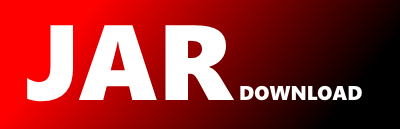
pl.poznan.put.structure.ImmutableAnalyzedBasePair Maven / Gradle / Ivy
package pl.poznan.put.structure;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import pl.poznan.put.notation.BPh;
import pl.poznan.put.notation.BR;
import pl.poznan.put.notation.LeontisWesthof;
import pl.poznan.put.notation.Saenger;
import pl.poznan.put.rna.InteractionType;
/**
* Immutable implementation of {@link AnalyzedBasePair}.
*
* Use the builder to create immutable instances:
* {@code ImmutableAnalyzedBasePair.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableAnalyzedBasePair.of()}.
*/
@Generated(from = "AnalyzedBasePair", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableAnalyzedBasePair extends AnalyzedBasePair {
private final BasePair basePair;
private final InteractionType interactionType;
private final Saenger saenger;
private final LeontisWesthof leontisWesthof;
private final BPh bph;
private final BR br;
private final boolean isRepresented;
private ImmutableAnalyzedBasePair(BasePair basePair) {
this.basePair = Objects.requireNonNull(basePair, "basePair");
this.interactionType = initShim.interactionType();
this.saenger = initShim.saenger();
this.leontisWesthof = initShim.leontisWesthof();
this.bph = initShim.bph();
this.br = initShim.br();
this.isRepresented = initShim.isRepresented();
this.initShim = null;
}
private ImmutableAnalyzedBasePair(ImmutableAnalyzedBasePair.Builder builder) {
this.basePair = builder.basePair;
if (builder.interactionType != null) {
initShim.interactionType(builder.interactionType);
}
if (builder.saenger != null) {
initShim.saenger(builder.saenger);
}
if (builder.leontisWesthof != null) {
initShim.leontisWesthof(builder.leontisWesthof);
}
if (builder.bph != null) {
initShim.bph(builder.bph);
}
if (builder.br != null) {
initShim.br(builder.br);
}
if (builder.isRepresentedIsSet()) {
initShim.isRepresented(builder.isRepresented);
}
this.interactionType = initShim.interactionType();
this.saenger = initShim.saenger();
this.leontisWesthof = initShim.leontisWesthof();
this.bph = initShim.bph();
this.br = initShim.br();
this.isRepresented = initShim.isRepresented();
this.initShim = null;
}
private ImmutableAnalyzedBasePair(
BasePair basePair,
InteractionType interactionType,
Saenger saenger,
LeontisWesthof leontisWesthof,
BPh bph,
BR br,
boolean isRepresented) {
this.basePair = basePair;
this.interactionType = interactionType;
this.saenger = saenger;
this.leontisWesthof = leontisWesthof;
this.bph = bph;
this.br = br;
this.isRepresented = isRepresented;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "AnalyzedBasePair", generator = "Immutables")
private final class InitShim {
private byte interactionTypeBuildStage = STAGE_UNINITIALIZED;
private InteractionType interactionType;
InteractionType interactionType() {
if (interactionTypeBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (interactionTypeBuildStage == STAGE_UNINITIALIZED) {
interactionTypeBuildStage = STAGE_INITIALIZING;
this.interactionType = Objects.requireNonNull(ImmutableAnalyzedBasePair.super.interactionType(), "interactionType");
interactionTypeBuildStage = STAGE_INITIALIZED;
}
return this.interactionType;
}
void interactionType(InteractionType interactionType) {
this.interactionType = interactionType;
interactionTypeBuildStage = STAGE_INITIALIZED;
}
private byte saengerBuildStage = STAGE_UNINITIALIZED;
private Saenger saenger;
Saenger saenger() {
if (saengerBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (saengerBuildStage == STAGE_UNINITIALIZED) {
saengerBuildStage = STAGE_INITIALIZING;
this.saenger = Objects.requireNonNull(ImmutableAnalyzedBasePair.super.saenger(), "saenger");
saengerBuildStage = STAGE_INITIALIZED;
}
return this.saenger;
}
void saenger(Saenger saenger) {
this.saenger = saenger;
saengerBuildStage = STAGE_INITIALIZED;
}
private byte leontisWesthofBuildStage = STAGE_UNINITIALIZED;
private LeontisWesthof leontisWesthof;
LeontisWesthof leontisWesthof() {
if (leontisWesthofBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (leontisWesthofBuildStage == STAGE_UNINITIALIZED) {
leontisWesthofBuildStage = STAGE_INITIALIZING;
this.leontisWesthof = Objects.requireNonNull(ImmutableAnalyzedBasePair.super.leontisWesthof(), "leontisWesthof");
leontisWesthofBuildStage = STAGE_INITIALIZED;
}
return this.leontisWesthof;
}
void leontisWesthof(LeontisWesthof leontisWesthof) {
this.leontisWesthof = leontisWesthof;
leontisWesthofBuildStage = STAGE_INITIALIZED;
}
private byte bphBuildStage = STAGE_UNINITIALIZED;
private BPh bph;
BPh bph() {
if (bphBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (bphBuildStage == STAGE_UNINITIALIZED) {
bphBuildStage = STAGE_INITIALIZING;
this.bph = Objects.requireNonNull(ImmutableAnalyzedBasePair.super.bph(), "bph");
bphBuildStage = STAGE_INITIALIZED;
}
return this.bph;
}
void bph(BPh bph) {
this.bph = bph;
bphBuildStage = STAGE_INITIALIZED;
}
private byte brBuildStage = STAGE_UNINITIALIZED;
private BR br;
BR br() {
if (brBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (brBuildStage == STAGE_UNINITIALIZED) {
brBuildStage = STAGE_INITIALIZING;
this.br = Objects.requireNonNull(ImmutableAnalyzedBasePair.super.br(), "br");
brBuildStage = STAGE_INITIALIZED;
}
return this.br;
}
void br(BR br) {
this.br = br;
brBuildStage = STAGE_INITIALIZED;
}
private byte isRepresentedBuildStage = STAGE_UNINITIALIZED;
private boolean isRepresented;
boolean isRepresented() {
if (isRepresentedBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (isRepresentedBuildStage == STAGE_UNINITIALIZED) {
isRepresentedBuildStage = STAGE_INITIALIZING;
this.isRepresented = ImmutableAnalyzedBasePair.super.isRepresented();
isRepresentedBuildStage = STAGE_INITIALIZED;
}
return this.isRepresented;
}
void isRepresented(boolean isRepresented) {
this.isRepresented = isRepresented;
isRepresentedBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (interactionTypeBuildStage == STAGE_INITIALIZING) attributes.add("interactionType");
if (saengerBuildStage == STAGE_INITIALIZING) attributes.add("saenger");
if (leontisWesthofBuildStage == STAGE_INITIALIZING) attributes.add("leontisWesthof");
if (bphBuildStage == STAGE_INITIALIZING) attributes.add("bph");
if (brBuildStage == STAGE_INITIALIZING) attributes.add("br");
if (isRepresentedBuildStage == STAGE_INITIALIZING) attributes.add("isRepresented");
return "Cannot build AnalyzedBasePair, attribute initializers form cycle " + attributes;
}
}
/**
* @return The value of the {@code basePair} attribute
*/
@Override
public BasePair basePair() {
return basePair;
}
/**
* @return The value of the {@code interactionType} attribute
*/
@Override
public InteractionType interactionType() {
InitShim shim = this.initShim;
return shim != null
? shim.interactionType()
: this.interactionType;
}
/**
* @return The value of the {@code saenger} attribute
*/
@Override
public Saenger saenger() {
InitShim shim = this.initShim;
return shim != null
? shim.saenger()
: this.saenger;
}
/**
* @return The value of the {@code leontisWesthof} attribute
*/
@Override
public LeontisWesthof leontisWesthof() {
InitShim shim = this.initShim;
return shim != null
? shim.leontisWesthof()
: this.leontisWesthof;
}
/**
* @return The value of the {@code bph} attribute
*/
@Override
public BPh bph() {
InitShim shim = this.initShim;
return shim != null
? shim.bph()
: this.bph;
}
/**
* @return The value of the {@code br} attribute
*/
@Override
public BR br() {
InitShim shim = this.initShim;
return shim != null
? shim.br()
: this.br;
}
/**
* @return The value of the {@code isRepresented} attribute
*/
@Override
public boolean isRepresented() {
InitShim shim = this.initShim;
return shim != null
? shim.isRepresented()
: this.isRepresented;
}
/**
* Copy the current immutable object by setting a value for the {@link AnalyzedBasePair#basePair() basePair} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for basePair
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnalyzedBasePair withBasePair(BasePair value) {
if (this.basePair == value) return this;
BasePair newValue = Objects.requireNonNull(value, "basePair");
return new ImmutableAnalyzedBasePair(
newValue,
this.interactionType,
this.saenger,
this.leontisWesthof,
this.bph,
this.br,
this.isRepresented);
}
/**
* Copy the current immutable object by setting a value for the {@link AnalyzedBasePair#interactionType() interactionType} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for interactionType
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnalyzedBasePair withInteractionType(InteractionType value) {
if (this.interactionType == value) return this;
InteractionType newValue = Objects.requireNonNull(value, "interactionType");
return new ImmutableAnalyzedBasePair(
this.basePair,
newValue,
this.saenger,
this.leontisWesthof,
this.bph,
this.br,
this.isRepresented);
}
/**
* Copy the current immutable object by setting a value for the {@link AnalyzedBasePair#saenger() saenger} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for saenger
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnalyzedBasePair withSaenger(Saenger value) {
if (this.saenger == value) return this;
Saenger newValue = Objects.requireNonNull(value, "saenger");
if (this.saenger.equals(newValue)) return this;
return new ImmutableAnalyzedBasePair(
this.basePair,
this.interactionType,
newValue,
this.leontisWesthof,
this.bph,
this.br,
this.isRepresented);
}
/**
* Copy the current immutable object by setting a value for the {@link AnalyzedBasePair#leontisWesthof() leontisWesthof} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for leontisWesthof
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnalyzedBasePair withLeontisWesthof(LeontisWesthof value) {
if (this.leontisWesthof == value) return this;
LeontisWesthof newValue = Objects.requireNonNull(value, "leontisWesthof");
if (this.leontisWesthof.equals(newValue)) return this;
return new ImmutableAnalyzedBasePair(
this.basePair,
this.interactionType,
this.saenger,
newValue,
this.bph,
this.br,
this.isRepresented);
}
/**
* Copy the current immutable object by setting a value for the {@link AnalyzedBasePair#bph() bph} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for bph
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnalyzedBasePair withBph(BPh value) {
if (this.bph == value) return this;
BPh newValue = Objects.requireNonNull(value, "bph");
if (this.bph.equals(newValue)) return this;
return new ImmutableAnalyzedBasePair(
this.basePair,
this.interactionType,
this.saenger,
this.leontisWesthof,
newValue,
this.br,
this.isRepresented);
}
/**
* Copy the current immutable object by setting a value for the {@link AnalyzedBasePair#br() br} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for br
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnalyzedBasePair withBr(BR value) {
if (this.br == value) return this;
BR newValue = Objects.requireNonNull(value, "br");
if (this.br.equals(newValue)) return this;
return new ImmutableAnalyzedBasePair(
this.basePair,
this.interactionType,
this.saenger,
this.leontisWesthof,
this.bph,
newValue,
this.isRepresented);
}
/**
* Copy the current immutable object by setting a value for the {@link AnalyzedBasePair#isRepresented() isRepresented} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isRepresented
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnalyzedBasePair withIsRepresented(boolean value) {
if (this.isRepresented == value) return this;
return new ImmutableAnalyzedBasePair(
this.basePair,
this.interactionType,
this.saenger,
this.leontisWesthof,
this.bph,
this.br,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableAnalyzedBasePair} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableAnalyzedBasePair
&& equalTo((ImmutableAnalyzedBasePair) another);
}
private boolean equalTo(ImmutableAnalyzedBasePair another) {
return basePair.equals(another.basePair)
&& interactionType.equals(another.interactionType)
&& saenger.equals(another.saenger)
&& leontisWesthof.equals(another.leontisWesthof)
&& bph.equals(another.bph)
&& br.equals(another.br);
}
/**
* Computes a hash code from attributes: {@code basePair}, {@code interactionType}, {@code saenger}, {@code leontisWesthof}, {@code bph}, {@code br}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + basePair.hashCode();
h += (h << 5) + interactionType.hashCode();
h += (h << 5) + saenger.hashCode();
h += (h << 5) + leontisWesthof.hashCode();
h += (h << 5) + bph.hashCode();
h += (h << 5) + br.hashCode();
return h;
}
/**
* Prints the immutable value {@code AnalyzedBasePair} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "AnalyzedBasePair{"
+ "basePair=" + basePair
+ ", interactionType=" + interactionType
+ ", saenger=" + saenger
+ ", leontisWesthof=" + leontisWesthof
+ ", bph=" + bph
+ ", br=" + br
+ "}";
}
/**
* Construct a new immutable {@code AnalyzedBasePair} instance.
* @param basePair The value for the {@code basePair} attribute
* @return An immutable AnalyzedBasePair instance
*/
public static ImmutableAnalyzedBasePair of(BasePair basePair) {
return new ImmutableAnalyzedBasePair(basePair);
}
/**
* Creates an immutable copy of a {@link AnalyzedBasePair} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable AnalyzedBasePair instance
*/
public static ImmutableAnalyzedBasePair copyOf(AnalyzedBasePair instance) {
if (instance instanceof ImmutableAnalyzedBasePair) {
return (ImmutableAnalyzedBasePair) instance;
}
return ImmutableAnalyzedBasePair.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableAnalyzedBasePair ImmutableAnalyzedBasePair}.
*
* ImmutableAnalyzedBasePair.builder()
* .basePair(pl.poznan.put.structure.BasePair) // required {@link AnalyzedBasePair#basePair() basePair}
* .interactionType(pl.poznan.put.rna.InteractionType) // optional {@link AnalyzedBasePair#interactionType() interactionType}
* .saenger(pl.poznan.put.notation.Saenger) // optional {@link AnalyzedBasePair#saenger() saenger}
* .leontisWesthof(pl.poznan.put.notation.LeontisWesthof) // optional {@link AnalyzedBasePair#leontisWesthof() leontisWesthof}
* .bph(pl.poznan.put.notation.BPh) // optional {@link AnalyzedBasePair#bph() bph}
* .br(pl.poznan.put.notation.BR) // optional {@link AnalyzedBasePair#br() br}
* .isRepresented(boolean) // optional {@link AnalyzedBasePair#isRepresented() isRepresented}
* .build();
*
* @return A new ImmutableAnalyzedBasePair builder
*/
public static ImmutableAnalyzedBasePair.Builder builder() {
return new ImmutableAnalyzedBasePair.Builder();
}
/**
* Builds instances of type {@link ImmutableAnalyzedBasePair ImmutableAnalyzedBasePair}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "AnalyzedBasePair", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_BASE_PAIR = 0x1L;
private static final long OPT_BIT_IS_REPRESENTED = 0x1L;
private long initBits = 0x1L;
private long optBits;
private @Nullable BasePair basePair;
private @Nullable InteractionType interactionType;
private @Nullable Saenger saenger;
private @Nullable LeontisWesthof leontisWesthof;
private @Nullable BPh bph;
private @Nullable BR br;
private boolean isRepresented;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.structure.ClassifiedBasePair} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ClassifiedBasePair instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.structure.AnalyzedBasePair} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AnalyzedBasePair instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
long bits = 0;
if (object instanceof ClassifiedBasePair) {
ClassifiedBasePair instance = (ClassifiedBasePair) object;
if ((bits & 0x1L) == 0) {
br(instance.br());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
interactionType(instance.interactionType());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
saenger(instance.saenger());
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
basePair(instance.basePair());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
leontisWesthof(instance.leontisWesthof());
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
bph(instance.bph());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
isRepresented(instance.isRepresented());
bits |= 0x40L;
}
}
if (object instanceof AnalyzedBasePair) {
AnalyzedBasePair instance = (AnalyzedBasePair) object;
if ((bits & 0x1L) == 0) {
br(instance.br());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
interactionType(instance.interactionType());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
saenger(instance.saenger());
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
basePair(instance.basePair());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
leontisWesthof(instance.leontisWesthof());
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
bph(instance.bph());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
isRepresented(instance.isRepresented());
bits |= 0x40L;
}
}
}
/**
* Initializes the value for the {@link AnalyzedBasePair#basePair() basePair} attribute.
* @param basePair The value for basePair
* @return {@code this} builder for use in a chained invocation
*/
public final Builder basePair(BasePair basePair) {
this.basePair = Objects.requireNonNull(basePair, "basePair");
initBits &= ~INIT_BIT_BASE_PAIR;
return this;
}
/**
* Initializes the value for the {@link AnalyzedBasePair#interactionType() interactionType} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AnalyzedBasePair#interactionType() interactionType}.
* @param interactionType The value for interactionType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder interactionType(InteractionType interactionType) {
this.interactionType = Objects.requireNonNull(interactionType, "interactionType");
return this;
}
/**
* Initializes the value for the {@link AnalyzedBasePair#saenger() saenger} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AnalyzedBasePair#saenger() saenger}.
* @param saenger The value for saenger
* @return {@code this} builder for use in a chained invocation
*/
public final Builder saenger(Saenger saenger) {
this.saenger = Objects.requireNonNull(saenger, "saenger");
return this;
}
/**
* Initializes the value for the {@link AnalyzedBasePair#leontisWesthof() leontisWesthof} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AnalyzedBasePair#leontisWesthof() leontisWesthof}.
* @param leontisWesthof The value for leontisWesthof
* @return {@code this} builder for use in a chained invocation
*/
public final Builder leontisWesthof(LeontisWesthof leontisWesthof) {
this.leontisWesthof = Objects.requireNonNull(leontisWesthof, "leontisWesthof");
return this;
}
/**
* Initializes the value for the {@link AnalyzedBasePair#bph() bph} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AnalyzedBasePair#bph() bph}.
* @param bph The value for bph
* @return {@code this} builder for use in a chained invocation
*/
public final Builder bph(BPh bph) {
this.bph = Objects.requireNonNull(bph, "bph");
return this;
}
/**
* Initializes the value for the {@link AnalyzedBasePair#br() br} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AnalyzedBasePair#br() br}.
* @param br The value for br
* @return {@code this} builder for use in a chained invocation
*/
public final Builder br(BR br) {
this.br = Objects.requireNonNull(br, "br");
return this;
}
/**
* Initializes the value for the {@link AnalyzedBasePair#isRepresented() isRepresented} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AnalyzedBasePair#isRepresented() isRepresented}.
* @param isRepresented The value for isRepresented
* @return {@code this} builder for use in a chained invocation
*/
public final Builder isRepresented(boolean isRepresented) {
this.isRepresented = isRepresented;
optBits |= OPT_BIT_IS_REPRESENTED;
return this;
}
/**
* Builds a new {@link ImmutableAnalyzedBasePair ImmutableAnalyzedBasePair}.
* @return An immutable instance of AnalyzedBasePair
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableAnalyzedBasePair build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableAnalyzedBasePair(this);
}
private boolean isRepresentedIsSet() {
return (optBits & OPT_BIT_IS_REPRESENTED) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_BASE_PAIR) != 0) attributes.add("basePair");
return "Cannot build AnalyzedBasePair, some of required attributes are not set " + attributes;
}
}
}