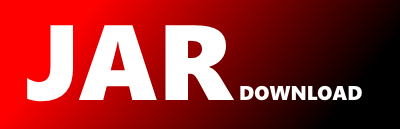
pl.allegro.tech.build.axion.release.domain.hooks.FileUpdateHookAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of axion-release-plugin Show documentation
Show all versions of axion-release-plugin Show documentation
Gradle release and version management plugin
package pl.allegro.tech.build.axion.release.domain.hooks;
import groovy.lang.Closure;
import pl.allegro.tech.build.axion.release.util.FileLoader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.BiFunction;
import java.util.regex.Pattern;
public class FileUpdateHookAction implements ReleaseHookAction {
private final Map arguments;
public FileUpdateHookAction(Map arguments) {
this.arguments = arguments;
}
@Override
public void act(final HookContext hookContext) {
arguments.computeIfAbsent("files", (k) -> Arrays.asList(arguments.get("file")));
((List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy