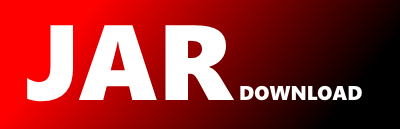
junitparams.internal.TestMethod Maven / Gradle / Ivy
package junitparams.internal;
import java.io.*;
import java.lang.annotation.*;
import java.lang.reflect.*;
import java.util.*;
import javax.lang.model.type.*;
import junitparams.*;
import junitparams.mappers.*;
import org.junit.*;
import org.junit.runner.*;
import org.junit.runners.model.*;
/**
* A wrapper for a test method
*
* @author Pawel Lipinski
*/
public class TestMethod {
private FrameworkMethod frameworkMethod;
private Class> testClass;
private Parameters parametersAnnotation;
private FileParameters fileParametersAnnotation;
private Object[] params;
public TestMethod(FrameworkMethod method, TestClass testClass) {
this.frameworkMethod = method;
this.testClass = testClass.getJavaClass();
this.parametersAnnotation = frameworkMethod.getAnnotation(Parameters.class);
this.fileParametersAnnotation = frameworkMethod.getAnnotation(FileParameters.class);
if (parametersAnnotation != null && fileParametersAnnotation != null) {
throw new IllegalArgumentException("Both @Parameters and @FileParameters exist on " + frameworkMethod.getName()
+ ". Remove one of them!");
}
}
public String name() {
return frameworkMethod.getName();
}
public static List listFrom(List annotatedMethods, TestClass testClass) {
List methods = new ArrayList();
for (FrameworkMethod frameworkMethod : annotatedMethods)
methods.add(new TestMethod(frameworkMethod, testClass));
return methods;
}
@Override
public int hashCode() {
return frameworkMethod.hashCode();
}
@Override
public boolean equals(Object obj) {
if (!(obj instanceof TestMethod))
return false;
if (!frameworkMethod.getName().equals(((TestMethod) obj).frameworkMethod.getName()))
return false;
if (!frameworkMethod.getMethod().getParameterTypes().equals(((TestMethod) obj).frameworkMethod.getMethod().getParameterTypes()))
return false;
return true;
}
Class> testClass() {
return testClass;
}
public boolean isIgnored() {
if (frameworkMethod.getAnnotation(Ignore.class) != null)
return true;
if (isParameterised() && parametersSets().length == 0)
return true;
return false;
}
public boolean isNotIgnored() {
return !isIgnored();
}
public Annotation[] annotations() {
return frameworkMethod.getAnnotations();
}
Description describe() {
if (isNotIgnored()) {
Description parametrised = Description.createSuiteDescription(name());
Object[] params = parametersSets();
for (int i = 0; i < params.length; i++) {
Object paramSet = params[i];
parametrised.addChild(
Description.createTestDescription(testClass(), Utils.stringify(paramSet, i) + " (" + name() + ")", annotations()));
}
return parametrised;
} else {
return Description.createTestDescription(testClass(), name(), annotations());
}
}
public Object[] parametersSets() {
if (params != null)
return params;
if (parametersAnnotation != null) {
params = paramsFromValue();
if (params.length == 0)
params = paramsFromSource();
if (params.length == 0)
params = paramsFromMethod(testClass());
}
else if (fileParametersAnnotation != null) {
params = paramsFromFile();
}
return params;
}
private Object[] paramsFromFile() {
try {
Reader reader = createProperReader();
DataMapper mapper = fileParametersAnnotation.mapper().newInstance();
try {
return mapper.map(reader);
} finally {
reader.close();
}
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException("Could not successfully read parameters from file: " + fileParametersAnnotation.value(), e);
}
}
private Reader createProperReader() throws IOException {
String filepath = fileParametersAnnotation.value();
if (filepath.indexOf(':') < 0)
return new FileReader(filepath);
String protocol = filepath.substring(0, filepath.indexOf(':'));
String filename = filepath.substring(filepath.indexOf(':') + 1);
if ("classpath".equals(protocol)) {
return new InputStreamReader(getClass().getClassLoader().getResourceAsStream(filename));
} else if ("file".equals(protocol)) {
return new FileReader(filename);
}
throw new IllegalArgumentException("Unknown file access protocol. Only 'file' and 'classpath' are supported!");
}
private Object[] paramsFromValue() {
return parametersAnnotation.value();
}
private Object[] paramsFromSource() {
if (sourceClassUndefined())
return new Object[] {};
Class> sourceClass = parametersAnnotation.source();
String method = parametersAnnotation.method();
if (method.isEmpty())
return fillResultWithAllParamProviderMethods(sourceClass);
else {
return paramsFromMethod(sourceClass);
}
}
private Object[] paramsFromMethod(Class> classWithMethod) {
String methodAnnotation = parametersAnnotation.method();
if (methodAnnotation.isEmpty())
return invokeMethodWithParams(defaultMethodName(), classWithMethod);
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy