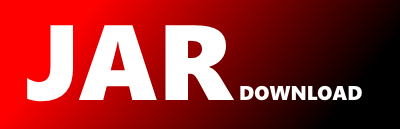
pl.poznan.put.structure.pseudoknots.ImmutableRegion Maven / Gradle / Ivy
Show all versions of BioCommons Show documentation
package pl.poznan.put.structure.pseudoknots;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import pl.poznan.put.structure.formats.BpSeq;
/**
* Immutable implementation of {@link Region}.
*
* Use the builder to create immutable instances:
* {@code ImmutableRegion.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableRegion.of()}.
*/
@Generated(from = "Region", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableRegion extends Region {
private final List entries;
private final int begin;
private final int end;
private final boolean isRemoved;
private ImmutableRegion(Iterable entries) {
this.entries = createUnmodifiableList(false, createSafeList(entries, true, false));
this.begin = initShim.begin();
this.end = initShim.end();
this.isRemoved = initShim.isRemoved();
this.initShim = null;
}
private ImmutableRegion(ImmutableRegion.Builder builder) {
this.entries = createUnmodifiableList(true, builder.entries);
if (builder.beginIsSet()) {
initShim.begin(builder.begin);
}
if (builder.endIsSet()) {
initShim.end(builder.end);
}
if (builder.isRemovedIsSet()) {
initShim.isRemoved(builder.isRemoved);
}
this.begin = initShim.begin();
this.end = initShim.end();
this.isRemoved = initShim.isRemoved();
this.initShim = null;
}
private ImmutableRegion(
List entries,
int begin,
int end,
boolean isRemoved) {
this.entries = entries;
this.begin = begin;
this.end = end;
this.isRemoved = isRemoved;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "Region", generator = "Immutables")
private final class InitShim {
private byte beginBuildStage = STAGE_UNINITIALIZED;
private int begin;
int begin() {
if (beginBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (beginBuildStage == STAGE_UNINITIALIZED) {
beginBuildStage = STAGE_INITIALIZING;
this.begin = ImmutableRegion.super.begin();
beginBuildStage = STAGE_INITIALIZED;
}
return this.begin;
}
void begin(int begin) {
this.begin = begin;
beginBuildStage = STAGE_INITIALIZED;
}
private byte endBuildStage = STAGE_UNINITIALIZED;
private int end;
int end() {
if (endBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (endBuildStage == STAGE_UNINITIALIZED) {
endBuildStage = STAGE_INITIALIZING;
this.end = ImmutableRegion.super.end();
endBuildStage = STAGE_INITIALIZED;
}
return this.end;
}
void end(int end) {
this.end = end;
endBuildStage = STAGE_INITIALIZED;
}
private byte isRemovedBuildStage = STAGE_UNINITIALIZED;
private boolean isRemoved;
boolean isRemoved() {
if (isRemovedBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (isRemovedBuildStage == STAGE_UNINITIALIZED) {
isRemovedBuildStage = STAGE_INITIALIZING;
this.isRemoved = ImmutableRegion.super.isRemoved();
isRemovedBuildStage = STAGE_INITIALIZED;
}
return this.isRemoved;
}
void isRemoved(boolean isRemoved) {
this.isRemoved = isRemoved;
isRemovedBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (beginBuildStage == STAGE_INITIALIZING) attributes.add("begin");
if (endBuildStage == STAGE_INITIALIZING) attributes.add("end");
if (isRemovedBuildStage == STAGE_INITIALIZING) attributes.add("isRemoved");
return "Cannot build Region, attribute initializers form cycle " + attributes;
}
}
/**
*@return The list of BPSEQ entries in this region.
*/
@Override
public List entries() {
return entries;
}
/**
*@return The first index of a region.
*/
@Override
public int begin() {
InitShim shim = this.initShim;
return shim != null
? shim.begin()
: this.begin;
}
/**
*@return The last index of a region.
*/
@Override
public int end() {
InitShim shim = this.initShim;
return shim != null
? shim.end()
: this.end;
}
/**
*@return True if this region was removed.
*/
@Override
public boolean isRemoved() {
InitShim shim = this.initShim;
return shim != null
? shim.isRemoved()
: this.isRemoved;
}
/**
* Copy the current immutable object with elements that replace the content of {@link Region#entries() entries}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRegion withEntries(BpSeq.Entry... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableRegion(newValue, this.begin, this.end, this.isRemoved);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Region#entries() entries}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of entries elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRegion withEntries(Iterable elements) {
if (this.entries == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableRegion(newValue, this.begin, this.end, this.isRemoved);
}
/**
* Copy the current immutable object by setting a value for the {@link Region#begin() begin} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for begin
* @return A modified copy of the {@code this} object
*/
public final ImmutableRegion withBegin(int value) {
if (this.begin == value) return this;
return new ImmutableRegion(this.entries, value, this.end, this.isRemoved);
}
/**
* Copy the current immutable object by setting a value for the {@link Region#end() end} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for end
* @return A modified copy of the {@code this} object
*/
public final ImmutableRegion withEnd(int value) {
if (this.end == value) return this;
return new ImmutableRegion(this.entries, this.begin, value, this.isRemoved);
}
/**
* Copy the current immutable object by setting a value for the {@link Region#isRemoved() isRemoved} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isRemoved
* @return A modified copy of the {@code this} object
*/
public final ImmutableRegion withIsRemoved(boolean value) {
if (this.isRemoved == value) return this;
return new ImmutableRegion(this.entries, this.begin, this.end, value);
}
/**
* This instance is equal to all instances of {@code ImmutableRegion} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableRegion
&& equalTo((ImmutableRegion) another);
}
private boolean equalTo(ImmutableRegion another) {
return entries.equals(another.entries)
&& begin == another.begin
&& end == another.end;
}
/**
* Computes a hash code from attributes: {@code entries}, {@code begin}, {@code end}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + entries.hashCode();
h += (h << 5) + begin;
h += (h << 5) + end;
return h;
}
/**
* Construct a new immutable {@code Region} instance.
* @param entries The value for the {@code entries} attribute
* @return An immutable Region instance
*/
public static ImmutableRegion of(List entries) {
return of((Iterable) entries);
}
/**
* Construct a new immutable {@code Region} instance.
* @param entries The value for the {@code entries} attribute
* @return An immutable Region instance
*/
public static ImmutableRegion of(Iterable entries) {
return new ImmutableRegion(entries);
}
/**
* Creates an immutable copy of a {@link Region} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Region instance
*/
public static ImmutableRegion copyOf(Region instance) {
if (instance instanceof ImmutableRegion) {
return (ImmutableRegion) instance;
}
return ImmutableRegion.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableRegion ImmutableRegion}.
*
* ImmutableRegion.builder()
* .addEntries|addAllEntries(pl.poznan.put.structure.formats.BpSeq.Entry) // {@link Region#entries() entries} elements
* .begin(int) // optional {@link Region#begin() begin}
* .end(int) // optional {@link Region#end() end}
* .isRemoved(boolean) // optional {@link Region#isRemoved() isRemoved}
* .build();
*
* @return A new ImmutableRegion builder
*/
public static ImmutableRegion.Builder builder() {
return new ImmutableRegion.Builder();
}
/**
* Builds instances of type {@link ImmutableRegion ImmutableRegion}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "Region", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long OPT_BIT_BEGIN = 0x1L;
private static final long OPT_BIT_END = 0x2L;
private static final long OPT_BIT_IS_REMOVED = 0x4L;
private long optBits;
private List entries = new ArrayList();
private int begin;
private int end;
private boolean isRemoved;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Region} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(Region instance) {
Objects.requireNonNull(instance, "instance");
addAllEntries(instance.entries());
begin(instance.begin());
end(instance.end());
isRemoved(instance.isRemoved());
return this;
}
/**
* Adds one element to {@link Region#entries() entries} list.
* @param element A entries element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addEntries(BpSeq.Entry element) {
this.entries.add(Objects.requireNonNull(element, "entries element"));
return this;
}
/**
* Adds elements to {@link Region#entries() entries} list.
* @param elements An array of entries elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addEntries(BpSeq.Entry... elements) {
for (BpSeq.Entry element : elements) {
this.entries.add(Objects.requireNonNull(element, "entries element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link Region#entries() entries} list.
* @param elements An iterable of entries elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder entries(Iterable elements) {
this.entries.clear();
return addAllEntries(elements);
}
/**
* Adds elements to {@link Region#entries() entries} list.
* @param elements An iterable of entries elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllEntries(Iterable elements) {
for (BpSeq.Entry element : elements) {
this.entries.add(Objects.requireNonNull(element, "entries element"));
}
return this;
}
/**
* Initializes the value for the {@link Region#begin() begin} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link Region#begin() begin}.
* @param begin The value for begin
* @return {@code this} builder for use in a chained invocation
*/
public final Builder begin(int begin) {
this.begin = begin;
optBits |= OPT_BIT_BEGIN;
return this;
}
/**
* Initializes the value for the {@link Region#end() end} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link Region#end() end}.
* @param end The value for end
* @return {@code this} builder for use in a chained invocation
*/
public final Builder end(int end) {
this.end = end;
optBits |= OPT_BIT_END;
return this;
}
/**
* Initializes the value for the {@link Region#isRemoved() isRemoved} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link Region#isRemoved() isRemoved}.
* @param isRemoved The value for isRemoved
* @return {@code this} builder for use in a chained invocation
*/
public final Builder isRemoved(boolean isRemoved) {
this.isRemoved = isRemoved;
optBits |= OPT_BIT_IS_REMOVED;
return this;
}
/**
* Builds a new {@link ImmutableRegion ImmutableRegion}.
* @return An immutable instance of Region
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableRegion build() {
return new ImmutableRegion(this);
}
private boolean beginIsSet() {
return (optBits & OPT_BIT_BEGIN) != 0;
}
private boolean endIsSet() {
return (optBits & OPT_BIT_END) != 0;
}
private boolean isRemovedIsSet() {
return (optBits & OPT_BIT_IS_REMOVED) != 0;
}
}
private static List createSafeList(Iterable iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection) {
int size = ((Collection) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList) {
((ArrayList) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}