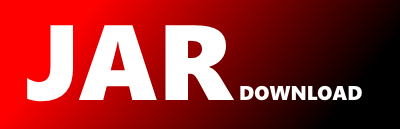
pl.poznan.put.pdb.ImmutablePdbAtomLine Maven / Gradle / Ivy
package pl.poznan.put.pdb;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link PdbAtomLine}.
*
* Use the builder to create immutable instances:
* {@code ImmutablePdbAtomLine.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutablePdbAtomLine.of()}.
*/
@Generated(from = "PdbAtomLine", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutablePdbAtomLine extends PdbAtomLine {
private final int serialNumber;
private final String atomName;
private final String alternateLocation;
private final String residueName;
private final String chainIdentifier;
private final int residueNumber;
private final String insertionCode;
private final double x;
private final double y;
private final double z;
private final double occupancy;
private final double temperatureFactor;
private final String elementSymbol;
private final String charge;
private ImmutablePdbAtomLine(
int serialNumber,
String atomName,
String alternateLocation,
String residueName,
String chainIdentifier,
int residueNumber,
String insertionCode,
double x,
double y,
double z,
double occupancy,
double temperatureFactor,
String elementSymbol,
String charge) {
this.serialNumber = serialNumber;
this.atomName = Objects.requireNonNull(atomName, "atomName");
this.alternateLocation = Objects.requireNonNull(alternateLocation, "alternateLocation");
this.residueName = Objects.requireNonNull(residueName, "residueName");
this.chainIdentifier = Objects.requireNonNull(chainIdentifier, "chainIdentifier");
this.residueNumber = residueNumber;
this.insertionCode = Objects.requireNonNull(insertionCode, "insertionCode");
this.x = x;
this.y = y;
this.z = z;
this.occupancy = occupancy;
this.temperatureFactor = temperatureFactor;
this.elementSymbol = Objects.requireNonNull(elementSymbol, "elementSymbol");
this.charge = Objects.requireNonNull(charge, "charge");
}
private ImmutablePdbAtomLine(
ImmutablePdbAtomLine original,
int serialNumber,
String atomName,
String alternateLocation,
String residueName,
String chainIdentifier,
int residueNumber,
String insertionCode,
double x,
double y,
double z,
double occupancy,
double temperatureFactor,
String elementSymbol,
String charge) {
this.serialNumber = serialNumber;
this.atomName = atomName;
this.alternateLocation = alternateLocation;
this.residueName = residueName;
this.chainIdentifier = chainIdentifier;
this.residueNumber = residueNumber;
this.insertionCode = insertionCode;
this.x = x;
this.y = y;
this.z = z;
this.occupancy = occupancy;
this.temperatureFactor = temperatureFactor;
this.elementSymbol = elementSymbol;
this.charge = charge;
}
/**
*@return The value of the {@code serialNumber} attribute
*/
@Override
public int serialNumber() {
return serialNumber;
}
/**
*@return The value of the {@code atomName} attribute
*/
@Override
public String atomName() {
return atomName;
}
/**
*@return The value of the {@code alternateLocation} attribute
*/
@Override
public String alternateLocation() {
return alternateLocation;
}
/**
*@return The value of the {@code residueName} attribute
*/
@Override
public String residueName() {
return residueName;
}
/**
*@return The value of the {@code chainIdentifier} attribute
*/
@Override
public String chainIdentifier() {
return chainIdentifier;
}
/**
*@return The value of the {@code residueNumber} attribute
*/
@Override
public int residueNumber() {
return residueNumber;
}
/**
*@return The value of the {@code insertionCode} attribute
*/
@Override
public String insertionCode() {
return insertionCode;
}
/**
*@return The value of the {@code x} attribute
*/
@Override
public double x() {
return x;
}
/**
*@return The value of the {@code y} attribute
*/
@Override
public double y() {
return y;
}
/**
*@return The value of the {@code z} attribute
*/
@Override
public double z() {
return z;
}
/**
*@return The value of the {@code occupancy} attribute
*/
@Override
public double occupancy() {
return occupancy;
}
/**
*@return The value of the {@code temperatureFactor} attribute
*/
@Override
public double temperatureFactor() {
return temperatureFactor;
}
/**
*@return The value of the {@code elementSymbol} attribute
*/
@Override
public String elementSymbol() {
return elementSymbol;
}
/**
*@return The value of the {@code charge} attribute
*/
@Override
public String charge() {
return charge;
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#serialNumber() serialNumber} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for serialNumber
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withSerialNumber(int value) {
if (this.serialNumber == value) return this;
return new ImmutablePdbAtomLine(
this,
value,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#atomName() atomName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for atomName
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withAtomName(String value) {
String newValue = Objects.requireNonNull(value, "atomName");
if (this.atomName.equals(newValue)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
newValue,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#alternateLocation() alternateLocation} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for alternateLocation
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withAlternateLocation(String value) {
String newValue = Objects.requireNonNull(value, "alternateLocation");
if (this.alternateLocation.equals(newValue)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
newValue,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#residueName() residueName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for residueName
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withResidueName(String value) {
String newValue = Objects.requireNonNull(value, "residueName");
if (this.residueName.equals(newValue)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
newValue,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#chainIdentifier() chainIdentifier} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for chainIdentifier
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withChainIdentifier(String value) {
String newValue = Objects.requireNonNull(value, "chainIdentifier");
if (this.chainIdentifier.equals(newValue)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
newValue,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#residueNumber() residueNumber} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for residueNumber
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withResidueNumber(int value) {
if (this.residueNumber == value) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
value,
this.insertionCode,
this.x,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#insertionCode() insertionCode} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for insertionCode
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withInsertionCode(String value) {
String newValue = Objects.requireNonNull(value, "insertionCode");
if (this.insertionCode.equals(newValue)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
newValue,
this.x,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#x() x} attribute.
* A value strict bits equality used to prevent copying of the same value by returning {@code this}.
* @param value A new value for x
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withX(double value) {
if (Double.doubleToLongBits(this.x) == Double.doubleToLongBits(value)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
value,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#y() y} attribute.
* A value strict bits equality used to prevent copying of the same value by returning {@code this}.
* @param value A new value for y
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withY(double value) {
if (Double.doubleToLongBits(this.y) == Double.doubleToLongBits(value)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
value,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#z() z} attribute.
* A value strict bits equality used to prevent copying of the same value by returning {@code this}.
* @param value A new value for z
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withZ(double value) {
if (Double.doubleToLongBits(this.z) == Double.doubleToLongBits(value)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
value,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#occupancy() occupancy} attribute.
* A value strict bits equality used to prevent copying of the same value by returning {@code this}.
* @param value A new value for occupancy
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withOccupancy(double value) {
if (Double.doubleToLongBits(this.occupancy) == Double.doubleToLongBits(value)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
this.z,
value,
this.temperatureFactor,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#temperatureFactor() temperatureFactor} attribute.
* A value strict bits equality used to prevent copying of the same value by returning {@code this}.
* @param value A new value for temperatureFactor
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withTemperatureFactor(double value) {
if (Double.doubleToLongBits(this.temperatureFactor) == Double.doubleToLongBits(value)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
this.z,
this.occupancy,
value,
this.elementSymbol,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#elementSymbol() elementSymbol} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for elementSymbol
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withElementSymbol(String value) {
String newValue = Objects.requireNonNull(value, "elementSymbol");
if (this.elementSymbol.equals(newValue)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
newValue,
this.charge);
}
/**
* Copy the current immutable object by setting a value for the {@link PdbAtomLine#charge() charge} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for charge
* @return A modified copy of the {@code this} object
*/
public final ImmutablePdbAtomLine withCharge(String value) {
String newValue = Objects.requireNonNull(value, "charge");
if (this.charge.equals(newValue)) return this;
return new ImmutablePdbAtomLine(
this,
this.serialNumber,
this.atomName,
this.alternateLocation,
this.residueName,
this.chainIdentifier,
this.residueNumber,
this.insertionCode,
this.x,
this.y,
this.z,
this.occupancy,
this.temperatureFactor,
this.elementSymbol,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutablePdbAtomLine} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutablePdbAtomLine
&& equalTo((ImmutablePdbAtomLine) another);
}
private boolean equalTo(ImmutablePdbAtomLine another) {
return atomName.equals(another.atomName)
&& residueName.equals(another.residueName)
&& chainIdentifier.equals(another.chainIdentifier)
&& residueNumber == another.residueNumber
&& insertionCode.equals(another.insertionCode)
&& Double.doubleToLongBits(x) == Double.doubleToLongBits(another.x)
&& Double.doubleToLongBits(y) == Double.doubleToLongBits(another.y)
&& Double.doubleToLongBits(z) == Double.doubleToLongBits(another.z);
}
/**
* Computes a hash code from attributes: {@code atomName}, {@code residueName}, {@code chainIdentifier}, {@code residueNumber}, {@code insertionCode}, {@code x}, {@code y}, {@code z}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + atomName.hashCode();
h += (h << 5) + residueName.hashCode();
h += (h << 5) + chainIdentifier.hashCode();
h += (h << 5) + residueNumber;
h += (h << 5) + insertionCode.hashCode();
h += (h << 5) + Double.hashCode(x);
h += (h << 5) + Double.hashCode(y);
h += (h << 5) + Double.hashCode(z);
return h;
}
/**
* Construct a new immutable {@code PdbAtomLine} instance.
* @param serialNumber The value for the {@code serialNumber} attribute
* @param atomName The value for the {@code atomName} attribute
* @param alternateLocation The value for the {@code alternateLocation} attribute
* @param residueName The value for the {@code residueName} attribute
* @param chainIdentifier The value for the {@code chainIdentifier} attribute
* @param residueNumber The value for the {@code residueNumber} attribute
* @param insertionCode The value for the {@code insertionCode} attribute
* @param x The value for the {@code x} attribute
* @param y The value for the {@code y} attribute
* @param z The value for the {@code z} attribute
* @param occupancy The value for the {@code occupancy} attribute
* @param temperatureFactor The value for the {@code temperatureFactor} attribute
* @param elementSymbol The value for the {@code elementSymbol} attribute
* @param charge The value for the {@code charge} attribute
* @return An immutable PdbAtomLine instance
*/
public static ImmutablePdbAtomLine of(int serialNumber, String atomName, String alternateLocation, String residueName, String chainIdentifier, int residueNumber, String insertionCode, double x, double y, double z, double occupancy, double temperatureFactor, String elementSymbol, String charge) {
return new ImmutablePdbAtomLine(serialNumber, atomName, alternateLocation, residueName, chainIdentifier, residueNumber, insertionCode, x, y, z, occupancy, temperatureFactor, elementSymbol, charge);
}
/**
* Creates an immutable copy of a {@link PdbAtomLine} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable PdbAtomLine instance
*/
public static ImmutablePdbAtomLine copyOf(PdbAtomLine instance) {
if (instance instanceof ImmutablePdbAtomLine) {
return (ImmutablePdbAtomLine) instance;
}
return ImmutablePdbAtomLine.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutablePdbAtomLine ImmutablePdbAtomLine}.
*
* ImmutablePdbAtomLine.builder()
* .serialNumber(int) // required {@link PdbAtomLine#serialNumber() serialNumber}
* .atomName(String) // required {@link PdbAtomLine#atomName() atomName}
* .alternateLocation(String) // required {@link PdbAtomLine#alternateLocation() alternateLocation}
* .residueName(String) // required {@link PdbAtomLine#residueName() residueName}
* .chainIdentifier(String) // required {@link PdbAtomLine#chainIdentifier() chainIdentifier}
* .residueNumber(int) // required {@link PdbAtomLine#residueNumber() residueNumber}
* .insertionCode(String) // required {@link PdbAtomLine#insertionCode() insertionCode}
* .x(double) // required {@link PdbAtomLine#x() x}
* .y(double) // required {@link PdbAtomLine#y() y}
* .z(double) // required {@link PdbAtomLine#z() z}
* .occupancy(double) // required {@link PdbAtomLine#occupancy() occupancy}
* .temperatureFactor(double) // required {@link PdbAtomLine#temperatureFactor() temperatureFactor}
* .elementSymbol(String) // required {@link PdbAtomLine#elementSymbol() elementSymbol}
* .charge(String) // required {@link PdbAtomLine#charge() charge}
* .build();
*
* @return A new ImmutablePdbAtomLine builder
*/
public static ImmutablePdbAtomLine.Builder builder() {
return new ImmutablePdbAtomLine.Builder();
}
/**
* Builds instances of type {@link ImmutablePdbAtomLine ImmutablePdbAtomLine}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "PdbAtomLine", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_SERIAL_NUMBER = 0x1L;
private static final long INIT_BIT_ATOM_NAME = 0x2L;
private static final long INIT_BIT_ALTERNATE_LOCATION = 0x4L;
private static final long INIT_BIT_RESIDUE_NAME = 0x8L;
private static final long INIT_BIT_CHAIN_IDENTIFIER = 0x10L;
private static final long INIT_BIT_RESIDUE_NUMBER = 0x20L;
private static final long INIT_BIT_INSERTION_CODE = 0x40L;
private static final long INIT_BIT_X = 0x80L;
private static final long INIT_BIT_Y = 0x100L;
private static final long INIT_BIT_Z = 0x200L;
private static final long INIT_BIT_OCCUPANCY = 0x400L;
private static final long INIT_BIT_TEMPERATURE_FACTOR = 0x800L;
private static final long INIT_BIT_ELEMENT_SYMBOL = 0x1000L;
private static final long INIT_BIT_CHARGE = 0x2000L;
private long initBits = 0x3fffL;
private int serialNumber;
private @Nullable String atomName;
private @Nullable String alternateLocation;
private @Nullable String residueName;
private @Nullable String chainIdentifier;
private int residueNumber;
private @Nullable String insertionCode;
private double x;
private double y;
private double z;
private double occupancy;
private double temperatureFactor;
private @Nullable String elementSymbol;
private @Nullable String charge;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.pdb.ChainNumberICode} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ChainNumberICode instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.pdb.PdbAtomLine} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(PdbAtomLine instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
long bits = 0;
if (object instanceof ChainNumberICode) {
ChainNumberICode instance = (ChainNumberICode) object;
if ((bits & 0x1L) == 0) {
insertionCode(instance.insertionCode());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
residueNumber(instance.residueNumber());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
chainIdentifier(instance.chainIdentifier());
bits |= 0x4L;
}
}
if (object instanceof PdbAtomLine) {
PdbAtomLine instance = (PdbAtomLine) object;
elementSymbol(instance.elementSymbol());
residueName(instance.residueName());
serialNumber(instance.serialNumber());
charge(instance.charge());
occupancy(instance.occupancy());
if ((bits & 0x1L) == 0) {
insertionCode(instance.insertionCode());
bits |= 0x1L;
}
atomName(instance.atomName());
temperatureFactor(instance.temperatureFactor());
alternateLocation(instance.alternateLocation());
if ((bits & 0x2L) == 0) {
residueNumber(instance.residueNumber());
bits |= 0x2L;
}
x(instance.x());
y(instance.y());
z(instance.z());
if ((bits & 0x4L) == 0) {
chainIdentifier(instance.chainIdentifier());
bits |= 0x4L;
}
}
}
/**
* Initializes the value for the {@link PdbAtomLine#serialNumber() serialNumber} attribute.
* @param serialNumber The value for serialNumber
* @return {@code this} builder for use in a chained invocation
*/
public final Builder serialNumber(int serialNumber) {
this.serialNumber = serialNumber;
initBits &= ~INIT_BIT_SERIAL_NUMBER;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#atomName() atomName} attribute.
* @param atomName The value for atomName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder atomName(String atomName) {
this.atomName = Objects.requireNonNull(atomName, "atomName");
initBits &= ~INIT_BIT_ATOM_NAME;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#alternateLocation() alternateLocation} attribute.
* @param alternateLocation The value for alternateLocation
* @return {@code this} builder for use in a chained invocation
*/
public final Builder alternateLocation(String alternateLocation) {
this.alternateLocation = Objects.requireNonNull(alternateLocation, "alternateLocation");
initBits &= ~INIT_BIT_ALTERNATE_LOCATION;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#residueName() residueName} attribute.
* @param residueName The value for residueName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder residueName(String residueName) {
this.residueName = Objects.requireNonNull(residueName, "residueName");
initBits &= ~INIT_BIT_RESIDUE_NAME;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#chainIdentifier() chainIdentifier} attribute.
* @param chainIdentifier The value for chainIdentifier
* @return {@code this} builder for use in a chained invocation
*/
public final Builder chainIdentifier(String chainIdentifier) {
this.chainIdentifier = Objects.requireNonNull(chainIdentifier, "chainIdentifier");
initBits &= ~INIT_BIT_CHAIN_IDENTIFIER;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#residueNumber() residueNumber} attribute.
* @param residueNumber The value for residueNumber
* @return {@code this} builder for use in a chained invocation
*/
public final Builder residueNumber(int residueNumber) {
this.residueNumber = residueNumber;
initBits &= ~INIT_BIT_RESIDUE_NUMBER;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#insertionCode() insertionCode} attribute.
* @param insertionCode The value for insertionCode
* @return {@code this} builder for use in a chained invocation
*/
public final Builder insertionCode(String insertionCode) {
this.insertionCode = Objects.requireNonNull(insertionCode, "insertionCode");
initBits &= ~INIT_BIT_INSERTION_CODE;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#x() x} attribute.
* @param x The value for x
* @return {@code this} builder for use in a chained invocation
*/
public final Builder x(double x) {
this.x = x;
initBits &= ~INIT_BIT_X;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#y() y} attribute.
* @param y The value for y
* @return {@code this} builder for use in a chained invocation
*/
public final Builder y(double y) {
this.y = y;
initBits &= ~INIT_BIT_Y;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#z() z} attribute.
* @param z The value for z
* @return {@code this} builder for use in a chained invocation
*/
public final Builder z(double z) {
this.z = z;
initBits &= ~INIT_BIT_Z;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#occupancy() occupancy} attribute.
* @param occupancy The value for occupancy
* @return {@code this} builder for use in a chained invocation
*/
public final Builder occupancy(double occupancy) {
this.occupancy = occupancy;
initBits &= ~INIT_BIT_OCCUPANCY;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#temperatureFactor() temperatureFactor} attribute.
* @param temperatureFactor The value for temperatureFactor
* @return {@code this} builder for use in a chained invocation
*/
public final Builder temperatureFactor(double temperatureFactor) {
this.temperatureFactor = temperatureFactor;
initBits &= ~INIT_BIT_TEMPERATURE_FACTOR;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#elementSymbol() elementSymbol} attribute.
* @param elementSymbol The value for elementSymbol
* @return {@code this} builder for use in a chained invocation
*/
public final Builder elementSymbol(String elementSymbol) {
this.elementSymbol = Objects.requireNonNull(elementSymbol, "elementSymbol");
initBits &= ~INIT_BIT_ELEMENT_SYMBOL;
return this;
}
/**
* Initializes the value for the {@link PdbAtomLine#charge() charge} attribute.
* @param charge The value for charge
* @return {@code this} builder for use in a chained invocation
*/
public final Builder charge(String charge) {
this.charge = Objects.requireNonNull(charge, "charge");
initBits &= ~INIT_BIT_CHARGE;
return this;
}
/**
* Builds a new {@link ImmutablePdbAtomLine ImmutablePdbAtomLine}.
* @return An immutable instance of PdbAtomLine
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutablePdbAtomLine build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutablePdbAtomLine(
null,
serialNumber,
atomName,
alternateLocation,
residueName,
chainIdentifier,
residueNumber,
insertionCode,
x,
y,
z,
occupancy,
temperatureFactor,
elementSymbol,
charge);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_SERIAL_NUMBER) != 0) attributes.add("serialNumber");
if ((initBits & INIT_BIT_ATOM_NAME) != 0) attributes.add("atomName");
if ((initBits & INIT_BIT_ALTERNATE_LOCATION) != 0) attributes.add("alternateLocation");
if ((initBits & INIT_BIT_RESIDUE_NAME) != 0) attributes.add("residueName");
if ((initBits & INIT_BIT_CHAIN_IDENTIFIER) != 0) attributes.add("chainIdentifier");
if ((initBits & INIT_BIT_RESIDUE_NUMBER) != 0) attributes.add("residueNumber");
if ((initBits & INIT_BIT_INSERTION_CODE) != 0) attributes.add("insertionCode");
if ((initBits & INIT_BIT_X) != 0) attributes.add("x");
if ((initBits & INIT_BIT_Y) != 0) attributes.add("y");
if ((initBits & INIT_BIT_Z) != 0) attributes.add("z");
if ((initBits & INIT_BIT_OCCUPANCY) != 0) attributes.add("occupancy");
if ((initBits & INIT_BIT_TEMPERATURE_FACTOR) != 0) attributes.add("temperatureFactor");
if ((initBits & INIT_BIT_ELEMENT_SYMBOL) != 0) attributes.add("elementSymbol");
if ((initBits & INIT_BIT_CHARGE) != 0) attributes.add("charge");
return "Cannot build PdbAtomLine, some of required attributes are not set " + attributes;
}
}
}