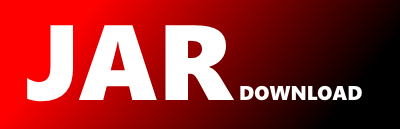
pl.poznan.put.structure.formats.ImmutableDefaultDotBracketFromPdb Maven / Gradle / Ivy
package pl.poznan.put.structure.formats;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import pl.poznan.put.pdb.PdbNamedResidueIdentifier;
import pl.poznan.put.pdb.analysis.PdbModel;
import pl.poznan.put.structure.DotBracketSymbol;
/**
* Immutable implementation of {@link DefaultDotBracketFromPdb}.
*
* Use the builder to create immutable instances:
* {@code ImmutableDefaultDotBracketFromPdb.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableDefaultDotBracketFromPdb.of()}.
*/
@Generated(from = "DefaultDotBracketFromPdb", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableDefaultDotBracketFromPdb
extends DefaultDotBracketFromPdb {
private final Set identifierSet;
private final PdbModel model;
private final List strands;
private final String sequence;
private final String structure;
private ImmutableDefaultDotBracketFromPdb(String sequence, String structure, PdbModel model) {
this.sequence = Objects.requireNonNull(sequence, "sequence");
this.structure = Objects.requireNonNull(structure, "structure");
this.model = Objects.requireNonNull(model, "model");
this.identifierSet = Collections.emptySet();
this.strands = createUnmodifiableList(false, createSafeList(super.strands(), true, false));
}
private ImmutableDefaultDotBracketFromPdb(ImmutableDefaultDotBracketFromPdb.Builder builder) {
this.identifierSet = createUnmodifiableSet(builder.identifierSet);
this.model = builder.model;
this.sequence = builder.sequence;
this.structure = builder.structure;
this.strands = builder.strandsIsSet()
? createUnmodifiableList(true, builder.strands)
: createUnmodifiableList(false, createSafeList(super.strands(), true, false));
}
private ImmutableDefaultDotBracketFromPdb(
Set identifierSet,
PdbModel model,
List strands,
String sequence,
String structure) {
this.identifierSet = identifierSet;
this.model = model;
this.strands = strands;
this.sequence = sequence;
this.structure = structure;
}
/**
*@return The set of residue identifiers used in this structure.
*/
@Override
public Set identifierSet() {
return identifierSet;
}
/**
* @return The value of the {@code model} attribute
*/
@Override
public PdbModel model() {
return model;
}
/**
* @return The value of the {@code strands} attribute
*/
@Override
public List strands() {
return strands;
}
/**
* @return The value of the {@code sequence} attribute
*/
@Override
public String sequence() {
return sequence;
}
/**
* @return The value of the {@code structure} attribute
*/
@Override
public String structure() {
return structure;
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultDotBracketFromPdb#identifierSet() identifierSet}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultDotBracketFromPdb withIdentifierSet(PdbNamedResidueIdentifier... elements) {
Set newValue = createUnmodifiableSet(createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableDefaultDotBracketFromPdb(newValue, this.model, this.strands, this.sequence, this.structure));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultDotBracketFromPdb#identifierSet() identifierSet}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of identifierSet elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultDotBracketFromPdb withIdentifierSet(Iterable extends PdbNamedResidueIdentifier> elements) {
if (this.identifierSet == elements) return this;
Set newValue = createUnmodifiableSet(createSafeList(elements, true, false));
return validate(new ImmutableDefaultDotBracketFromPdb(newValue, this.model, this.strands, this.sequence, this.structure));
}
/**
* Copy the current immutable object by setting a value for the {@link DefaultDotBracketFromPdb#model() model} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for model
* @return A modified copy of the {@code this} object
*/
public final ImmutableDefaultDotBracketFromPdb withModel(PdbModel value) {
if (this.model == value) return this;
PdbModel newValue = Objects.requireNonNull(value, "model");
return validate(new ImmutableDefaultDotBracketFromPdb(this.identifierSet, newValue, this.strands, this.sequence, this.structure));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultDotBracketFromPdb#strands() strands}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultDotBracketFromPdb withStrands(Strand... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableDefaultDotBracketFromPdb(this.identifierSet, this.model, newValue, this.sequence, this.structure));
}
/**
* Copy the current immutable object with elements that replace the content of {@link DefaultDotBracketFromPdb#strands() strands}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of strands elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDefaultDotBracketFromPdb withStrands(Iterable extends Strand> elements) {
if (this.strands == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableDefaultDotBracketFromPdb(this.identifierSet, this.model, newValue, this.sequence, this.structure));
}
/**
* Copy the current immutable object by setting a value for the {@link DefaultDotBracketFromPdb#sequence() sequence} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for sequence
* @return A modified copy of the {@code this} object
*/
public final ImmutableDefaultDotBracketFromPdb withSequence(String value) {
String newValue = Objects.requireNonNull(value, "sequence");
if (this.sequence.equals(newValue)) return this;
return validate(new ImmutableDefaultDotBracketFromPdb(this.identifierSet, this.model, this.strands, newValue, this.structure));
}
/**
* Copy the current immutable object by setting a value for the {@link DefaultDotBracketFromPdb#structure() structure} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for structure
* @return A modified copy of the {@code this} object
*/
public final ImmutableDefaultDotBracketFromPdb withStructure(String value) {
String newValue = Objects.requireNonNull(value, "structure");
if (this.structure.equals(newValue)) return this;
return validate(new ImmutableDefaultDotBracketFromPdb(this.identifierSet, this.model, this.strands, this.sequence, newValue));
}
/**
* This instance is equal to all instances of {@code ImmutableDefaultDotBracketFromPdb} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableDefaultDotBracketFromPdb
&& equalTo((ImmutableDefaultDotBracketFromPdb) another);
}
private boolean equalTo(ImmutableDefaultDotBracketFromPdb another) {
return identifierSet.equals(another.identifierSet)
&& model.equals(another.model)
&& sequence.equals(another.sequence)
&& structure.equals(another.structure);
}
/**
* Computes a hash code from attributes: {@code identifierSet}, {@code model}, {@code sequence}, {@code structure}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + identifierSet.hashCode();
h += (h << 5) + model.hashCode();
h += (h << 5) + sequence.hashCode();
h += (h << 5) + structure.hashCode();
return h;
}
/**
* Prints the immutable value {@code DefaultDotBracketFromPdb} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "DefaultDotBracketFromPdb{"
+ "identifierSet=" + identifierSet
+ ", model=" + model
+ ", sequence=" + sequence
+ ", structure=" + structure
+ "}";
}
private transient volatile long lazyInitBitmap;
private static final long PAIRS_LAZY_INIT_BIT = 0x1L;
private transient Map pairs;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link DefaultDotBracketFromPdb#pairs() pairs} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code pairs} attribute
*/
@Override
public Map pairs() {
if ((lazyInitBitmap & PAIRS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & PAIRS_LAZY_INIT_BIT) == 0) {
this.pairs = Objects.requireNonNull(super.pairs(), "pairs");
lazyInitBitmap |= PAIRS_LAZY_INIT_BIT;
}
}
}
return pairs;
}
private static final long RESIDUE_TO_SYMBOL_LAZY_INIT_BIT = 0x2L;
private transient Map residueToSymbol;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link DefaultDotBracketFromPdb#residueToSymbol() residueToSymbol} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code residueToSymbol} attribute
*/
@Override
protected Map residueToSymbol() {
if ((lazyInitBitmap & RESIDUE_TO_SYMBOL_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & RESIDUE_TO_SYMBOL_LAZY_INIT_BIT) == 0) {
this.residueToSymbol = Objects.requireNonNull(super.residueToSymbol(), "residueToSymbol");
lazyInitBitmap |= RESIDUE_TO_SYMBOL_LAZY_INIT_BIT;
}
}
}
return residueToSymbol;
}
private static final long SYMBOL_TO_RESIDUE_LAZY_INIT_BIT = 0x4L;
private transient Map symbolToResidue;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link DefaultDotBracketFromPdb#symbolToResidue() symbolToResidue} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code symbolToResidue} attribute
*/
@Override
protected Map symbolToResidue() {
if ((lazyInitBitmap & SYMBOL_TO_RESIDUE_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & SYMBOL_TO_RESIDUE_LAZY_INIT_BIT) == 0) {
this.symbolToResidue = Objects.requireNonNull(super.symbolToResidue(), "symbolToResidue");
lazyInitBitmap |= SYMBOL_TO_RESIDUE_LAZY_INIT_BIT;
}
}
}
return symbolToResidue;
}
/**
* Construct a new immutable {@code DefaultDotBracketFromPdb} instance.
* @param sequence The value for the {@code sequence} attribute
* @param structure The value for the {@code structure} attribute
* @param model The value for the {@code model} attribute
* @return An immutable DefaultDotBracketFromPdb instance
*/
public static ImmutableDefaultDotBracketFromPdb of(String sequence, String structure, PdbModel model) {
return validate(new ImmutableDefaultDotBracketFromPdb(sequence, structure, model));
}
private static ImmutableDefaultDotBracketFromPdb validate(ImmutableDefaultDotBracketFromPdb instance) {
instance = (ImmutableDefaultDotBracketFromPdb) instance.validate();
return instance;
}
/**
* Creates an immutable copy of a {@link DefaultDotBracketFromPdb} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable DefaultDotBracketFromPdb instance
*/
public static ImmutableDefaultDotBracketFromPdb copyOf(DefaultDotBracketFromPdb instance) {
if (instance instanceof ImmutableDefaultDotBracketFromPdb) {
return (ImmutableDefaultDotBracketFromPdb) instance;
}
return ImmutableDefaultDotBracketFromPdb.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableDefaultDotBracketFromPdb ImmutableDefaultDotBracketFromPdb}.
*
* ImmutableDefaultDotBracketFromPdb.builder()
* .addIdentifierSet|addAllIdentifierSet(pl.poznan.put.pdb.PdbNamedResidueIdentifier) // {@link DefaultDotBracketFromPdb#identifierSet() identifierSet} elements
* .model(pl.poznan.put.pdb.analysis.PdbModel) // required {@link DefaultDotBracketFromPdb#model() model}
* .addStrands|addAllStrands(pl.poznan.put.structure.formats.Strand) // {@link DefaultDotBracketFromPdb#strands() strands} elements
* .sequence(String) // required {@link DefaultDotBracketFromPdb#sequence() sequence}
* .structure(String) // required {@link DefaultDotBracketFromPdb#structure() structure}
* .build();
*
* @return A new ImmutableDefaultDotBracketFromPdb builder
*/
public static ImmutableDefaultDotBracketFromPdb.Builder builder() {
return new ImmutableDefaultDotBracketFromPdb.Builder();
}
/**
* Builds instances of type {@link ImmutableDefaultDotBracketFromPdb ImmutableDefaultDotBracketFromPdb}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "DefaultDotBracketFromPdb", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_MODEL = 0x1L;
private static final long INIT_BIT_SEQUENCE = 0x2L;
private static final long INIT_BIT_STRUCTURE = 0x4L;
private static final long OPT_BIT_STRANDS = 0x1L;
private long initBits = 0x7L;
private long optBits;
private List identifierSet = new ArrayList();
private @Nullable PdbModel model;
private List strands = new ArrayList();
private @Nullable String sequence;
private @Nullable String structure;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.structure.formats.DefaultDotBracketFromPdb} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(DefaultDotBracketFromPdb instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.structure.formats.DotBracketFromPdb} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(DotBracketFromPdb instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.structure.formats.DotBracket} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(DotBracket instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
long bits = 0;
if (object instanceof DefaultDotBracketFromPdb) {
DefaultDotBracketFromPdb instance = (DefaultDotBracketFromPdb) object;
if ((bits & 0x1L) == 0) {
sequence(instance.sequence());
bits |= 0x1L;
}
model(instance.model());
if ((bits & 0x2L) == 0) {
structure(instance.structure());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
addAllStrands(instance.strands());
bits |= 0x4L;
}
}
if (object instanceof DotBracketFromPdb) {
DotBracketFromPdb instance = (DotBracketFromPdb) object;
addAllIdentifierSet(instance.identifierSet());
}
if (object instanceof DotBracket) {
DotBracket instance = (DotBracket) object;
if ((bits & 0x1L) == 0) {
sequence(instance.sequence());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
structure(instance.structure());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
addAllStrands(instance.strands());
bits |= 0x4L;
}
}
}
/**
* Adds one element to {@link DefaultDotBracketFromPdb#identifierSet() identifierSet} set.
* @param element A identifierSet element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addIdentifierSet(PdbNamedResidueIdentifier element) {
this.identifierSet.add(Objects.requireNonNull(element, "identifierSet element"));
return this;
}
/**
* Adds elements to {@link DefaultDotBracketFromPdb#identifierSet() identifierSet} set.
* @param elements An array of identifierSet elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addIdentifierSet(PdbNamedResidueIdentifier... elements) {
for (PdbNamedResidueIdentifier element : elements) {
this.identifierSet.add(Objects.requireNonNull(element, "identifierSet element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link DefaultDotBracketFromPdb#identifierSet() identifierSet} set.
* @param elements An iterable of identifierSet elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder identifierSet(Iterable extends PdbNamedResidueIdentifier> elements) {
this.identifierSet.clear();
return addAllIdentifierSet(elements);
}
/**
* Adds elements to {@link DefaultDotBracketFromPdb#identifierSet() identifierSet} set.
* @param elements An iterable of identifierSet elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllIdentifierSet(Iterable extends PdbNamedResidueIdentifier> elements) {
for (PdbNamedResidueIdentifier element : elements) {
this.identifierSet.add(Objects.requireNonNull(element, "identifierSet element"));
}
return this;
}
/**
* Initializes the value for the {@link DefaultDotBracketFromPdb#model() model} attribute.
* @param model The value for model
* @return {@code this} builder for use in a chained invocation
*/
public final Builder model(PdbModel model) {
this.model = Objects.requireNonNull(model, "model");
initBits &= ~INIT_BIT_MODEL;
return this;
}
/**
* Adds one element to {@link DefaultDotBracketFromPdb#strands() strands} list.
* @param element A strands element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addStrands(Strand element) {
this.strands.add(Objects.requireNonNull(element, "strands element"));
optBits |= OPT_BIT_STRANDS;
return this;
}
/**
* Adds elements to {@link DefaultDotBracketFromPdb#strands() strands} list.
* @param elements An array of strands elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addStrands(Strand... elements) {
for (Strand element : elements) {
this.strands.add(Objects.requireNonNull(element, "strands element"));
}
optBits |= OPT_BIT_STRANDS;
return this;
}
/**
* Sets or replaces all elements for {@link DefaultDotBracketFromPdb#strands() strands} list.
* @param elements An iterable of strands elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder strands(Iterable extends Strand> elements) {
this.strands.clear();
return addAllStrands(elements);
}
/**
* Adds elements to {@link DefaultDotBracketFromPdb#strands() strands} list.
* @param elements An iterable of strands elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllStrands(Iterable extends Strand> elements) {
for (Strand element : elements) {
this.strands.add(Objects.requireNonNull(element, "strands element"));
}
optBits |= OPT_BIT_STRANDS;
return this;
}
/**
* Initializes the value for the {@link DefaultDotBracketFromPdb#sequence() sequence} attribute.
* @param sequence The value for sequence
* @return {@code this} builder for use in a chained invocation
*/
public final Builder sequence(String sequence) {
this.sequence = Objects.requireNonNull(sequence, "sequence");
initBits &= ~INIT_BIT_SEQUENCE;
return this;
}
/**
* Initializes the value for the {@link DefaultDotBracketFromPdb#structure() structure} attribute.
* @param structure The value for structure
* @return {@code this} builder for use in a chained invocation
*/
public final Builder structure(String structure) {
this.structure = Objects.requireNonNull(structure, "structure");
initBits &= ~INIT_BIT_STRUCTURE;
return this;
}
/**
* Builds a new {@link ImmutableDefaultDotBracketFromPdb ImmutableDefaultDotBracketFromPdb}.
* @return An immutable instance of DefaultDotBracketFromPdb
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableDefaultDotBracketFromPdb build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return ImmutableDefaultDotBracketFromPdb.validate(new ImmutableDefaultDotBracketFromPdb(this));
}
private boolean strandsIsSet() {
return (optBits & OPT_BIT_STRANDS) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_MODEL) != 0) attributes.add("model");
if ((initBits & INIT_BIT_SEQUENCE) != 0) attributes.add("sequence");
if ((initBits & INIT_BIT_STRUCTURE) != 0) attributes.add("structure");
return "Cannot build DefaultDotBracketFromPdb, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
/** Unmodifiable set constructed from list to avoid rehashing. */
private static Set createUnmodifiableSet(List list) {
switch(list.size()) {
case 0: return Collections.emptySet();
case 1: return Collections.singleton(list.get(0));
default:
Set set = new LinkedHashSet<>(list.size());
set.addAll(list);
return Collections.unmodifiableSet(set);
}
}
}