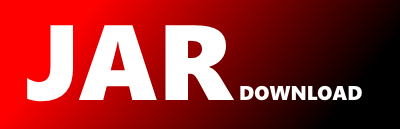
pl.allegro.tech.hermes.management.infrastructure.query.MatcherQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hermes-management Show documentation
Show all versions of hermes-management Show documentation
Fast and reliable message broker built on top of Kafka.
package pl.allegro.tech.hermes.management.infrastructure.query;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.Map;
import java.util.function.Predicate;
import java.util.stream.Stream;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import pl.allegro.tech.hermes.api.Query;
import pl.allegro.tech.hermes.management.infrastructure.query.matcher.Matcher;
import pl.allegro.tech.hermes.management.infrastructure.query.matcher.MatcherException;
import pl.allegro.tech.hermes.management.infrastructure.query.matcher.MatcherInputException;
public class MatcherQuery implements Query {
private static final Logger logger = LoggerFactory.getLogger(MatcherQuery.class);
private final Matcher matcher;
private final ObjectMapper objectMapper;
private MatcherQuery(Matcher matcher, ObjectMapper objectMapper) {
this.matcher = matcher;
this.objectMapper = objectMapper;
}
@Override
public Stream filter(Stream input) {
return input.filter(getPredicate());
}
@Override
public Stream filterNames(Stream input) {
return input.filter(getSoftPredicate());
}
public Predicate getPredicate() {
return (value) -> {
try {
return matcher.match(convertToMap(value));
} catch (MatcherException e) {
logger.info("Failed to match {}, skipping", value, e);
return false;
} catch (MatcherInputException e) {
logger.error("Not existing query property", e);
throw new IllegalArgumentException(e);
}
};
}
public Predicate getSoftPredicate() {
return (value) -> {
try {
return matcher.match(convertToMap(value));
} catch (MatcherException e) {
logger.info("Failed to match {}, skipping", value, e);
return false;
} catch (MatcherInputException e) {
// Non-existing property is normal when querying objects with non-default class
return true;
}
};
}
@SuppressWarnings("unchecked")
// workaround for type which is not java bean
private Map convertToMap(K value) {
return objectMapper.convertValue(value, Map.class);
}
public static Query fromMatcher(Matcher matcher, ObjectMapper objectMapper) {
return new MatcherQuery<>(matcher, objectMapper);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy