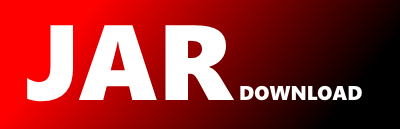
mk.gdx.firebase.distributions.DatabaseDistribution Maven / Gradle / Ivy
Show all versions of gdx-fireapp-core Show documentation
/*
* Copyright 2017 mk
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package mk.gdx.firebase.distributions;
import java.util.Map;
import mk.gdx.firebase.callbacks.CompleteCallback;
import mk.gdx.firebase.callbacks.DataCallback;
import mk.gdx.firebase.callbacks.TransactionCallback;
import mk.gdx.firebase.database.FilterType;
import mk.gdx.firebase.database.OrderByMode;
import mk.gdx.firebase.listeners.ConnectedListener;
import mk.gdx.firebase.listeners.DataChangeListener;
/**
* Provides access to Firebase database.
*
* Before you do some operations on database you should chose on which parts of data you want to operate.
* To do that you need to call {@link #inReference(String)} before each of following methods:
*
* - {@link #setValue(Object)}
*
- {@link #setValue(Object, CompleteCallback)}
*
- {@link #updateChildren(Map)}
*
- {@link #updateChildren(Map, CompleteCallback)}
*
- {@link #onDataChange(Class, DataChangeListener)}
*
- {@link #readValue(Class, DataCallback)}
*
- {@link #push()}
*
- {@link #transaction(Class, TransactionCallback, CompleteCallback)}
*
* If you do not do this {@code RuntimeException} will be thrown.
*/
public interface DatabaseDistribution {
/**
* Listens for database connection events.
*
* Catch moment when application is going to to be connected or disconnected to the database.
*
* @param connectedListener Listener that handles moments when connection status was change, if null: connection listeners will be detached.
*/
void onConnect(ConnectedListener connectedListener);
/**
* Sets database path you want to deal with in next action.
*
* @param databasePath Reference inside your database for ex. {@code "/users"}
* @return this instance with the path set
*/
DatabaseDistribution inReference(String databasePath);
/**
* Sets value for path given by {@code inReference(String)}.
*
* @param value Any value which you want to store. Given object will be transformed to Firebase-like data type.
* @throws RuntimeException if {@link #inReference(String)} was not call before.
*/
void setValue(Object value);
/**
* Sets value for path given by {@code inReference(String)} and gives response by {@code CompleteCallback}.
*
* @param value Any value which you want to store. Given object will be transformed to Firebase-like data type.
* @param completeCallback Callback that handles response
* @throws RuntimeException if {@link #inReference(String)} was not call before.
* @see CompleteCallback
*/
void setValue(Object value, CompleteCallback completeCallback);
/**
* Reads value from path given by {@code inReference(String)} and gives response by {@code DataCallback}.
*
* POJO objects received from each platform should be represented as Map. Conversion will be guarantee later by {@link mk.gdx.firebase.deserialization.DataCallbackMitmConverter}
*
* @param dataType Class you want to retrieve
* @param callback Callback that handles response
* @param Type of data you want to retrieve, associated with {@code dataType} for ex. {@code List.class}
* @param More specific type of data you want to retrieve associated with {@code callback} - should be not-abstract type.
* @throws RuntimeException if {@link #inReference(String)} was not call before.
* @see DataCallback
*/
void readValue(Class dataType, DataCallback callback);
/**
* Handles value changes for path given by {@code inReference(String)} and gives response by {@code DataChangeListener}.
*
* Remember to set database reference earlier by calling the {@link #inReference(String)} method.
*
* POJO objects received from each platform should be represented as Map. Conversion will be guarantee later by {@link mk.gdx.firebase.deserialization.DataChangeListenerMitmConverter}
*
* @param dataType Class you want to retrieve
* @param listener Listener, may by null - if null all listeners for specified database reference will be removed.
* @param Type of data you want to retrieve, associated with {@code dataType} for ex. {@code List.class}
* @param More specific type of data you want to retrieve associated with {@code listener} - should be not-abstract type.
* @throws RuntimeException if {@link #inReference(String)} was not call before.
* @see DataChangeListener
*/
void onDataChange(Class dataType, DataChangeListener listener);
/**
* Applies filter to the next database query.
*
* It should be applied only before {@link #readValue(Class, DataCallback)} or {@link #onDataChange(Class, DataChangeListener)} execution.
* You can read more about filtering here: firebase filtering
*
* @param filterType Filter type that you want to applied, not null
* @param filterArguments Arguments that will be pass to filter method
* @param Type of filter argument, it should be one of the following: Integer, Double, String, Boolean
* @return this
*/
DatabaseDistribution filter(FilterType filterType, V... filterArguments);
/**
* Applies order-by to the next database query.
*
* Only one orderBy can be applied to one query otherwise error will be throw.
*
* For now, the only mode which process argument is: {@link OrderByMode#ORDER_BY_CHILD}
*
* @param orderByMode Order-by mode, not null
* @param argument Order by func argument, may be null
* @return this
*/
DatabaseDistribution orderBy(OrderByMode orderByMode, String argument);
/**
* Creates new object inside database and return {@code this instance} with reference to it set by {@code DatabaseDistribution#inReference()}
*
* Remember to set database reference earlier by calling the {@link #inReference(String)} method.
*
* @return this
* @throws RuntimeException if {@link #inReference(String)} was not call before.
*/
DatabaseDistribution push();
/**
* Removes value in path given by {@code inReference(String)}.
*
* Remember to set database reference earlier by calling the {@link #inReference(String)} method.
*
* @throws RuntimeException if {@link #inReference(String)} was not call before.
*/
void removeValue();
/**
* Removes value for path given by {@code inReference(String)} and gives response by {@code DataChangeListener}.
*
* Remember to set database reference earlier by calling the {@link #inReference(String)} method.
*
* @param completeCallback Complete callback
* @throws RuntimeException if {@link #inReference(String)} was not call before.
* @see CompleteCallback
*/
void removeValue(CompleteCallback completeCallback);
/**
* Updates children's for path given by {@code inReference(String)}.
*
* Remember to set database reference earlier by calling the {@link #inReference(String)} method.
*
* @param data New data
* @throws RuntimeException if {@link #inReference(String)} was not call before.
*/
void updateChildren(Map data);
/**
* Updates children's for path given by {@code inReference(String)} and gives response by {@code CompleteCallback}.
*
* Remember to set database reference earlier by calling the {@link #inReference(String)} method.
*
* @param data New data
* @param completeCallback Callback when done
* @throws RuntimeException if {@link #inReference(String)} was not call before.
*/
void updateChildren(Map data, CompleteCallback completeCallback);
/**
* Provides transaction for value describe by path given by {@code inReference(String)} and gives response by {@code CompleteCallback}
*
* Value that you want to change will be get in {@link TransactionCallback#run(Object)} - there you should
* modify data and returns a new one.
*
* Remember to set database reference earlier by calling the {@link #inReference(String)} method.
*
* @param dataType Type of data you want to get.
* @param transactionCallback Callback called when transaction is complete.
* @param completeCallback Can be null
* @throws RuntimeException if {@link #inReference(String)} was not call before call this method.
* @see CompleteCallback
* @see TransactionCallback
*/
void transaction(Class dataType, TransactionCallback transactionCallback, CompleteCallback completeCallback);
/**
* Keeps your data for offline usage.
*
* You can read more here and here
*
* @param enabled e
*/
void setPersistenceEnabled(boolean enabled);
/**
* Keeps data fresh.
*
* You can read more here and here
*
* Remember to set database reference earlier by calling the {@link #inReference(String)} method.
*
* @param synced If true sync for specified database path will be enabled
* @throws RuntimeException if {@link #inReference(String)} was not call before call this method.
*/
void keepSynced(boolean synced);
}