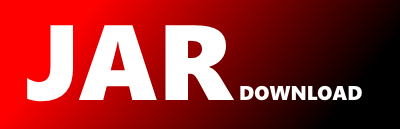
pl.allegro.tech.hermes.management.domain.owner.OwnerSources Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hermes-management Show documentation
Show all versions of hermes-management Show documentation
Fast and reliable message broker built on top of Kafka.
package pl.allegro.tech.hermes.management.domain.owner;
import com.google.common.collect.ImmutableList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.Function;
import java.util.stream.Collectors;
import pl.allegro.tech.hermes.api.ErrorCode;
import pl.allegro.tech.hermes.common.exception.HermesException;
public class OwnerSources implements Iterable {
private final Map ownerSourcesByNames;
private final List ownerSources;
public OwnerSources(List ownerSources) {
if (ownerSources.isEmpty()) {
throw new IllegalArgumentException("At least one owner source must be configured");
}
this.ownerSourcesByNames =
ownerSources.stream()
.collect(
Collectors.toMap(
OwnerSource::name,
Function.identity(),
(a, b) -> {
throw new IllegalArgumentException("Duplicate owner source " + a.name());
}));
this.ownerSources = ImmutableList.copyOf(ownerSources);
}
public Optional getByName(String name) {
return Optional.ofNullable(ownerSourcesByNames.get(name));
}
public OwnerSource.Autocompletion getAutocompletionFor(String name) {
OwnerSource source = getByName(name).orElseThrow(() -> new OwnerSourceNotFound(name));
return source.autocompletion().orElseThrow(() -> new AutocompleteNotSupportedException(source));
}
public Iterator iterator() {
return ownerSources.iterator();
}
private static class AutocompleteNotSupportedException extends HermesException {
AutocompleteNotSupportedException(OwnerSource source) {
super("Owner source '" + source.name() + "' doesn't support autocomplete");
}
@Override
public ErrorCode getCode() {
return ErrorCode.OWNER_SOURCE_DOESNT_SUPPORT_AUTOCOMPLETE;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy