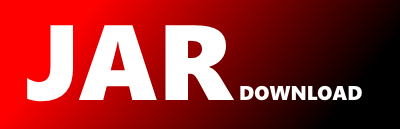
pl.allegro.tech.build.axion.release.domain.VersionConfig.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of axion-release-plugin Show documentation
Show all versions of axion-release-plugin Show documentation
Gradle release and version management plugin
The newest version!
package pl.allegro.tech.build.axion.release.domain
import org.gradle.api.Action
import org.gradle.api.Incubating
import org.gradle.api.file.Directory
import org.gradle.api.provider.MapProperty
import org.gradle.api.provider.Property
import org.gradle.api.provider.Provider
import org.gradle.api.provider.SetProperty
import org.gradle.api.tasks.Input
import org.gradle.api.tasks.Internal
import org.gradle.api.tasks.Nested
import pl.allegro.tech.build.axion.release.domain.hooks.HooksConfig
import pl.allegro.tech.build.axion.release.domain.properties.VersionProperties
import pl.allegro.tech.build.axion.release.domain.scm.ScmPosition
import pl.allegro.tech.build.axion.release.infrastructure.di.MemoizedVersionSupplier
import pl.allegro.tech.build.axion.release.infrastructure.di.VersionSupplier
import javax.inject.Inject
import java.util.regex.Pattern
import static pl.allegro.tech.build.axion.release.TagPrefixConf.defaultPrefix
abstract class VersionConfig extends BaseExtension {
private final VersionSupplier versionSupplier = new VersionSupplier()
private final MemoizedVersionSupplier cachedVersionSupplier = new MemoizedVersionSupplier()
private static final String DRY_RUN_FLAG = 'release.dryRun'
private static final String IGNORE_UNCOMMITTED_CHANGES_PROPERTY = 'release.ignoreUncommittedChanges'
private static final String FORCE_SNAPSHOT_PROPERTY = 'release.forceSnapshot'
private static final String USE_HIGHEST_VERSION_PROPERTY = 'release.useHighestVersion'
private static final String LOCAL_ONLY = "release.localOnly"
private static final String FORCE_VERSION_PROPERTY = 'release.version'
private static final String DEPRECATED_FORCE_VERSION_PROPERTY = 'release.forceVersion'
private static final String VERSION_INCREMENTER_PROPERTY = 'release.versionIncrementer'
private static final String VERSION_CREATOR_PROPERTY = 'release.versionCreator'
private static final String RELEASE_ONLY_ON_RELEASE_BRANCHES_PROPERTY = 'release.releaseOnlyOnReleaseBranches'
private static final String RELEASE_BRANCH_NAMES_PROPERTY = 'release.releaseBranchNames'
@Inject
VersionConfig(Directory repositoryDirectory) {
getDryRun().convention(gradlePropertyPresent(DRY_RUN_FLAG).orElse(false))
getLocalOnly().convention(false)
getIgnoreUncommittedChanges().convention(true)
getUseHighestVersion().convention(false)
getUnshallowRepoOnCI().convention(false)
getIgnoreGlobalGitConfig().convention(false)
getReleaseBranchNames().convention(gradlePropertyAsSet(RELEASE_BRANCH_NAMES_PROPERTY).orElse(['master', 'main'] as Set))
getReleaseOnlyOnReleaseBranches().convention(gradlePropertyPresent(RELEASE_ONLY_ON_RELEASE_BRANCHES_PROPERTY).orElse(false))
getReleaseBranchPattern().convention(Pattern.compile('^' + defaultPrefix() + '(/.*)?$'))
getSanitizeVersion().convention(true)
getCreateReleaseCommit().convention(false)
getVersionCreator().convention(PredefinedVersionCreator.VERSION_WITH_BRANCH.versionCreator)
getVersionIncrementer().convention((VersionProperties.Incrementer) { VersionIncrementerContext context -> return context.currentVersion.incrementPatchVersion() })
getSnapshotCreator().convention(PredefinedSnapshotCreator.SIMPLE.snapshotCreator)
getReleaseCommitMessage().convention(PredefinedReleaseCommitMessageCreator.DEFAULT.commitMessageCreator)
repository = objects.newInstance(RepositoryConfig, repositoryDirectory)
}
@Nested
final RepositoryConfig repository
@Nested
final TagNameSerializationConfig tag = objects.newInstance(TagNameSerializationConfig)
@Nested
final ChecksConfig checks = objects.newInstance(ChecksConfig)
@Nested
final NextVersionConfig nextVersion = objects.newInstance(NextVersionConfig)
@Nested
final HooksConfig hooks = objects.newInstance(HooksConfig)
@Nested
final MonorepoConfig monorepoConfig = objects.newInstance(MonorepoConfig)
@Internal
abstract Property getLocalOnly()
@Internal
abstract Property getDryRun()
@Internal
abstract Property getIgnoreUncommittedChanges()
@Internal
abstract SetProperty getReleaseBranchNames()
@Internal
abstract Property getReleaseOnlyOnReleaseBranches()
@Internal
abstract Property getIgnoreGlobalGitConfig()
@Internal
@Incubating
abstract Property getUnshallowRepoOnCI();
@Internal
abstract MapProperty getBranchVersionIncrementer();
@Internal
abstract Property getReleaseBranchPattern();
@Internal
abstract Property getSanitizeVersion()
@Internal
abstract Property getCreateReleaseCommit()
@Internal
abstract Property getVersionCreator()
@Internal
abstract Property getSnapshotCreator()
@Internal
abstract MapProperty getBranchVersionCreator()
@Internal
abstract Property getVersionIncrementer()
@Internal
abstract Property getReleaseCommitMessage()
@Internal
abstract Property getUseHighestVersion();
Provider ignoreUncommittedChanges() {
gradlePropertyPresent(IGNORE_UNCOMMITTED_CHANGES_PROPERTY)
.orElse(ignoreUncommittedChanges)
}
Provider forceSnapshot() {
gradlePropertyPresent(FORCE_SNAPSHOT_PROPERTY).orElse(false)
}
Provider useHighestVersion() {
gradlePropertyPresent(USE_HIGHEST_VERSION_PROPERTY).orElse(useHighestVersion)
}
Provider localOnly() {
gradlePropertyPresent(LOCAL_ONLY).orElse(localOnly)
}
Provider forcedVersion() {
gradleProperty(FORCE_VERSION_PROPERTY)
.orElse(gradleProperty(DEPRECATED_FORCE_VERSION_PROPERTY))
.map({ it.trim() })
.map({ it.isBlank() ? null : it })
}
Provider versionIncrementerType() {
gradleProperty(VERSION_INCREMENTER_PROPERTY)
}
Provider versionCreatorType() {
gradleProperty(VERSION_CREATOR_PROPERTY)
}
void repository(Action action) {
action.execute(repository)
}
void tag(Action action) {
action.execute(tag)
}
void checks(Action action) {
action.execute(checks)
}
void nextVersion(Action action) {
action.execute(nextVersion)
}
void hooks(Action action) {
action.execute(hooks)
}
void monorepo(Action action) {
action.execute(monorepoConfig)
}
void versionCreator(String type) {
this.versionCreator.set(PredefinedVersionCreator.versionCreatorFor(type))
}
@Deprecated
void releaseCommitMessage(PredefinedReleaseCommitMessageCreator.CommitMessageCreator commitMessageCreator) {
this.releaseCommitMessage.set(commitMessageCreator)
}
@Deprecated
void createReleaseCommit(boolean createReleaseCommit) {
this.createReleaseCommit.set(createReleaseCommit)
}
void versionCreator(VersionProperties.Creator versionCreator) {
this.versionCreator.set(versionCreator)
}
void snapshotCreator(VersionProperties.Creator snapshotCreator) {
this.snapshotCreator.set(snapshotCreator)
}
void branchVersionCreator(Map creators) {
this.branchVersionCreator.putAll(creators)
}
void branchVersionCreators(Map creators) {
this.branchVersionCreator.putAll(creators)
}
void versionIncrementer(String ruleName) {
this.versionIncrementer.set(PredefinedVersionIncrementer.versionIncrementerFor(ruleName))
}
void versionIncrementer(String ruleName, Map configuration) {
this.versionIncrementer.set(PredefinedVersionIncrementer.versionIncrementerFor(ruleName, configuration))
}
void versionIncrementer(VersionProperties.Incrementer versionIncrementer) {
this.versionIncrementer.set(versionIncrementer)
}
void branchVersionIncrementer(Map creators) {
this.branchVersionIncrementer.putAll(creators)
}
Provider versionProvider() {
def cachedVersionSupplier = this.cachedVersionSupplier
providers.provider({ cachedVersionSupplier.resolve(this, layout.projectDirectory) })
}
Provider uncachedVersionProvider() {
def versionSupplier = this.versionSupplier
providers.provider({ versionSupplier.resolve(this, layout.projectDirectory) })
}
@Nested
VersionService.DecoratedVersion getUncached() {
return uncachedVersionProvider().get()
}
@Input
String getVersion() {
return versionProvider().map({ it.decoratedVersion }).get()
}
@Input
String getPreviousVersion() {
return versionProvider().map({ it.previousVersion }).get()
}
@Input
String getUndecoratedVersion() {
return versionProvider().map({ it.undecoratedVersion }).get()
}
@Nested
ScmPosition getScmPosition() {
return versionProvider().map({ it.position }).get()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy