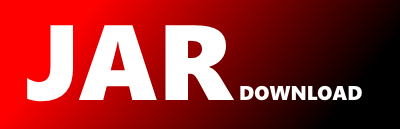
pl.allegro.tech.hermes.management.infrastructure.prometheus.RestTemplatePrometheusClient Maven / Gradle / Ivy
package pl.allegro.tech.hermes.management.infrastructure.prometheus;
import static java.net.URLEncoder.encode;
import static java.nio.charset.StandardCharsets.UTF_8;
import com.google.common.base.Preconditions;
import io.micrometer.core.instrument.MeterRegistry;
import java.net.URI;
import java.time.Duration;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.TimeUnit;
import java.util.stream.Collectors;
import org.apache.commons.lang3.tuple.Pair;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.web.client.HttpStatusCodeException;
import org.springframework.web.client.RestTemplate;
import pl.allegro.tech.hermes.api.MetricDecimalValue;
import pl.allegro.tech.hermes.management.infrastructure.metrics.MonitoringMetricsContainer;
public class RestTemplatePrometheusClient implements PrometheusClient {
private static final Logger logger = LoggerFactory.getLogger(RestTemplatePrometheusClient.class);
private final URI prometheusUri;
private final RestTemplate restTemplate;
private final ExecutorService executorService;
private final Duration fetchingTimeout;
private final MeterRegistry meterRegistry;
public RestTemplatePrometheusClient(
RestTemplate restTemplate,
URI prometheusUri,
ExecutorService executorService,
Duration fetchingTimeoutMillis,
MeterRegistry meterRegistry) {
this.restTemplate = restTemplate;
this.prometheusUri = prometheusUri;
this.executorService = executorService;
this.fetchingTimeout = fetchingTimeoutMillis;
this.meterRegistry = meterRegistry;
}
@Override
public MonitoringMetricsContainer readMetrics(List queries) {
return fetchInParallelFromPrometheus(queries);
}
private MonitoringMetricsContainer fetchInParallelFromPrometheus(List queries) {
CompletableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy