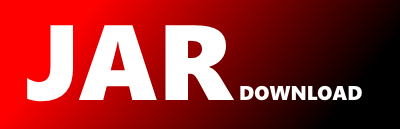
pl.chilldev.web.faces.context.FacesPageMetaModelResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of web-faces Show documentation
Show all versions of web-faces Show documentation
Facelets tags definition.
/**
* This file is part of the ChillDev-Web.
*
* @license http://mit-license.org/ The MIT license
* @copyright 2014 - 2015 © by Rafał Wrzeszcz - Wrzasq.pl.
*/
package pl.chilldev.web.faces.context;
import javax.faces.view.facelets.FaceletContext;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import pl.chilldev.web.core.markup.Generator;
import pl.chilldev.web.core.page.PageMetaModel;
/**
* Resolves page meta model from Facelets context attribute.
*/
public class FacesPageMetaModelResolver implements PageMetaModelResolver
{
/**
* Default Facelets attribute key for storing current page model.
*/
public static final String ATTRIBUTE_NAME_DEFAULT = "pl.chilldev.web.core.page.PageMetaModel";
/**
* Logger.
*/
private Logger logger = LoggerFactory.getLogger(FacesPageMetaModelResolver.class);
/**
* Storage attribute name.
*/
private String attribute;
/**
* Markup generator.
*/
private Generator generator;
/**
* Initializes resolver for given attribute name and with given markup generator.
*
* @param attribute Attribute name.
* @param generator Markup generator.
*/
public FacesPageMetaModelResolver(String attribute, Generator generator)
{
this.attribute = attribute;
this.generator = generator;
}
/**
* Initializes resolver for given attribute name.
*
* @param attribute Attribute name.
*/
public FacesPageMetaModelResolver(String attribute)
{
this(attribute, Generator.DEFAULT_GENERATOR);
}
/**
* Initializes resolver with specified markup generator.
*
* @param generator Markup generator.
*/
public FacesPageMetaModelResolver(Generator generator)
{
this(FacesPageMetaModelResolver.ATTRIBUTE_NAME_DEFAULT, generator);
}
/**
* Initializes resolver for default attribute name with default markup generator.
*/
public FacesPageMetaModelResolver()
{
this(FacesPageMetaModelResolver.ATTRIBUTE_NAME_DEFAULT, Generator.DEFAULT_GENERATOR);
}
/**
* {@inheritDoc}
*/
@Override
public PageMetaModel getPageMetaModel(FaceletContext context)
throws
PageMetaModelContextException
{
Object value = context.getAttribute(this.attribute);
PageMetaModel page;
if (value == null) {
// initialize new model
this.logger.debug(
"Attribtue \"{}\" not found in Facelets context, initializing new page model.",
this.attribute
);
page = this.createPageMetaModel();
context.setAttribute(this.attribute, page);
} else if (value instanceof PageMetaModel) {
// cast existing model
this.logger.debug("Attribtue \"{}\" found in Facelets context.", this.attribute);
page = (PageMetaModel) value;
} else {
this.logger.error(
"Attribtue \"{}\" found in Facelets context had type {}.",
this.attribute,
value.getClass()
);
throw new PageMetaModelContextException(
String.format(
"Attribute \"%s\" in current Facelets context is of type %s.",
this.attribute,
value.getClass()
)
);
}
return page;
}
/**
* Initializes new meta model.
*
* @return Newly created meta model.
*/
private PageMetaModel createPageMetaModel()
{
return new PageMetaModel(this.generator);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy