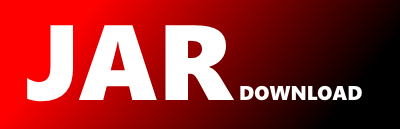
pl.poznan.put.pdb.analysis.ImmutableDefaultPdbModel Maven / Gradle / Ivy
package pl.poznan.put.pdb.analysis;
import java.io.ObjectStreamException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import pl.poznan.put.pdb.PdbAtomLine;
import pl.poznan.put.pdb.PdbExpdtaLine;
import pl.poznan.put.pdb.PdbHeaderLine;
import pl.poznan.put.pdb.PdbModresLine;
import pl.poznan.put.pdb.PdbRemark2Line;
import pl.poznan.put.pdb.PdbRemark465Line;
import pl.poznan.put.pdb.PdbResidueIdentifier;
/**
* Immutable implementation of {@link DefaultPdbModel}.
*
* Use the builder to create immutable instances:
* {@code ImmutableDefaultPdbModel.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableDefaultPdbModel.of()}.
*/
@Generated(from = "DefaultPdbModel", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableDefaultPdbModel extends DefaultPdbModel {
private final PdbHeaderLine header;
private final PdbExpdtaLine experimentalData;
private final PdbRemark2Line resolution;
private final int modelNumber;
private final List atoms;
private final List modifiedResidues;
private final List missingResidues;
private final String title;
private final Set chainTerminatedAfter;
private ImmutableDefaultPdbModel(
PdbHeaderLine header,
PdbExpdtaLine experimentalData,
PdbRemark2Line resolution,
int modelNumber,
Iterable extends PdbAtomLine> atoms,
Iterable extends PdbModresLine> modifiedResidues,
Iterable extends PdbRemark465Line> missingResidues,
String title,
Iterable extends PdbResidueIdentifier> chainTerminatedAfter) {
this.header = Objects.requireNonNull(header, "header");
this.experimentalData = Objects.requireNonNull(experimentalData, "experimentalData");
this.resolution = Objects.requireNonNull(resolution, "resolution");
this.modelNumber = modelNumber;
this.atoms = createUnmodifiableList(false, createSafeList(atoms, true, false));
this.modifiedResidues = createUnmodifiableList(false, createSafeList(modifiedResidues, true, false));
this.missingResidues = createUnmodifiableList(false, createSafeList(missingResidues, true, false));
this.title = Objects.requireNonNull(title, "title");
this.chainTerminatedAfter = createUnmodifiableSet(createSafeList(chainTerminatedAfter, true, false));
}
private ImmutableDefaultPdbModel(
ImmutableDefaultPdbModel original,
PdbHeaderLine header,
PdbExpdtaLine experimentalData,
PdbRemark2Line resolution,
int modelNumber,
List atoms,
List modifiedResidues,
List missingResidues,
String title,
Set chainTerminatedAfter) {
this.header = header;
this.experimentalData = experimentalData;
this.resolution = resolution;
this.modelNumber = modelNumber;
this.atoms = atoms;
this.modifiedResidues = modifiedResidues;
this.missingResidues = missingResidues;
this.title = title;
this.chainTerminatedAfter = chainTerminatedAfter;
}
/**
* @return The value of the {@code header} attribute
*/
@Override
public PdbHeaderLine header() {
return header;
}
/**
* @return The value of the {@code experimentalData} attribute
*/
@Override
public PdbExpdtaLine experimentalData() {
return experimentalData;
}
/**
* @return The value of the {@code resolution} attribute
*/
@Override
public PdbRemark2Line resolution() {
return resolution;
}
/**
* @return The value of the {@code modelNumber} attribute
*/
@Override
public int modelNumber() {
return modelNumber;
}
/**
* @return The value of the {@code atoms} attribute
*/
@Override
public List atoms() {
return atoms;
}
/**
* @return The value of the {@code modifiedResidues} attribute
*/
@Override
public List modifiedResidues() {
return modifiedResidues;
}
/**
* @return The value of the {@code missingResidues} attribute
*/
@Override
public List missingResidues() {
return missingResidues;
}
/**
* @return The value of the {@code title} attribute
*/
@Override
public String title() {
return title;
}
/**
* @return The value of the {@code chainTerminatedAfter} attribute
*/
@Override
public Set chainTerminatedAfter() {
return chainTerminatedAfter;
}
/**
* Copy the current immutable object by setting a value for the {@link DefaultPdbModel#header() header} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for header
* @return A modified copy of the {@code this} object
*/
public final ImmutableDefaultPdbModel withHeader(PdbHeaderLine value) {
if (this.header == value) return this;
PdbHeaderLine newValue = Objects.requireNonNull(value, "header");
return validate(new ImmutableDefaultPdbModel(
this,
newValue,
this.experimentalData,
this.resolution,
this.modelNumber,
this.atoms,
this.modifiedResidues,
this.missingResidues,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object by setting a value for the {@link DefaultPdbModel#experimentalData() experimentalData} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for experimentalData
* @return A modified copy of the {@code this} object
*/
public final ImmutableDefaultPdbModel withExperimentalData(PdbExpdtaLine value) {
if (this.experimentalData == value) return this;
PdbExpdtaLine newValue = Objects.requireNonNull(value, "experimentalData");
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
newValue,
this.resolution,
this.modelNumber,
this.atoms,
this.modifiedResidues,
this.missingResidues,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object by setting a value for the {@link DefaultPdbModel#resolution() resolution} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for resolution
* @return A modified copy of the {@code this} object
*/
public final ImmutableDefaultPdbModel withResolution(PdbRemark2Line value) {
if (this.resolution == value) return this;
PdbRemark2Line newValue = Objects.requireNonNull(value, "resolution");
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
newValue,
this.modelNumber,
this.atoms,
this.modifiedResidues,
this.missingResidues,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object by setting a value for the {@link DefaultPdbModel#modelNumber() modelNumber} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for modelNumber
* @return A modified copy of the {@code this} object
*/
public final ImmutableDefaultPdbModel withModelNumber(int value) {
if (this.modelNumber == value) return this;
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
value,
this.atoms,
this.modifiedResidues,
this.missingResidues,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultPdbModel#atoms() atoms}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultPdbModel withAtoms(PdbAtomLine... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
this.modelNumber,
newValue,
this.modifiedResidues,
this.missingResidues,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultPdbModel#atoms() atoms}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of atoms elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultPdbModel withAtoms(Iterable extends PdbAtomLine> elements) {
if (this.atoms == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
this.modelNumber,
newValue,
this.modifiedResidues,
this.missingResidues,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultPdbModel#modifiedResidues() modifiedResidues}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultPdbModel withModifiedResidues(PdbModresLine... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
this.modelNumber,
this.atoms,
newValue,
this.missingResidues,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultPdbModel#modifiedResidues() modifiedResidues}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of modifiedResidues elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultPdbModel withModifiedResidues(Iterable extends PdbModresLine> elements) {
if (this.modifiedResidues == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
this.modelNumber,
this.atoms,
newValue,
this.missingResidues,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultPdbModel#missingResidues() missingResidues}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultPdbModel withMissingResidues(PdbRemark465Line... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
this.modelNumber,
this.atoms,
this.modifiedResidues,
newValue,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultPdbModel#missingResidues() missingResidues}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of missingResidues elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultPdbModel withMissingResidues(Iterable extends PdbRemark465Line> elements) {
if (this.missingResidues == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
this.modelNumber,
this.atoms,
this.modifiedResidues,
newValue,
this.title,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object by setting a value for the {@link DefaultPdbModel#title() title} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for title
* @return A modified copy of the {@code this} object
*/
public final ImmutableDefaultPdbModel withTitle(String value) {
String newValue = Objects.requireNonNull(value, "title");
if (this.title.equals(newValue)) return this;
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
this.modelNumber,
this.atoms,
this.modifiedResidues,
this.missingResidues,
newValue,
this.chainTerminatedAfter));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultPdbModel#chainTerminatedAfter() chainTerminatedAfter}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultPdbModel withChainTerminatedAfter(PdbResidueIdentifier... elements) {
Set newValue = createUnmodifiableSet(createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
this.modelNumber,
this.atoms,
this.modifiedResidues,
this.missingResidues,
this.title,
newValue));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultPdbModel#chainTerminatedAfter() chainTerminatedAfter}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of chainTerminatedAfter elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultPdbModel withChainTerminatedAfter(Iterable extends PdbResidueIdentifier> elements) {
if (this.chainTerminatedAfter == elements) return this;
Set newValue = createUnmodifiableSet(createSafeList(elements, true, false));
return validate(new ImmutableDefaultPdbModel(
this,
this.header,
this.experimentalData,
this.resolution,
this.modelNumber,
this.atoms,
this.modifiedResidues,
this.missingResidues,
this.title,
newValue));
}
/**
* This instance is equal to all instances of {@code ImmutableDefaultPdbModel} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableDefaultPdbModel
&& equalTo((ImmutableDefaultPdbModel) another);
}
private boolean equalTo(ImmutableDefaultPdbModel another) {
return atoms.equals(another.atoms);
}
/**
* Computes a hash code from attributes: {@code atoms}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + atoms.hashCode();
return h;
}
/**
* Prints the immutable value {@code DefaultPdbModel} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "DefaultPdbModel{"
+ "atoms=" + atoms
+ "}";
}
private transient volatile long lazyInitBitmap;
private static final long CHAINS_LAZY_INIT_BIT = 0x1L;
private transient List chains;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link DefaultPdbModel#chains() chains} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code chains} attribute
*/
@Override
public List chains() {
if ((lazyInitBitmap & CHAINS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & CHAINS_LAZY_INIT_BIT) == 0) {
this.chains = Objects.requireNonNull(super.chains(), "chains");
lazyInitBitmap |= CHAINS_LAZY_INIT_BIT;
}
}
}
return chains;
}
private static final long RESIDUES_LAZY_INIT_BIT = 0x2L;
private transient List residues;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link DefaultPdbModel#residues() residues} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code residues} attribute
*/
@Override
public List residues() {
if ((lazyInitBitmap & RESIDUES_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & RESIDUES_LAZY_INIT_BIT) == 0) {
this.residues = Objects.requireNonNull(super.residues(), "residues");
lazyInitBitmap |= RESIDUES_LAZY_INIT_BIT;
}
}
}
return residues;
}
/**
* Construct a new immutable {@code DefaultPdbModel} instance.
* @param header The value for the {@code header} attribute
* @param experimentalData The value for the {@code experimentalData} attribute
* @param resolution The value for the {@code resolution} attribute
* @param modelNumber The value for the {@code modelNumber} attribute
* @param atoms The value for the {@code atoms} attribute
* @param modifiedResidues The value for the {@code modifiedResidues} attribute
* @param missingResidues The value for the {@code missingResidues} attribute
* @param title The value for the {@code title} attribute
* @param chainTerminatedAfter The value for the {@code chainTerminatedAfter} attribute
* @return An immutable DefaultPdbModel instance
*/
public static ImmutableDefaultPdbModel of(PdbHeaderLine header, PdbExpdtaLine experimentalData, PdbRemark2Line resolution, int modelNumber, List atoms, List modifiedResidues, List missingResidues, String title, Set chainTerminatedAfter) {
return of(header, experimentalData, resolution, modelNumber, (Iterable extends PdbAtomLine>) atoms, (Iterable extends PdbModresLine>) modifiedResidues, (Iterable extends PdbRemark465Line>) missingResidues, title, (Iterable extends PdbResidueIdentifier>) chainTerminatedAfter);
}
/**
* Construct a new immutable {@code DefaultPdbModel} instance.
* @param header The value for the {@code header} attribute
* @param experimentalData The value for the {@code experimentalData} attribute
* @param resolution The value for the {@code resolution} attribute
* @param modelNumber The value for the {@code modelNumber} attribute
* @param atoms The value for the {@code atoms} attribute
* @param modifiedResidues The value for the {@code modifiedResidues} attribute
* @param missingResidues The value for the {@code missingResidues} attribute
* @param title The value for the {@code title} attribute
* @param chainTerminatedAfter The value for the {@code chainTerminatedAfter} attribute
* @return An immutable DefaultPdbModel instance
*/
public static ImmutableDefaultPdbModel of(PdbHeaderLine header, PdbExpdtaLine experimentalData, PdbRemark2Line resolution, int modelNumber, Iterable extends PdbAtomLine> atoms, Iterable extends PdbModresLine> modifiedResidues, Iterable extends PdbRemark465Line> missingResidues, String title, Iterable extends PdbResidueIdentifier> chainTerminatedAfter) {
return validate(new ImmutableDefaultPdbModel(header, experimentalData, resolution, modelNumber, atoms, modifiedResidues, missingResidues, title, chainTerminatedAfter));
}
private static ImmutableDefaultPdbModel validate(ImmutableDefaultPdbModel instance) {
instance.check();
return instance;
}
/**
* Creates an immutable copy of a {@link DefaultPdbModel} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable DefaultPdbModel instance
*/
public static ImmutableDefaultPdbModel copyOf(DefaultPdbModel instance) {
if (instance instanceof ImmutableDefaultPdbModel) {
return (ImmutableDefaultPdbModel) instance;
}
return ImmutableDefaultPdbModel.builder()
.from(instance)
.build();
}
private Object readResolve() throws ObjectStreamException {
return validate(this);
}
/**
* Creates a builder for {@link ImmutableDefaultPdbModel ImmutableDefaultPdbModel}.
*
* ImmutableDefaultPdbModel.builder()
* .header(pl.poznan.put.pdb.PdbHeaderLine) // required {@link DefaultPdbModel#header() header}
* .experimentalData(pl.poznan.put.pdb.PdbExpdtaLine) // required {@link DefaultPdbModel#experimentalData() experimentalData}
* .resolution(pl.poznan.put.pdb.PdbRemark2Line) // required {@link DefaultPdbModel#resolution() resolution}
* .modelNumber(int) // required {@link DefaultPdbModel#modelNumber() modelNumber}
* .addAtoms|addAllAtoms(pl.poznan.put.pdb.PdbAtomLine) // {@link DefaultPdbModel#atoms() atoms} elements
* .addModifiedResidues|addAllModifiedResidues(pl.poznan.put.pdb.PdbModresLine) // {@link DefaultPdbModel#modifiedResidues() modifiedResidues} elements
* .addMissingResidues|addAllMissingResidues(pl.poznan.put.pdb.PdbRemark465Line) // {@link DefaultPdbModel#missingResidues() missingResidues} elements
* .title(String) // required {@link DefaultPdbModel#title() title}
* .addChainTerminatedAfter|addAllChainTerminatedAfter(pl.poznan.put.pdb.PdbResidueIdentifier) // {@link DefaultPdbModel#chainTerminatedAfter() chainTerminatedAfter} elements
* .build();
*
* @return A new ImmutableDefaultPdbModel builder
*/
public static ImmutableDefaultPdbModel.Builder builder() {
return new ImmutableDefaultPdbModel.Builder();
}
/**
* Builds instances of type {@link ImmutableDefaultPdbModel ImmutableDefaultPdbModel}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "DefaultPdbModel", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_HEADER = 0x1L;
private static final long INIT_BIT_EXPERIMENTAL_DATA = 0x2L;
private static final long INIT_BIT_RESOLUTION = 0x4L;
private static final long INIT_BIT_MODEL_NUMBER = 0x8L;
private static final long INIT_BIT_TITLE = 0x10L;
private long initBits = 0x1fL;
private @Nullable PdbHeaderLine header;
private @Nullable PdbExpdtaLine experimentalData;
private @Nullable PdbRemark2Line resolution;
private int modelNumber;
private List atoms = new ArrayList();
private List modifiedResidues = new ArrayList();
private List missingResidues = new ArrayList();
private @Nullable String title;
private List chainTerminatedAfter = new ArrayList();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.pdb.analysis.PdbModel} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(PdbModel instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.pdb.analysis.DefaultPdbModel} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(DefaultPdbModel instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
long bits = 0;
if (object instanceof PdbModel) {
PdbModel instance = (PdbModel) object;
if ((bits & 0x1L) == 0) {
addAllChainTerminatedAfter(instance.chainTerminatedAfter());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
addAllModifiedResidues(instance.modifiedResidues());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
addAllMissingResidues(instance.missingResidues());
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
experimentalData(instance.experimentalData());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
header(instance.header());
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
modelNumber(instance.modelNumber());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
title(instance.title());
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
resolution(instance.resolution());
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
addAllAtoms(instance.atoms());
bits |= 0x100L;
}
}
if (object instanceof DefaultPdbModel) {
DefaultPdbModel instance = (DefaultPdbModel) object;
if ((bits & 0x1L) == 0) {
addAllChainTerminatedAfter(instance.chainTerminatedAfter());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
addAllModifiedResidues(instance.modifiedResidues());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
addAllMissingResidues(instance.missingResidues());
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
experimentalData(instance.experimentalData());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
header(instance.header());
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
modelNumber(instance.modelNumber());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
title(instance.title());
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
resolution(instance.resolution());
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
addAllAtoms(instance.atoms());
bits |= 0x100L;
}
}
}
/**
* Initializes the value for the {@link DefaultPdbModel#header() header} attribute.
* @param header The value for header
* @return {@code this} builder for use in a chained invocation
*/
public final Builder header(PdbHeaderLine header) {
this.header = Objects.requireNonNull(header, "header");
initBits &= ~INIT_BIT_HEADER;
return this;
}
/**
* Initializes the value for the {@link DefaultPdbModel#experimentalData() experimentalData} attribute.
* @param experimentalData The value for experimentalData
* @return {@code this} builder for use in a chained invocation
*/
public final Builder experimentalData(PdbExpdtaLine experimentalData) {
this.experimentalData = Objects.requireNonNull(experimentalData, "experimentalData");
initBits &= ~INIT_BIT_EXPERIMENTAL_DATA;
return this;
}
/**
* Initializes the value for the {@link DefaultPdbModel#resolution() resolution} attribute.
* @param resolution The value for resolution
* @return {@code this} builder for use in a chained invocation
*/
public final Builder resolution(PdbRemark2Line resolution) {
this.resolution = Objects.requireNonNull(resolution, "resolution");
initBits &= ~INIT_BIT_RESOLUTION;
return this;
}
/**
* Initializes the value for the {@link DefaultPdbModel#modelNumber() modelNumber} attribute.
* @param modelNumber The value for modelNumber
* @return {@code this} builder for use in a chained invocation
*/
public final Builder modelNumber(int modelNumber) {
this.modelNumber = modelNumber;
initBits &= ~INIT_BIT_MODEL_NUMBER;
return this;
}
/**
* Adds one element to {@link DefaultPdbModel#atoms() atoms} list.
* @param element A atoms element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAtoms(PdbAtomLine element) {
this.atoms.add(Objects.requireNonNull(element, "atoms element"));
return this;
}
/**
* Adds elements to {@link DefaultPdbModel#atoms() atoms} list.
* @param elements An array of atoms elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAtoms(PdbAtomLine... elements) {
for (PdbAtomLine element : elements) {
this.atoms.add(Objects.requireNonNull(element, "atoms element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link DefaultPdbModel#atoms() atoms} list.
* @param elements An iterable of atoms elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder atoms(Iterable extends PdbAtomLine> elements) {
this.atoms.clear();
return addAllAtoms(elements);
}
/**
* Adds elements to {@link DefaultPdbModel#atoms() atoms} list.
* @param elements An iterable of atoms elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllAtoms(Iterable extends PdbAtomLine> elements) {
for (PdbAtomLine element : elements) {
this.atoms.add(Objects.requireNonNull(element, "atoms element"));
}
return this;
}
/**
* Adds one element to {@link DefaultPdbModel#modifiedResidues() modifiedResidues} list.
* @param element A modifiedResidues element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addModifiedResidues(PdbModresLine element) {
this.modifiedResidues.add(Objects.requireNonNull(element, "modifiedResidues element"));
return this;
}
/**
* Adds elements to {@link DefaultPdbModel#modifiedResidues() modifiedResidues} list.
* @param elements An array of modifiedResidues elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addModifiedResidues(PdbModresLine... elements) {
for (PdbModresLine element : elements) {
this.modifiedResidues.add(Objects.requireNonNull(element, "modifiedResidues element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link DefaultPdbModel#modifiedResidues() modifiedResidues} list.
* @param elements An iterable of modifiedResidues elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder modifiedResidues(Iterable extends PdbModresLine> elements) {
this.modifiedResidues.clear();
return addAllModifiedResidues(elements);
}
/**
* Adds elements to {@link DefaultPdbModel#modifiedResidues() modifiedResidues} list.
* @param elements An iterable of modifiedResidues elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllModifiedResidues(Iterable extends PdbModresLine> elements) {
for (PdbModresLine element : elements) {
this.modifiedResidues.add(Objects.requireNonNull(element, "modifiedResidues element"));
}
return this;
}
/**
* Adds one element to {@link DefaultPdbModel#missingResidues() missingResidues} list.
* @param element A missingResidues element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMissingResidues(PdbRemark465Line element) {
this.missingResidues.add(Objects.requireNonNull(element, "missingResidues element"));
return this;
}
/**
* Adds elements to {@link DefaultPdbModel#missingResidues() missingResidues} list.
* @param elements An array of missingResidues elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMissingResidues(PdbRemark465Line... elements) {
for (PdbRemark465Line element : elements) {
this.missingResidues.add(Objects.requireNonNull(element, "missingResidues element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link DefaultPdbModel#missingResidues() missingResidues} list.
* @param elements An iterable of missingResidues elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder missingResidues(Iterable extends PdbRemark465Line> elements) {
this.missingResidues.clear();
return addAllMissingResidues(elements);
}
/**
* Adds elements to {@link DefaultPdbModel#missingResidues() missingResidues} list.
* @param elements An iterable of missingResidues elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllMissingResidues(Iterable extends PdbRemark465Line> elements) {
for (PdbRemark465Line element : elements) {
this.missingResidues.add(Objects.requireNonNull(element, "missingResidues element"));
}
return this;
}
/**
* Initializes the value for the {@link DefaultPdbModel#title() title} attribute.
* @param title The value for title
* @return {@code this} builder for use in a chained invocation
*/
public final Builder title(String title) {
this.title = Objects.requireNonNull(title, "title");
initBits &= ~INIT_BIT_TITLE;
return this;
}
/**
* Adds one element to {@link DefaultPdbModel#chainTerminatedAfter() chainTerminatedAfter} set.
* @param element A chainTerminatedAfter element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addChainTerminatedAfter(PdbResidueIdentifier element) {
this.chainTerminatedAfter.add(Objects.requireNonNull(element, "chainTerminatedAfter element"));
return this;
}
/**
* Adds elements to {@link DefaultPdbModel#chainTerminatedAfter() chainTerminatedAfter} set.
* @param elements An array of chainTerminatedAfter elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addChainTerminatedAfter(PdbResidueIdentifier... elements) {
for (PdbResidueIdentifier element : elements) {
this.chainTerminatedAfter.add(Objects.requireNonNull(element, "chainTerminatedAfter element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link DefaultPdbModel#chainTerminatedAfter() chainTerminatedAfter} set.
* @param elements An iterable of chainTerminatedAfter elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder chainTerminatedAfter(Iterable extends PdbResidueIdentifier> elements) {
this.chainTerminatedAfter.clear();
return addAllChainTerminatedAfter(elements);
}
/**
* Adds elements to {@link DefaultPdbModel#chainTerminatedAfter() chainTerminatedAfter} set.
* @param elements An iterable of chainTerminatedAfter elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllChainTerminatedAfter(Iterable extends PdbResidueIdentifier> elements) {
for (PdbResidueIdentifier element : elements) {
this.chainTerminatedAfter.add(Objects.requireNonNull(element, "chainTerminatedAfter element"));
}
return this;
}
/**
* Builds a new {@link ImmutableDefaultPdbModel ImmutableDefaultPdbModel}.
* @return An immutable instance of DefaultPdbModel
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableDefaultPdbModel build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return ImmutableDefaultPdbModel.validate(new ImmutableDefaultPdbModel(
null,
header,
experimentalData,
resolution,
modelNumber,
createUnmodifiableList(true, atoms),
createUnmodifiableList(true, modifiedResidues),
createUnmodifiableList(true, missingResidues),
title,
createUnmodifiableSet(chainTerminatedAfter)));
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_HEADER) != 0) attributes.add("header");
if ((initBits & INIT_BIT_EXPERIMENTAL_DATA) != 0) attributes.add("experimentalData");
if ((initBits & INIT_BIT_RESOLUTION) != 0) attributes.add("resolution");
if ((initBits & INIT_BIT_MODEL_NUMBER) != 0) attributes.add("modelNumber");
if ((initBits & INIT_BIT_TITLE) != 0) attributes.add("title");
return "Cannot build DefaultPdbModel, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
/** Unmodifiable set constructed from list to avoid rehashing. */
private static Set createUnmodifiableSet(List list) {
switch(list.size()) {
case 0: return Collections.emptySet();
case 1: return Collections.singleton(list.get(0));
default:
Set set = new LinkedHashSet<>(list.size());
set.addAll(list);
return Collections.unmodifiableSet(set);
}
}
}