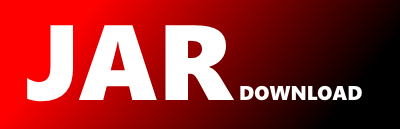
pl.poznan.put.structure.formats.ImmutableExtendedEntry Maven / Gradle / Ivy
package pl.poznan.put.structure.formats;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link Ct.ExtendedEntry}.
*
* Use the builder to create immutable instances:
* {@code ImmutableExtendedEntry.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableExtendedEntry.of()}.
*/
@Generated(from = "Ct.ExtendedEntry", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableExtendedEntry extends Ct.ExtendedEntry {
private final int index;
private final char seq;
private final int before;
private final int after;
private final int pair;
private final int original;
private final String comment;
private ImmutableExtendedEntry(int index, char seq, int before, int after, int pair, int original) {
this.index = index;
this.seq = seq;
this.before = before;
this.after = after;
this.pair = pair;
this.original = original;
this.comment = Objects.requireNonNull(super.comment(), "comment");
}
private ImmutableExtendedEntry(ImmutableExtendedEntry.Builder builder) {
this.index = builder.index;
this.seq = builder.seq;
this.before = builder.before;
this.after = builder.after;
this.pair = builder.pair;
this.original = builder.original;
this.comment = builder.comment != null
? builder.comment
: Objects.requireNonNull(super.comment(), "comment");
}
private ImmutableExtendedEntry(int index, char seq, int before, int after, int pair, int original, String comment) {
this.index = index;
this.seq = seq;
this.before = before;
this.after = after;
this.pair = pair;
this.original = original;
this.comment = comment;
}
/**
*@return The value of `index` column.
*/
@Override
public int index() {
return index;
}
/**
*@return The value of `seq` column.
*/
@Override
public char seq() {
return seq;
}
/**
*@return The value of `before` column.
*/
@Override
public int before() {
return before;
}
/**
*@return The value of `after` column.
*/
@Override
public int after() {
return after;
}
/**
*@return The value of `pair` column.
*/
@Override
public int pair() {
return pair;
}
/**
*@return The value of `original` column.
*/
@Override
public int original() {
return original;
}
/**
*@return An optional comment.
*/
@Override
public String comment() {
return comment;
}
/**
* Copy the current immutable object by setting a value for the {@link Ct.ExtendedEntry#index() index} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for index
* @return A modified copy of the {@code this} object
*/
public final ImmutableExtendedEntry withIndex(int value) {
if (this.index == value) return this;
return new ImmutableExtendedEntry(value, this.seq, this.before, this.after, this.pair, this.original, this.comment);
}
/**
* Copy the current immutable object by setting a value for the {@link Ct.ExtendedEntry#seq() seq} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for seq
* @return A modified copy of the {@code this} object
*/
public final ImmutableExtendedEntry withSeq(char value) {
if (this.seq == value) return this;
return new ImmutableExtendedEntry(this.index, value, this.before, this.after, this.pair, this.original, this.comment);
}
/**
* Copy the current immutable object by setting a value for the {@link Ct.ExtendedEntry#before() before} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for before
* @return A modified copy of the {@code this} object
*/
public final ImmutableExtendedEntry withBefore(int value) {
if (this.before == value) return this;
return new ImmutableExtendedEntry(this.index, this.seq, value, this.after, this.pair, this.original, this.comment);
}
/**
* Copy the current immutable object by setting a value for the {@link Ct.ExtendedEntry#after() after} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for after
* @return A modified copy of the {@code this} object
*/
public final ImmutableExtendedEntry withAfter(int value) {
if (this.after == value) return this;
return new ImmutableExtendedEntry(this.index, this.seq, this.before, value, this.pair, this.original, this.comment);
}
/**
* Copy the current immutable object by setting a value for the {@link Ct.ExtendedEntry#pair() pair} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for pair
* @return A modified copy of the {@code this} object
*/
public final ImmutableExtendedEntry withPair(int value) {
if (this.pair == value) return this;
return new ImmutableExtendedEntry(this.index, this.seq, this.before, this.after, value, this.original, this.comment);
}
/**
* Copy the current immutable object by setting a value for the {@link Ct.ExtendedEntry#original() original} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for original
* @return A modified copy of the {@code this} object
*/
public final ImmutableExtendedEntry withOriginal(int value) {
if (this.original == value) return this;
return new ImmutableExtendedEntry(this.index, this.seq, this.before, this.after, this.pair, value, this.comment);
}
/**
* Copy the current immutable object by setting a value for the {@link Ct.ExtendedEntry#comment() comment} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for comment
* @return A modified copy of the {@code this} object
*/
public final ImmutableExtendedEntry withComment(String value) {
String newValue = Objects.requireNonNull(value, "comment");
if (this.comment.equals(newValue)) return this;
return new ImmutableExtendedEntry(this.index, this.seq, this.before, this.after, this.pair, this.original, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableExtendedEntry} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableExtendedEntry
&& equalTo((ImmutableExtendedEntry) another);
}
private boolean equalTo(ImmutableExtendedEntry another) {
return index == another.index
&& seq == another.seq
&& before == another.before
&& after == another.after
&& pair == another.pair
&& original == another.original
&& comment.equals(another.comment);
}
/**
* Computes a hash code from attributes: {@code index}, {@code seq}, {@code before}, {@code after}, {@code pair}, {@code original}, {@code comment}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + index;
h += (h << 5) + Character.hashCode(seq);
h += (h << 5) + before;
h += (h << 5) + after;
h += (h << 5) + pair;
h += (h << 5) + original;
h += (h << 5) + comment.hashCode();
return h;
}
/**
* Construct a new immutable {@code ExtendedEntry} instance.
* @param index The value for the {@code index} attribute
* @param seq The value for the {@code seq} attribute
* @param before The value for the {@code before} attribute
* @param after The value for the {@code after} attribute
* @param pair The value for the {@code pair} attribute
* @param original The value for the {@code original} attribute
* @return An immutable ExtendedEntry instance
*/
public static ImmutableExtendedEntry of(int index, char seq, int before, int after, int pair, int original) {
return new ImmutableExtendedEntry(index, seq, before, after, pair, original);
}
/**
* Creates an immutable copy of a {@link Ct.ExtendedEntry} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ExtendedEntry instance
*/
public static ImmutableExtendedEntry copyOf(Ct.ExtendedEntry instance) {
if (instance instanceof ImmutableExtendedEntry) {
return (ImmutableExtendedEntry) instance;
}
return ImmutableExtendedEntry.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableExtendedEntry ImmutableExtendedEntry}.
*
* ImmutableExtendedEntry.builder()
* .index(int) // required {@link Ct.ExtendedEntry#index() index}
* .seq(char) // required {@link Ct.ExtendedEntry#seq() seq}
* .before(int) // required {@link Ct.ExtendedEntry#before() before}
* .after(int) // required {@link Ct.ExtendedEntry#after() after}
* .pair(int) // required {@link Ct.ExtendedEntry#pair() pair}
* .original(int) // required {@link Ct.ExtendedEntry#original() original}
* .comment(String) // optional {@link Ct.ExtendedEntry#comment() comment}
* .build();
*
* @return A new ImmutableExtendedEntry builder
*/
public static ImmutableExtendedEntry.Builder builder() {
return new ImmutableExtendedEntry.Builder();
}
/**
* Builds instances of type {@link ImmutableExtendedEntry ImmutableExtendedEntry}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "Ct.ExtendedEntry", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_INDEX = 0x1L;
private static final long INIT_BIT_SEQ = 0x2L;
private static final long INIT_BIT_BEFORE = 0x4L;
private static final long INIT_BIT_AFTER = 0x8L;
private static final long INIT_BIT_PAIR = 0x10L;
private static final long INIT_BIT_ORIGINAL = 0x20L;
private long initBits = 0x3fL;
private int index;
private char seq;
private int before;
private int after;
private int pair;
private int original;
private @Nullable String comment;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ExtendedEntry} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(Ct.ExtendedEntry instance) {
Objects.requireNonNull(instance, "instance");
index(instance.index());
seq(instance.seq());
before(instance.before());
after(instance.after());
pair(instance.pair());
original(instance.original());
comment(instance.comment());
return this;
}
/**
* Initializes the value for the {@link Ct.ExtendedEntry#index() index} attribute.
* @param index The value for index
* @return {@code this} builder for use in a chained invocation
*/
public final Builder index(int index) {
this.index = index;
initBits &= ~INIT_BIT_INDEX;
return this;
}
/**
* Initializes the value for the {@link Ct.ExtendedEntry#seq() seq} attribute.
* @param seq The value for seq
* @return {@code this} builder for use in a chained invocation
*/
public final Builder seq(char seq) {
this.seq = seq;
initBits &= ~INIT_BIT_SEQ;
return this;
}
/**
* Initializes the value for the {@link Ct.ExtendedEntry#before() before} attribute.
* @param before The value for before
* @return {@code this} builder for use in a chained invocation
*/
public final Builder before(int before) {
this.before = before;
initBits &= ~INIT_BIT_BEFORE;
return this;
}
/**
* Initializes the value for the {@link Ct.ExtendedEntry#after() after} attribute.
* @param after The value for after
* @return {@code this} builder for use in a chained invocation
*/
public final Builder after(int after) {
this.after = after;
initBits &= ~INIT_BIT_AFTER;
return this;
}
/**
* Initializes the value for the {@link Ct.ExtendedEntry#pair() pair} attribute.
* @param pair The value for pair
* @return {@code this} builder for use in a chained invocation
*/
public final Builder pair(int pair) {
this.pair = pair;
initBits &= ~INIT_BIT_PAIR;
return this;
}
/**
* Initializes the value for the {@link Ct.ExtendedEntry#original() original} attribute.
* @param original The value for original
* @return {@code this} builder for use in a chained invocation
*/
public final Builder original(int original) {
this.original = original;
initBits &= ~INIT_BIT_ORIGINAL;
return this;
}
/**
* Initializes the value for the {@link Ct.ExtendedEntry#comment() comment} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link Ct.ExtendedEntry#comment() comment}.
* @param comment The value for comment
* @return {@code this} builder for use in a chained invocation
*/
public final Builder comment(String comment) {
this.comment = Objects.requireNonNull(comment, "comment");
return this;
}
/**
* Builds a new {@link ImmutableExtendedEntry ImmutableExtendedEntry}.
* @return An immutable instance of ExtendedEntry
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableExtendedEntry build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableExtendedEntry(this);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_INDEX) != 0) attributes.add("index");
if ((initBits & INIT_BIT_SEQ) != 0) attributes.add("seq");
if ((initBits & INIT_BIT_BEFORE) != 0) attributes.add("before");
if ((initBits & INIT_BIT_AFTER) != 0) attributes.add("after");
if ((initBits & INIT_BIT_PAIR) != 0) attributes.add("pair");
if ((initBits & INIT_BIT_ORIGINAL) != 0) attributes.add("original");
return "Cannot build ExtendedEntry, some of required attributes are not set " + attributes;
}
}
}