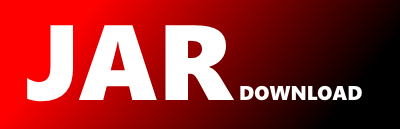
pl.allegro.tech.hermes.consumers.consumer.rate.maxrate.ConsumerMaxRates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hermes-consumers Show documentation
Show all versions of hermes-consumers Show documentation
Fast and reliable message broker built on top of Kafka.
package pl.allegro.tech.hermes.consumers.consumer.rate.maxrate;
import pl.allegro.tech.hermes.api.SubscriptionName;
import pl.allegro.tech.hermes.consumers.subscription.id.SubscriptionId;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.stream.Collectors;
class ConsumerMaxRates {
private final Map maxRates;
ConsumerMaxRates() {
this.maxRates = new ConcurrentHashMap<>();
}
void setAllMaxRates(ConsumerMaxRates newMaxRates) {
maxRates.clear();
maxRates.putAll(newMaxRates.maxRates);
}
Optional getMaxRate(SubscriptionName subscription) {
return Optional.ofNullable(maxRates.get(subscription));
}
void setMaxRate(SubscriptionName subscription, MaxRate maxRate) {
maxRates.put(subscription, maxRate);
}
public void cleanup(Set subscriptions) {
this.maxRates.entrySet()
.removeIf(entry -> !subscriptions.contains(entry.getKey()));
}
int size() {
return maxRates.size();
}
Map toSubscriptionsIdsMap(SubscriptionIdMapper subscriptionIdMapping) {
return maxRates.keySet().stream()
.map(subscriptionIdMapping::mapToSubscriptionId)
.filter(Optional::isPresent)
.collect(Collectors.toMap(Optional::get, subscriptionId -> maxRates.get(subscriptionId.get().getSubscriptionName())));
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ConsumerMaxRates that = (ConsumerMaxRates) o;
return Objects.equals(maxRates, that.maxRates);
}
@Override
public int hashCode() {
return Objects.hash(maxRates);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy