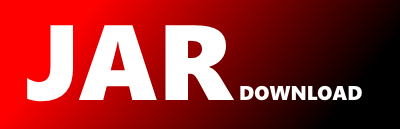
pl.poznan.put.structure.formats.ImmutableStrandView Maven / Gradle / Ivy
package pl.poznan.put.structure.formats;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import pl.poznan.put.structure.DotBracketSymbol;
/**
* Immutable implementation of {@link StrandView}.
*
* Use the builder to create immutable instances:
* {@code ImmutableStrandView.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableStrandView.of()}.
*/
@Generated(from = "StrandView", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableStrandView extends StrandView {
private final List combineStrands;
private final String name;
private final int begin;
private final int end;
private final DotBracket parent;
private ImmutableStrandView(String name, DotBracket parent, int begin, int end) {
this.name = Objects.requireNonNull(name, "name");
this.parent = Objects.requireNonNull(parent, "parent");
this.begin = begin;
this.end = end;
this.combineStrands = Collections.emptyList();
}
private ImmutableStrandView(
List combineStrands,
String name,
int begin,
int end,
DotBracket parent) {
this.combineStrands = combineStrands;
this.name = name;
this.begin = begin;
this.end = end;
this.parent = parent;
}
/**
* Combines strands which share a base pair into a new dot-bracket instance and returns a list of
* those.
* @return The list of dot-bracket instances, each containing strands which only pair with each
* other.
*/
@Override
public List combineStrands() {
return combineStrands;
}
/**
* @return The value of the {@code name} attribute
*/
@Override
public String name() {
return name;
}
/**
* @return The value of the {@code begin} attribute
*/
@Override
public int begin() {
return begin;
}
/**
* @return The value of the {@code end} attribute
*/
@Override
public int end() {
return end;
}
/**
*@return The parent dot-bracket structure of this strand.
*/
@Override
public DotBracket parent() {
return parent;
}
/**
* Copy the current immutable object with elements that replace the content of {@link StrandView#combineStrands() combineStrands}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableStrandView withCombineStrands(DotBracket... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableStrandView(newValue, this.name, this.begin, this.end, this.parent);
}
/**
* Copy the current immutable object with elements that replace the content of {@link StrandView#combineStrands() combineStrands}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of combineStrands elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableStrandView withCombineStrands(Iterable extends DotBracket> elements) {
if (this.combineStrands == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableStrandView(newValue, this.name, this.begin, this.end, this.parent);
}
/**
* Copy the current immutable object by setting a value for the {@link StrandView#name() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableStrandView withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableStrandView(this.combineStrands, newValue, this.begin, this.end, this.parent);
}
/**
* Copy the current immutable object by setting a value for the {@link StrandView#begin() begin} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for begin
* @return A modified copy of the {@code this} object
*/
public final ImmutableStrandView withBegin(int value) {
if (this.begin == value) return this;
return new ImmutableStrandView(this.combineStrands, this.name, value, this.end, this.parent);
}
/**
* Copy the current immutable object by setting a value for the {@link StrandView#end() end} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for end
* @return A modified copy of the {@code this} object
*/
public final ImmutableStrandView withEnd(int value) {
if (this.end == value) return this;
return new ImmutableStrandView(this.combineStrands, this.name, this.begin, value, this.parent);
}
/**
* Copy the current immutable object by setting a value for the {@link StrandView#parent() parent} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for parent
* @return A modified copy of the {@code this} object
*/
public final ImmutableStrandView withParent(DotBracket value) {
if (this.parent == value) return this;
DotBracket newValue = Objects.requireNonNull(value, "parent");
return new ImmutableStrandView(this.combineStrands, this.name, this.begin, this.end, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableStrandView} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableStrandView
&& equalTo((ImmutableStrandView) another);
}
private boolean equalTo(ImmutableStrandView another) {
return combineStrands.equals(another.combineStrands)
&& name.equals(another.name)
&& begin == another.begin
&& end == another.end
&& parent.equals(another.parent);
}
/**
* Computes a hash code from attributes: {@code combineStrands}, {@code name}, {@code begin}, {@code end}, {@code parent}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + combineStrands.hashCode();
h += (h << 5) + name.hashCode();
h += (h << 5) + begin;
h += (h << 5) + end;
h += (h << 5) + parent.hashCode();
return h;
}
private transient volatile long lazyInitBitmap;
private static final long SYMBOLS_LAZY_INIT_BIT = 0x1L;
private transient List symbols;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link StrandView#symbols() symbols} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code symbols} attribute
*/
@Override
public List symbols() {
if ((lazyInitBitmap & SYMBOLS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & SYMBOLS_LAZY_INIT_BIT) == 0) {
this.symbols = Objects.requireNonNull(super.symbols(), "symbols");
lazyInitBitmap |= SYMBOLS_LAZY_INIT_BIT;
}
}
}
return symbols;
}
/**
* Construct a new immutable {@code StrandView} instance.
* @param name The value for the {@code name} attribute
* @param parent The value for the {@code parent} attribute
* @param begin The value for the {@code begin} attribute
* @param end The value for the {@code end} attribute
* @return An immutable StrandView instance
*/
public static ImmutableStrandView of(String name, DotBracket parent, int begin, int end) {
return new ImmutableStrandView(name, parent, begin, end);
}
/**
* Creates an immutable copy of a {@link StrandView} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable StrandView instance
*/
public static ImmutableStrandView copyOf(StrandView instance) {
if (instance instanceof ImmutableStrandView) {
return (ImmutableStrandView) instance;
}
return ImmutableStrandView.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableStrandView ImmutableStrandView}.
*
* ImmutableStrandView.builder()
* .addCombineStrands|addAllCombineStrands(pl.poznan.put.structure.formats.DotBracket) // {@link StrandView#combineStrands() combineStrands} elements
* .name(String) // required {@link StrandView#name() name}
* .begin(int) // required {@link StrandView#begin() begin}
* .end(int) // required {@link StrandView#end() end}
* .parent(pl.poznan.put.structure.formats.DotBracket) // required {@link StrandView#parent() parent}
* .build();
*
* @return A new ImmutableStrandView builder
*/
public static ImmutableStrandView.Builder builder() {
return new ImmutableStrandView.Builder();
}
/**
* Builds instances of type {@link ImmutableStrandView ImmutableStrandView}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "StrandView", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long INIT_BIT_BEGIN = 0x2L;
private static final long INIT_BIT_END = 0x4L;
private static final long INIT_BIT_PARENT = 0x8L;
private long initBits = 0xfL;
private List combineStrands = new ArrayList();
private @Nullable String name;
private int begin;
private int end;
private @Nullable DotBracket parent;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.structure.formats.DotBracket} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(DotBracket instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.structure.formats.StrandView} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(StrandView instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code pl.poznan.put.structure.formats.Strand} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(Strand instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
long bits = 0;
if (object instanceof DotBracket) {
DotBracket instance = (DotBracket) object;
addAllCombineStrands(instance.combineStrands());
}
if (object instanceof StrandView) {
StrandView instance = (StrandView) object;
if ((bits & 0x1L) == 0) {
name(instance.name());
bits |= 0x1L;
}
parent(instance.parent());
if ((bits & 0x2L) == 0) {
end(instance.end());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
begin(instance.begin());
bits |= 0x4L;
}
}
if (object instanceof Strand) {
Strand instance = (Strand) object;
if ((bits & 0x1L) == 0) {
name(instance.name());
bits |= 0x1L;
}
if ((bits & 0x4L) == 0) {
begin(instance.begin());
bits |= 0x4L;
}
if ((bits & 0x2L) == 0) {
end(instance.end());
bits |= 0x2L;
}
}
}
/**
* Adds one element to {@link StrandView#combineStrands() combineStrands} list.
* @param element A combineStrands element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addCombineStrands(DotBracket element) {
this.combineStrands.add(Objects.requireNonNull(element, "combineStrands element"));
return this;
}
/**
* Adds elements to {@link StrandView#combineStrands() combineStrands} list.
* @param elements An array of combineStrands elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addCombineStrands(DotBracket... elements) {
for (DotBracket element : elements) {
this.combineStrands.add(Objects.requireNonNull(element, "combineStrands element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link StrandView#combineStrands() combineStrands} list.
* @param elements An iterable of combineStrands elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder combineStrands(Iterable extends DotBracket> elements) {
this.combineStrands.clear();
return addAllCombineStrands(elements);
}
/**
* Adds elements to {@link StrandView#combineStrands() combineStrands} list.
* @param elements An iterable of combineStrands elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllCombineStrands(Iterable extends DotBracket> elements) {
for (DotBracket element : elements) {
this.combineStrands.add(Objects.requireNonNull(element, "combineStrands element"));
}
return this;
}
/**
* Initializes the value for the {@link StrandView#name() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link StrandView#begin() begin} attribute.
* @param begin The value for begin
* @return {@code this} builder for use in a chained invocation
*/
public final Builder begin(int begin) {
this.begin = begin;
initBits &= ~INIT_BIT_BEGIN;
return this;
}
/**
* Initializes the value for the {@link StrandView#end() end} attribute.
* @param end The value for end
* @return {@code this} builder for use in a chained invocation
*/
public final Builder end(int end) {
this.end = end;
initBits &= ~INIT_BIT_END;
return this;
}
/**
* Initializes the value for the {@link StrandView#parent() parent} attribute.
* @param parent The value for parent
* @return {@code this} builder for use in a chained invocation
*/
public final Builder parent(DotBracket parent) {
this.parent = Objects.requireNonNull(parent, "parent");
initBits &= ~INIT_BIT_PARENT;
return this;
}
/**
* Builds a new {@link ImmutableStrandView ImmutableStrandView}.
* @return An immutable instance of StrandView
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableStrandView build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableStrandView(createUnmodifiableList(true, combineStrands), name, begin, end, parent);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_BEGIN) != 0) attributes.add("begin");
if ((initBits & INIT_BIT_END) != 0) attributes.add("end");
if ((initBits & INIT_BIT_PARENT) != 0) attributes.add("parent");
return "Cannot build StrandView, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}