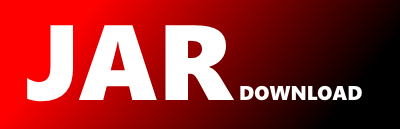
pl.allegro.tech.hermes.consumers.consumer.sender.MultiMessageSendingResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hermes-consumers Show documentation
Show all versions of hermes-consumers Show documentation
Fast and reliable message broker built on top of Kafka.
package pl.allegro.tech.hermes.consumers.consumer.sender;
import com.google.common.collect.ImmutableList;
import java.net.URI;
import java.util.Comparator;
import java.util.List;
import java.util.Optional;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import static java.util.stream.Collectors.joining;
public class MultiMessageSendingResult implements MessageSendingResult {
private final List children;
public MultiMessageSendingResult(List children) {
this.children = ImmutableList.copyOf(children);
}
@Override
public int getStatusCode() {
if (succeeded()) {
return children.stream().mapToInt(MessageSendingResult::getStatusCode).max().orElse(0);
} else {
return children.stream().filter(child -> !child.succeeded()).mapToInt(MessageSendingResult::getStatusCode).max().orElse(0);
}
}
@Override
public boolean isLoggable() {
return children.stream().anyMatch(MessageSendingResult::isLoggable);
}
@Override
public boolean ignoreInRateCalculation(boolean retryClientErrors, boolean isOAuthSecuredSubscription) {
return children.stream().allMatch(r -> r.ignoreInRateCalculation(retryClientErrors, isOAuthSecuredSubscription));
}
@Override
public Optional getRetryAfterMillis() {
return children.stream()
.map(MessageSendingResult::getRetryAfterMillis)
.filter(Optional::isPresent).map(Optional::get)
.min(Comparator.naturalOrder());
}
@Override
public boolean succeeded() {
return !children.isEmpty() && children.stream().allMatch(MessageSendingResult::succeeded);
}
@Override
public boolean isClientError() {
List failed = children.stream().filter(child -> !child.succeeded()).collect(Collectors.toList());
return !failed.isEmpty() &&
failed.stream().allMatch(MessageSendingResult::isClientError);
}
@Override
public boolean isTimeout() {
return !children.isEmpty() && children.stream().anyMatch(MessageSendingResult::isTimeout);
}
@Override
public boolean isRetryLater() {
return children.isEmpty() || children.stream().anyMatch(MessageSendingResult::isRetryLater);
}
@Override
public List getLogInfo() {
return children.stream().map(child ->
new MessageSendingResultLogInfo(child.getRequestUri(), child.getFailure(), child.getRootCause()))
.collect(Collectors.toList());
}
@Override
public List getSucceededUris(Predicate filter) {
return children.stream()
.filter(filter)
.map(SingleMessageSendingResult::getRequestUri)
.filter(Optional::isPresent)
.map(Optional::get)
.collect(Collectors.toList());
}
public List getChildren() {
return children;
}
@Override
public String getRootCause() {
return children.stream()
.map(child -> child.getRequestUri().map(Object::toString).orElse("") + ":" + child.getRootCause())
.collect(joining(";"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy