Number lua_tonumber (lua_State *L, int index);
*
*
*
* Equivalent to lua_tonumberx
with isnum
equal to NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native double lua_tonumber(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jdouble returnValueReceiver = (jdouble) lua_tonumber((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_tonumberx
*
*
* [-0, +0, –]
*
*
*
* lua_Number lua_tonumberx (lua_State *L, int index, int *isnum);
*
*
*
* Converts the Lua value at the given index
* to the C type lua_Number
(see lua_Number
).
* The Lua value must be a number or a string convertible to a number
* (see §3.4.3);
* otherwise, lua_tonumberx
returns 0.
*
*
*
* If isnum
is not NULL
,
* its referent is assigned a boolean value that
* indicates whether the operation succeeded.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @param isnum pointer to a boolean to be assigned
* @return see description
*/
protected native double lua_tonumberx(long ptr, int index, long isnum); /*
lua_State * L = (lua_State *) ptr;
jdouble returnValueReceiver = (jdouble) lua_tonumberx((lua_State *) L, (int) index, (int *) isnum);
return returnValueReceiver;
*/
/**
* Wrapper of lua_topointer
*
*
* [-0, +0, –]
*
*
*
* const void *lua_topointer (lua_State *L, int index);
*
*
*
* Converts the value at the given index to a generic
* C pointer (void*
).
* The value can be a userdata, a table, a thread, or a function;
* otherwise, lua_topointer
returns NULL
.
* Different objects will give different pointers.
* There is no way to convert the pointer back to its original value.
*
*
*
* Typically this function is used only for hashing and debug information.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native long lua_topointer(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_topointer((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_tostring
*
*
* [-0, +0, m]
*
*
*
* const char *lua_tostring (lua_State *L, int index);
*
*
*
* Equivalent to lua_tolstring
with len
equal to NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native String lua_tostring(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) lua_tostring((lua_State *) L, (int) index);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of lua_tothread
*
*
* [-0, +0, –]
*
*
*
* lua_State *lua_tothread (lua_State *L, int index);
*
*
*
* Converts the value at the given index to a Lua thread
* (represented as lua_State*
).
* This value must be a thread;
* otherwise, the function returns NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native long lua_tothread(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_tothread((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_touserdata
*
*
* [-0, +0, –]
*
*
*
* void *lua_touserdata (lua_State *L, int index);
*
*
*
* If the value at the given index is a full userdata,
* returns its block address.
* If the value is a light userdata,
* returns its pointer.
* Otherwise, returns NULL
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native long lua_touserdata(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_touserdata((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_type
*
*
* [-0, +0, –]
*
*
*
* int lua_type (lua_State *L, int index);
*
*
*
* Returns the type of the value in the given valid index,
* or LUA_TNONE
for a non-valid (but acceptable) index.
* The types returned by lua_type
are coded by the following constants
* defined in lua.h
:
* LUA_TNIL
(0),
* LUA_TNUMBER
,
* LUA_TBOOLEAN
,
* LUA_TSTRING
,
* LUA_TTABLE
,
* LUA_TFUNCTION
,
* LUA_TUSERDATA
,
* LUA_TTHREAD
,
* and
* LUA_TLIGHTUSERDATA
.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int lua_type(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_type((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of lua_typename
*
*
* [-0, +0, –]
*
*
*
* const char *lua_typename (lua_State *L, int tp);
*
*
*
* Returns the name of the type encoded by the value tp
,
* which must be one the values returned by lua_type
.
*
*
* @param ptr the lua_State*
pointer
* @param tp type id
* @return see description
*/
protected native String lua_typename(long ptr, int tp); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) lua_typename((lua_State *) L, (int) tp);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of lua_upvalueindex
*
*
* [-0, +0, –]
*
*
*
* int lua_upvalueindex (int i);
*
*
*
* Returns the pseudo-index that represents the i
-th upvalue of
* the running function (see §4.4).
*
*
* @param i i
* @return see description
*/
protected native int lua_upvalueindex(int i); /*
jint returnValueReceiver = (jint) lua_upvalueindex((int) i);
return returnValueReceiver;
*/
/**
* Wrapper of lua_version
*
*
* [-0, +0, –]
*
*
*
* const lua_Number *lua_version (lua_State *L);
*
*
*
* Returns the address of the version number
* (a C static variable)
* stored in the Lua core.
* When called with a valid lua_State
,
* returns the address of the version used to create that state.
* When called with NULL
,
* returns the address of the version running the call.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native long lua_version(long ptr); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_version((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_xmove
*
*
* [-?, +?, –]
*
*
*
* void lua_xmove (lua_State *from, lua_State *to, int n);
*
*
*
* Exchange values between different threads of the same state.
*
*
*
* This function pops n
values from the stack from
,
* and pushes them onto the stack to
.
*
*
* @param from a thread
* @param to another thread
* @param n the number of elements
*/
protected native void lua_xmove(long from, long to, int n); /*
lua_xmove((lua_State *) from, (lua_State *) to, (int) n);
*/
/**
* Wrapper of lua_yield
*
*
* [-?, +?, e]
*
*
*
* int lua_yield (lua_State *L, int nresults);
*
*
*
* This function is equivalent to lua_yieldk
,
* but it has no continuation (see §4.7).
* Therefore, when the thread resumes,
* it continues the function that called
* the function calling lua_yield
.
*
*
* @param ptr the lua_State*
pointer
* @param nresults the number of results, or LUA_MULTRET
* @return see description
*/
protected native int lua_yield(long ptr, int nresults); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_yield((lua_State *) L, (int) nresults);
return returnValueReceiver;
*/
/**
* Wrapper of lua_gethookcount
*
*
* [-0, +0, –]
*
*
*
* int lua_gethookcount (lua_State *L);
*
*
*
* Returns the current hook count.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_gethookcount(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_gethookcount((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_gethookmask
*
*
* [-0, +0, –]
*
*
*
* int lua_gethookmask (lua_State *L);
*
*
*
* Returns the current hook mask.
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int lua_gethookmask(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) lua_gethookmask((lua_State *) L);
return returnValueReceiver;
*/
/**
* Wrapper of lua_getupvalue
*
*
* [-0, +(0|1), –]
*
*
*
* const char *lua_getupvalue (lua_State *L, int funcindex, int n);
*
*
*
* Gets information about the n
-th upvalue
* of the closure at index funcindex
.
* It pushes the upvalue's value onto the stack
* and returns its name.
* Returns NULL
(and pushes nothing)
* when the index n
is greater than the number of upvalues.
*
*
*
* For C functions, this function uses the empty string ""
* as a name for all upvalues.
* (For Lua functions,
* upvalues are the external local variables that the function uses,
* and that are consequently included in its closure.)
*
*
*
* Upvalues have no particular order,
* as they are active through the whole function.
* They are numbered in an arbitrary order.
*
*
* @param ptr the lua_State*
pointer
* @param funcindex the stack position of the closure
* @param n the index in the upvalue
* @return see description
*/
protected native String lua_getupvalue(long ptr, int funcindex, int n); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) lua_getupvalue((lua_State *) L, (int) funcindex, (int) n);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of lua_setupvalue
*
*
* [-(0|1), +0, –]
*
*
*
* const char *lua_setupvalue (lua_State *L, int funcindex, int n);
*
*
*
* Sets the value of a closure's upvalue.
* It assigns the value at the top of the stack
* to the upvalue and returns its name.
* It also pops the value from the stack.
*
*
*
* Returns NULL
(and pops nothing)
* when the index n
is greater than the number of upvalues.
*
*
*
* Parameters funcindex
and n
are as in function lua_getupvalue
.
*
*
* @param ptr the lua_State*
pointer
* @param funcindex the stack position of the closure
* @param n the index in the upvalue
* @return see description
*/
protected native String lua_setupvalue(long ptr, int funcindex, int n); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) lua_setupvalue((lua_State *) L, (int) funcindex, (int) n);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of lua_upvalueid
*
*
* [-0, +0, –]
*
*
*
* void *lua_upvalueid (lua_State *L, int funcindex, int n);
*
*
*
* Returns a unique identifier for the upvalue numbered n
* from the closure at index funcindex
.
*
*
*
* These unique identifiers allow a program to check whether different
* closures share upvalues.
* Lua closures that share an upvalue
* (that is, that access a same external local variable)
* will return identical ids for those upvalue indices.
*
*
*
* Parameters funcindex
and n
are as in function lua_getupvalue
,
* but n
cannot be greater than the number of upvalues.
*
*
* @param ptr the lua_State*
pointer
* @param funcindex the stack position of the closure
* @param n the index in the upvalue
* @return see description
*/
protected native long lua_upvalueid(long ptr, int funcindex, int n); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) lua_upvalueid((lua_State *) L, (int) funcindex, (int) n);
return returnValueReceiver;
*/
/**
* Wrapper of lua_upvaluejoin
*
*
* [-0, +0, –]
*
*
*
* void lua_upvaluejoin (lua_State *L, int funcindex1, int n1,
* int funcindex2, int n2);
*
*
*
* Make the n1
-th upvalue of the Lua closure at index funcindex1
* refer to the n2
-th upvalue of the Lua closure at index funcindex2
.
*
*
* @param ptr the lua_State*
pointer
* @param funcindex1 the stack position of the closure
* @param n1 n1
* @param funcindex2 the stack position of the closure
* @param n2 n2
*/
protected native void lua_upvaluejoin(long ptr, int funcindex1, int n1, int funcindex2, int n2); /*
lua_State * L = (lua_State *) ptr;
lua_upvaluejoin((lua_State *) L, (int) funcindex1, (int) n1, (int) funcindex2, (int) n2);
*/
/**
* Wrapper of luaL_callmeta
*
*
* [-0, +(0|1), e]
*
*
*
* int luaL_callmeta (lua_State *L, int obj, const char *e);
*
*
*
* Calls a metamethod.
*
*
*
* If the object at index obj
has a metatable and this
* metatable has a field e
,
* this function calls this field passing the object as its only argument.
* In this case this function returns true and pushes onto the
* stack the value returned by the call.
* If there is no metatable or no metamethod,
* this function returns false (without pushing any value on the stack).
*
*
* @param ptr the lua_State*
pointer
* @param obj the stack position of the object
* @param e field name
* @return see description
*/
protected native int luaL_callmeta(long ptr, int obj, String e); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_callmeta((lua_State *) L, (int) obj, (const char *) e);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_dostring
*
*
* [-0, +?, –]
*
*
*
* int luaL_dostring (lua_State *L, const char *str);
*
*
*
* Loads and runs the given string.
* It is defined as the following macro:
*
*
*
*
* (luaL_loadstring(L, str) || lua_pcall(L, 0, LUA_MULTRET, 0))
*
*
*
* It returns false if there are no errors
* or true in case of errors.
*
*
* @param ptr the lua_State*
pointer
* @param str string
* @return see description
*/
protected native int luaL_dostring(long ptr, String str); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_dostring((lua_State *) L, (const char *) str);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_execresult
*
*
* [-0, +3, m]
*
*
*
* int luaL_execresult (lua_State *L, int stat);
*
*
*
* This function produces the return values for
* process-related functions in the standard library
* (os.execute
and io.close
).
*
*
* @param ptr the lua_State*
pointer
* @param stat (I have no idea)
* @return see description
*/
protected native int luaL_execresult(long ptr, int stat); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_execresult((lua_State *) L, (int) stat);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_fileresult
*
*
* [-0, +(1|3), m]
*
*
*
* int luaL_fileresult (lua_State *L, int stat, const char *fname);
*
*
*
* This function produces the return values for
* file-related functions in the standard library
* (io.open
, os.rename
, file:seek
, etc.).
*
*
* @param ptr the lua_State*
pointer
* @param stat (I have no idea)
* @param fname the filename
* @return see description
*/
protected native int luaL_fileresult(long ptr, int stat, String fname); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_fileresult((lua_State *) L, (int) stat, (const char *) fname);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_getmetafield
*
*
* [-0, +(0|1), m]
*
*
*
* int luaL_getmetafield (lua_State *L, int obj, const char *e);
*
*
*
* Pushes onto the stack the field e
from the metatable
* of the object at index obj
and returns the type of the pushed value.
* If the object does not have a metatable,
* or if the metatable does not have this field,
* pushes nothing and returns LUA_TNIL
.
*
*
* @param ptr the lua_State*
pointer
* @param obj the stack position of the object
* @param e field name
* @return see description
*/
protected native int luaL_getmetafield(long ptr, int obj, String e); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_getmetafield((lua_State *) L, (int) obj, (const char *) e);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_getmetatable
*
*
* [-0, +1, m]
*
*
*
* int luaL_getmetatable (lua_State *L, const char *tname);
*
*
*
* Pushes onto the stack the metatable associated with name tname
* in the registry (see luaL_newmetatable
)
* (nil if there is no metatable associated with that name).
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param tname type name
* @return see description
*/
protected native int luaL_getmetatable(long ptr, String tname); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_getmetatable((lua_State *) L, (const char *) tname);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_getmetatable
*
*
* [-0, +1, m]
*
*
*
* int luaL_getmetatable (lua_State *L, const char *tname);
*
*
*
* Pushes onto the stack the metatable associated with name tname
* in the registry (see luaL_newmetatable
)
* (nil if there is no metatable associated with that name).
* Returns the type of the pushed value.
*
*
* @param ptr the lua_State*
pointer
* @param tname type name
*/
protected native void luaJ_getmetatable(long ptr, String tname); /*
lua_State * L = (lua_State *) ptr;
luaL_getmetatable((lua_State *) L, (const char *) tname);
*/
/**
* Wrapper of luaL_getsubtable
*
*
* [-0, +1, e]
*
*
*
* int luaL_getsubtable (lua_State *L, int idx, const char *fname);
*
*
*
* Ensures that the value t[fname]
,
* where t
is the value at index idx
,
* is a table,
* and pushes that table onto the stack.
* Returns true if it finds a previous table there
* and false if it creates a new table.
*
*
* @param ptr the lua_State*
pointer
* @param idx the stack position
* @param fname the filename
* @return see description
*/
protected native int luaL_getsubtable(long ptr, int idx, String fname); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_getsubtable((lua_State *) L, (int) idx, (const char *) fname);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_gsub
*
*
* [-0, +1, m]
*
*
*
* const char *luaL_gsub (lua_State *L,
* const char *s,
* const char *p,
* const char *r);
*
*
*
* Creates a copy of string s
by replacing
* any occurrence of the string p
* with the string r
.
* Pushes the resulting string on the stack and returns it.
*
*
* @param ptr the lua_State*
pointer
* @param s the string
* @param p the replaced sequence
* @param r the replacing string
* @return see description
*/
protected native String luaL_gsub(long ptr, String s, String p, String r); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) luaL_gsub((lua_State *) L, (const char *) s, (const char *) p, (const char *) r);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of luaL_len
*
*
* [-0, +0, e]
*
*
*
* lua_Integer luaL_len (lua_State *L, int index);
*
*
*
* Returns the "length" of the value at the given index
* as a number;
* it is equivalent to the '#
' operator in Lua (see §3.4.7).
* Raises an error if the result of the operation is not an integer.
* (This case only can happen through metamethods.)
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int luaL_len(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_len((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_loadstring
*
*
* [-0, +1, –]
*
*
*
* int luaL_loadstring (lua_State *L, const char *s);
*
*
*
* Loads a string as a Lua chunk.
* This function uses lua_load
to load the chunk in
* the zero-terminated string s
.
*
*
*
* This function returns the same results as lua_load
.
*
*
*
* Also as lua_load
, this function only loads the chunk;
* it does not run it.
*
*
* @param ptr the lua_State*
pointer
* @param s the string
* @return see description
*/
protected native int luaL_loadstring(long ptr, String s); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_loadstring((lua_State *) L, (const char *) s);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_newmetatable
*
*
* [-0, +1, m]
*
*
*
* int luaL_newmetatable (lua_State *L, const char *tname);
*
*
*
* If the registry already has the key tname
,
* returns 0.
* Otherwise,
* creates a new table to be used as a metatable for userdata,
* adds to this new table the pair __name = tname
,
* adds to the registry the pair [tname] = new table
,
* and returns 1.
* (The entry __name
is used by some error-reporting functions.)
*
*
*
* In both cases pushes onto the stack the final value associated
* with tname
in the registry.
*
*
* @param ptr the lua_State*
pointer
* @param tname type name
* @return see description
*/
protected native int luaL_newmetatable(long ptr, String tname); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_newmetatable((lua_State *) L, (const char *) tname);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_newmetatable
*
*
* [-0, +1, m]
*
*
*
* int luaL_newmetatable (lua_State *L, const char *tname);
*
*
*
* If the registry already has the key tname
,
* returns 0.
* Otherwise,
* creates a new table to be used as a metatable for userdata,
* adds to this new table the pair __name = tname
,
* adds to the registry the pair [tname] = new table
,
* and returns 1.
* (The entry __name
is used by some error-reporting functions.)
*
*
*
* In both cases pushes onto the stack the final value associated
* with tname
in the registry.
*
*
* @param ptr the lua_State*
pointer
* @param tname type name
*/
protected native void luaJ_newmetatable(long ptr, String tname); /*
lua_State * L = (lua_State *) ptr;
luaL_newmetatable((lua_State *) L, (const char *) tname);
*/
/**
* Wrapper of luaL_newstate
*
*
* [-0, +0, –]
*
*
*
* lua_State *luaL_newstate (void);
*
*
*
* Creates a new Lua state.
* It calls lua_newstate
with an
* allocator based on the standard C realloc
function
* and then sets a panic function (see §4.6) that prints
* an error message to the standard error output in case of fatal
* errors.
*
*
*
* Returns the new state,
* or NULL
if there is a memory allocation error.
*
*
* @param lid the id of the Lua state, to be used to identify between Java and Lua
* @return see description
*/
protected native long luaL_newstate(int lid); /*
lua_State* L = luaL_newstate();
luaJavaSetup(L, env, lid);
return (jlong) L;
*/
/**
* Wrapper of luaL_openlibs
*
*
* [-0, +0, e]
*
*
*
* void luaL_openlibs (lua_State *L);
*
*
*
* Opens all standard Lua libraries into the given state.
*
*
* @param ptr the lua_State*
pointer
*/
protected native void luaL_openlibs(long ptr); /*
lua_State * L = (lua_State *) ptr;
luaL_openlibs((lua_State *) L);
*/
/**
* Wrapper of luaL_ref
*
*
* [-1, +0, m]
*
*
*
* int luaL_ref (lua_State *L, int t);
*
*
*
* Creates and returns a reference,
* in the table at index t
,
* for the object at the top of the stack (and pops the object).
*
*
*
* A reference is a unique integer key.
* As long as you do not manually add integer keys into table t
,
* luaL_ref
ensures the uniqueness of the key it returns.
* You can retrieve an object referred by reference r
* by calling lua_rawgeti(L, t, r)
.
* Function luaL_unref
frees a reference and its associated object.
*
*
*
* If the object at the top of the stack is nil,
* luaL_ref
returns the constant LUA_REFNIL
.
* The constant LUA_NOREF
is guaranteed to be different
* from any reference returned by luaL_ref
.
*
*
* @param ptr the lua_State*
pointer
* @param t the stack index
* @return see description
*/
protected native int luaL_ref(long ptr, int t); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaL_ref((lua_State *) L, (int) t);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_setmetatable
*
*
* [-0, +0, –]
*
*
*
* void luaL_setmetatable (lua_State *L, const char *tname);
*
*
*
* Sets the metatable of the object at the top of the stack
* as the metatable associated with name tname
* in the registry (see luaL_newmetatable
).
*
*
* @param ptr the lua_State*
pointer
* @param tname type name
*/
protected native void luaL_setmetatable(long ptr, String tname); /*
lua_State * L = (lua_State *) ptr;
luaL_setmetatable((lua_State *) L, (const char *) tname);
*/
/**
* Wrapper of luaL_testudata
*
*
* [-0, +0, m]
*
*
*
* void *luaL_testudata (lua_State *L, int arg, const char *tname);
*
*
*
* This function works like luaL_checkudata
,
* except that, when the test fails,
* it returns NULL
instead of raising an error.
*
*
* @param ptr the lua_State*
pointer
* @param arg function argument index
* @param tname type name
* @return see description
*/
protected native long luaL_testudata(long ptr, int arg, String tname); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) luaL_testudata((lua_State *) L, (int) arg, (const char *) tname);
return returnValueReceiver;
*/
/**
* Wrapper of luaL_tolstring
*
*
* [-0, +1, e]
*
*
*
* const char *luaL_tolstring (lua_State *L, int idx, size_t *len);
*
*
*
* Converts any Lua value at the given index to a C string
* in a reasonable format.
* The resulting string is pushed onto the stack and also
* returned by the function.
* If len
is not NULL
,
* the function also sets *len
with the string length.
*
*
*
* If the value has a metatable with a __tostring
field,
* then luaL_tolstring
calls the corresponding metamethod
* with the value as argument,
* and uses the result of the call as its result.
*
*
* @param ptr the lua_State*
pointer
* @param idx the stack position
* @param len pointer to length
* @return see description
*/
protected native String luaL_tolstring(long ptr, int idx, long len); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) luaL_tolstring((lua_State *) L, (int) idx, (size_t *) len);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of luaL_traceback
*
*
* [-0, +1, m]
*
*
*
* void luaL_traceback (lua_State *L, lua_State *L1, const char *msg,
* int level);
*
*
*
* Creates and pushes a traceback of the stack L1
.
* If msg
is not NULL
it is appended
* at the beginning of the traceback.
* The level
parameter tells at which level
* to start the traceback.
*
*
* @param ptr the lua_State*
pointer
* @param L1 a lua_State*
pointer
* @param msg a message
* @param level the running level
*/
protected native void luaL_traceback(long ptr, long L1, String msg, int level); /*
lua_State * L = (lua_State *) ptr;
luaL_traceback((lua_State *) L, (lua_State *) L1, (const char *) msg, (int) level);
*/
/**
* Wrapper of luaL_typename
*
*
* [-0, +0, –]
*
*
*
* const char *luaL_typename (lua_State *L, int index);
*
*
*
* Returns the name of the type of the value at the given index.
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native String luaL_typename(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
const char * returnValueReceiver = (const char *) luaL_typename((lua_State *) L, (int) index);
return env->NewStringUTF(returnValueReceiver);
*/
/**
* Wrapper of luaL_unref
*
*
* [-0, +0, –]
*
*
*
* void luaL_unref (lua_State *L, int t, int ref);
*
*
*
* Releases reference ref
from the table at index t
* (see luaL_ref
).
* The entry is removed from the table,
* so that the referred object can be collected.
* The reference ref
is also freed to be used again.
*
*
*
* If ref
is LUA_NOREF
or LUA_REFNIL
,
* luaL_unref
does nothing.
*
*
* @param ptr the lua_State*
pointer
* @param t the stack index
* @param ref the reference
*/
protected native void luaL_unref(long ptr, int t, int ref); /*
lua_State * L = (lua_State *) ptr;
luaL_unref((lua_State *) L, (int) t, (int) ref);
*/
/**
* Wrapper of luaL_where
*
*
* [-0, +1, m]
*
*
*
* void luaL_where (lua_State *L, int lvl);
*
*
*
* Pushes onto the stack a string identifying the current position
* of the control at level lvl
in the call stack.
* Typically this string has the following format:
*
*
*
*
* chunkname:currentline:
*
*
*
* Level 0 is the running function,
* level 1 is the function that called the running function,
* etc.
*
*
*
* This function is used to build a prefix for error messages.
*
*
* @param ptr the lua_State*
pointer
* @param lvl the running level
*/
protected native void luaL_where(long ptr, int lvl); /*
lua_State * L = (lua_State *) ptr;
luaL_where((lua_State *) L, (int) lvl);
*/
/**
* A wrapper function
*
*
* Open a library indivisually, alternative to luaL_openlibs
*
*
* @param ptr the lua_State*
pointer
* @param lib library name
*/
protected native void luaJ_openlib(long ptr, String lib); /*
lua_State * L = (lua_State *) ptr;
luaJ_openlib((lua_State *) L, (const char *) lib);
*/
/**
* A wrapper function
*
*
* See lua_compare
*
*
* @param ptr the lua_State*
pointer
* @param index1 the stack position of the first element
* @param index2 the stack position of the second element
* @param op the operator
* @return see description
*/
protected native int luaJ_compare(long ptr, int index1, int index2, int op); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_compare((lua_State *) L, (int) index1, (int) index2, (int) op);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Wrapper of lua_(obj)len
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int luaJ_len(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_len((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Load a direct buffer
*
*
* @param ptr the lua_State*
pointer
* @param buffer the buffer (expecting direct)
* @param size size
* @param name the name
* @return see description
*/
protected native int luaJ_loadbuffer(long ptr, Buffer buffer, int size, String name); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_loadbuffer((lua_State *) L, (unsigned char *) buffer, (int) size, (const char *) name);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Run a direct buffer
*
*
* @param ptr the lua_State*
pointer
* @param buffer the buffer (expecting direct)
* @param size size
* @param name the name
* @return see description
*/
protected native int luaJ_dobuffer(long ptr, Buffer buffer, int size, String name); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_dobuffer((lua_State *) L, (unsigned char *) buffer, (int) size, (const char *) name);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Protected call
*
*
* @param ptr the lua_State*
pointer
* @param nargs the number of arguments that you pushed onto the stack
* @param nresults the number of results, or LUA_MULTRET
* @return see description
*/
protected native int luaJ_pcall(long ptr, int nargs, int nresults); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_pcall((lua_State *) L, (int) nargs, (int) nresults);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Resume a coroutine
*
*
* @param ptr the lua_State*
pointer
* @param nargs the number of arguments that you pushed onto the stack
* @return see description
*/
protected native int luaJ_resume(long ptr, int nargs); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_resume((lua_State *) L, (int) nargs);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Push a Java object
*
*
* @param ptr the lua_State*
pointer
* @param obj the Java object
*/
protected native void luaJ_pushobject(long ptr, Object obj); /*
lua_State * L = (lua_State *) ptr;
luaJ_pushobject((JNIEnv *) env, (lua_State *) L, (jobject) obj);
*/
/**
* A wrapper function
*
*
* Push a Java class
*
*
* @param ptr the lua_State*
pointer
* @param clazz the Java class
*/
protected native void luaJ_pushclass(long ptr, Object clazz); /*
lua_State * L = (lua_State *) ptr;
luaJ_pushclass((JNIEnv *) env, (lua_State *) L, (jobject) clazz);
*/
/**
* A wrapper function
*
*
* Push a Java array
*
*
* @param ptr the lua_State*
pointer
* @param array the Java array
*/
protected native void luaJ_pusharray(long ptr, Object array); /*
lua_State * L = (lua_State *) ptr;
luaJ_pusharray((JNIEnv *) env, (lua_State *) L, (jobject) array);
*/
/**
* A wrapper function
*
*
* Push a JFunction
*
*
* @param ptr the lua_State*
pointer
* @param func the function object
*/
protected native void luaJ_pushfunction(long ptr, Object func); /*
lua_State * L = (lua_State *) ptr;
luaJ_pushfunction((JNIEnv *) env, (lua_State *) L, (jobject) func);
*/
/**
* A wrapper function
*
*
* Is a Java object (including object, array or class)
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native int luaJ_isobject(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_isobject((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Convert to Java object if it is one
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native Object luaJ_toobject(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jobject returnValueReceiver = (jobject) luaJ_toobject((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Create a new thread
*
*
* @param ptr the lua_State*
pointer
* @param lid the id of the Lua state, to be used to identify between Java and Lua
* @return see description
*/
protected native long luaJ_newthread(long ptr, int lid); /*
lua_State * L = (lua_State *) ptr;
jlong returnValueReceiver = (jlong) luaJ_newthread((lua_State *) L, (int) lid);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Append a searcher loading from Java side into package.searchers / loaders
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native int luaJ_initloader(long ptr); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_initloader((lua_State *) L);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Runs {@code CallNonvirtualMethodA}. See AbstractLua for usages.
* Parameters should be boxed and pushed on stack.
*
*
* @param ptr the lua_State*
pointer
* @param clazz the Java class
* @param method the method name
* @param sig the method signature used in {@code GetMethodID}
* @param obj the Java object
* @param params encoded parameter types
* @return see description
*/
protected native int luaJ_invokespecial(long ptr, Class clazz, String method, String sig, Object obj, String params); /*
lua_State * L = (lua_State *) ptr;
jint returnValueReceiver = (jint) luaJ_invokespecial((JNIEnv *) env, (lua_State *) L, (jclass) clazz, (const char *) method, (const char *) sig, (jobject) obj, (const char *) params);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* Removes the thread from the global registry, thus allowing it to get garbage collected
*
*
* @param ptr the lua_State*
pointer
*/
protected native void luaJ_removestateindex(long ptr); /*
lua_State * L = (lua_State *) ptr;
luaJ_removestateindex((lua_State *) L);
*/
/**
* A wrapper function
*
*
* Performs a full garbage-collection cycle
*
*
* @param ptr the lua_State*
pointer
*/
protected native void luaJ_gc(long ptr); /*
lua_State * L = (lua_State *) ptr;
luaJ_gc((lua_State *) L);
*/
/**
* A wrapper function
*
*
* See lua_dump
*
*
* @param ptr the lua_State*
pointer
* @return see description
*/
protected native Object luaJ_dumptobuffer(long ptr); /*
lua_State * L = (lua_State *) ptr;
jobject returnValueReceiver = (jobject) luaJ_dumptobuffer((lua_State *) L);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* See lua_tolstring
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native Object luaJ_tobuffer(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jobject returnValueReceiver = (jobject) luaJ_tobuffer((lua_State *) L, (int) index);
return returnValueReceiver;
*/
/**
* A wrapper function
*
*
* See lua_tolstring
*
*
* @param ptr the lua_State*
pointer
* @param index the stack position of the element
* @return see description
*/
protected native Object luaJ_todirectbuffer(long ptr, int index); /*
lua_State * L = (lua_State *) ptr;
jobject returnValueReceiver = (jobject) luaJ_todirectbuffer((lua_State *) L, (int) index);
return returnValueReceiver;
*/
}