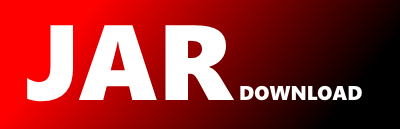
pl.rspective.voucherify.client.model.Customer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of voucherify-java-sdk Show documentation
Show all versions of voucherify-java-sdk Show documentation
Voucherify-java-sdk is a Java client (can be used in Android application as well) which was created to simplify integration with Voucherify backend (http://www.voucherify.io)
package pl.rspective.voucherify.client.model;
import com.google.gson.annotations.SerializedName;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
public class Customer {
private String id;
@SerializedName("source_id")
private String sourceId;
private String name;
private String email;
private String description;
@SerializedName("created_at")
private Date createdAt;
private Map metadata;
private String object;
public String getId() {
return id;
}
public String getSourceId() {
return sourceId;
}
public Customer setSourceId(String sourceId) {
this.sourceId = sourceId;
return this;
}
public String getName() {
return name;
}
public Customer setName(String name) {
this.name = name;
return this;
}
public String getEmail() {
return email;
}
public Customer setEmail(String email) {
this.email = email;
return this;
}
public String getDescription() {
return description;
}
public Customer setDescription(String description) {
this.description = description;
return this;
}
public Date getCreatedAt() {
return createdAt;
}
public Map getMetadata() {
return metadata;
}
public Customer setMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
public String getObject() {
return object;
}
public static class Builder {
private String id;
private String sourceId;
private String name;
private String email;
private String description;
private Map metadata;
public Builder setId(String id) {
this.id = id;
return this;
}
public Builder setSourceId(String sourceId) {
this.sourceId = sourceId;
return this;
}
public Builder setName(String name) {
this.name = name;
return this;
}
public Builder setEmail(String email) {
this.email = email;
return this;
}
public Builder setDescription(String description) {
this.description = description;
return this;
}
public Builder setMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
public Builder addMetadata(String key, Object value) {
if (metadata == null) {
metadata = new HashMap<>();
}
metadata.put(key, value);
return this;
}
public Customer build() {
Customer customer = new Customer();
customer.id = id;
customer.sourceId = sourceId;
customer.name = name;
customer.email = email;
customer.description = description;
customer.metadata = metadata;
customer.object = "customer";
return customer;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy